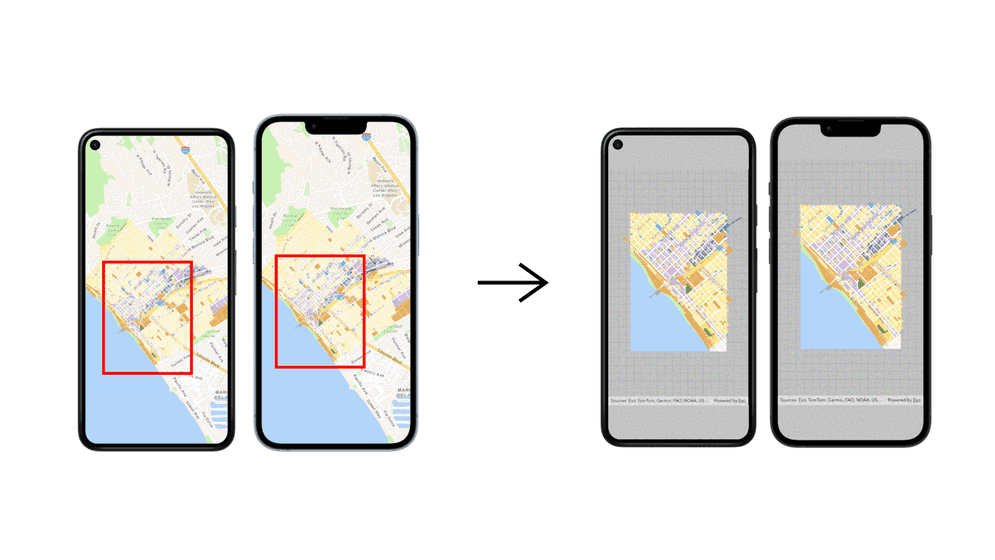
In this workflow, you will learn how to prepare a feature service and a web map, integrate the service into your web map, enable the map for offline use, and then use it to create a partially offline app. Later on, your app will request for an area within the map to download. This is also known as the on-demand method because you are defining which areas in the map to take offline on demand.
Prerequisites
You need an account for ArcGIS Online or ArcGIS Enterprise to host data for offline use and get an access token. If you need an account, go to Get started.
Steps
1. Prepare the map and data
Import data to create a feature service
For this workflow, you will use the Santa Monica Parcels dataset to create a hosted feature layer for your web map.
-
In your web browser, go to the Santa Monica Parcels item.
-
Click the Download button to download the zip file locally. Do not unzip this file.
-
Import the shapefile into ArcGIS using either a data management tool or a scripting API.
In your web browser, go to ArcGIS.com and sign in with your ArcGIS Online account.
-
In the top navigation bar, click Content.
-
Click New item. To upload the Santa Monica Parcels shapefile, you can either:
- Drag and drop the file.
- Or, click Your device and navigate to the file path.
-
Select Add Santa Monica Parcels.zip to publish the file as a hosted feature layer.
-
In Fields, leave all fields at their default settings and click Next.
-
In Location settings, leave the default settings and click Next.
-
Set the following information in the item details pane:
- Title:
Santa Monica Parcels
- Tags:
Santa Monica
Parcels
. - Summary:
Parcels in the Santa Monica Mountains.
- Title:
-
Click Next to create the new feature layer and feature service.
- Import the required libraries.
- Provide an access token.
- Create and publish a portal item.
- Handle the results.
Use dark colors for code blocks Copy # local path to shapefile zip file input_file_path = str( Path(__file__).parent / "Santa_Monica_Public_Parcels.zip" ) # add the zip file as an item to portal shp_item = portal.content.add( { "title": "Santa Monica Public Parcels", "description": "Santa Monica public parcels", "tags": "Santa, Monica, public, parcels", "type": "Shapefile", }, input_file_path, ) # publish the item to create a hosted featurelayer shp_service = shp_item.publish(None) print(f"New item id : {shp_service.id, }, url: {shp_service.layers[0].url}")
-
The feature layer will look something like this:
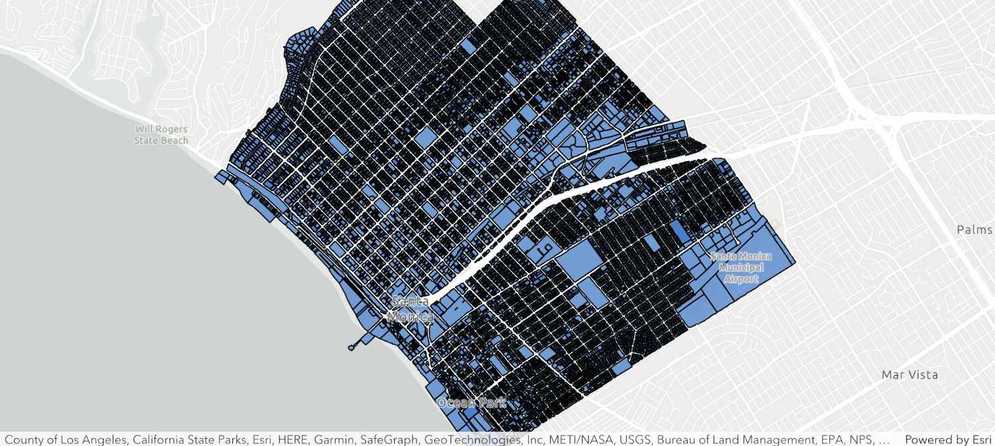
Set the feature styles
-
Style the parcels based on the
usetype
field.Use the Visualization tab in the item page in ArcGIS.com to style the Santa Monica parcels layer.
-
Go back to the item page > Visualization.
-
In the left panel, click the Layers and select the Santa Monica parcels layer. In the right panel, click Styles.
-
Click + Field, and add
usetype
. -
Under Pick a style, click Style options, update Types (unique symbols)
-
Click on the symbol next to each of the use type values to update the symbol color.
-
Set each of the
usetype
values to the following properties:- Fill Color:
- Residential:
#ffde3e
- Commercial:
#c29ed7
- Industrial:
#004c73
- Government:
#fc921f
- Institutional:
#149ece
- Recreational:
#267300
- Miscellaneous:
#b7814a
- Residential:
- Fill Color:
-
Click the X to exit out of Symbol style.
-
Click the pencil icon next to symbol style. Set the following properties for all the use types:
- Fill transparency:
30%
- Outline color:
#ffffff
- Outline transparency:
65%
- Outline width:
1
- Adjust width automatically: false
- Fill transparency:
-
Click the X to exit out of Symbol style. Then click Done twice.
-
Click Save to save the style in the feature layer item.
-
The styled layer will look something like this:
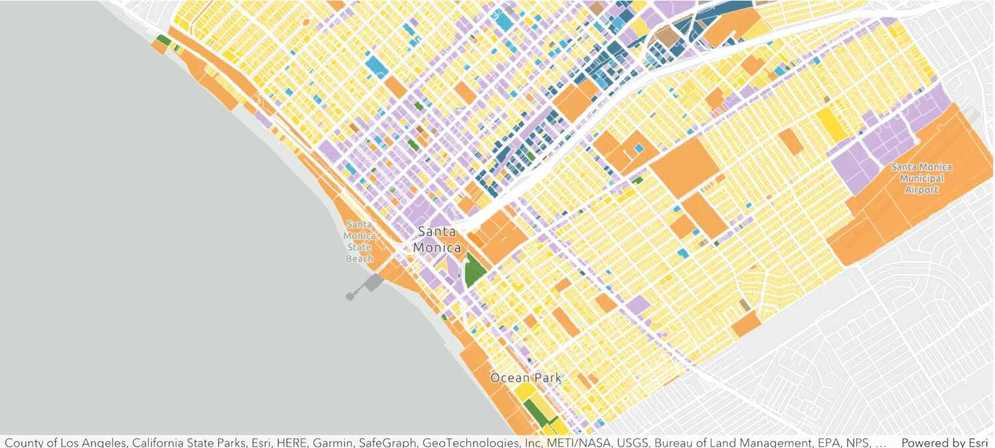
Manage service settings
In your portal, use the Settings tab to configure the service edit settings.
- In the item settings pane, scroll down to the Editing section.
- Click the Enable editing check box.
- Under the setting What kind of editing is allowed?, uncheck the Add and Delete privilege.
- This will only allow editors to update existing features.
- Under the Update privilege, click Attributes only.
- In our case, we only want editors to manage the feature attributes. They will be unable to remove or create new features.
- Click the Enable sync (required for offline use and collaboration) check box.
- Click Save.
Your edit settings should look like this:
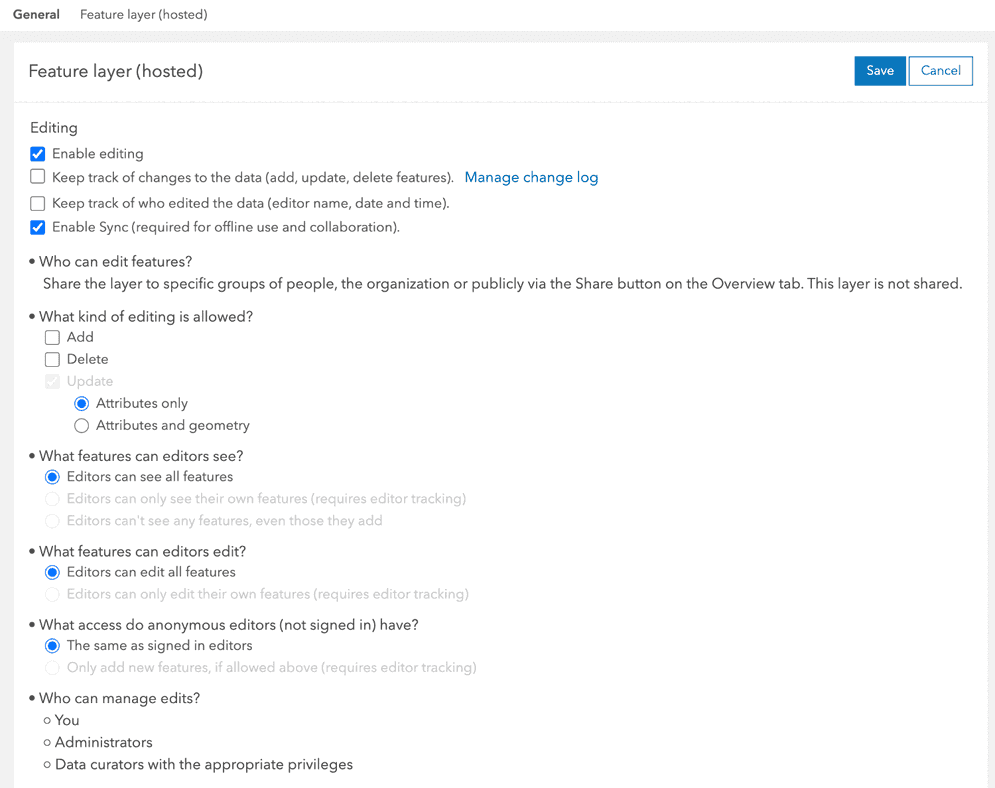
Create a web map with data layer
-
In the item page of your feature layer, go to the Overview tab in the top menu bar.
-
In the right panel, click Open in Map Viewer.
You should see a web map that looks something like this.
Save the web map
Now that you have a web map, you need to save it to your portal.
- In the left panel, click Save > Save as. At minimum, you need to provide a title and tags for your web map.
By default, your web map sharing level is set to Owner. To access the offline map in your application, you need an access token with the appropriate privileges.
Enable web map for offline use
-
In your web browser, find the item ID at the end of the URL for the web map you just saved.
- For example:
8ab76e9c5352400d87ca3315db9ba08e
- For example:
-
In a new browser tab, copy and paste the following URL and insert your item ID. This will return the item page for your web map, where you can configure it for offline use.
https
://www.arcgis.com/home/item.html?id= <ITEM _ID > - For example: https://www.arcgis.com/home/item.html?id=8ab76e9c5352400d87ca3315db9ba08e
-
Click Settings on the top bar of the item page.
-
Scroll down to the Offline menu.
-
If you don't see the Offline enabled icon at the top right corner, click Check Compatibility and turn on Offline.
-
Optionally, you can also manage advanced offline settings for your web map by clicking Options. These settings allow you to choose what data or updates mobile devices get and use a tile package for your offline basemap.
Under Features and attachments, you can configure download behaviors for both editable and read-only features. Adjusting how attachments and features are delivered can reduce the time it takes to synchronize edits, especially in areas of poor connectivity, as well as the amount of cellular data used.
Under Basemap and tile package, you can choose which basemap you want to use on your map. If you choose to use a local tile package, you will provide the filename of the package on your device. If you choose to use a tile package from your organization, you will browse a tile package from your ArcGIS organization.
-
Click Save. Your web map is enabled for offline use now.
2. Build the app
Set up offline app
-
Install and setup the ArcGIS Maps SDK for .NET on your development machine. Make sure you fulfill the SDK's system requirements.
-
Follow the Display a map tutorial or download the completed solution to get a starter code for this workflow.
-
Open the
.sln
file in Visual Studio.The Visual Studio solution, project, and the namespace for all classes currently use the name
Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.A Map -
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....A Map - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press F6).
Get an access token
By default, the sharing level of an item is set to Owner and requires an access token, such as an API key.
-
In your web browser, go to ArcGIS.com and sign in with your ArcGIS Online account.
- Click Content > My Content.
- Click the New Item > Developer credentials.
- In the Create developer credentials window, select API key credentials. Click Next.
- Set an expiration date for your API key. API keys can be valid for up to one year. Then, click Next.
- In the Privileges menu, make sure you have the following privileges:
- Admin privileges > Content > View all
- In the Grant item access menu, click Browse items.
- Select the web map and feature layer you just created. Then, click Next.
- Fill out the following properties:
- Title:
AP
I key - Offline app with a web map - Tags:
offline
,offline map
,web map
,workflow
- Summary: API key used to access Santa Monica Web Map in an offline app.
- Title:
- Click Next.
- Review your selections and, when you are ready, click Next.
- Click Generate the API key and go to item details page. I am ready to copy and save the key.
- Click Next.
- Copy the API key and store it safely. You will not be able to view it again later. If you lose it, you will have to generate another key.
-
Paste the access token into your code.
App.xaml.csUse dark colors for code blocks 28 29 30 31 32 33 34 35 36 38 39 40 41Change line public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); // Note: it is not best practice to store API keys in source code. // The API key is referenced here for the convenience of this example. Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
Download and display the offline map
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
Add additional required
using
statements at the top of the class.MapViewModel.csUse dark colors for code blocks 2 3 4 5 6 7 8 9 10 11 12Add line. Add line. Add line. Add line. Add line. Add line. using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices; using System.Threading.Tasks; using Esri.ArcGISRuntime.Portal; using Esri.ArcGISRuntime.Symbology; using Esri.ArcGISRuntime.Tasks.Offline; using Esri.ArcGISRuntime.UI; using System.Windows; using System.Diagnostics; using System.Drawing;
-
Get the web map item ID from your portal, for example
8ab76e9c5352400d87ca3315db9ba08e
. -
In MapViewModel.cs, modify the
Setup
function to update the web map's item ID to that of the Santa Monica Parcels.Map() The existing code creates a
Portal
using the item ID and aItem Portal
referencing ArcGIS Online. It sets theMap
property to a newView Model. Map Map
created using thePortal
.Item MapViewModel.csUse dark colors for code blocks 45 46 47 48 49 50 51 52 53 54 55 56 57 58private async Task SetupMap() { // Create a portal pointing to ArcGIS Online. ArcGISPortal portal = await ArcGISPortal.CreateAsync(); // Create a portal item for a specific web map id. string webMapId = "YOUR_ITEM_ID"; // Replace with your web map ID PortalItem mapItem = await PortalItem.CreateAsync(portal, webMapId); // Create the map from the item. Map map = new Map(mapItem); // Set the view model "Map" property. this.Map = map;
-
In the MapViewModel class, create a new property named
Graphics
. This will be a collection ofOverlays Graphics
to display a graphic of the area to take offline.Overlay Use dark colors for code blocks 34 35 36 37 38 39 40 41 42 43 44Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. private Map? _map; public Map? Map { get { return _map; } set { _map = value; OnPropertyChanged(); } } private GraphicsOverlayCollection? _graphicsOverlays; public GraphicsOverlayCollection? GraphicsOverlays { get { return _graphicsOverlays; } set { _graphicsOverlays = value; OnPropertyChanged(); } }
-
Modify the
Setup
function. Use anMap() Envelope
to create a newBuilder Envelope
that defines an area to take offline.MapViewModel.csUse dark colors for code blocks 68 69 70Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // Set the view model "Map" property. this.Map = map; // Define area of interest (envelope) to take offline. EnvelopeBuilder envelopeBldr = new EnvelopeBuilder(SpatialReferences.Wgs84) { XMin = -118.5064, XMax = -118.4800, YMin = 34.0094, YMax = 34.0259 }; Envelope offlineArea = envelopeBldr.ToGeometry();
-
Display a graphic of the area to take offline.
Use a
Simple
and aLine Symbol Simple
to display a newFill Symbol Graphic
of theoffline
with a red outline. Add the graphic to a newArea Graphics
and set theOverlay Map
property to display it in the map view.View Model. Graphics Overlays MapViewModel.csUse dark colors for code blocks 80 81Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Envelope offlineArea = envelopeBldr.ToGeometry(); // Create a graphic to display the area to take offline. SimpleLineSymbol lineSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, Color.Red, 2); SimpleFillSymbol fillSymbol = new SimpleFillSymbol(SimpleFillSymbolStyle.Solid, Color.Transparent, lineSymbol); Graphic offlineAreaGraphic = new Graphic(offlineArea, fillSymbol); // Create a graphics overlay and add the graphic. GraphicsOverlay areaOverlay = new GraphicsOverlay(); areaOverlay.Graphics.Add(offlineAreaGraphic); // Add the overlay to a new graphics overlay collection. GraphicsOverlayCollection overlays = new GraphicsOverlayCollection { areaOverlay }; // Set the view model's "GraphicsOverlays" property (will be consumed by the map view). this.GraphicsOverlays = overlays;
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml to open the file.
-
Use data binding to bind the
Graphics
property of theOverlays Map
to theView Model Map
control.View Data binding and the Model-View-ViewModel (MVVM) design pattern allow you to separate the logic in your app (the view model) from the presentation layer (the view).
MainWindow.xamlUse dark colors for code blocks 18 19 21Add line. <esri:MapView x:Name="MainMapView" Map="{Binding Map, Source={StaticResource MapViewModel}}" GraphicsOverlays="{Binding GraphicsOverlays, Source={StaticResource MapViewModel}}" />
-
Return to the MapViewModel.cs file and add code to the
Setup
function to create anMap Offline
that references the online map by calling the staticMap Task Offline
method.Map Task. Create Async MapViewModel.csUse dark colors for code blocks 97 98 99Add line. Add line. // Set the view model's "GraphicsOverlays" property (will be consumed by the map view). this.GraphicsOverlays = overlays; // Create an offline map task using the current map. OfflineMapTask offlineMapTask = await OfflineMapTask.CreateAsync(map);
-
Get default parameters to generate and download the offline map. Modify them to download a read-only offline map.
This tutorial does not involve editing and updating the contents of the offline map. When an offline map is editable, metadata is stored in ArcGIS to track and synchronize edits. Setting the
Generate
toOffline Map Update Mode No
avoids the overhead of maintaining this metadata in ArcGIS.Updates MapViewModel.csUse dark colors for code blocks // Create an offline map task using the current map. OfflineMapTask offlineMapTask = await OfflineMapTask.CreateAsync(map); // Create a default set of parameters for generating the offline map from the area of interest. GenerateOfflineMapParameters parameters = await offlineMapTask.CreateDefaultGenerateOfflineMapParametersAsync(offlineArea); parameters.UpdateMode = GenerateOfflineMapUpdateMode.NoUpdates;
-
Set a download location for the offline map.
MapViewModel.csUse dark colors for code blocks 103 104 105 106Add line. Add line. Add line. // Create a default set of parameters for generating the offline map from the area of interest. GenerateOfflineMapParameters parameters = await offlineMapTask.CreateDefaultGenerateOfflineMapParametersAsync(offlineArea); parameters.UpdateMode = GenerateOfflineMapUpdateMode.NoUpdates; // Build a folder path named with today's date/time in the "My Documents" folder. string documentsFolder = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments); string downloadDirectory = System.IO.Path.Combine(documentsFolder, "OfflineMap_" + DateTime.Now.ToFileTime().ToString());
This tutorial code creates a new unique folder in the documents folder using the current date and time.
-
Create a new
Generate
using theOffline Map Job parameters
anddownload
.Directory MapViewModel.csUse dark colors for code blocks 107 108 109 110Add line. // Build a folder path named with today's date/time in the "My Documents" folder. string documentsFolder = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments); string downloadDirectory = System.IO.Path.Combine(documentsFolder, "OfflineMap_" + DateTime.Now.ToFileTime().ToString()); GenerateOfflineMapJob generateJob = offlineMapTask.GenerateOfflineMap(parameters, downloadDirectory);
-
Create a function called
Generate
. This function will respond when the job completes or fails and will track the percent complete as the job runs. Add aJob _Progress Changed try/catch
block to handle exceptions and start by getting a reference to theGenerate
, passed in as theOffline Map Job sender
argument.MapViewModel.csUse dark colors for code blocks 107 108 109 110 111 112 113 114Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // Build a folder path named with today's date/time in the "My Documents" folder. string documentsFolder = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments); string downloadDirectory = System.IO.Path.Combine(documentsFolder, "OfflineMap_" + DateTime.Now.ToFileTime().ToString()); GenerateOfflineMapJob generateJob = offlineMapTask.GenerateOfflineMap(parameters, downloadDirectory); } private async void GenerateJob_ProgressChanged(object? sender, EventArgs e) { try { var generateJob = sender as GenerateOfflineMapJob; if(generateJob == null) { return; } } catch (Exception ex) { MessageBox.Show($"Error generating offline map: {ex.Message}"); } }
-
If the job succeeds, set the
Map
property with the offline map result. If it fails, notify the user. If the job is running, write the percent complete to the console.View Model. Map MapViewModel.csUse dark colors for code blocks 118 119 120 121 122 140 141 142 143 144 145Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. try { var generateJob = sender as GenerateOfflineMapJob; if(generateJob == null) { return; } // If the job succeeds, show the offline map in the map view. if (generateJob.Status == Esri.ArcGISRuntime.Tasks.JobStatus.Succeeded) { var result = await generateJob.GetResultAsync(); this.Map = result.OfflineMap; Debug.WriteLine("Generate offline map: Complete"); } // If the job fails, notify the user. else if (generateJob.Status == Esri.ArcGISRuntime.Tasks.JobStatus.Failed) { MessageBox.Show($"Unable to generate a map for that area: {generateJob?.Error?.Message}"); } else { int percentComplete = generateJob.Progress; Debug.WriteLine($"Generate offline map: {percentComplete}%"); } } catch (Exception ex) { MessageBox.Show($"Error generating offline map: {ex.Message}"); }
-
Return to your code in
Setup
and assign theMap Generate
function to handle theJob _Progress Changed Job.
event.Progress Changed() MapViewModel.csUse dark colors for code blocks 111 112Add line. GenerateOfflineMapJob generateJob = offlineMapTask.GenerateOfflineMap(parameters, downloadDirectory); generateJob.ProgressChanged += GenerateJob_ProgressChanged;
-
Start the
generate
by calling itsJob Start
method.MapViewModel.csUse dark colors for code blocks 111 112 113 114 116 117Add line. GenerateOfflineMapJob generateJob = offlineMapTask.GenerateOfflineMap(parameters, downloadDirectory); generateJob.ProgressChanged += GenerateJob_ProgressChanged; generateJob.Start(); }
-
Click Debug > Start Debugging (or press F5 on the keyboard) to run the app.
Access the offline map
-
Inside
Map
, create aView Model.cs private async
function returning aTask
calledAccess
.Map() MapViewModel.csUse dark colors for code blocks Copy 68 69 70 71private async Task AccessMap() { }
-
Inside the function, create a
Mobile
that references the path to the downloaded offline map area.Map Package MapViewModel.csUse dark colors for code blocks 68 69 70 72 73Add line. private async Task AccessMap() { var mobileMapPackage = await MobileMapPackage.OpenAsync(_downloadLocation); }
-
Load the mobile map package and read the
Map
from it.MapViewModel.csUse dark colors for code blocks 68 69 70 71 72 74 76 77Add line. Add line. private async Task AccessMap() { var mobileMapPackage = await MobileMapPackage.OpenAsync(_downloadLocation); await mobileMapPackage.LoadAsync(); this.Map = mobileMapPackage.Maps.First(); }
-
The function is now ready to be called to access a previously downloaded offline map area. You can trigger this function from a UI button click or by calling it directly from the constructor.
-
Click Debug > Start Debugging (or press F5 on the keyboard) to run the app.
Synchronize the offline map
-
Inside
Map
, create aView Model.cs private async
function returning aTask
calledSynchronize
.Map() MapViewModel.csUse dark colors for code blocks Copy 79 80 81 82private async Task SynchronizeMap() { }
-
Create an
Offline
referencing the offline map.Map Sync Task MapViewModel.csUse dark colors for code blocks 79 80 81 83 84Add line. private async Task SynchronizeMap() { OfflineMapSyncTask offlineMapSyncTask = await OfflineMapSyncTask.CreateAsync(Map); }
-
Use the
Offline
to get defaultMap Sync Task Offline
. Optionally modify these (for example, by specifying a synchronization direction).Map Sync Parameters MapViewModel.csUse dark colors for code blocks 79 80 81 82 83 85 86Add line. private async Task SynchronizeMap() { OfflineMapSyncTask offlineMapSyncTask = await OfflineMapSyncTask.CreateAsync(Map); OfflineMapSyncParameters syncParameters = await offlineMapSyncTask.CreateDefaultOfflineMapSyncParametersAsync(); }
-
Use the parameters to create an
Offline
.Map Sync Job MapViewModel.csUse dark colors for code blocks 79 80 81 82 83 84 85 87 88Add line. private async Task SynchronizeMap() { OfflineMapSyncTask offlineMapSyncTask = await OfflineMapSyncTask.CreateAsync(Map); OfflineMapSyncParameters syncParameters = await offlineMapSyncTask.CreateDefaultOfflineMapSyncParametersAsync(); OfflineMapSyncJob syncJob = offlineMapSyncTask.SyncOfflineMap(syncParameters); }
-
Start the
Offline
. If it completes without errors, the offline map and web map will be in sync.Map Sync Job MapViewModel.csUse dark colors for code blocks 79 80 81 82 83 84 85 86 87 89 90Add line. private async Task SynchronizeMap() { OfflineMapSyncTask offlineMapSyncTask = await OfflineMapSyncTask.CreateAsync(Map); OfflineMapSyncParameters syncParameters = await offlineMapSyncTask.CreateDefaultOfflineMapSyncParametersAsync(); OfflineMapSyncJob syncJob = offlineMapSyncTask.SyncOfflineMap(syncParameters); OfflineMapSyncResult syncResult = await syncJob.GetResultAsync(); }
-
The function is now ready to be called to synchronize an offline map. You can trigger this function from a UI button click or by calling it directly from the constructor.
-
Click Debug > Start Debugging (or press F5 on the keyboard) to run the app.