Learn how to display point, line, and polygon graphics in a map.
Graphics are visual elements used to display points, lines, polygons, and text in a map or scene. Graphics are composed of a geometry, symbol, and attributes, and can display a pop-up when clicked. You typically use graphics to display geographic data that is not connected to a database (i.e. a GPS location).
In this tutorial, you will learn how to display points, lines, and polygons on a map as graphics.
Prerequisites
The ArcGIS API for Python tutorials use Jupyter Notebooks to execute Python code. If you are new to this environment, please see the guide to install the API and use notebooks locally.
Steps
Import modules and log in
-
Import the
arcgis.gis
andarcgis.geometry
modules. TheGIS
class provides the information model for a GIS hosted in ArcGIS Online or Portal for ArcGIS. Thegeometry
module provides functions for managing and working with different geometric types.Use dark colors for code blocks from arcgis.gis import GIS from arcgis.geometry import Point, Polyline, Polygon
-
Log in anonymously to access publicly shared content. The data used in this tutorial is public so you do not need credentials to access it. If it were private data, you would be required to provide authentication.
Use dark colors for code blocks from arcgis.gis import GIS from arcgis.geometry import Point, Polyline, Polygon portal = GIS() # anonymously
Create the graphics
-
Use the
Point
class to create a new graphic. Use adict
object to create the symbol and attributes.Use dark colors for code blocks portal = GIS() # anonymously point = Point({"x": -118.8066, "y": 34.0006, "z": 100}) point_attributes = {"name": "Point", "description": "I am a point"} simple_marker_symbol = { "type": "esriSMS", "style": "esriSMSCircle", "color": [0, 0, 0], "outline": {"color": [255, 255, 255], "width": 1}, }
-
Use the
Polyline
class to create a new graphic object. Use adict
objects to create the symbol and attributes objects.Use dark colors for code blocks point = Point({"x": -118.8066, "y": 34.0006, "z": 100}) point_attributes = {"name": "Point", "description": "I am a point"} simple_marker_symbol = { "type": "esriSMS", "style": "esriSMSCircle", "color": [0, 0, 0], "outline": {"color": [255, 255, 255], "width": 1}, } polyline = Polyline( { "paths": [ [-118.821527826096, 34.0139576938577], [-118.814893761649, 34.0080602407843], [-118.808878330345, 34.0016642996246], ] } ) polyline_attributes = {"name": "Polyline", "description": "I am a Polyline"} simple_line_symbol = { "type": "esriSLS", "style": "esriSLSolid", "color": [255, 155, 128], "width": 2, }
-
Use the
Polygon
class to create a new graphic object. Use adict
objects to create the symbol and attributes objects.Use dark colors for code blocks polyline = Polyline( { "paths": [ [-118.821527826096, 34.0139576938577], [-118.814893761649, 34.0080602407843], [-118.808878330345, 34.0016642996246], ] } ) polyline_attributes = {"name": "Polyline", "description": "I am a Polyline"} simple_line_symbol = { "type": "esriSLS", "style": "esriSLSolid", "color": [255, 155, 128], "width": 2, } polygon = Polygon( { "rings": [ [ [-118.818984489994, 34.0137559967283], [-118.806796597377, 34.0215816298725], [-118.791432890735, 34.0163883241613], [-118.79596686535, 34.008564864635], [-118.808558110679, 34.0035027131376], ] ] } ) polygon_attributes = {"name": "Polygon", "description": "I am a Polygon"} simple_fill_symbol = { "type": "esriSFS", "color": [50, 100, 200, 125], "outline": {"color": [255, 255, 255], "width": 1}, }
Display the results
-
Use the
map
method to create a new map widget.Use dark colors for code blocks polygon = Polygon( { "rings": [ [ [-118.818984489994, 34.0137559967283], [-118.806796597377, 34.0215816298725], [-118.791432890735, 34.0163883241613], [-118.79596686535, 34.008564864635], [-118.808558110679, 34.0035027131376], ] ] } ) polygon_attributes = {"name": "Polygon", "description": "I am a Polygon"} simple_fill_symbol = { "type": "esriSFS", "color": [50, 100, 200, 125], "outline": {"color": [255, 255, 255], "width": 1}, } map = portal.map() map
-
Use the
draw
method to add each of the graphics, with their associated symbology and attributes, to the map. Use thepopup
parameter to build a popup object from attribute values.Use dark colors for code blocks map = portal.map() map map.draw( shape=point, symbol=simple_marker_symbol, attributes=point_attributes, popup={ "title": point_attributes["name"], "content": point_attributes["description"], }, ) map.draw( shape=polyline, symbol=simple_line_symbol, attributes=polyline_attributes, popup={ "title": polyline_attributes["name"], "content": polyline_attributes["description"], }, ) map.draw( shape=polygon, symbol=simple_fill_symbol, attributes=polygon_attributes, popup={ "title": polygon_attributes["name"], "content": polygon_attributes["description"], }, )
-
Set the map center by passing in coordinates and provide a scale level. This will update the map extent so that the newly added graphics are displayed prominently in the view.
Use dark colors for code blocks map.draw( shape=point, symbol=simple_marker_symbol, attributes=point_attributes, popup={ "title": point_attributes["name"], "content": point_attributes["description"], }, ) map.draw( shape=polyline, symbol=simple_line_symbol, attributes=polyline_attributes, popup={ "title": polyline_attributes["name"], "content": polyline_attributes["description"], }, ) map.draw( shape=polygon, symbol=simple_fill_symbol, attributes=polygon_attributes, popup={ "title": polygon_attributes["name"], "content": polygon_attributes["description"], }, ) map.center = [34.0110, -118.8047] map.zoom = 14
-
Optional: Use the
export_
method to export the current state of the map widget to a static HTML file which can be viewed in any web browser.t o_ html Use dark colors for code blocks map.center = [34.0110, -118.8047] map.zoom = 14 import os file_dir = os.path.join(os.getcwd(), "home") if not os.path.isdir(file_dir): os.mkdir(file_dir) file_path = os.path.join(file_dir, "add-a-point-line-and-polygon.html") map.export_to_html(file_path, title="Add a point, line, and polygon")
Your map should display three graphics in the Santa Monica Mountains. Click on a graphic to display its popup.
What's next?
Learn how to use additional functionality in these tutorials:
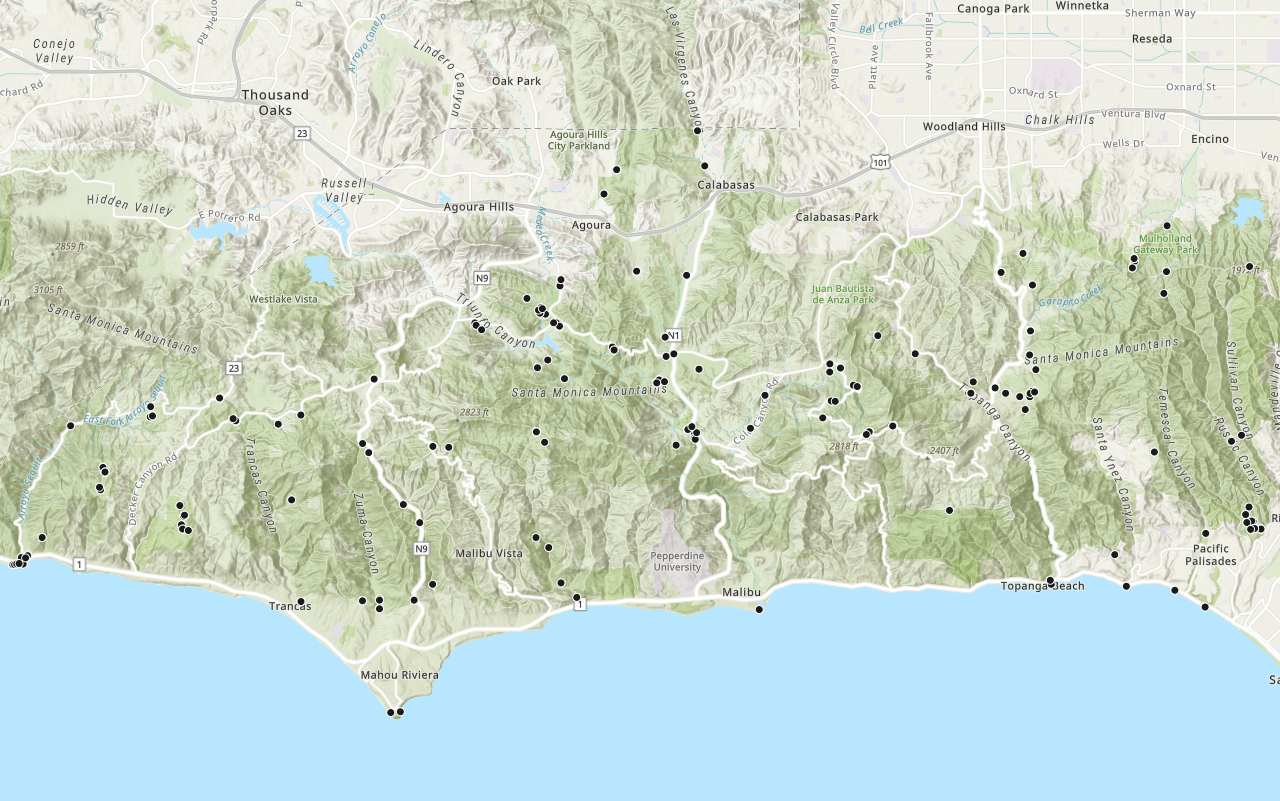
Add a layer from a portal item
Learn how to use a portal item to access and display point features from a feature service.
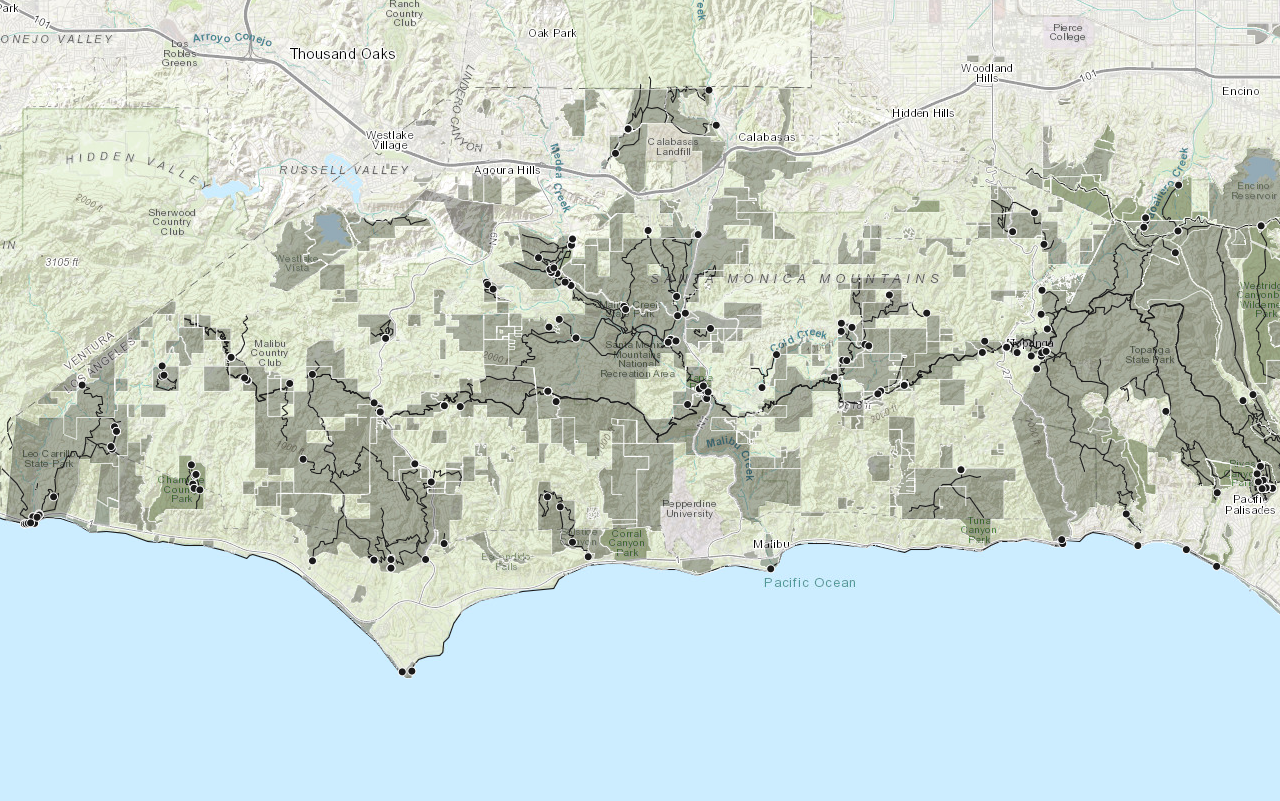
Add a feature layer
Learn how to access and display point, line, and polygon features from a feature service.
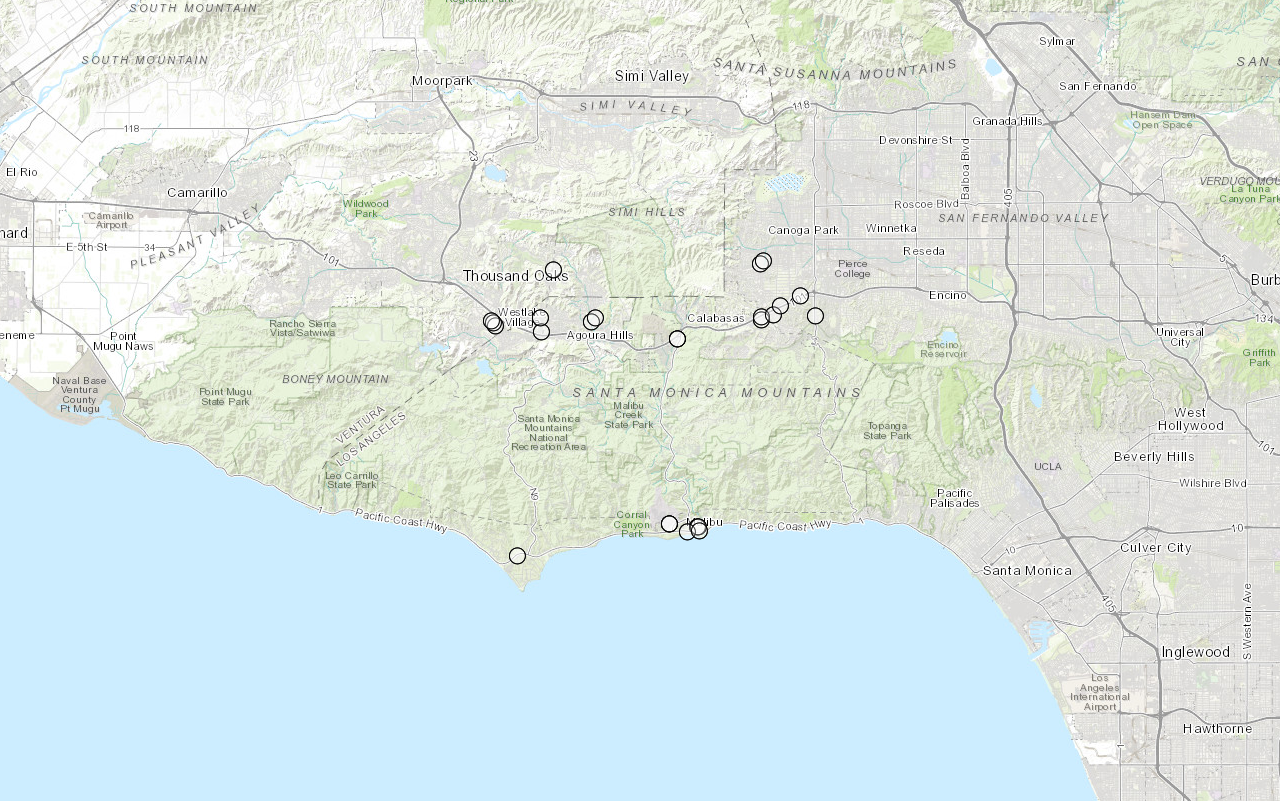
Find place addresses
Search for places such as coffee shops, gas stations, or restaurants.
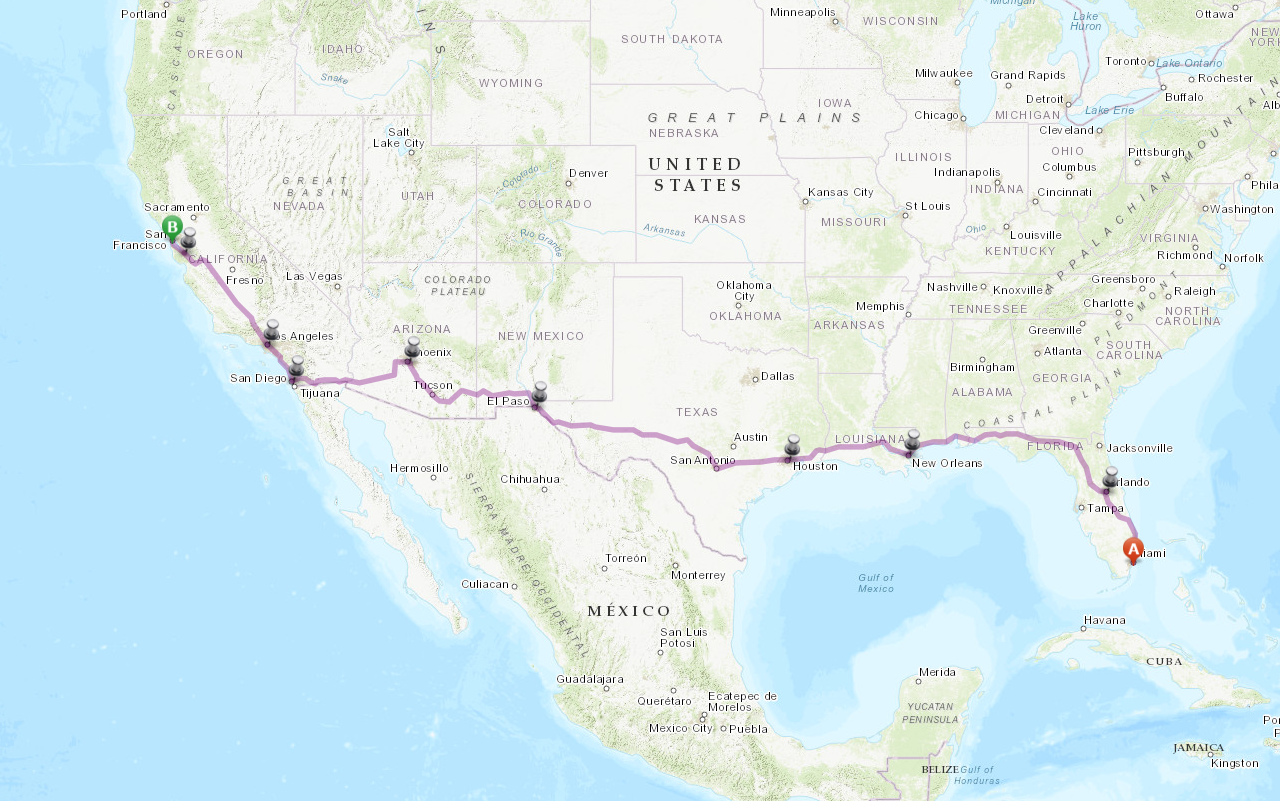
Find a route
Guide: Learn how to find a route and directions between locations with the routing service.