What is a geometry calculation analysis?
A geometry calculation analysis is the process of using operations such as buffer, intersect, union, and nearest to create a new output geometry. The resulting geometry is commonly displayed as a shape on a map or used as input for another analysis. To execute the analysis, you can use ArcGIS Maps SDK for JavaScript, ArcGIS Maps SDKs for Native Apps, or ArcGIS API for Python. See the code examples below.
You can use geometry calculations to:
- Buffer points, lines, and polygons.
- Intersect two or more geometries of the same type.
- Union two or more geometries of the same type.
- Find the closest coordinate.
- Split a geometry into multiple geometries.
- Add vertices or densify a geometry.
- Simplify or correct a geometry.
How to calculate geometries
To perform a calculation on a geometry you typically:
- Create one or more geometries.
- Perform the operation.
Types of calculations
Below is a list of common operations supported by most ArcGIS client APIs. For specific details about how to use the operations, see the examples below.
The table below includes some of the most popular operations for performing geometric calculations. Most operations return a measurement value or a new geometry. Multipoint, multipolyline, and multipolygon geometry types are also supported.
Operation | Description | Example |
---|---|---|
Boundary | Returns a polyline or polygon for the area of a geometry. | |
Buffer | Returns a polygon that surrounds a geometry at a specified distance. | |
Clip | Returns a geometry where a target geometry and envelope intersect. | |
Convex Hull | Returns a polygon with the shortest perimeter that encloses one or more geometries. | |
Cut | Returns geometries where an input polyline crosses an existing geometry. | |
Densify | Returns a geometry that has intermediate points at a specified distance between existing vertices. | |
Difference | Returns one or more geometries that are the result of the set theoretic difference between one geometry and another geometry. | |
Extent | Returns a rectangle geometry that encompasses the coordinates of an input geometry. | |
Generalize | Returns a geometry where the vertices that contribute least to the shape of the input polygon or polyline are removed, while retaining the characteristics of the shape. The number of vertices removed depends on a given spatial deviation distance | |
Intersect | Returns a geometry based on set theoretic intersection of the input geometries. | |
Nearest Coordinate | Returns the coordinate of a geometry that is closest to a specified point. | |
Nearest Vertex | Returns the vertex of a geometry that is closest to a specified point. | |
Offset | Returns a geometry that is a constant distance from an input polyline or polygon. | |
Reshape | Returns a geometry that takes the shape of the reshaper input where the reshaper geometry intersects the input geometry. | |
Simplify | Returns a geometry that is topologically correct. | |
Trim | Returns a geometry that intersects the input geometry and trim polyline. | |
Union | Returns a geometry based on the set theoretic union of the input geometries. |
Code examples
Buffer
Create 1000 meter buffers around different types of geometry.
const bufferGeometry = geometryEngine.geodesicBuffer(
geometry,
2000,
"meters"
);
console.log("The buffer geometry is:" + bufferGeometry);
Intersect
Intersect two geometries.
const intersectGeometry = geometryEngine.intersect(polygon1, polygon2);
console.log("The intersected geometry is:" + intersectGeometry);
Union
Union two geometries.
const unionGeometry = geometryEngine.union(polygon1, polygon2);
console.log("The union geometry is:" + unionGeometry);
Nearest
Find the vertex in a polygon that is closest to each point. In this case, the polygon is a buffer created from a line. It contains many vertices.
const nearestGeometry = geometryEngine.nearestVertex(bufferGeometry, geometry);
console.log("The nearestGeometry geometry is:" + nearestGeometry);
Tutorials
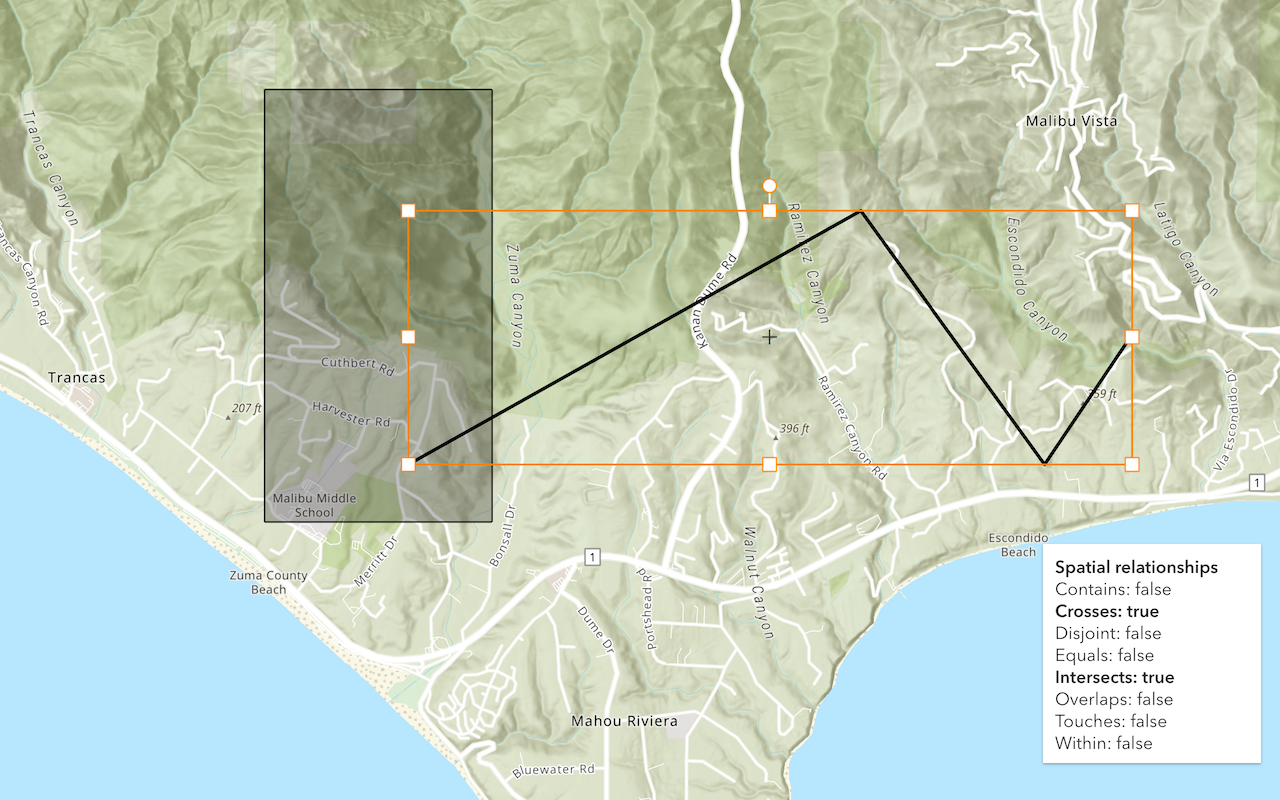
Find spatial relationships
Determine the spatial relationship between two geometries.
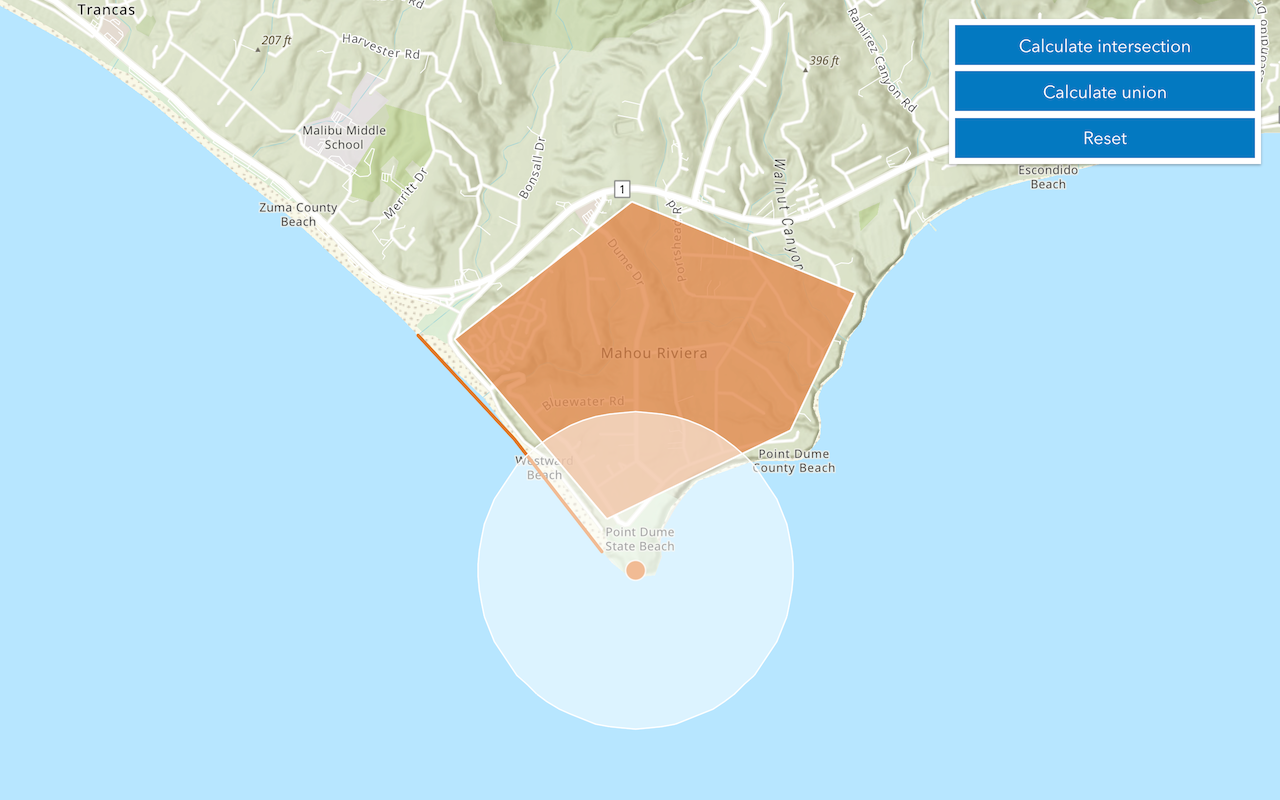
Calculate geometries
Perform buffer, intersect, union, and other geometric operations.
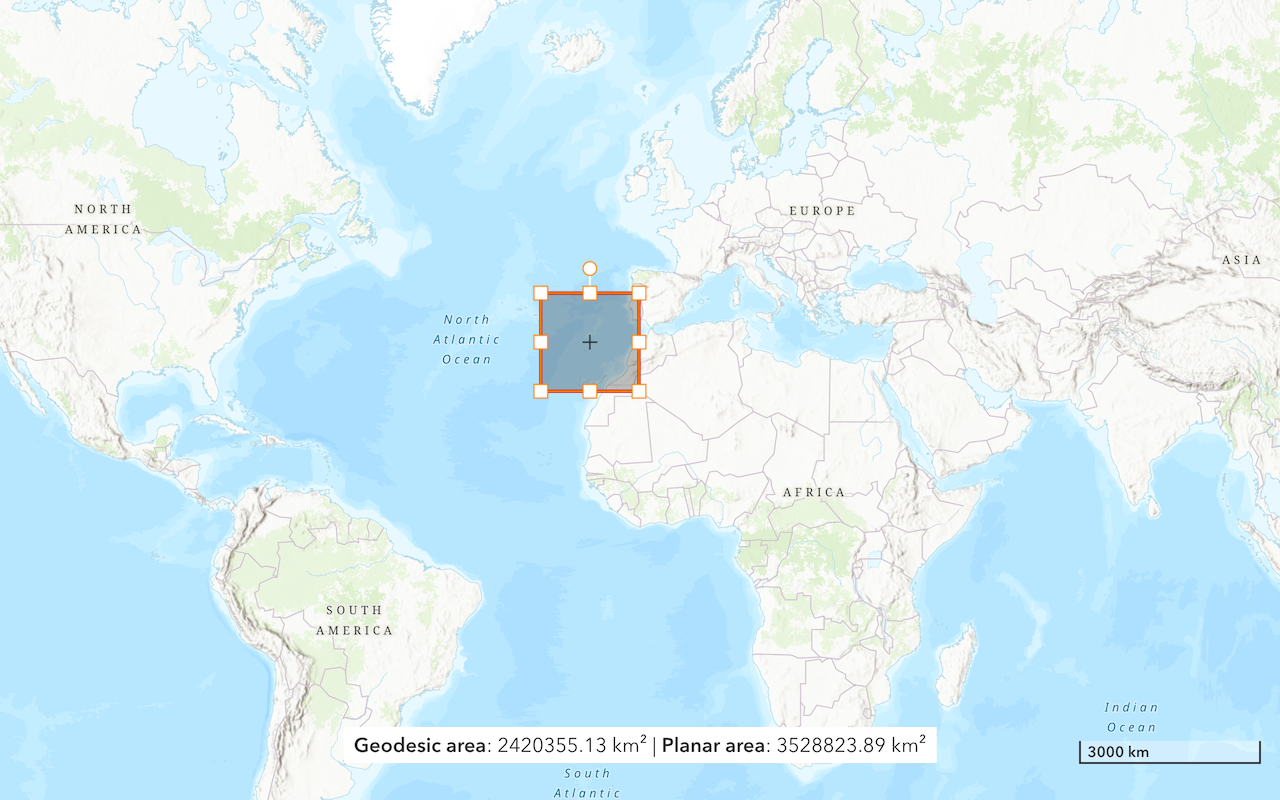
Find length and area
Get the length of a line and the area of a polygon.
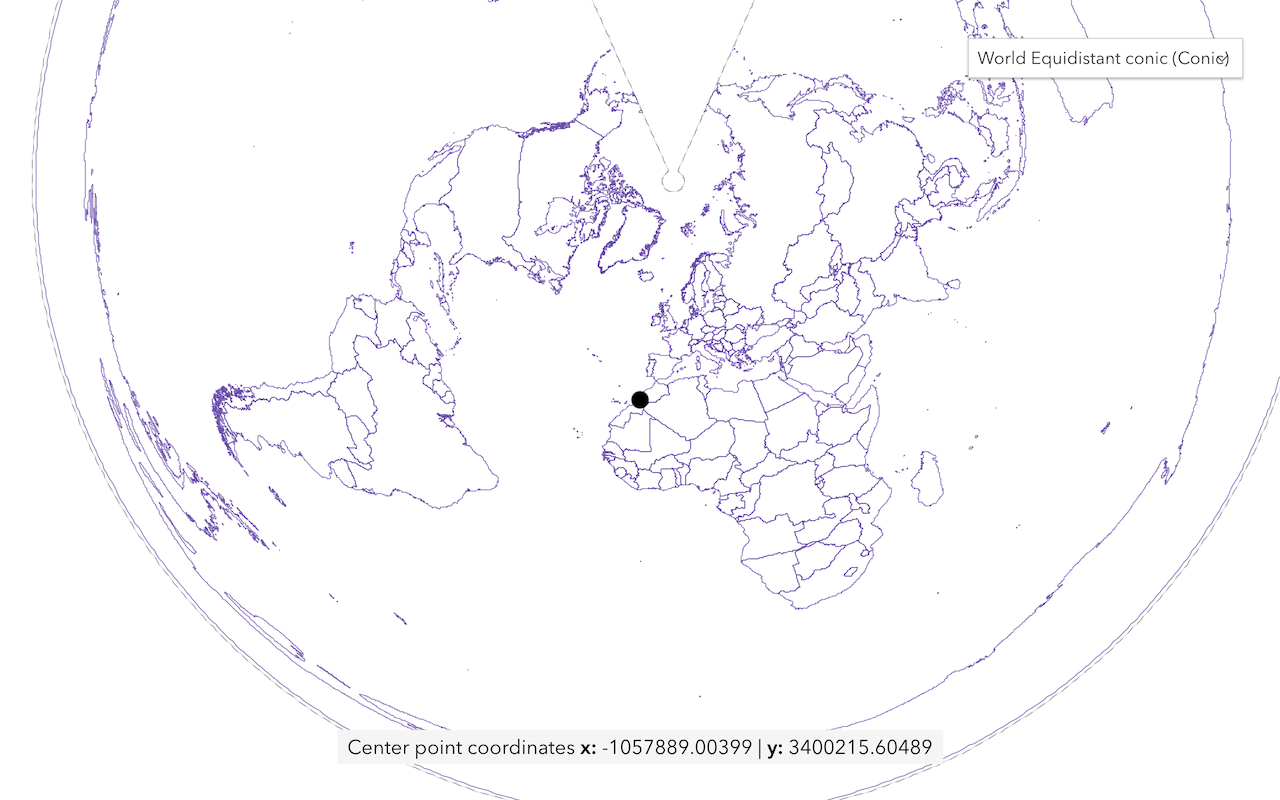
Display projected geometries
Project geometries to a new spatial reference in a map.
API support
Spatial relationship | Geometric calculation | Length and area | Projection | |
---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | ||||
ArcGIS Maps SDK for .NET | ||||
ArcGIS Maps SDK for Kotlin | ||||
ArcGIS Maps SDK for Swift | ||||
ArcGIS Maps SDK for Java | ||||
ArcGIS Maps SDK for Qt | ||||
ArcGIS API for Python | ||||
ArcGIS REST JS | ||||
Esri Leaflet | ||||
MapBox GL JS | ||||
OpenLayers |