This authentication method is ideal for serverless web applications and applications that run entirely in a browser using the OAuth 2.0 implicit
grant type.
Overview
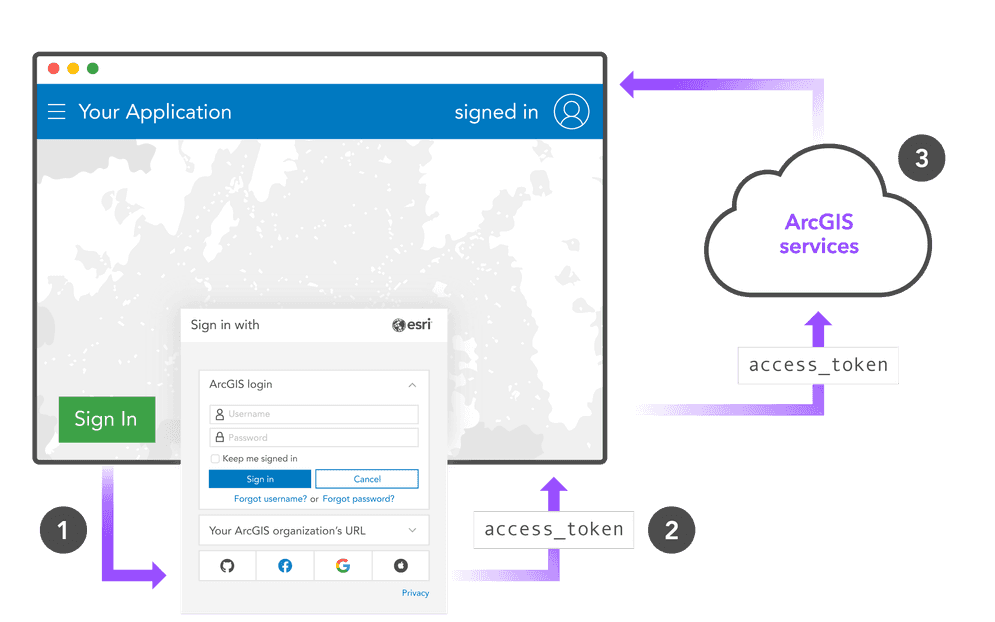
-
Direct your users to the authorization endpoint in a browser (pop-up or a new tab) with your
client_
,id response_
, atype=token redirect_
, and an optionaluri expiration
. -
The user successfully provides their username + password.
-
The pop-up or tab is redirected back to your application's
redirect_
from step 1 and theuri access_
andtoken expiration
are appended to the URL as a hash:http:
.//app.example.com/cb#access_ token=2Yotn FZFEjr1z Csic M W p AA&expires_ in=3600 Your application parses the URL for the user's token.
-
Include this
access_
in all future requests that require a token.token
Process details
Configure the redirect URI
-
Sign into your dashboard and click the OAuth 2.0 tab.
-
Follow the steps in the Add a redirect URI tutorial.
You can also configure a redirect URI in ArcGIS Online or ArcGIS Enterprise
Get an access token
-
Direct your user to an authorization endpoint (either a pop-up or a new tab) to sign in with their username + password. Ensure that you include the authorization parameters.
A sample authorization URL for an ArcGIS Online account might look like this:
Use dark colors for code blocks Copy https://www.arcgis.com/sharing/rest/oauth2/authorize?client_id=<APP_CLIENT_ID>&response_type=token&expiration=20160&redirect_uri=<REDIRECT_URI>
You could also construct an
<
tag:a> Use dark colors for code blocks Copy <a href="https://www.arcgis.com/sharing/rest/oauth2/authorize?client_id=<APP_CLIENT_ID>&response_type=token&expiration=20160&redirect_uri=<REDIRECT_URI>">Sign In With ArcGIS</a>
Or open the authorization page in a popup window:
Use dark colors for code blocks Copy const clientId = 'APP_CLIENT_ID'; const redirectUri = 'REDIRECT_URI'; const signInButton = document.getElementById('sign-in'); // do this on a button click to avoid popup blockers document.addEventListener('click', function(){ window.open('https://www.arcgis.com/sharing/rest/oauth2/authorize?client_id='+clientId+'&response_type=token&expiration=20160&redirect_uri=' + window.encodeURIComponent(redirectUri), 'oauth-window', 'height=400,width=600,menubar=no,location=yes,resizable=yes,scrollbars=yes,status=yes') });
-
The user provides their credentials.
-
The pop-up or tab redirects the user to your application's
redirect_
with anuri access_
andtoken expires_
appended to the URL as a hash:in http:
.//app.example.com/cb#access_ token=2Yotn FZFEjr1z Csic M W p AA&expires_ in=3600
Authorization endpoints
https:
https:
Authorization parameters
Parameter | Required | Format | Description |
---|---|---|---|
client_ | ✓ | string | Your application's client_ . |
redirect_ | ✓ | string | The redirect_ configured previously. The user is redirected to this endpoint with the access_ . The URI must match a URI you define in the developer dashboard, otherwise, the authorization will be rejected. |
response_ . | ✓ | string | You must include this OAuth 2.0 grant type. The type for implicit grant authorization is the string token . |
expiration | number | Optional expiration after which the token will expire. Defined in minutes with a maximum of two weeks (20160 minutes). |
Parse the token from the URL
The redirect_
is a callback page on your web application, typically something like http:
, to which the user is sent after successfully entering their sign-in credentials.
The user's access_
and its expiration (expires_
, expressed in seconds) are appended to the URL:
http://example.com/oauth-callback.html#access_token=mpd9uhQPuHBOkQPCqkT7vhAMk8P2YNA-dEMhwYt5Gp535k92BshScRcZ8dEIuAxvFALxOn6M0RTonme9DzQ9PdwT3vr17yoOdxON5vnlpXUQcOWOu3u8EBT-xSR_zWRpna7Y2-avCVtND55HNX5Egg..&expires_in=3600
Your page needs to parse the access_
from window.location.hash
and either pass it to the parent page (if a pop-up) or redirect the user to another page after it stores the token in a cookie or other persisted storage.
Parse the token from a pop-up
Here is an example of parsing the access_
if the window opens as a pop-up:
<!DOCTYPE html>
<head></head>
<body>
<script>
// try to match an access token in window.location.hash
const match = (window.location.hash) ? window.location.hash.match(/#access_token=([^&]+)/) : false;
// if we found an access token in the URL pass the token up to a global function in
if(match[1]) {
const parentWindow = (window.opener && window.opener.parent) ? window.opener.parent : window
parentWindow.oauthCallback(match[1]);
}
window.close();
</script>
</body>
</html>
You can also use a regular expression to extract the user's username
from window.location.hash
. User names are guaranteed to be unique across the entire platform.
Token expiration
Tokens obtained through this workflow will always expire and cannot be refreshed; if a token expires, the user must sign in again. If your app requires longer sessions or if you need to refresh the user's token, use the server-based workflow.