What is a merge analysis?
A merge analysis is the process combining two feature datasets into a single dataset. To execute the analysis, use the spatial analysis service and the Merge
operation.
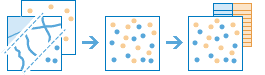
The input feature data for merge must contain the same type of geometry (point, polyline, or polygon). The attributes can be added, removed, renamed, or matched.
Real-world examples of this analysis include the following:
- Combining feature layers for England, Wales, and Scotland into a single layer of Great Britain.
- Merging park and seismic hazard zones to create an exclusion zone where a new park cannot be developed.
- Combining parcel information for contiguous townships into a single layer and keeping only the fields that have the same name and type.
How to perform a merge analysis
The general steps to performing a merge analysis are as follows:
- Review the parameters for the
Merge
operation.Layers - Send a request to get the spatial analysis service URL.
- Execute a job request with the following URL and parameters:
- URL:
https:
//< YOUR_ ANALYSIS_ SERVICE>/arcgis/rest/services/tasks/GPServer/Merge Layers/submit Job - Parameters:
input
: Your hosted feature layer dataset or feature collection.Layer merge
: The hosted feature layer dataset or feature collection to merge with theLayer input
.Layer merging
: An array of values that describe how fields from theAttributes merge
are to be modified. By default, all fields from both inputs will be carried across to the outputLayer merged
.Layer output
: A string representing the name of the hosted feature layer to reutrn with the results.Name
- URL:
- Check the status.
- Get the output layer results.
To see examples using ArcGIS API for Python, ArcGIS REST JS, and the ArcGIS REST API, go to Examples below.
URL request
http://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/MergeLayers/submitJob?<parameters>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token | An OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< |
input | The point, polyline, or polygon features to merge with the merge . | {"url": < {"layer |
merge | The point, polyline, or polygon features to merge with the input . The mergeLayer must contain the same feature type (point, polyline, or polygon) as the inputLayer. | {"url": < {"layer |
Key parameters
Name | Description | Examples |
---|---|---|
merging | An array of values that describe how fields from the merge are to be modified. By default, all fields from both inputs will be carried across to the output mergedLayer. | ['Temp Remove', 'Risk |
output | A string representing the name of the hosted feature layer to return with the results. NOTE: If you do not include this parameter, the results are returned as a feature collection (JSON). | {"service |
Code examples
Merge school layers
This example uses the Merge
operation to combine two point layers for different school types (public and private) into a single point layer containing both.
In the analysis, the input
value is the Public schools hosted feature layer. The merge
value is the Private schools hosted feature layer. By default, all fields from both layers are available in the resulting layer.
APIs
input_layer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/SA_Merge_Public_Schools/FeatureServer/0"
merge_layer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/SA_Merge_Private_School/FeatureServer/0"
results = merge_layers(
input_layer=input_layer,
merge_layer=merge_layer,
# Output results as a new hosted feature layer
output_name="Merge layers results",
)
result_features = results.layers[0].query()
print(f"Merged layer contains {len(result_features.features)} new records")
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/MergeLayers/submitJob HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
&inputLayer={"url":"https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/SA_Merge_Public_Schools/FeatureServer/0"}
&mergeLayer={"url":"https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/SA_Merge_Private_School/FeatureServer/0"}
&outputName={"serviceProperties":{"name":"Merge layers results"}}
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSubmitted",
"results": {},
"inputs": {},
"messages": []
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/MergeLayers/jobs/<JOB_ID> HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSucceeded",
"results": {
"mergedLayer": {
"paramUrl": "results/mergedLayer"
}
},
"inputs": {},
"messages": []
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/MergeLayers/jobs/<JOB_ID>/results/mergedLayer HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"paramName": "mergedLayer",
"dataType": "GPString",
"value": {
"featureSet": {
"features": [
{
"attributes": {
"OBJECTID": 1,
"X": -80.2532848806367,
"Y": 25.8523688474433,
"NAME": "Madison Middle",
"ADDRESS": "3400 NW 87th St",
"UNIT": " ",
"CITY": "Miami-Dade",
"ZIPCODE": 33147,
"TYPE": "M",
"GRADES": "6-8",
"CAPACITY": 804,
"ENROLLMNT": 458,
"LAT": 25.85236,
"LON": -80.25328,
"POINT_X": 901736.382180452,
"POINT_Y": 552729.823876381,
"GRDLEVEL": null,
"COED": null,
"STREGFLG": null,
"CHLDCAREID": null,
"CreationDate": null,
"Creator": null,
"EditDate": null,
"Editor": null
},
"geometry": {
"x": -8933754.2641,
"y": 2980806.5582999997
}
}
// more features...
],
"geometryType": "esriGeometryPoint",
"spatialReference": {
"wkid": 102100,
"latestWkid": 3857
}
},
"layerDefinition": {
"geometryType": "esriGeometryPoint",
"spatialReference": {
"wkid": 102100,
"latestWkid": 3857
},
"objectIdField": "OBJECTID",
"type": "Feature Layer",
"fields": [
// field definitions
],
"drawingInfo": {
"renderer": {
"type": "simple",
"symbol": {
"type": "esriSMS",
"style": "esriSMSCircle",
"color": [79, 129, 189, 255],
"size": 10,
"angle": 0,
"xoffset": 0,
"yoffset": 0,
"outline": {
"color": [54, 93, 141, 255],
"width": 1
}
}
}
}
}
}
}
Merge transportation layers
This example uses the Merge
operation to combine two polyline layers for different ground transportation types.
In the analysis, the input
value is the Bike routes hosted feature layer. The merge
is the Light rail line hosted layer.
The merging
parameter is used to rename the fields from the rail lines layer in the resulting feature data:
STATUS
-->RAIL_
STATUS TYPE
-->RAIL_
TYPE LINE
-->RAIL_
LINE
APIs
input_layer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Portland Bike Routes/FeatureServer/0"
merge_layer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Light Rail Lines/FeatureServer/0"
results = merge_layers(
input_layer=input_layer,
merge_layer=merge_layer,
merging_attributes=[
"STATUS Rename RAIL_STATUS",
"TYPE Rename RAIL_TYPE",
"LINE Rename RAIL_LINE",
],
context={
"extent": {
"xmin": -13659922.688640626,
"ymin": 5701881.312173232,
"xmax": -13652866.595467059,
"ymax": 5705965.915872216,
"spatialReference": {"wkid": 102100, "latestWkid": 3857},
},
},
# Outputs results as a hosted feature layer.
output_name="Merge layers results",
)
result_features = results.layers[0].query()
print(f"Merged layer contains {len(result_features.features)} new records")
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/MergeLayers/submitJob HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
&inputLayer={"url":"https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Portland Bike Routes/FeatureServer/0"}
&mergeLayer={"url":"https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Light Rail Lines/FeatureServer/0"}
&mergingAttributes=["STATUS Rename RAIL_STATUS","TYPE Rename RAIL_TYPE","LINE Rename RAIL_LINE"]
&context={"extent":{"xmin":-13659922.688640626,"ymin":5701881.312173232,"xmax":-13652866.595467059,"ymax":5705965.915872216,"spatialReference":{"wkid":102100,"latestWkid":3857}}}
&outputName={"serviceProperties":{"name":"Merge layers results"}}
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSubmitted",
"results": {},
"inputs": {},
"messages": []
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/MergeLayers/jobs/<JOB_ID> HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSucceeded",
"results": {
"mergedLayer": {
"paramUrl": "results/mergedLayer"
}
},
"inputs": {},
"messages": []
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/MergeLayers/jobs/<JOB_ID>/results/mergedLayer HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"paramName": "mergedLayer",
"dataType": "GPString",
"value": {
"featureSet": {
"features": [
{
"attributes": {
"OBJECTID": 1,
"X": -80.2532848806367,
"Y": 25.8523688474433,
"NAME": "Madison Middle",
"ADDRESS": "3400 NW 87th St",
"UNIT": " ",
"CITY": "Miami-Dade",
"ZIPCODE": 33147,
"TYPE": "M",
"GRADES": "6-8",
"CAPACITY": 804,
"ENROLLMNT": 458,
"LAT": 25.85236,
"LON": -80.25328,
"POINT_X": 901736.382180452,
"POINT_Y": 552729.823876381,
"GRDLEVEL": null,
"COED": null,
"STREGFLG": null,
"CHLDCAREID": null,
"CreationDate": null,
"Creator": null,
"EditDate": null,
"Editor": null
},
"geometry": {
"x": -8933754.2641,
"y": 2980806.5582999997
}
}
// more features...
],
"geometryType": "esriGeometryPoint",
"spatialReference": {
"wkid": 102100,
"latestWkid": 3857
}
},
"layerDefinition": {
"geometryType": "esriGeometryPoint",
"spatialReference": {
"wkid": 102100,
"latestWkid": 3857
},
"objectIdField": "OBJECTID",
"type": "Feature Layer",
"fields": [
// field definitions
],
"drawingInfo": {
"renderer": {
"type": "simple",
"symbol": {
"type": "esriSMS",
"style": "esriSMSCircle",
"color": [79, 129, 189, 255],
"size": 10,
"angle": 0,
"xoffset": 0,
"yoffset": 0,
"outline": {
"color": [54, 93, 141, 255],
"width": 1
}
}
}
}
}
}
}
Tutorials
Learn how to perform related analyses interactively with Map Viewer and programmatically with ArcGIS API for Python, ArcGIS REST JS, and ArcGIS REST API.
Services
Spatial analysis service
Process spatial datasets to discover relationships and patterns.
API support
- 1. Access with geoprocessing task
- 2. Access via ArcGIS REST JS
Tools
Developer dashboard
Manage API keys, service usage, and data with the ArcGIS Developers website.
ArcGIS.com
Create, manage, and share content and data with GIS tools.
Map Viewer
Create, explore, and share web maps for 2D applications.
ArcGIS Pro
Explore, visualize, and analyze both 2D and 3D data with desktop GIS tools.