Learn how to find a route and directions with the route service.
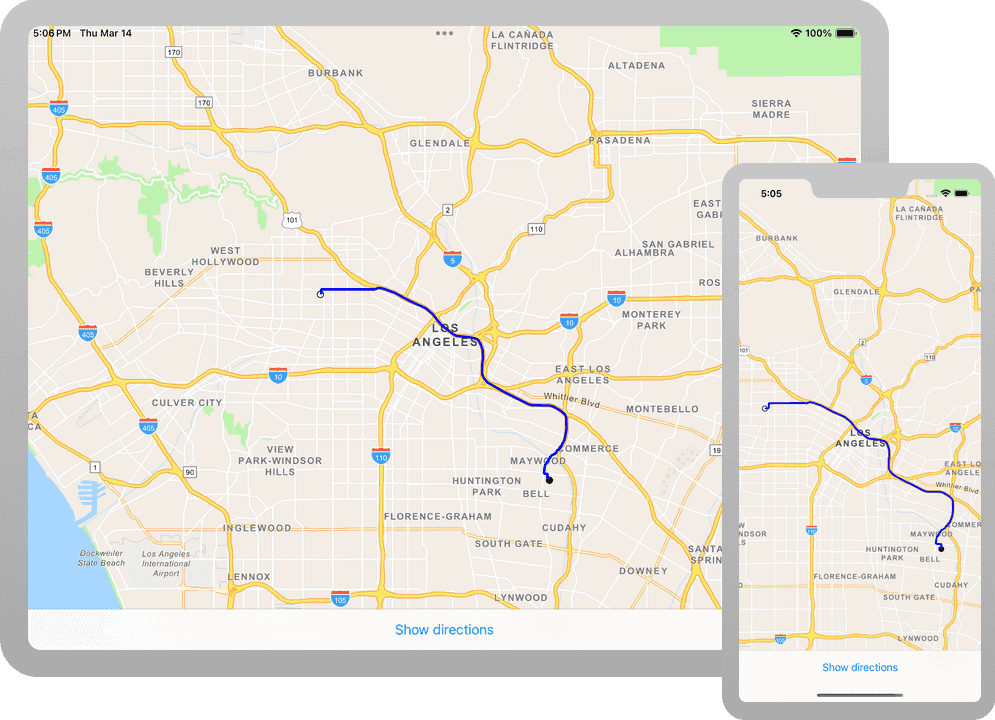
Routing is the process of finding the path from an origin to a destination in a street network. You can use the Routing service to find routes, get driving directions, calculate drive times, and solve complicated, multiple vehicle routing problems. To create a route, you typically define a set of stops (origin and one or more destinations) and use the service to find a route with directions. You can also use a number of additional parameters such as barriers and mode of travel to refine the results.
In this tutorial, you define an origin and destination by clicking on the map. These values are used to get a route and directions from the route service. The directions are also displayed on the map.
Prerequisites
The following are required for this tutorial:
- An ArcGIS account to access your API keys. If you don't have an account, sign up for free.
- Your system meets the system requirements.
Steps
Open the Xcode project
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.xcodeproj
file in Xcode. -
If you downloaded the solution project, set your API key.
An API Key enables access to services, web maps, and web scenes hosted in ArcGIS Online.
-
Go to your developer dashboard to get your API key. For these tutorials, use your default API key. It is scoped to include all of the services demonstrated in the tutorials.
- In Xcode, in the Project Navigator, click MainApp.swift.
- In the Editor, set the
ArcGISEnvironment.apiKey
property on theArcGISEnvironment
with your API key.
MainApp.swiftUse dark colors for code blocks import SwiftUI import ArcGIS @main struct MainApp: App { init() { ArcGISEnvironment.apiKey = APIKey("<#your-API-key#>") } var body: some SwiftUI.Scene { WindowGroup { ContentView() .ignoresSafeArea() } } }
-
Update the map
A navigation basemap layer is typically used in routing applications. Update the basemap to use the .arc
basemap style, and change the position of the map to center on Los Angeles.
-
Update the
Basemap
style property from.arc
toGISTopographic .arc
and update the latitude and longitude coordinates to center on Los Angeles.GISNavigation ContentView.swiftUse dark colors for code blocks Change line Change line struct ContentView: View { @State var map = { let map = Map(basemapStyle: .arcGISNavigation) map.initialViewpoint = Viewpoint(latitude: 34.05293, longitude: -118.24368, scale: 2e5) return map }()
-
Create a private class named
Model
of typeObservable
and add aObject @State
variable of theObject Model
to theContent
. See the programming patterns page for more information on how to manage states.View ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. import SwiftUI import ArcGIS class Model: ObservableObject { } struct ContentView: View { @StateObject private var model = Model() @State var map = { let map = Map(basemapStyle: .arcGISNavigation) map.initialViewpoint = Viewpoint(latitude: 34.05293, longitude: -118.24368, scale: 2e5) return map }() }
Add graphics to the map view
A graphics overlay is a container for graphics. Graphics are added as a visual means to display the search result on the map.
-
In the
Model
class, create aGraphicsOverlay
namedgraphics
. In theOverlay Content
, add the graphics overlay to the map view.View A graphics overlay is a container for graphics. It is used with a map view to display graphics on a map. You can add more than one graphics overlay to a map view. Graphics overlays are displayed on top of all the other layers.
ContentView.swiftUse dark colors for code blocks Add line. Change line import SwiftUI import ArcGIS class Model: ObservableObject { let graphicsOverlay = GraphicsOverlay() } struct ContentView: View { @StateObject private var model = Model() @State var map = { let map = Map(basemapStyle: .arcGISNavigation) map.initialViewpoint = Viewpoint(latitude: 34.05293, longitude: -118.24368, scale: 2e5) return map }() var body: some View { MapView(map: map, graphicsOverlays: [model.graphicsOverlay]) } }
-
Create a private
Graphic
property namedstart
to theGraphic Model
. Symbolize the graphic with a white circle and black outline. This graphic will be used to display the route's start location.An
SimpleMarkerSymbol
is used to display a location on the map view.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. class Model: ObservableObject { let graphicsOverlay = GraphicsOverlay() let startGraphic: Graphic = { let symbol = SimpleMarkerSymbol(style: .circle, color: .white, size: 8) symbol.outline = SimpleLineSymbol(style: .solid, color: .black, width: 1) let graphic = Graphic(symbol: symbol) return graphic }() }
-
Create a private
Graphic
property namedend
. Symbolize the graphic with a black circle. This graphic will be used to display the route's end location.Graphic ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. class Model: ObservableObject { let graphicsOverlay = GraphicsOverlay() let startGraphic: Graphic = { let symbol = SimpleMarkerSymbol(style: .circle, color: .white, size: 8) symbol.outline = SimpleLineSymbol(style: .solid, color: .black, width: 1) let graphic = Graphic(symbol: symbol) return graphic }() let endGraphic: Graphic = { let symbol = SimpleMarkerSymbol(style: .circle, color: .black, size: 9) let graphic = Graphic(symbol: symbol) return graphic }() }
-
Create a private
Graphic
property namedroute
. Symbolize the graphic with a blue line. This graphic will be used to display the route line.Graphic An
SimpleLineSymbol
is used to display a line on the map view.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. let endGraphic: Graphic = { let symbol = SimpleMarkerSymbol(style: .circle, color: .black, size: 9) let graphic = Graphic(symbol: symbol) return graphic }() let routeGraphic: Graphic = { let symbol = SimpleLineSymbol(style: .solid, color: .blue, width: 3) let graphic = Graphic(symbol: symbol) return graphic }()
-
Create an
init()
method in theModel
that addsstart
,Graphic end
, andGraphic route
to the graphics overlay. This method will be called whenGraphic Model
is initialized.Because
start
,Graphic end
, andGraphic route
haven't yet specified aGraphic Geometry
, they will not be visible.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. let routeGraphic: Graphic = { let symbol = SimpleLineSymbol(style: .solid, color: .blue, width: 3) let graphic = Graphic(symbol: symbol) return graphic }() init() { graphicsOverlay.addGraphics([routeGraphic, startGraphic, endGraphic]) }
Create a route task and route parameters
A task makes a request to a service and returns the results. Use the RouteTask
class to access a routing service.
A routing service with global coverage is part of ArcGIS location services. You can also publish custom routing services using ArcGIS Enterprise.
-
Continuing in the
Model
, create a privateRouteTask
property namedroute
with the routing service.Task ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. init() { graphicsOverlay.addGraphics([routeGraphic, startGraphic, endGraphic]) } private let routeTask = RouteTask( url: URL(string: "https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World")! )
-
Create a variable named
directions
defined as an array ofDirectionManeuver
objects. This will contain the step by step directions from the start to the end point.ContentView.swiftUse dark colors for code blocks Add line. private let routeTask = RouteTask( url: URL(string: "https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World")! ) var directions: [DirectionManeuver] = []
-
Define a private, asynchronous function named
solve
that takes a start and endRoute(start: end: ) Point
. This method will be called when both the start and end points have been placed on the map.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. private let routeTask = RouteTask( url: URL(string: "https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World")! ) var directions: [DirectionManeuver] = [] func solveRoute(from start: Point, to end: Point) async throws { }
-
Create default
RouteParameters
from theroute
namedTask route
. Configure the parameters by setting two stops (the start and end points) and specify that directions are returned.Parameters ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. func solveRoute(from start: Point, to end: Point) async throws { let routeParameters = try await routeTask.makeDefaultParameters() routeParameters.returnsDirections = true routeParameters.setStops([Stop(point: start), Stop(point: end)]) }
-
Call the
solve
function on theRoute(using: ) route
and pass in theTask route
. To display the solved route, get the first route from theParameters RouteResult
and assign itsgeometry
toroute
. To display the directions, assign theGraphic direction
value from the first route to theManeuvers directions
variable.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. func solveRoute(from start: Point, to end: Point) async throws { let routeParameters = try await routeTask.makeDefaultParameters() routeParameters.returnsDirections = true routeParameters.setStops([Stop(point: start), Stop(point: end)]) let routeResult = try await routeTask.solveRoute(using: routeParameters) if let firstRoute = routeResult.routes.first { routeGraphic.geometry = firstRoute.geometry directions = firstRoute.directionManeuvers } }
Handle map view touch events
The app will use locations derived from a user tapping the map view to generate the stops on a route. Configure the view to handle touch events from the map view. The locations derived from a user tapping the map view will be used to generate routes in a later step.
-
In the
Content
struct, create twoView @State
private variables of typePoint
namedstart
andPoint end
. These will contain the start and end locations of the route.Point ContentView.swiftUse dark colors for code blocks Add line. Add line. struct ContentView: View { @StateObject private var model = Model() @State private var startPoint: Point? @State private var endPoint: Point? @State var map = { let map = Map(basemapStyle: .arcGISNavigation) map.initialViewpoint = Viewpoint(latitude: 34.05293, longitude: -118.24368, scale: 2e5) return map }() var body: some View { MapView(map: map, graphicsOverlays: [model.graphicsOverlay]) } }
-
Add the
onSingleTapGesture
method to the map view. If it is the user's first tap, set thestart
to the currentPoint map
. Otherwise, set thePoint end
to the currentPoint map
. Assign the geometries forPoint start
andGraphic end
accordingly.Graphic ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. var body: some View { MapView(map: map, graphicsOverlays: [model.graphicsOverlay]) .onSingleTapGesture { _, mapPoint in if startPoint == nil { startPoint = mapPoint model.startGraphic.geometry = startPoint } else { endPoint = mapPoint model.endGraphic.geometry = endPoint } } }
-
Add a
.task
modifier to the map view withend
as an identifier. This task is called if the value ofPoint end
changes. Ensure that the start and end points are not nil and pass them into the model'sPoint solve
method. This attempts to solve the route using the start and end points.Route(start: end: ) ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. var body: some View { MapView(map: map, graphicsOverlays: [model.graphicsOverlay]) .onSingleTapGesture { _, mapPoint in if startPoint == nil { startPoint = mapPoint model.startGraphic.geometry = startPoint } else { endPoint = mapPoint model.endGraphic.geometry = endPoint } } .task(id: endPoint) { guard let startPoint = startPoint, let endPoint = endPoint else { return } do { try await model.solveRoute(from: startPoint, to: endPoint) } catch { print(error) } } }
Add a UI to display driving directions
To display the turn-by-turn directions from the route, some UI element is required.
-
In the ContentView struct, add a
Bool
variable namedi
to indicate if the directions are shown or not. Set its initial value tos Showing Directions false
.ContentView.swiftUse dark colors for code blocks Add line. struct ContentView: View { @StateObject private var model = Model() @State private var isShowingDirections = false @State private var startPoint: Point? @State private var endPoint: Point? @State var map = { let map = Map(basemapStyle: .arcGISNavigation) map.initialViewpoint = Viewpoint(latitude: 34.05293, longitude: -118.24368, scale: 2e5) return map }()
-
Add a
.toolbar
andToolbar
to the bottom of the map view.Item Group ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. var body: some View { MapView(map: map, graphicsOverlays: [model.graphicsOverlay]) .onSingleTapGesture { _, mapPoint in if startPoint == nil { startPoint = mapPoint model.startGraphic.geometry = startPoint } else { endPoint = mapPoint model.endGraphic.geometry = endPoint } } .task(id: endPoint) { guard let startPoint = startPoint, let endPoint = endPoint else { return } do { try await model.solveRoute(from: startPoint, to: endPoint) } catch { print(error) } } .toolbar { ToolbarItemGroup(placement: .bottomBar) { } } }
-
Add a
Button
, labeled "Show directions", to the toolbar. This button indicates that the user wants to show the directions so toggle thei
value tos Showing Directions true
.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. .toolbar { ToolbarItemGroup(placement: .bottomBar) { Button("Show directions") { isShowingDirections = true } } }
-
Add a
.popover
with aNavigation
to the toolbar. Set the popover'sView i
parameter tos Presented i
. The popover will display according to thes Showing Directions i
value. Customize the navigation view.s Showing Directions ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. .toolbar { ToolbarItemGroup(placement: .bottomBar) { Button("Show directions") { isShowingDirections = true } .popover(isPresented: $isShowingDirections) { NavigationView { } .navigationViewStyle(.stack) .frame(idealWidth: 320, idealHeight: 428) } } }
-
Within the
Navigation
content, create aView List
withdirections
which contains an array ofDirectionManeuver
objects. Configure the navigation view with a title, display mode, and "Done" button. When the button is tapped,i
is set tos Showing Directions false
which closes the popover.ContentView.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. .popover(isPresented: $isShowingDirections) { NavigationView { List(model.directions, id: \.text) { directionManeuver in Text(directionManeuver.text) } .navigationTitle("Directions") .navigationBarTitleDisplayMode(.inline) .toolbar { ToolbarItem(placement: .confirmationAction) { Button("Done") { isShowingDirections = false } } } } .navigationViewStyle(.stack) .frame(idealWidth: 320, idealHeight: 428) }
-
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Monterey 12.5, Xcode 15, iOS 17. If you are using a physical device, then refer to the system requirements.
The map should support two taps to create an origin and destination point and then use the route service to display the resulting route.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: