This sample demonstrates how to symbolize features based on unique values or types. The data is stored in a single layer where the visualization of each feature depends on the value of one or more fields.
Prior to completing the following steps, you should be familiar with views, Map, and FeatureLayer. If necessary, complete the following tutorials first:
The basic components of this app, such as creating instances of the Map and MapView classes and understanding HTML and CSS structure will not be reviewed. See the tutorials listed above if you need to familiarize yourself with those components in this application. As a general rule the introductory principles discussed in the tutorials above apply to most samples in the documentation.
1. Create a symbol for each type
In this sample we add a new renderer to a layer representing major highways and roads in the United States. For the purposes of this app, we want to visualize each highway based on whether it's an interstate, U.S. highway, or other major highway or road.
First, we'll define a separate symbol for each type programmatically. We'll use a SimpleLineSymbol to visualize each type since they are polyline geometries.
const colors = ["#d92b30", "#3cccb4", "#ffdf3c", "#c27c30", "#f260a1"];
const commonProperties = {
type: "simple-line",
width: "4px",
style: "solid"
};
// Symbol for Interstate highways
const fwySym = {
...commonProperties,
color: colors[0]
};
// Symbol for U.S. Highways
const hwySym = {
...commonProperties,
color: colors[1]
};
// Symbol for state highways
const stateSym = {
...commonProperties,
color: colors[2]
};
// Symbol for other major highways
const majorSym = {
...commonProperties,
color: colors[3]
};
// Symbol for other major highways
const otherSym = {
...commonProperties,
color: colors[4]
};
2. Create an instance of UniqueValueRenderer
We must use a UniqueValueRenderer when defining how features should be visualized based on field values. Up to three fields may be used to create various combinations of types. In this case we're only visualizing each feature based on one field: RTTYP
(i.e. Route Type).
const hwyRenderer = {
type: "unique-value", // autocasts as new UniqueValueRenderer()
field: "RTTYP",
};
3. Match unique values with each symbol
You can match symbols with unique field values in one of two ways: Using uniqueValueInfos in the constructor...
const hwyRenderer = {
type: "unique-value", // autocasts as new UniqueValueRenderer()
legendOptions: {
title: "Route type"
},
defaultSymbol: otherSym,
defaultLabel: "Other",
field: "RTTYP",
uniqueValueInfos: [
{
value: "I", // code for interstates/freeways
symbol: fwySym,
label: "Interstate"
},
{
value: "U", // code for U.S. highways
symbol: hwySym,
label: "US Highway"
},
{
value: "S", // code for U.S. highways
symbol: stateSym,
label: "State Highway"
},
{
value: "M", // code for U.S. highways
symbol: majorSym,
label: "Major road"
}
]
};
Or with the addUniqueValueInfo() method.
hwyRenderer.addUniqueValueInfo("I", fwySym);
4. Summary
Once the renderer is defined, you can set it on the layer and the view and legend will automatically update. Click the sandbox button below to see the full code of the app.
Related samples and resources
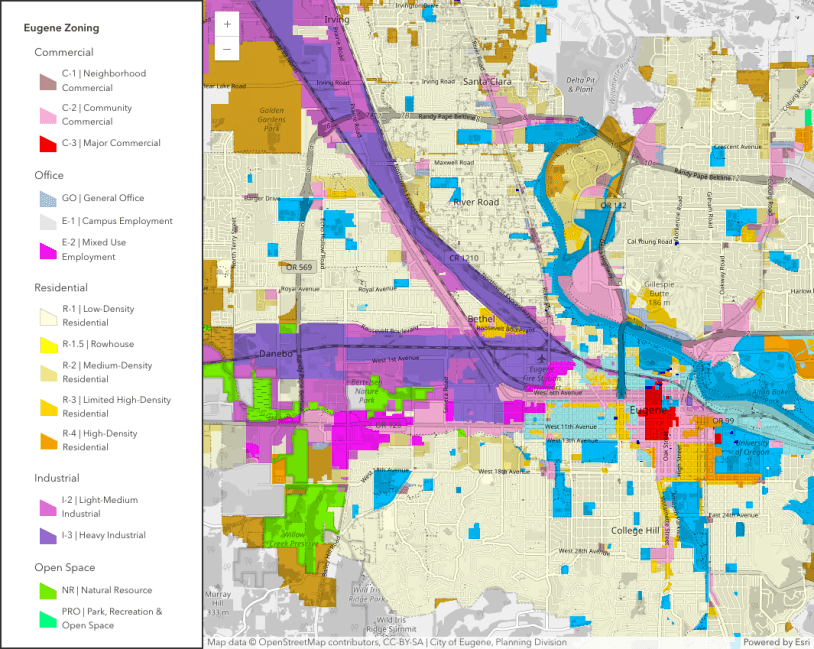
Unique value groups with headings
This sample demonstrates how to categorize unique values into groups with headings.
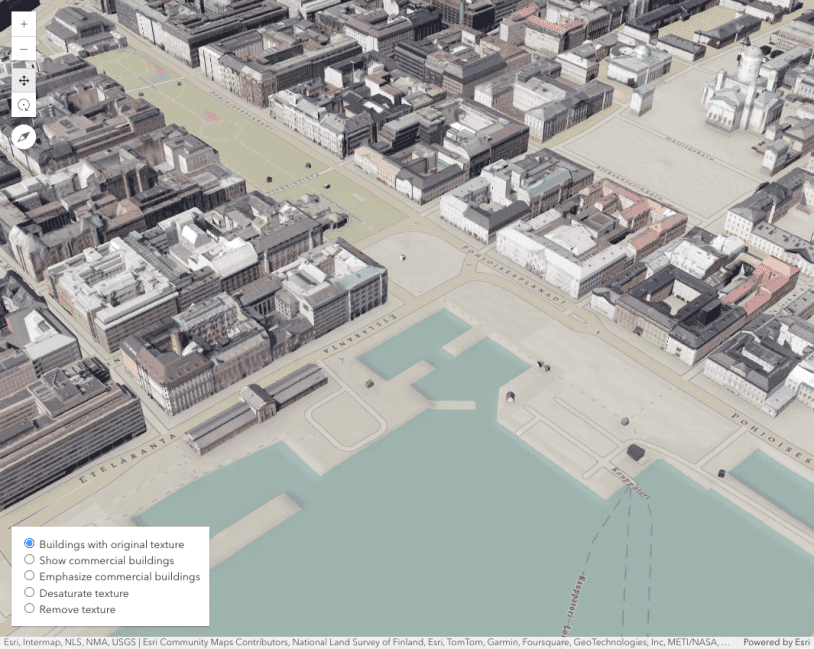
Coloring options for textured buildings
Coloring options for textured buildings
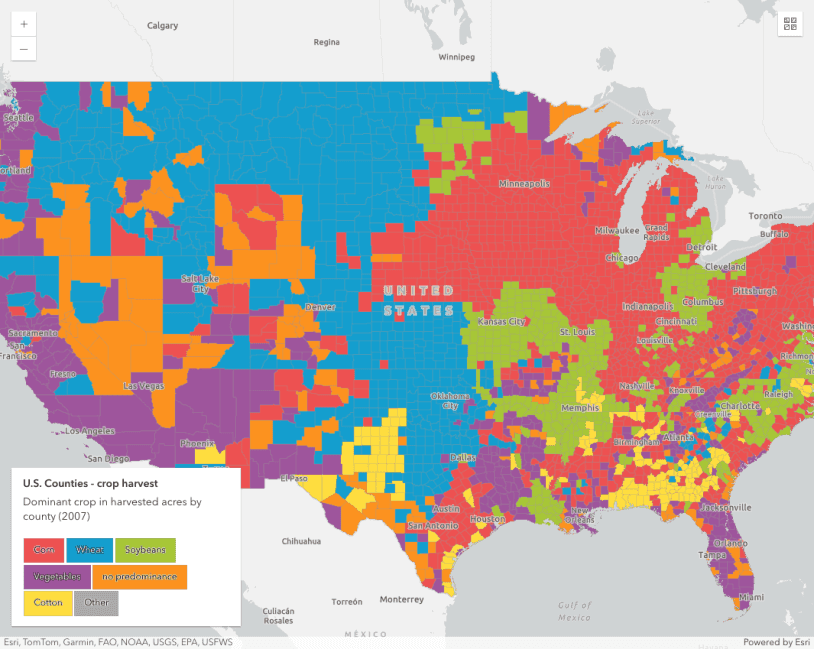
Generate data-driven visualization of unique values
Generate data-driven visualization of unique values
UniqueValueRenderer
Read the API Reference for more information.