Learn how to execute a SQL query to return features from a feature layer based on spatial and attribute criteria.
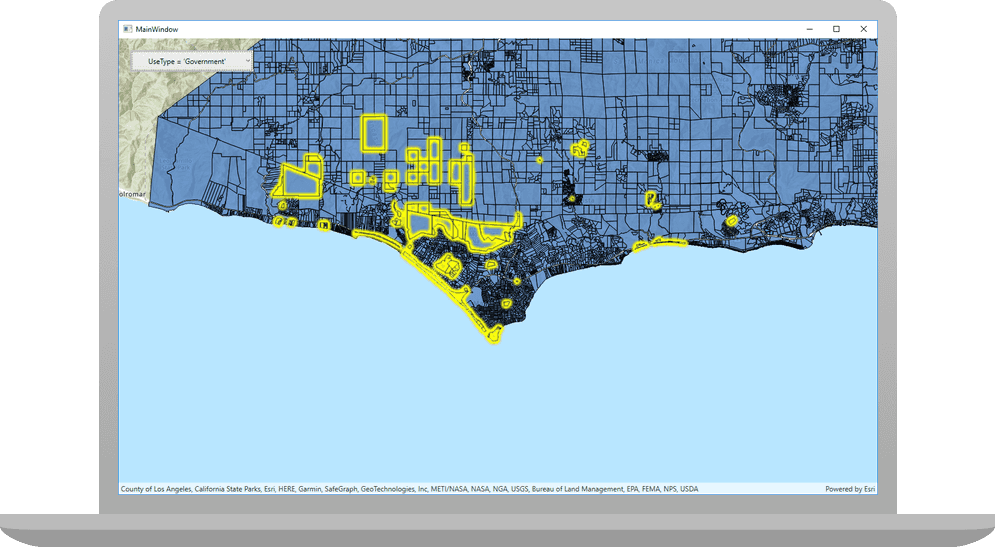
A feature layer can contain a large number of features stored in ArcGIS. You can query a layer to access a subset of its features using any combination of spatial and attribute criteria. You can control whether or not each feature's geometry is returned, as well as which attributes are included in the results. Queries allow you to return a well-defined subset of your hosted data for analysis or display in your app.
In this tutorial, you'll write code to perform SQL queries that return a subset of features in the LA County Parcel feature layer (containing over 2.4 million features). Features that meet the query criteria are selected in the map.
Prerequisites
The following are required for this tutorial:
- An ArcGIS account to access your API keys. If you don't have an account, sign up for free.
- Your system meets the system requirements.
Steps
Open the Xcode project
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.xcodeproj
file in Xcode. -
If you downloaded the solution project, set your API key.
An API Key enables access to services, web maps, and web scenes hosted in ArcGIS Online.
-
Go to your developer dashboard to get your API key. For these tutorials, use your default API key. It is scoped to include all of the services demonstrated in the tutorials.
- In Xcode, in the Project Navigator, click MainApp.swift.
- In the Editor, set the
ArcGISEnvironment.apiKey
property on theArcGISEnvironment
with your API key.
MainApp.swiftUse dark colors for code blocks import SwiftUI import ArcGIS @main struct MainApp: App { init() { ArcGISEnvironment.apiKey = APIKey("<#your-API-key#>") } var body: some SwiftUI.Scene { WindowGroup { ContentView() .ignoresSafeArea() } } }
-
Update the map
-
In Xcode, in the Project Navigator, click ContentView.swift.
-
In the editor, modify the
map
variable's initial viewpoint.ContentView.swiftUse dark colors for code blocks Change line let map: Map = { let map = Map(basemapStyle: .arcGISTopographic) map.initialViewpoint = Viewpoint(latitude: 34.03000, longitude: -118.80500, scale: 6e4) return map }()
-
Create a private extension named
Model
with a class of typeObservable
. Move the map variable there.Object ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. private extension ContentView { class Model: ObservableObject { let map: Map = { let map = Map(basemapStyle: .arcGISTopographic) map.initialViewpoint = Viewpoint(latitude: 34.03000, longitude: -118.80500, scale: 6e4) return map }() } }
-
Create a
ServiceFeatureTable
from the parcels service URL, then use it to make aFeatureLayer
. Then, add the parcels feature layer to the map as an operational layer.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. class Model: ObservableObject { let map: Map = { let map = Map(basemapStyle: .arcGISTopographic) map.initialViewpoint = Viewpoint(latitude: 34.03000, longitude: -118.80500, scale: 6e4) let parcelsURL = URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/LA_County_Parcels/FeatureServer/0")! let featureTable = ServiceFeatureTable(url: parcelsURL) let featureLayer = FeatureLayer(featureTable: featureTable) map.addOperationalLayer(featureLayer) return map }() }
-
To access the model's map in the
Content
struct, create a private variable of typeView Model
with a@State
property wrapper and modify theObject Map
to display the model's map.View ContentView.swiftUse dark colors for code blocks Add line. Change line struct ContentView: View { @StateObject private var model = Model() @State private var geoViewExtent: Envelope? var body: some View { MapView(map: model.map) .onVisibleAreaChanged { newVisibleArea in geoViewExtent = newVisibleArea.extent } } }
Make an enumeration for predefined where clauses
To allow the user to query the parcels feature layer, create an enum
that contains a set of predefined where clauses.
-
In the
Content
extension, make anView enum
type calledWhere
that isClause Case
andIterable Equatable
. Add a switch statement and a case option for each where clause.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. private extension ContentView { enum WhereClause: CaseIterable, Equatable { case government, residential, farm, taxRate10853, taxRate10860, landValueGreaterThan, landValueLessThan var expression: String { switch self { case .government: return "UseType = 'Government'" case .residential: return "UseType = 'Residential'" case .farm: return "UseType = 'Irrigated Farm'" case .taxRate10853: return "TaxRateArea = 10853" case .taxRate10860: return "TaxRateArea = 10860" case .landValueGreaterThan: return "Roll_LandValue > 1000000" case .landValueLessThan: return "Roll_LandValue < 1000000" } } } class Model: ObservableObject { let map: Map = { let map = Map(basemapStyle: .arcGISTopographic) map.initialViewpoint = Viewpoint(latitude: 34.03000, longitude: -118.80500, scale: 6e4) let parcelsURL = URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/LA_County_Parcels/FeatureServer/0")! let featureTable = ServiceFeatureTable(url: parcelsURL) let featureLayer = FeatureLayer(featureTable: featureTable) map.addOperationalLayer(featureLayer) return map }() } }
Create a function to query the parcels layer and select the result
Create a new function that queries the parcels Feature
with the chosen where clause and current extent. Clear any currently selected features and create a new Query
object. Set the selected
property to the option selected by the user and geo
to the current visible area. Asynchronously, query the feature table using the Query
and select the features returned in the Feature
.
-
Create a private variable of type
Envelope
with the@State
property wrapper namedgeo
. This variable keeps track of the current, visible extent.View Extent ContentView.swiftUse dark colors for code blocks Add line. struct ContentView: View { @StateObject private var model = Model() @State private var geoViewExtent: Envelope? var body: some View { MapView(map: model.map) } }
-
To update the
geo
variable when the visible area is changed, add theView Extent o
task modifer to the map view. When the visible area has changed, updaten Visible Area Changed(perform: ) geo
to the new area'sView Extent extent
.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. struct ContentView: View { @StateObject private var model = Model() @State private var geoViewExtent: Envelope? var body: some View { MapView(map: model.map) .onVisibleAreaChanged { newVisibleArea in geoViewExtent = newVisibleArea.extent } } }
-
Create a new method called
query
in theFeature Layer(selected Where Clause: extent: ) Model
. Specify that it accepts parameters of typeWhere
andClause Envelope
.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. class Model: ObservableObject { let map: Map = { let map = Map(basemapStyle: .arcGISTopographic) map.initialViewpoint = Viewpoint(latitude: 34.03000, longitude: -118.80500, scale: 6e4) let parcelsURL = URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/LA_County_Parcels/FeatureServer/0")! let featureTable = ServiceFeatureTable(url: parcelsURL) let featureLayer = FeatureLayer(featureTable: featureTable) map.addOperationalLayer(featureLayer) return map }() func queryFeatureLayer(with selectedWhereClause: WhereClause, in extent: Envelope) async { guard let parcelsFeatureLayer = map.operationalLayers.first as? FeatureLayer, let featureTable = parcelsFeatureLayer.featureTable else { return } parcelsFeatureLayer.clearSelection() let parameters = QueryParameters() parameters.whereClause = selectedWhereClause.expression parameters.geometry = extent do { let result = try await featureTable.queryFeatures(using: parameters) let features = result.features() parcelsFeatureLayer.selectFeatures(features) } catch { print(error) } } }
Add UI for selecting a predefined where clause
To allow the user to choose a where clause that queries the parcels dataset, add a Picker that presents the list of predefined where clauses.
-
In the
Content
, create aView @State
variable namedselected
of typeWhere Clause Where
. AssignClause .government
as the default enum. The@State
variableselected
allows the model'sWhere Clause query
method to track which where clause the user has selected.Feature Layer(selected Where Clause: extent: ) ContentView.swiftUse dark colors for code blocks Add line. struct ContentView: View { @StateObject private var model = Model() @State private var geoViewExtent: Envelope? @State private var selectedWhereClause: WhereClause = .government var body: some View { MapView(map: model.map) .onVisibleAreaChanged { newVisibleArea in geoViewExtent = newVisibleArea.extent } } }
-
In the body, add a
Toolbar
and aToolbar
withItem Group .bottom
placement to theBar Map
.View ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. var body: some View { MapView(map: model.map) .onVisibleAreaChanged { newVisibleArea in geoViewExtent = newVisibleArea.extent } .toolbar { ToolbarItemGroup(placement: .bottomBar) { } } }
-
Add a
Picker
to the toolbar item group and label it, "Choose an SQLwhere
clause". Set the selection to$selected
. Iterating throughWhere Clause .all
of theCases Where
to populate the Picker with all theClause Where
enums.Clause ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. var body: some View { MapView(map: model.map) .onVisibleAreaChanged { newVisibleArea in geoViewExtent = newVisibleArea.extent } .toolbar { ToolbarItemGroup(placement: .bottomBar) { Spacer() Picker("Choose an SQL `where` clause", selection: $selectedWhereClause) { ForEach(WhereClause.allCases, id: \.self) { clause in Text(clause.expression) } } Spacer() } } }
-
Lastly, add a
.task
modifier that calls the model'squery
function using theFeature Layer(selected Where Clause: extent: ) selected
property.Where Clause ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. var body: some View { MapView(map: model.map) .onVisibleAreaChanged { newVisibleArea in geoViewExtent = newVisibleArea.extent } .toolbar { ToolbarItemGroup(placement: .bottomBar) { Spacer() Picker("Choose an SQL `where` clause", selection: $selectedWhereClause) { ForEach(WhereClause.allCases, id: \.self) { clause in Text(clause.expression) } .task(id: selectedWhereClause) { guard let geoViewExtent = geoViewExtent else { return } await model.queryFeatureLayer(with: selectedWhereClause, in: geoViewExtent) } } Spacer() } } }
Run the app
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Monterey 12.5, Xcode 15, iOS 17. If you are using a physical device, then refer to the system requirements.
The app loads with the map centered on the Santa Monica Mountains in California with the parcels feature layer displayed. Choose an attribute expression and to display parcels that meet the selected criteria.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: