Learn how to create and display a map with a basemap layer.
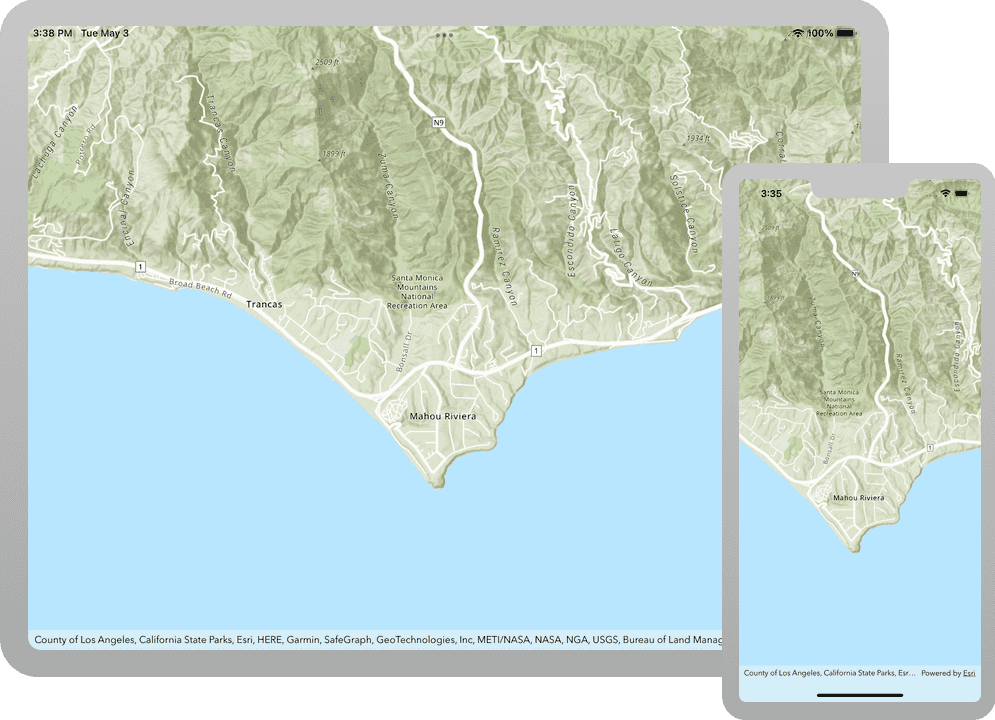
A map contains layers of geographic data. A map contains a basemap layer and, optionally, one or more data layers. You can display a specific area of a map by using a map view and setting the location and zoom level.
In this tutorial, you create and display a map of the Santa Monica Mountains in California using the topographic basemap layer.
The map and code will be used as the starting point for other 2D tutorials.
Prerequisites
The following are required for this tutorial:
- An ArcGIS account to access your API keys. If you don't have an account, sign up for free.
- Your system meets the system requirements.
Steps
Create a new Xcode project
Use Xcode to create an iOS app and configure it to reference the API.
-
Open Xcode. In the menu bar, click File > New > Project > iOS > App > Next.
- In the Choose options window, set the following properties:
- Product Name:
<your app name>
- Language: Swift
- Interface: SwiftUI
- Organization Identifier:
<your organization>
- Product Name:
- Uncheck all other options.
- Click Next.
- Choose a location to store your project. Click Create.
- In the Choose options window, set the following properties:
-
In the Project Navigator, click
<your app name>
. In the Editor, right click on the struct name,App <your app name>
. Select Refactor then Rename... to rename the struct toApp Main
. Click the Rename button in the top right to confirm the new name. This will rename the struct and file in all affected areas. This file and struct will be namedApp Main
for all tutorials here on out.App -
Add a reference to the API using Swift Package Manager.
Add a map
Create a map that contains a topographic basemap layer. The map will be centered on the Santa Monica Mountains in California.
-
In the Project Navigator, click ContentView.swift.
-
In the Editor, add an
import
statement to reference the API.ContentView.swiftUse dark colors for code blocks Add line. import SwiftUI import ArcGIS
-
Add a
@State
property wrapper namedmap
of typeMap
with a default value. Create aMap
with anarc
basemap style and return it.GISTopographic ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. struct ContentView: View { @State private var map = { let map = Map(basemapStyle: .arcGISTopographic) return map }() }
A
GeoModel
like aMap
orScene
can be expensive to create and holds state. To ensure that a geo model and other model objects are only created as needed, you can apply the@State
or@State
property wrapper to them, as in the above code. See Managing model data in your app for more information.Object -
Set the map's
initial
property with aViewpoint Viewpoint
using the Santa Monica Mountains coordinates.ContentView.swiftUse dark colors for code blocks Add line. struct ContentView: View { @State private var map = { let map = Map(basemapStyle: .arcGISTopographic) map.initialViewpoint = Viewpoint(latitude: 34.02700, longitude: -118.80500, scale: 72_000) return map }() }
Add a map view
A map view is a UI component that displays a map. It also handles user interactions with the map, including navigating with touch gestures. Use Xcode and SwiftUI to add a map view.
-
In the body, create a
MapView
with the map created in the previous step.ContentView.swiftUse dark colors for code blocks Add line. Add line. struct ContentView: View { @State private var map = { let map = Map(basemapStyle: .arcGISTopographic) map.initialViewpoint = Viewpoint(latitude: 34.02700, longitude: -118.80500, scale: 72_000) return map }() var body: some View { // Displays the map. MapView(map: map) } }
-
In the Project Navigator, click MainApp.swift.
-
In the body, add an
.ignores
modifier to theSafe Area() Content
. TheView Content
's body contains theView Map
and this modifier ensures that the map view is displayed beyond the safe area to all edges.View MainApp.swiftUse dark colors for code blocks Add line. var body: some SwiftUI.Scene { WindowGroup { ContentView() .ignoresSafeArea() } }
Set your API key
An API key is required to enable access to services, web maps, and web scenes hosted in ArcGIS Online.
If you haven't already, go to your developer dashboard to get your API key. For these tutorials, use your default API key. It is scoped to include all of the services demonstrated in the tutorials.
-
In the Project Navigator, click MainApp.swift.
-
In the Editor, add an
import
statement to reference the API.MainApp.swiftUse dark colors for code blocks Add line. import SwiftUI import ArcGIS
-
Implement an initializer in the
Display
struct. Set theAMap ArcGISEnvironment.apiKey
property on theArcGISEnvironment
with your API Key.MainApp.swiftUse dark colors for code blocks Add line. Add line. Add line. import SwiftUI import ArcGIS @main struct MainApp: App { init() { ArcGISEnvironment.apiKey = APIKey("<#your-API-key#>") } var body: some SwiftUI.Scene { WindowGroup { ContentView() .ignoresSafeArea() } } }
-
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Monterey 12.5, Xcode 15, iOS 17. If you are using a physical device, then refer to the system requirements.
You should see a map with the topographic basemap layer centered on the Santa Monica Mountains in California. Pinch, drag, and double-tap the map view to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: