Learn how to use the ArcGIS API for Python and the basemap styles service to display a map.
A map contains layers of geographic data. A map contains a basemap layer and, optionally, one or more data layers. You can display a specific area of a map using a map view and setting the location and zoom level.
This tutorial shows you how to create and display a map of the Santa Monica Mountains in California using the topographic basemap layer.
Prerequisites
The ArcGIS API for Python tutorials use Jupyter Notebooks to execute Python code. If you are new to this environment, please see the guide to install the API and use notebooks locally.
Steps
Get an API key
To access location services, you need an API key, OAuth 2.0 access token, or ArcGIS Online account. To learn how to create and scope your key, visit the Create an API key tutorial.
- Go to your developer dashboard to get an API key.
- Copy the key as it will be used in a following step.
Import modules and log in
-
Import the
arcgis.gis
module. This module is the entry point into the GIS and provides the functionality to manage GIS content, users, and groups.Use dark colors for code blocks from arcgis.gis import GIS
-
Use the
GIS
class to log in. ReplaceYOUR_
with the API key you previously copied from the developer dashboard as a value to theAPI_ KEY api_
parameter.key Use dark colors for code blocks from arcgis.gis import GIS gis = GIS(api_key="YOUR_API_KEY")
Display the map
-
Create an instance of the map widget and set the basemap.
Use dark colors for code blocks gis = GIS(api_key="YOUR_API_KEY") map = gis.map() map.basemap = "topo-vector"
-
Set the map center and zoom level.
Use dark colors for code blocks map = gis.map() map.basemap = "topo-vector" map.center = [34.027, -118.805] map.zoom = 13 map # display the map
-
Optional: Use the
export_
method to export the current state of the map widget to a static HTML file which can be viewed in any web browser.t o_ html Use dark colors for code blocks from os import path, getcwd export_dir = path.join(getcwd(), "home") if not path.isdir(export_dir): os.mkdir(export_dir) export_path = path.join(export_dir, "display-a-map.html") map.export_to_html(export_path, title="Display a map")
You should see a map with the topographic basemap layer centered on the Santa Monica Mountains in California.
What's next?
Learn how to use additional functionality in these tutorials:
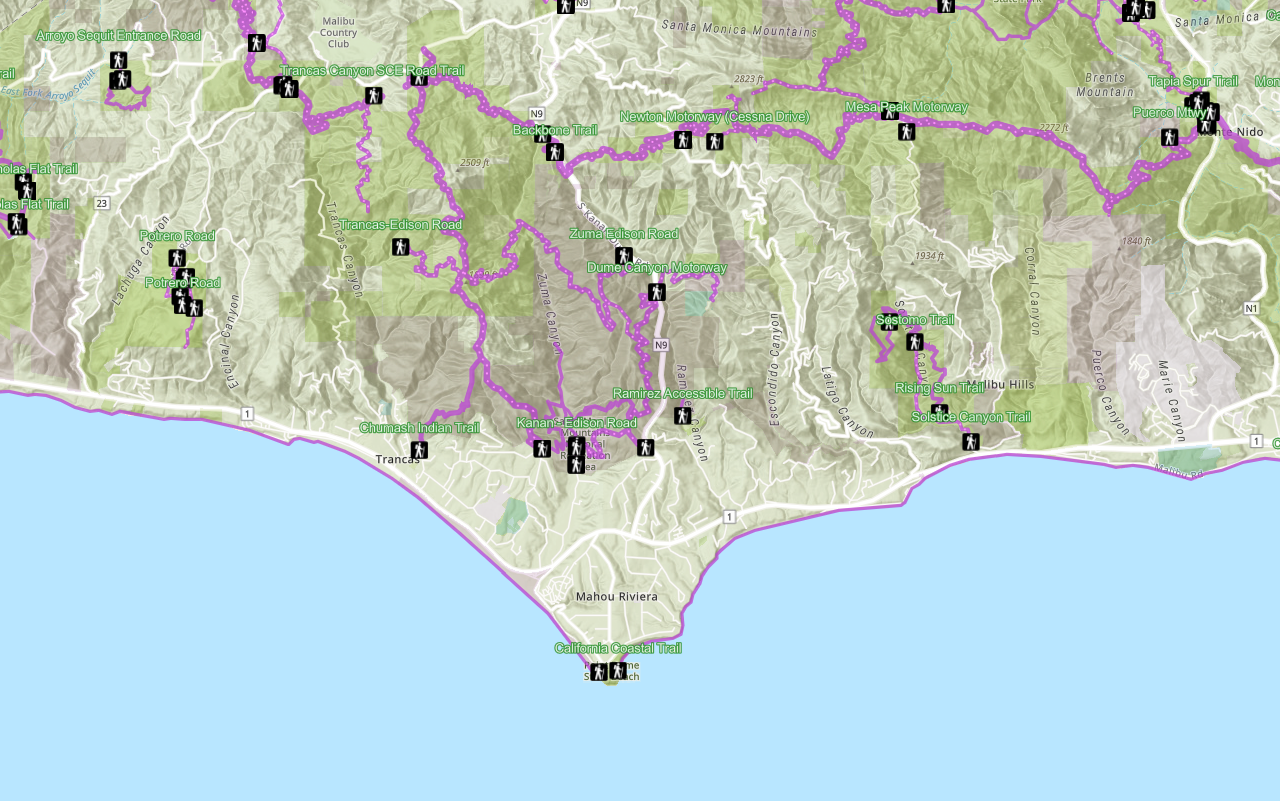
Display a web map
Search ArcGIS Online for an existing web map and display it.
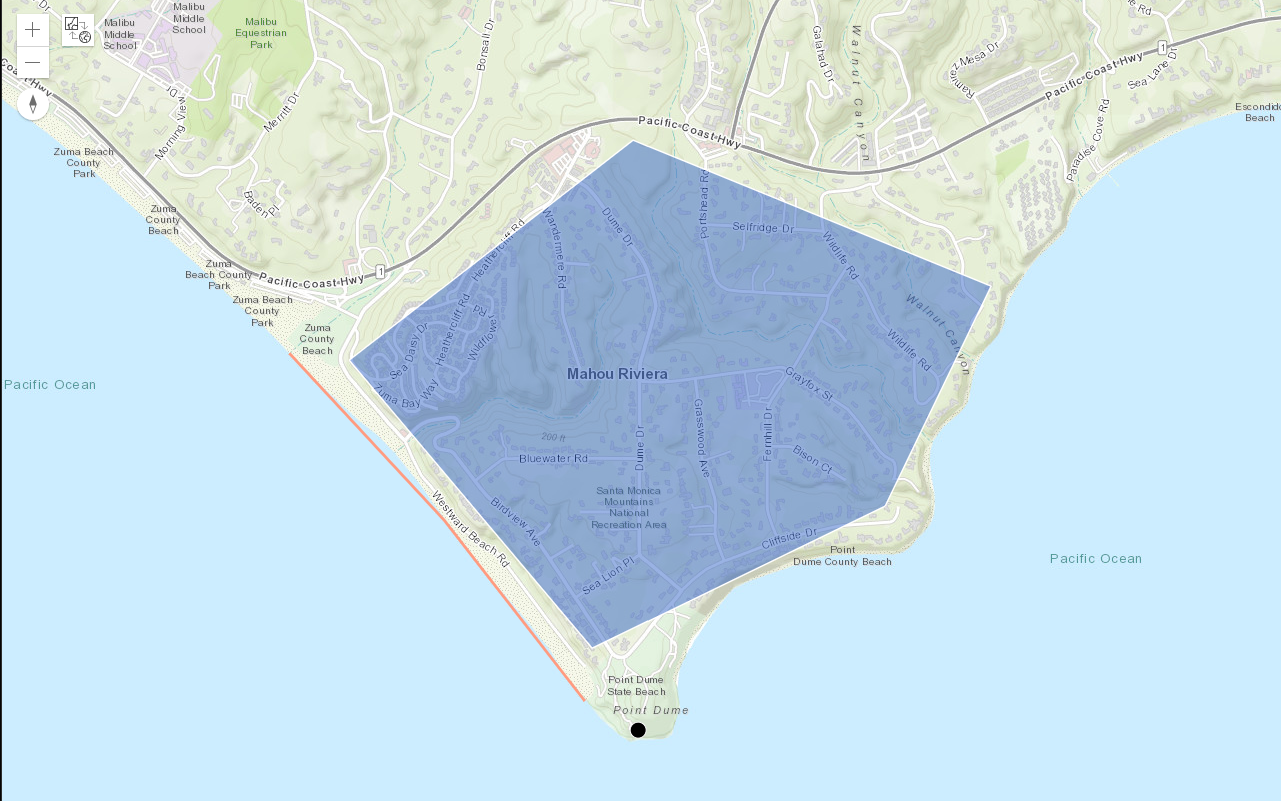
Add a point, line, and polygon
Learn how to display point, line, and polygon graphics in a map.
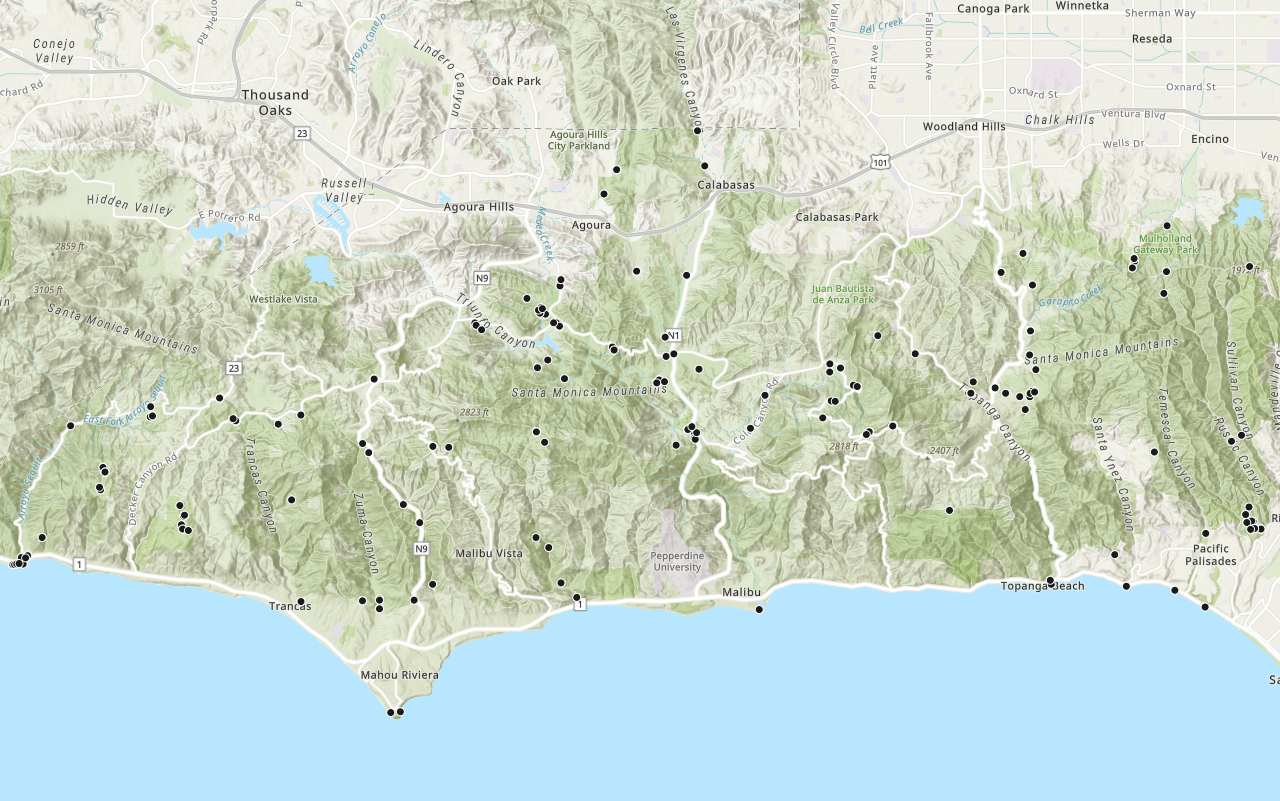
Add a layer from a portal item
Learn how to use a portal item to access and display point features from a feature service.
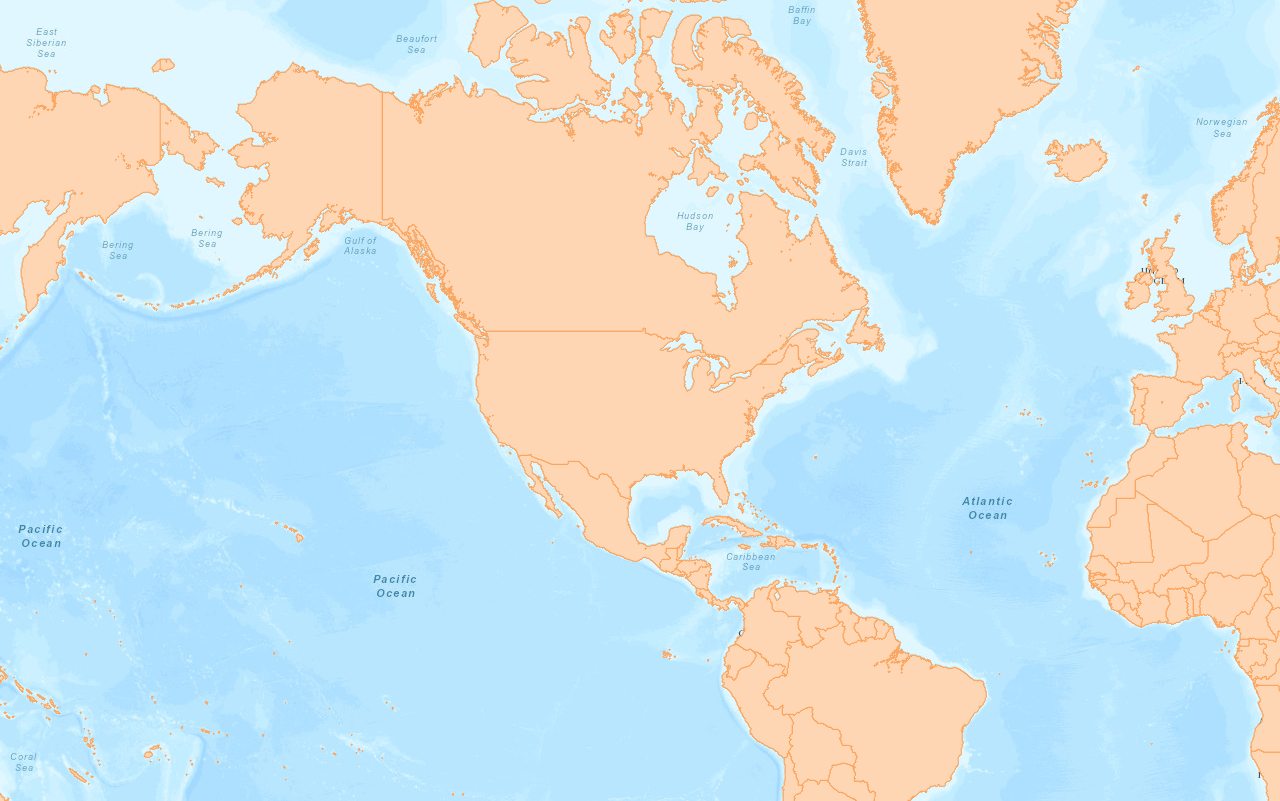
Save a web map
Guide: Learn how to save a map as a web map in ArcGIS.