Learn how to set a basemap, elevation source, and data layers and display a specific area by using the C# API and the High Definition Render Pipeline from Unity.
In this tutorial, you will create a Unity local scene using a basemap layer service, and data layers. The surface of the scene is defined with an elevation layer and the camera is positioned to display a cropped area of New York City.
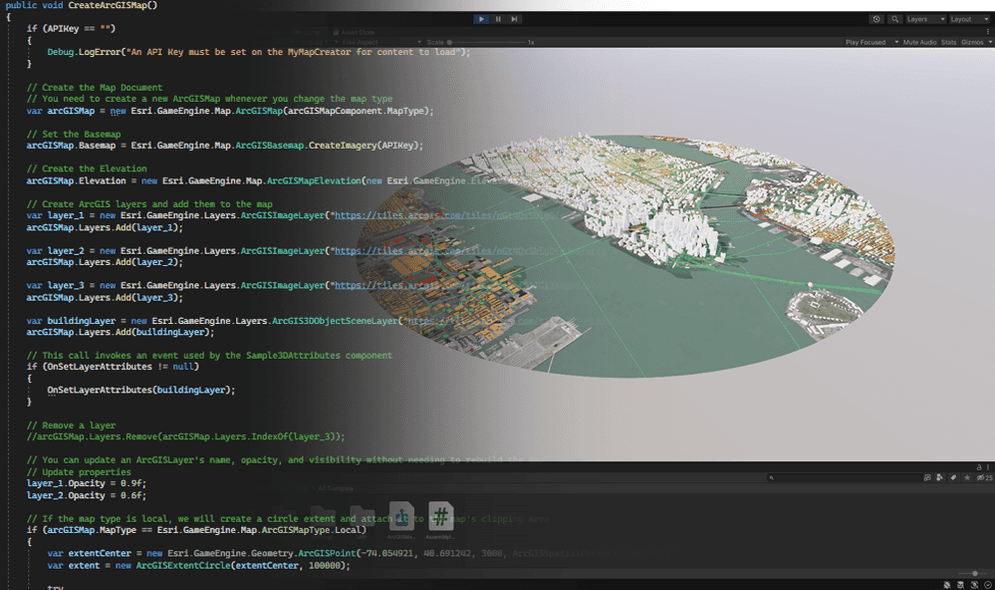
Prerequisites
Before starting this tutorial, you should:
-
Have an ArcGIS Location Platform account or ArcGIS Online account, and an API key to access ArcGIS Online services while you are developing. If you don't have an account, sign up one for free.
-
Ensure your development environment meets the system requirements.
-
Take install and setup steps and install the plugin. Make sure to import the sample assets.
-
Create a new HDRP project if you have not already done so.
For more information about Unity scripting, visit the Unity Scripting page.
Steps
Create a new scene
-
In the top menu, click File > New Scene and open the pop-up window.
-
Select the Basic Outdoors (HDRP) template and click the Create button, then save the scene with a scene name.
Prepare the script
-
Go to Assets > Create > C# Script to create a new script and name the script.
-
Add the following namespaces that you will use in this tutorial.
Use dark colors for code blocks using Esri.ArcGISMapsSDK.Components; using Esri.ArcGISMapsSDK.Samples.Components; using Esri.ArcGISMapsSDK.Utils.GeoCoord; using Esri.GameEngine.Extent; using Esri.GameEngine.Geometry; using Esri.Unity; using Unity.Mathematics; #if UNITY_EDITOR using UnityEditor; #endif using UnityEngine; using System;
-
The
Execute
attribute allows a script to run both in the editor mode and during the play mode.Always Use dark colors for code blocks [ExecuteAlways]
The Editor mode can be disabled from the Unity editor together with other options. For more information about the Editor mode, refer to the Editor mode page.
Set the API key
When you use the C# API to set up an ArcGIS Map, you have the option to set different API keys for basemap, elevation, and data layers. In this tutorial, you will set an API key as a global variable and use it for the basemap.
-
Go to your portal to create an API key. The API key must be scoped to access the basemap layer service used in this tutorial.
-
Create a global variable to store your API key under the main class as the code below. Copy and paste your obtained API key in the field, between
"
of the below code.Use dark colors for code blocks Copy public string APIKey = "";
For more information about API key, refer to the API keys section.
Add ArcGIS Map component
In this part of the tutorial, you will use ArcGIS Map component to create an ArcGISMap with the local
mode.
ArcGIS Map component has the necessary elements to create an ArcGIS Map and communicates with High Precision Framework to set the Origin Position where its value is specified with the ArcGISPoint
. All geographically located objects need to be a parent of the game object that has the ArcGIS Map component attached.
In this tutorial, use the following values for the Origin Position for New York City.
- Longitude: -74.054921;
- Latitude: 40.691242;
- Altitude: 3000;
- Spatial Reference WKID (Well-Known ID): 4326 (WGS84)
Add the ArcGIS Map component variable and set the ArcGISPoint
variable in the main class:
private ArcGISMapComponent arcGISMapComponent;
private ArcGISPoint geographicCoordinates = new ArcGISPoint(-74.054921, 40.691242, 3000, ArcGISSpatialReference.WGS84());
The method below will find if there is an existing ArcGIS Map component in the scene when the script is attached to a game object. Otherwise, it will create a game object named ArcGISMap with an ArcGIS Map component attached. You can set the ArcGISMap
for the ArcGISMap with the ArcGIS Map component. We are creating a Local
scene for this tutorial example.
private void CreateArcGISMapComponent()
{
arcGISMapComponent = FindObjectOfType<ArcGISMapComponent>();
if (!arcGISMapComponent)
{
var arcGISMapGameObject = new GameObject("ArcGISMap");
arcGISMapComponent = arcGISMapGameObject.AddComponent<ArcGISMapComponent>();
}
arcGISMapComponent.OriginPosition = geographicCoordinates;
arcGISMapComponent.MapType = Esri.GameEngine.Map.ArcGISMapType.Local;
arcGISMapComponent.MapTypeChanged += new ArcGISMapComponent.MapTypeChangedEventHandler(CreateArcGISMap);
}
Create ArcGIS Map and set a basemap
ArcGIS Map is a container of GIS content and is used with ArcGIS Renderer to visualize the content that is included in the ArcGIS Map component.
Set up a method (in this case Create
) that creates the ArcGISMap container:
public void CreateArcGISMap()
{
var arcGISMap = new Esri.GameEngine.Map.ArcGISMap(arcGISMapComponent.MapType);
}
To set up a basemap, use the ArcGISBasemap
class. There are three ways to create a basemap to use in your ArcGIS Map. In this tutorial, use ArcGISImagery
of ArcGISBasemap
class to use a basemap from the basemap styles service with the API key that is configured in the previous section. You can also set the API key directly in the constructor instead of using a global variable. For other ways to create a basemap, refer to the Basemap section.
public void CreateArcGISMap()
{
var arcGISMap = new Esri.GameEngine.Map.ArcGISMap(arcGISMapComponent.MapType);
arcGISMap.Basemap = new Esri.GameEngine.Map.ArcGISBasemap(Esri.GameEngine.Map.ArcGISBasemapStyle.ArcGISImagery, APIKey);
}
Set elevation
Use ArcGISMap
to create relief with elevation sources and their names. Use ArcGISImage
to use an elevation layer as the source.
In this tutorial, use the Terrain 3D elevation layer from Esri.
public void CreateArcGISMap()
{
var arcGISMap = new Esri.GameEngine.Map.ArcGISMap(arcGISMapComponent.MapType);
arcGISMap.Basemap = new Esri.GameEngine.Map.ArcGISBasemap(Esri.GameEngine.Map.ArcGISBasemapStyle.ArcGISImagery, APIKey);
arcGISMap.Elevation = new Esri.GameEngine.Map.ArcGISMapElevation(new Esri.GameEngine.Elevation.ArcGISImageElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer", "Terrain 3D", ""));
}
For more information about elevation, refer to the Elevation page.
Add data layers
In this section, you will add the following three image tile layers and one 3D object scene layer with their name.
-
Image tile layers
- Layer name:
M
y Layer_ 1 - ArcGIS Online item: UrbanObservatory_NYC_TransitFrequency.
- Layer name:
M
y Layer_ 2 - ArcGIS Online item: New_York_Industrial
- Layer name:
M
y Layer_ 3 - ArcGIS Online item: NewYorkCity_PopDensity
- Layer name:
-
3D object scene layer
- Layer name:
Building Layer
- ArcGIS Online item: New York, USA Buildings
- Layer name:
-
Add image tile layers to the ArcGIS Map by using
ArcGISImage
, and set the opacity toLayer 1.0f
and visibility totrue
.Use dark colors for code blocks public void CreateArcGISMap() { var arcGISMap = new Esri.GameEngine.Map.ArcGISMap(arcGISMapComponent.MapType); arcGISMap.Basemap = new Esri.GameEngine.Map.ArcGISBasemap(Esri.GameEngine.Map.ArcGISBasemapStyle.ArcGISImagery, APIKey); arcGISMap.Elevation = new Esri.GameEngine.Map.ArcGISMapElevation(new Esri.GameEngine.Elevation.ArcGISImageElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer", "Terrain 3D", "")); var layer_1 = new Esri.GameEngine.Layers.ArcGISImageLayer("https://tiles.arcgis.com/tiles/nGt4QxSblgDfeJn9/arcgis/rest/services/UrbanObservatory_NYC_TransitFrequency/MapServer", "MyLayer_1", 1.0f, true, ""); arcGISMap.Layers.Add(layer_1); var layer_2 = new Esri.GameEngine.Layers.ArcGISImageLayer("https://tiles.arcgis.com/tiles/nGt4QxSblgDfeJn9/arcgis/rest/services/New_York_Industrial/MapServer", "MyLayer_2", 1.0f, true, ""); arcGISMap.Layers.Add(layer_2); var layer_3 = new Esri.GameEngine.Layers.ArcGISImageLayer("https://tiles.arcgis.com/tiles/4yjifSiIG17X0gW4/arcgis/rest/services/NewYorkCity_PopDensity/MapServer", "MyLayer_3", 1.0f, true, ""); arcGISMap.Layers.Add(layer_3); }
-
Add the 3D object scene layer to the ArcGIS Map by using
ArcGIS3DObject
, and set the opacity toScene Layer 1.0f
and visibility totrue
.Use dark colors for code blocks public void CreateArcGISMap() { var arcGISMap = new Esri.GameEngine.Map.ArcGISMap(arcGISMapComponent.MapType); arcGISMap.Basemap = new Esri.GameEngine.Map.ArcGISBasemap(Esri.GameEngine.Map.ArcGISBasemapStyle.ArcGISImagery, APIKey); arcGISMap.Elevation = new Esri.GameEngine.Map.ArcGISMapElevation(new Esri.GameEngine.Elevation.ArcGISImageElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer", "Terrain 3D", "")); // Create ArcGIS layers and add them to the map var layer_1 = new Esri.GameEngine.Layers.ArcGISImageLayer("https://tiles.arcgis.com/tiles/nGt4QxSblgDfeJn9/arcgis/rest/services/UrbanObservatory_NYC_TransitFrequency/MapServer", "MyLayer_1", 1.0f, true, ""); arcGISMap.Layers.Add(layer_1); var layer_2 = new Esri.GameEngine.Layers.ArcGISImageLayer("https://tiles.arcgis.com/tiles/nGt4QxSblgDfeJn9/arcgis/rest/services/New_York_Industrial/MapServer", "MyLayer_2", 1.0f, true, ""); arcGISMap.Layers.Add(layer_2); var layer_3 = new Esri.GameEngine.Layers.ArcGISImageLayer("https://tiles.arcgis.com/tiles/4yjifSiIG17X0gW4/arcgis/rest/services/NewYorkCity_PopDensity/MapServer", "MyLayer_3", 1.0f, true, ""); arcGISMap.Layers.Add(layer_3); var buildingLayer = new Esri.GameEngine.Layers.ArcGIS3DObjectSceneLayer("https://tiles.arcgis.com/tiles/P3ePLMYs2RVChkJx/arcgis/rest/services/Buildings_NewYork_17/SceneServer", "Building Layer", 1.0f, true, ""); arcGISMap.Layers.Add(buildingLayer); }
For information about data layers that you can use and layer management, refer to the Data layers section.
Set map extent
Use ArcGISExtent
in the Create
method to set up a circular extent. The code below will create a circle extent based on the parameter values and attach it to the map's clipping area.
- Latitude: 40.691242
- Longitude: -74.054921
- Altitude: 3000 Meters
- Radius: 10000 Meters
arcGISMapComponent.EnableExtent = true;
var extentCenter = new Esri.GameEngine.Geometry.ArcGISPoint(-74.054921, 40.691242, 3000, ArcGISSpatialReference.WGS84());
var extent = new ArcGISExtentCircle(extentCenter, 10000);
arcGISMap.ClippingArea = extent;
If you want to configure a rectangular extent, use ArcGISExtent
instead of ArcGISExtent
and configure (extent
.
Assign the ArcGISMap object to the View
Once all the setup is completed, add the following code at the end in the Create
method to assign the ArcGISMap object to the View:
arcGISMapComponent.View.Map = arcGISMap;
Create a camera
ArcGIS Camera will load better LODs for areas closer to the view and lower LODs for the areas that are further away. Set up a Camera component variable under the main class:
private ArcGISCameraComponent cameraComponent;
The method below will first check whether there is an existing camera game object with an ArcGIS Camera and a Location component respectively. If not, those components will be added to a Camera game object that has a MainCamera tag to enable map rendering, player movement, and tile loading. A rebase component is also attached in case of exploring large areas.
private void CreateArcGISCamera()
{
cameraComponent = Camera.main.gameObject.GetComponent<ArcGISCameraComponent>();
if (!cameraComponent)
{
var cameraGameObject = Camera.main.gameObject;
cameraGameObject.transform.SetParent(arcGISMapComponent.transform, false);
cameraComponent = cameraGameObject.AddComponent<ArcGISCameraComponent>();
cameraGameObject.AddComponent<ArcGISCameraControllerComponent>();
cameraGameObject.AddComponent<ArcGISRebaseComponent>();
}
var cameraLocationComponent = cameraComponent.GetComponent<ArcGISLocationComponent>();
if (!cameraLocationComponent)
{
cameraLocationComponent = cameraComponent.gameObject.AddComponent<ArcGISLocationComponent>();
cameraLocationComponent.Position = geographicCoordinates;
cameraLocationComponent.Rotation = new ArcGISRotation(65, 68, 0);
}
}
You can set the camera position by using an ArcGISPoint
. Use ArcGISRotation
to set the pitch, roll and heading with the values below.
- Heading = 65;
- Pitch = 68;
- Roll = 0;
For information about the camera, see the Camera page.
Attach the script
Before proceeding with this section, make sure all the methods you created above are called in the Unity Start()
method.
private void Start()
{
CreateArcGISMapComponent();
CreateArcGISCamera();
CreateSkyComponent();
CreateArcGISMap();
}
Save your finished script and attach it to an empty game object or a camera game object that has a MainCamera tag in the Hierarchy window. The map should be loaded into the scene. You will also notice that the camera game object with the MainCamera tag is automatically moved under the newly generated ArcGISMap game object in the Hierarchy window.
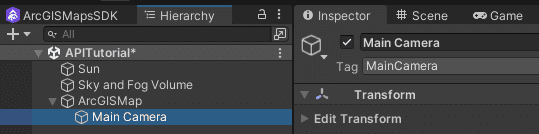
Adjust the Sun
-
In the Hierarchy window, click the Sun game object.
-
In the Inspector window, set the rotation to the following values in Transform section.
- X:
36
- Y:
110
- Z:
85
- X:
-
Open the Emission section and set the Intensity value to
10000
.
This is the result of the Sun game object.
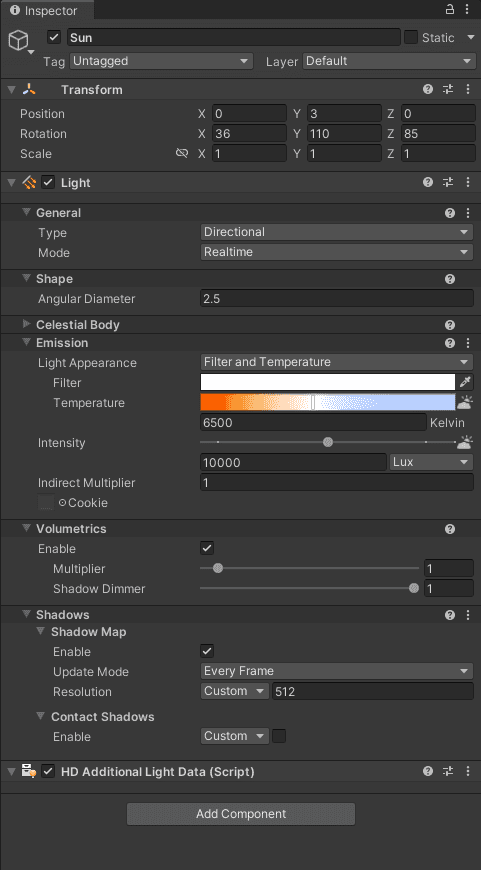
Adjust the Sky and Fog Volume
-
Click the Sky and Fog Volume in the Hierarchy window.
-
ArcGIS Maps SDK for Unity has a sample profile for Sky and Fog volume for HDRP. Click the icon next to the Profile name in the Inspector window Volume section.
-
Select the Sky and Fog Settings SampleScenes Profile in the pop-up window.
An ArcGIS Sky Reposition component adjusts the sky's parameters to account for differences between global and local map types. If you are going to switch the MapType to global and explore a larger area than a small extent area, you can either attach ArcGIS Sky Reposition component to the Sky and fog volume from the Inspector window or attach it with the script.
private void CreateSkyComponent()
{
#if USE_HDRP_PACKAGE
var currentSky = FindObjectOfType<UnityEngine.Rendering.Volume>();
if (currentSky)
{
ArcGISSkyRepositionComponent skyComponent = currentSky.gameObject.GetComponent<ArcGISSkyRepositionComponent>();
if (!skyComponent)
{
skyComponent = currentSky.gameObject.AddComponent<ArcGISSkyRepositionComponent>();
}
if (!skyComponent.arcGISMapComponent)
{
skyComponent.arcGISMapComponent = arcGISMapComponent;
}
if (!skyComponent.CameraComponent)
{
skyComponent.CameraComponent = cameraComponent;
}
}
#endif
}
When you double-click the camera game object, your Scene view camera is moved to the position. To adjust pitch, heading, and roll, hold down the right mouse button in the Scene view.
You have now successfully configured a map with the C# API. Click the Play icon on the Toolbar to see your map in the Game view.
Use the WASD keys to move left/right/forward/backward. Use the left mouse button to pan around the scene, the right button to orbit and the scroll wheel to zoom in or out.