The Python API, along with the Jupyter Dashboard project enables Python developers to quickly build and prototype interactive web apps. This sample illustrates one such app which can be used to detect the changes in vegetation between the two dates. Increases in vegetation are shown in green, and decreases are shown in magenta.
This sample uses the fast on-the-fly processing power of raster functions available in the raster
module of the Python API.
To run this sample you need
jupyter_dashboards
package in your conda environment. You can install it as shown below. For information on this, refer to the install instructions.
conda install jupyter_dashboards -c conda-forge
This Dashboard uses the Geoenrichment API to alow users to pan around the world and explore the population of a selected AOI. Let's start by logging into ArcGIS Online and importing packages.
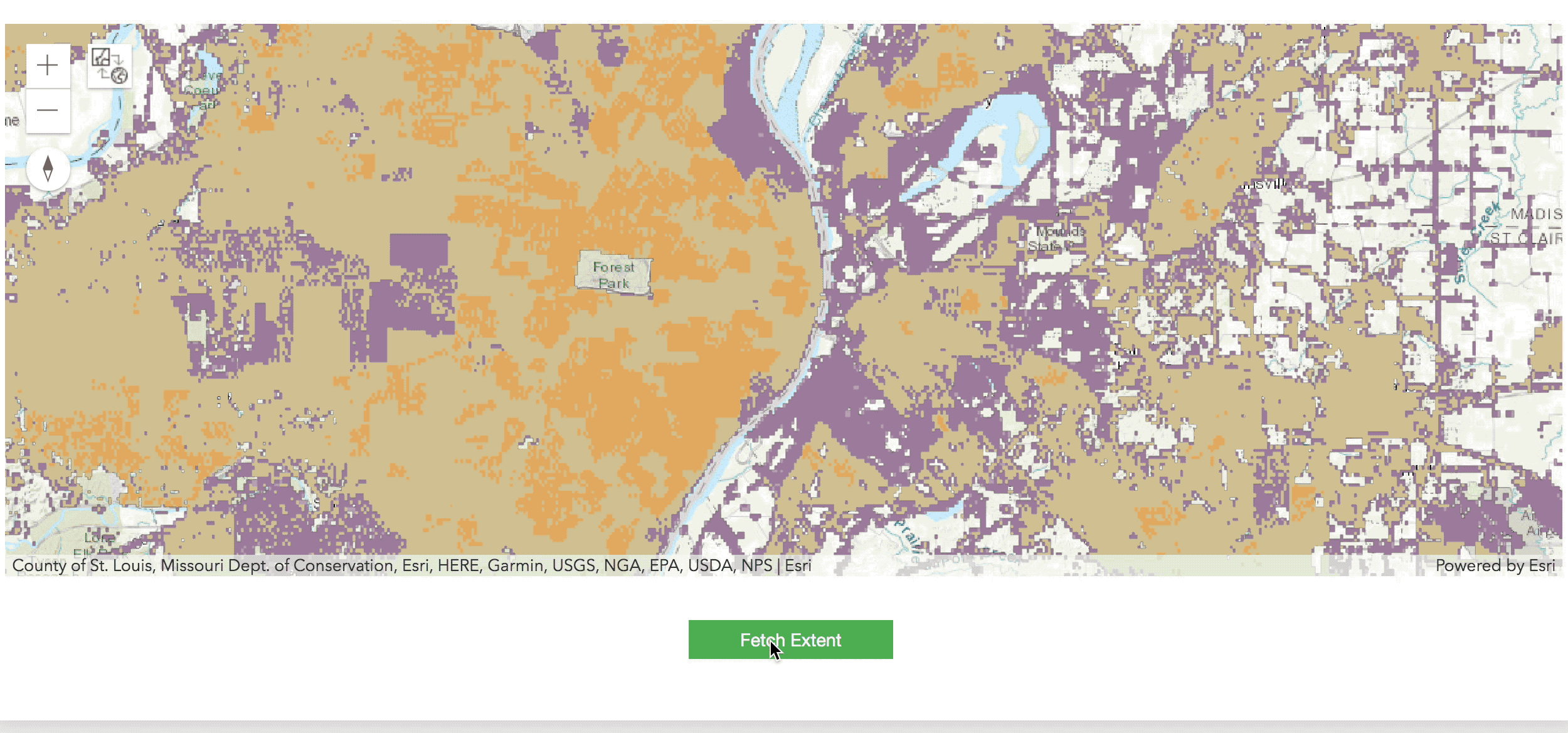
!conda install jupyter_dashboards -c conda-forge -y
from IPython.display import display, clear_output
import ipywidgets as widgets
from arcgis.geoenrichment import enrich
from arcgis.raster import ImageryLayer
from arcgis.geometry import Geometry
from arcgis.gis import GIS
gis = GIS(profile='your_online_profile')
img_svc ='https://worldpop.arcgis.com/arcgis/rest/services/WorldPop_Population_Density_100m/ImageServer'
img_lyr = ImageryLayer(img_svc, gis=gis)
%%html
<style>
.intro {
padding: 10px;
color: #202020;
font-family: 'Helvetica'
}
.map {
border: solid;
height: 450;
}
</style>
def running(button_object):
viz_map.clear_graphics()
the_geom = Geometry(viz_map.extent)
enriched = enrich([the_geom], gis=gis)
if 'TOTPOP' not in list(enriched):
enriched_pop = -1
else:
enriched_pop = enriched.TOTPOP[0]
print('the Geom: {}'.format(the_geom))
print('Sample Count: {}'.format(img_lyr.properties.maxImageHeight))
samples = img_lyr.get_samples(the_geom)
sample_sum = sum([float(sample['value']) for sample in samples])
print('GeoEnrichment Population Results: {}'.format(enriched_pop))
title = 'GeoEnriched Cell'
for feature in enriched.spatial.to_featureset():
# Expand Attributes With New Lines
content = "{}".format(
'<br/>'.join(['%s: %s' % (key.upper(), value) for (key, value) in feature.attributes.items()])
)
# Add Cell to Map
viz_map.draw(feature.geometry, popup={'title': title, 'content': content})
# Create & Display Map
viz_map = gis.map('St Louis')
viz_map.add_layer(img_lyr)
display(viz_map)
# Create Submit Button & Set on_click
run_btn = widgets.Button(
description='Fetch Extent',
button_style='success',
tooltip='Collect GeoEnrichment for Extent',
layout=widgets.Layout(justify_content='center', margin='0px 0px 0px 10px')
)
run_btn.on_click(running)
# Handle Widget Layout
params = widgets.HBox(
[run_btn],
layout=widgets.Layout(justify_content='center', margin='10px')
)
display(params)
the Geom: {'spatialReference': {'latestWkid': 3857, 'wkid': 102100}, 'xmin': -10116183.12480997, 'ymin': 4637924.2856790265, 'xmax': -9965755.053144686, 'ymax': 4699073.90830719} Sample Count: 4100 GeoEnrichment Population Results: -1