Learn how to display a map from a mobile map package (MMPK).
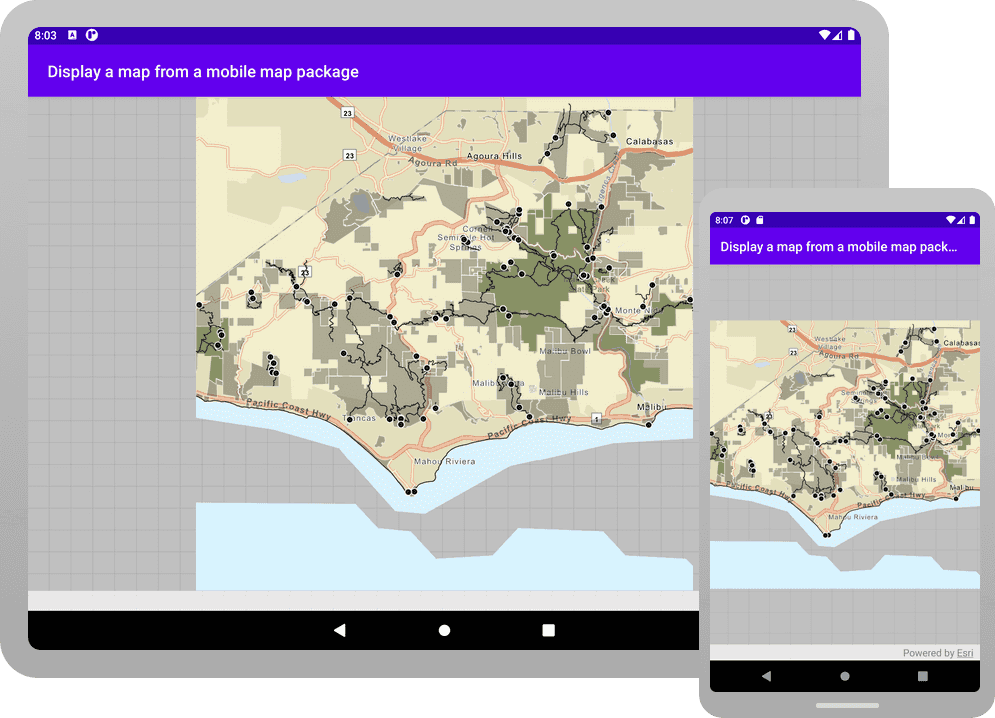
In this tutorial you will display a fully interactive map from a mobile map package (MMPK). The map contains a basemap layer and data layers and does not require a network connection.
Prerequisites
The following are required for this tutorial:
- An ArcGIS account to access API keys. If you don't have an account, sign up for free.
- Confirm that your system meets the system requirements.
- An IDE for Android development in Kotlin.
Steps
In this tutorial, you will first code, build, and install the app on a device, and then add the Mahou
.
Open an Android Studio project
-
To start this tutorial, complete the Display a map tutorial. Or download and unzip the Display a map solution in a new folder.
-
Modify the old project for use in this new tutorial. Expand More info for instructions.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android tool window, open app > res > values > strings.xml.
In the
<string name="app_
element, change the text content to Display a map from a mobile map package.name"> strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Display a map from a mobile map package</string> </resources>
-
In the Android tool window, open Gradle Scripts > settings.gradle.
Change the value of
root
to "Display a map from a mobile map package".Project.name settings.gradleUse dark colors for code blocks rootProject.name = "Display a map from a mobile map package" include ':app'
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
If you downloaded the
Display a map
solution project, get your API key and set it in your app.An API Key enables access to services, web maps, and web scenes hosted in ArcGIS Online.
-
Go to your developer dashboard to get your API key. For these tutorials, use your default API key. It is scoped to include all of the services demonstrated in the tutorials.
-
In Android Studio: in the Android tool window, open app > java > com.example.app > MainActivity.
-
In the
set
method, find theA p i K e y F o r App() ArcGISRuntime
call and paste your API key inside the quotes, replacing YOUR_API_KEY.Environment.set A p i Key("YOUR_ API_ KEY") MainActivity.ktUse dark colors for code blocks override fun onDestroy() { mapView.dispose() super.onDestroy() } private fun setApiKeyForApp(){ // set your API key // Note: it is not best practice to store API keys in source code. The API key is referenced // here for the convenience of this tutorial. ArcGISRuntimeEnvironment.setApiKey("YOUR_API_KEY") }
-
Add import statements
-
Replace app-specific import statements with the imports needed for this tutorial.
MainActivity.ktUse dark colors for code blocks Change line Change line Change line Change line Change line Change line package com.example.app import android.os.Bundle import androidx.appcompat.app.AppCompatActivity import android.util.Log import android.widget.Toast import com.esri.arcgisruntime.ArcGISRuntimeEnvironment import com.esri.arcgisruntime.loadable.LoadStatus import com.esri.arcgisruntime.mapping.MobileMapPackage import com.esri.arcgisruntime.mapping.view.MapView import com.example.app.databinding.ActivityMainBinding
Open the mobile map package and display a map
Select a map from the maps contained in a mobile map package and display the map in a map view. Use the Mobile
class to access the mobile map package and load it to read its contents.
-
In Android Studio, in the Android tool window, open Gradle Scripts > build.gradle (Module:Display_a_map_from_a_mobile_map_package.app). Make sure your file matches the one below. In particular, verify that you have the
build
andFeatures packaging
blocks shown.Options build.gradle (Module:Display_a_map_from_a_mobile_map_package.app)Use dark colors for code blocks Copy kotlinOptions { jvmTarget = JavaVersion.VERSION_1_8.toString() } buildFeatures { viewBinding true } packagingOptions { exclude 'META-INF/DEPENDENCIES' } }
-
In the Android tool window, open app > res > values > strings.xml. Add a string resource for
Mahou
.Riviera Trials.mmpk strings.xmlUse dark colors for code blocks Add line. <resources> <string name="app_name">Display a map from a mobile map package</string> <string name="mahourivieratrails_mmpk">/MahouRivieraTrails.mmpk</string> </resources>
-
In the
Main
function, create a read-only property namedActivity() TAG
for the logger, and create a late-initializing property namedmap
of typePackage Mobile
M a p Package MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. class MainActivity : AppCompatActivity() { private val activityMainBinding by lazy { ActivityMainBinding.inflate(layoutInflater) } private val mapView: MapView by lazy { activityMainBinding.mapView } val TAG: String = MainActivity::class.java.simpleName private lateinit var mapPackage: MobileMapPackage override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState)
-
In the
o
function, replace then Create() setup
call with aMap() load
call.Mobile M a p Package() MainActivity.ktUse dark colors for code blocks Change line Change line override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(activityMainBinding.root) setApiKeyForApp() // access the mobile map package from the filesystem and load it into a MapView loadMobileMapPackage(getExternalFilesDir(null)?.path + getString(R.string.mahourivieratrails_mmpk)) }
-
Find the
set
function and delete it.Up Map() MainActivity.ktUse dark colors for code blocks // set up your map here. You will call this method from onCreate() private fun setupMap() { // create a map with the BasemapStyle streets val map = ArcGISMap(BasemapStyle.ARCGIS_TOPOGRAPHIC) // set the map to be displayed in the layout's MapView mapView.map = map // set the viewpoint, Viewpoint(latitude, longitude, scale) mapView.setViewpoint(Viewpoint(34.0270, -118.8050, 72000.0)) }
-
Create a
loadl
function that takes theMobile M a p Package() .mmpk
's path and file name. In the function, create theMobile
and load the package.M a p Package MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // load your mobile map package here. You call this method from onCreate() private fun loadMobileMapPackage(mmpkFilePath: String) { // create the mobile map package mapPackage = MobileMapPackage(mmpkFilePath) // load the mobile map package mapPackage.loadAsync() } // end of loadMobileMapPackage()
-
Add a done loading listener, and pass it a lambda that will be invoked when the mobile map package has loaded. Then check whether the mobile map package has loaded and if it has any maps. Finally, add the first map from the mobile map package to the
Map
.View MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // load your mobile map package here. You call this method from onCreate() private fun loadMobileMapPackage(mmpkFilePath: String) { // create the mobile map package mapPackage = MobileMapPackage(mmpkFilePath) // load the mobile map package mapPackage.loadAsync() // add done listener which will invoke when mobile map package has loaded mapPackage.addDoneLoadingListener() { // check load status and that the mobile map package has maps if (mapPackage.loadStatus === LoadStatus.LOADED && mapPackage.maps.isNotEmpty()) { // add the map from the mobile map package to the MapView mapView.map = mapPackage.maps[0] } } } // end of loadMobileMapPackage()
-
Add a call to
log
for cases where the mobile map package status is not loaded or the package has no maps in it.Error() MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. // add done listener which will invoke when mobile map package has loaded mapPackage.addDoneLoadingListener() { // check load status and that the mobile map package has maps if (mapPackage.loadStatus === LoadStatus.LOADED && mapPackage.maps.isNotEmpty()) { // add the map from the mobile map package to the MapView mapView.map = mapPackage.maps[0] } else { // log an error if the mobile map package fails to load logError(mapPackage.loadError.message) } }
-
Create a
log
function that logs errors to logcat and to the screen via Toast. The parameter passed to the function will be logged as the message.Error() MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. } // end of loadMobileMapPackage() private fun logError(message: String?) { message?.let { Log.e(TAG, it) Toast.makeText(this, it, Toast.LENGTH_LONG).show() } }
Build and install your app
This is a necessary step, because the app install process creates the path (sdcard > Android > data > com.example.app > files) where you will upload your mobile map package to the device. Do not try to create the path manually in Device File Explorer, as you will get a Operation not permitted error.
-
Click Run > Run > app to run the app.
The Android Emulator should display and run your app in the Android Virtual Devcie (AVD) selected in the Android Studio toolbar:
If your app builds but no AVD displays, you need to add one. Click Tools > AVD Manager > Create Virtual Device...
Your app will be built and installed on the Android Virtual Device (AVD) that is currently selected in the Android Studio toolbar. On the AVD, you should see a blank window titled Display a map from a mobile map package, with the message File not found. displaying briefly at the bottom. This is expected.
Next you will add the mobile map package.
Add a mobile map package using Device File Explorer
Add a mobile map package (.mmpk) to the device file system for use by your app.
-
Create or download the
Mahou
mobile map package to your development computer. Either complete the Create a mobile map package tutorial to create the package yourself, or download theRiviera Trails.mmpk Mahou
mobile map package.Riviera Trails.mmpk -
In Android Studio, verify that your Android Virtual Device (AVD) is still connected. If it is not, expand More info below.
If you already closed the AVD on which you installed your app, then launch the Android Emulator manually.
- Click Tools > AVD Manager. In the Android Virtual Device Manager, double-click on the AVD you used before.
-
Display the Device File Explorer tool window, which shows the file system on your AVD. Click View > Tool Windows > Device File Explorer and wait until Device File Manager connects to your AVD and shows the file system tree.
-
Upload the MahouRivieraTrails.mmpk file from your development computer.
-
In Device File Manager, right-click on the sdcard > Android > data directory and click Synchronize.
-
Right-click on sdcard > Android > data > com.example.app > files and click Upload. Navigate to the MahouRivieraTrails.mmpk file on your development computer. Then click OK.
You should see a Device File Explorer Toast in the lower corner confirming success.
-
Restart the app on your AVD
-
On your AVD, shut down your app. On many recent Android devices, you shut down an app by tapping the square icon and swiping up on the app window.
-
Then go to the All Apps screen and start the app again by clicking the Display a mobile map package app icon.
You will see a map of trailheads, trails, and parks for the area south of the Santa Monica mountains. You can pinch (to zoom and rotate), drag, and double-tap the map view to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: