Learn how to find an address or place using a search box and the geocoding service.
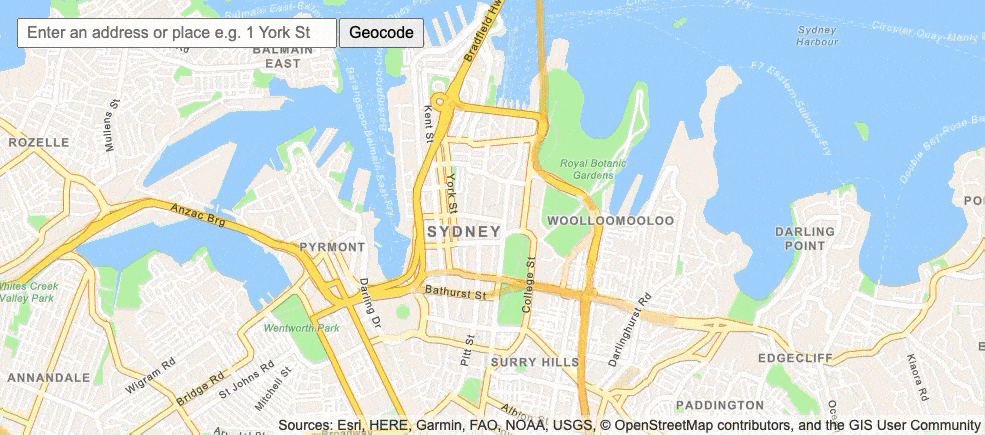
Find the location of addresses with the geocoding service.
Geocoding is the process of converting address or place text to a location. The geocoding service provides address and place geocoding and reverse geocoding.
In this tutorial, you use ArcGIS REST JS to access the geocoding service. You use a simple input control to accept text and a button to execute a search for an address or place. When an address or place is located, a pop-up will appear with the name, location, and address, and the view will pan to it.
Prerequisites
You need an ArcGIS Developer or ArcGIS Online account to access the developer dashboard and create an API key.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Set the API key
To access location services, you need an API key or OAuth 2.0 access token. To learn how to create and scope your key, visit the Create an API key tutorial.
-
Go to your dashboard to get an API key. The API key must be scoped to access the services used in this tutorial.
-
In CodePen, update
api
to use your key.Key Use dark colors for code blocks const apiKey = "YOUR_API_KEY"; const basemapEnum = "arcgis/streets"; const map = new maplibregl.Map({ container: "map", // the id of the div element style: `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapEnum}?token=${apiKey}`, zoom: 12, // starting zoom center: [-118.805, 34.027] // starting location [longitude, latitude] });
Add references to ArcGIS REST JS
This tutorial uses ArcGIS REST JS for geocoding.
- In the
<head>
element, add references to the ArcGIS REST JS library.
<head>
<meta charset="utf-8" />
<meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no" />
<script src=https://unpkg.com/maplibre-gl@3.2.1/dist/maplibre-gl.js></script>
<link href=https://unpkg.com/maplibre-gl@3.2.1/dist/maplibre-gl.css rel="stylesheet" />
<script src="https://unpkg.com/@esri/arcgis-rest-request@4.0.0/dist/bundled/request.umd.js"></script>
<script src="https://unpkg.com/@esri/arcgis-rest-geocoding@4.0.0/dist/bundled/geocoding.umd.js"></script>
Update the map
A navigation basemap layer is typically used in geocoding and routing applications. Update the basemap layer to use arcgis/navigation
.
-
Update the basemap and the map initialization to center on location
[151.2093, -33.8688]
, Sydney.Use dark colors for code blocks const apiKey = "YOUR_API_KEY"; const basemapEnum = "arcgis/navigation"; const map = new maplibregl.Map({ container: "map", style: `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapEnum}?token=${apiKey}`, zoom: 13, center: [151.2093, -33.8688] // Sydney });
Create geocoder controls
Use an HTML <input>
control to type an address into, and a <button>
control to initiate the query. Wrap them in a <div>
.
To add the <div>
element as a Map
control, you create an object that implements the MapLibre GL JS IControl interface.
-
Create a
Geocode
class with anControl o
function. Inside, create an Add <div>
element with an<input>
and a<button>
inside. You can usedocument.create
to create aElement template
with this HTML inside. Return this element.Use dark colors for code blocks const map = new maplibregl.Map({ container: "map", style: `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapEnum}?token=${apiKey}`, zoom: 13, center: [151.2093, -33.8688] // Sydney }); class GeocodeControl { onAdd(map) { const template = document.createElement("template"); template.innerHTML = ` <div id="geocode-container"> <input id="geocode-input" class="maplibregl-ctrl" type="text" placeholder="Enter an address or place e.g. 1 York St" size="50" /> <button id="geocode-button" class="maplibregl-ctrl">Geocode</button> </div> `; return template.content; } }
-
Add the
geocode
element to theControl top-left
of the view.Use dark colors for code blocks return template.content; } } const geocodeControl = new GeocodeControl(); map.addControl(geocodeControl, "top-left");
-
Style the input box and button to be the same height.
Use dark colors for code blocks <style> html, body, #map { padding: 0; margin: 0; height: 100%; width: 100%; font-family: Arial, Helvetica, sans-serif; font-size: 14px; color: #323232; } #geocode-container { display: inline-flex; margin: 20px; } #geocode-input, #geocode-button { font-size: 16px; margin: 0 2px 0 0; padding: 4px 8px; } #geocode-input { width: 300px; } </style>
Call the geocoding service
When you click the button, call arcgis
with the value of the search query. This uses the find
operation in the geocoding service.
For more control over geocoding, you can pass an IGeocode
instead of a simple string.
-
Attach a click event handler to the button.
Use dark colors for code blocks const geocodeControl = new GeocodeControl(); map.addControl(geocodeControl, "top-left"); document.getElementById("geocode-button").addEventListener("click", () => { });
-
Inside the click handler, create a new
arcgis
to access the geocoding service. CallRest.Api K e y Manager arcgis
with the user's search query. Set theRest.geocode params
to include the center of the map and setout
toFields *
to return all fields.Use dark colors for code blocks document.getElementById("geocode-button").addEventListener("click", () => { const query = document.getElementById("geocode-input").value; const authentication = arcgisRest.ApiKeyManager.fromKey(apiKey); arcgisRest .geocode({ singleLine: query, authentication, params: { location: map.getCenter().toArray().join(","), // center of map as longitude,latitude outFields: "*" // return all fields } }) });
Show the result
If the query is successful, the candidates
property will contain at least one value. To display the first candidate on the map, create a pop-up and add it to the map.
-
Add a response handler. Inside, check that
candidates
contains at least one value. Use thelocation
property to add a popup to the map and set the HTML of the popup toLong
to display the full address.Label To add a popup to the map, you use the
Popup
class in MapLibre GL JS. See the Display a pop-up tutorial for more information.Use dark colors for code blocks arcgisRest .geocode({ singleLine: query, authentication, params: { location: map.getCenter().toArray().join(","), // center of map as longitude,latitude outFields: "*" // return all fields } }) .then((response) => { const result = response.candidates[0]; if (!result) { alert("That query didn't match any geocoding results."); return; } const lngLat = [result.location.x, result.location.y]; new maplibregl.Popup().setLngLat(lngLat).setHTML(result.attributes.LongLabel).addTo(map); })
-
Use MapLibre GL JS's
pan
method to center the map at the result.T o This transitions the map smoothly from the current location to the result. To instantly reposition the map to that location, you could use
jump
instead.T o Use dark colors for code blocks new maplibregl.Popup().setLngLat(lngLat).setHTML(result.attributes.LongLabel).addTo(map); map.panTo(lngLat);
Handle errors
It is good practice to handle situations where there is a problem accessing the service. This could happen due to network disruption or a problem with your API key, for instance.
-
Check for issues accessing the geocoding service and alert the user.
Use dark colors for code blocks new maplibregl.Popup().setLngLat(lngLat).setHTML(result.attributes.LongLabel).addTo(map); map.panTo(lngLat); }) .catch((error) => { alert("There was a problem using the geocoder. See the console for details."); console.error(error); });
Run the app
In CodePen, run your code to display the map.
In the input box, type "1 King St." or "Starbucks" and then click the Geocode button to find it's location. If a location is found, the map will zoom to it and display a pop-up with the address.
What's next?
Learn how to use additional ArcGIS location services in these tutorials: