Learn how to display the current device location on a map or scene.
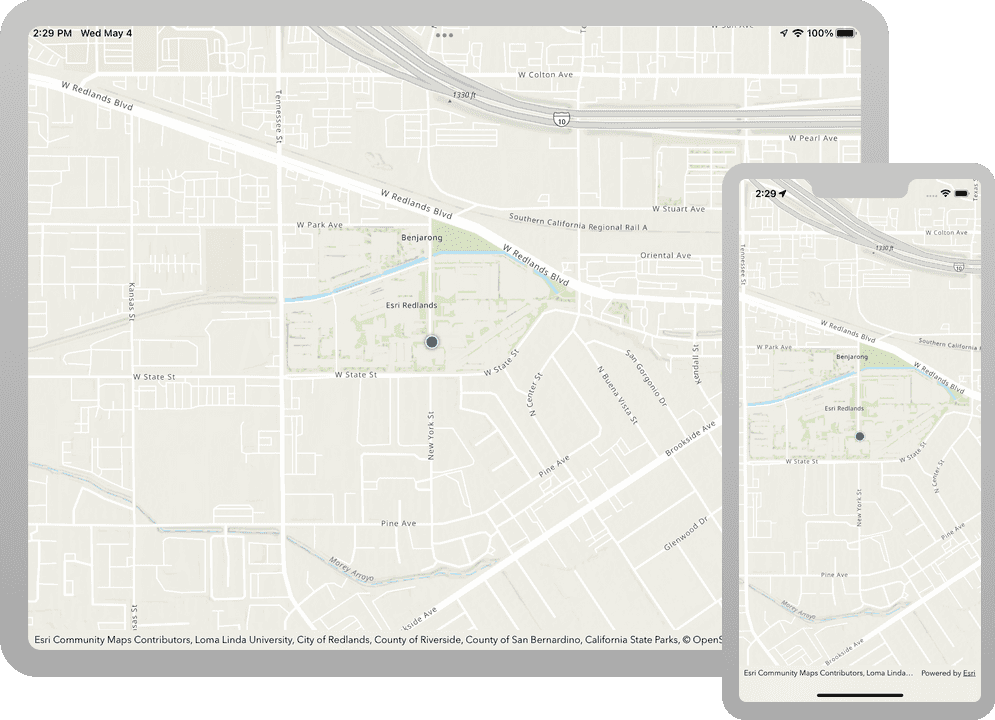
You can display the device location on a map or scene. This is important for workflows that require the user's current location, such as finding nearby businesses, navigating from the current location, or identifying and collecting geospatial information.
By default, location display uses the device's location provider. Your app can also process input from other location providers, such as an external GPS receiver or a provider that returns a simulated location. For more information, see the Show device location topic.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
Steps
Open the Xcode project
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.xcodeproj
file in Xcode. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Xcode, in the Project Navigator, click MainApp.swift.
-
In the Editor, set the
ArcGISEnvironment.apiKey
property on theArcGISEnvironment
with your access token.MainApp.swiftUse dark colors for code blocks init() { ArcGISEnvironment.apiKey = APIKey("<#YOUR-ACCESS-TOKEN#>") }
-
Add a custom iOS target property
The app must provide a message that tells the user why the app is requesting access to the user's location when the app is in use.
-
In Xcode, in the Project navigator, select the target app.
-
Click the Info tab and add the "Privacy - Location When In Use Usage Description" key under Custom iOS Target Properties.
-
Add a string value describing how the app would use the device location. For example, "Your location is used to show your position on the map."
To learn more about requesting user authorization, see the Note in the Location data sources section of the Device location guide topic.
Import Core Location
-
In Xcode, in the Project Navigator, click ContentView.swift.
-
In the Editor, import the CoreLocation framework. This is required to request the user's location.
ContentView.swiftUse dark colors for code blocks import ArcGIS import CoreLocation
Initialize the map
-
The map will zoom to the extent of the current location, so you don't need to set the viewpoint anymore. Remove the map
State
and replace it with just a map initializer.Object ContentView.swiftUse dark colors for code blocks struct ContentView: View { private let map = Map(basemapStyle: .arcGISTopographic)
Add location display to map view
-
Create the location display using the system location data source.
ContentView.swiftUse dark colors for code blocks struct ContentView: View { private let map = Map(basemapStyle: .arcGISTopographic) private let locationDisplay = LocationDisplay(dataSource: SystemLocationDataSource())
-
Add the location display modifier to the map view.
ContentView.swiftUse dark colors for code blocks var body: some View { MapView(map: map) .locationDisplay(locationDisplay) } }
Request user authorization to use location
The app must request authorization from the user to access the device location. The user can grant or deny the request.
-
In the task modifier, create a
CLLocation
.Manager -
If the authorization status is not determined, request "in use authorization".
ContentView.swiftUse dark colors for code blocks var body: some View { MapView(map: map) .locationDisplay(locationDisplay) .task { let locationManager = CLLocationManager() if locationManager.authorizationStatus == .notDetermined { locationManager.requestWhenInUseAuthorization() } } }
Show the current location
A map view provides LocationDisplay
for showing the current location of the device. The location symbol is displayed on top of all content in the map view.
Instances of this class manage the display of device location on a map view: the symbols, animation, auto pan behavior, and so on. Location display is an overlay of the map view, and displays above everything else, including graphics overlays.
The location display does not retrieve location information, that is the job of the associated data source, which provides location updates on a regular basis. In addition to the default system location data source, you can use location providers based on external GPS devices or a simulated location source.
Each map view has its own instance of a location display and instances of location display and location data source are not shared by multiple map views. This allows you to start and stop location display independently on multiple map views without affecting each other.
-
Start displaying the location by calling the data source's
start()
method.ContentView.swiftUse dark colors for code blocks var body: some View { MapView(map: map) .locationDisplay(locationDisplay) .task { let locationManager = CLLocationManager() if locationManager.authorizationStatus == .notDetermined { locationManager.requestWhenInUseAuthorization() } do { try await locationDisplay.dataSource.start() } catch { } } }
-
Set the initial zoom scale and the auto pan mode.
ContentView.swiftUse dark colors for code blocks var body: some View { MapView(map: map) .locationDisplay(locationDisplay) .task { let locationManager = CLLocationManager() if locationManager.authorizationStatus == .notDetermined { locationManager.requestWhenInUseAuthorization() } do { try await locationDisplay.dataSource.start() locationDisplay.initialZoomScale = 40_000 locationDisplay.autoPanMode = .recenter } catch { } } }
Error handling
-
Add a
Bool
calledfailed
.To Start ContentView.swiftUse dark colors for code blocks struct ContentView: View { private let map = Map(basemapStyle: .arcGISTopographic) private let locationDisplay = LocationDisplay(dataSource: SystemLocationDataSource()) @State private var failedToStart = false
-
In the catch closure, set the
failed
boolean to true. If the location display fails to start, the value will be changed.To Start ContentView.swiftUse dark colors for code blocks do { try await locationDisplay.dataSource.start() locationDisplay.initialZoomScale = 40_000 locationDisplay.autoPanMode = .recenter } catch { self.failedToStart = true }
-
Present an alert if the location display failed to start.
ContentView.swiftUse dark colors for code blocks var body: some View { MapView(map: map) .locationDisplay(locationDisplay) .task { let locationManager = CLLocationManager() if locationManager.authorizationStatus == .notDetermined { locationManager.requestWhenInUseAuthorization() } do { try await locationDisplay.dataSource.start() locationDisplay.initialZoomScale = 40_000 locationDisplay.autoPanMode = .recenter } catch { self.failedToStart = true } } .alert("Location display failed to start", isPresented: $failedToStart) {} }
Run the app
-
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Monterey 12.5, Xcode 15, iOS 17. If you are using a physical device, then refer to the system requirements.
-
When the app runs, you'll see the system prompt requesting to use the device location. Tap to either Allow once or While using the app.
-
If the app is running on a simulator, you'll have to provide a simulated location. Go to the Features menu of the Simulator. Under Location, choose a predefined option or enter a custom Location.
Your map should now show the device's location, either simulated or actual.