Learn how to customize an existing story in ArcGIS StoryMaps using Python.
The ArcGIS API for Python provides a set of tools and functionalities to interact with ArcGIS Online, ArcGIS Enterprise, and ArcGIS StoryMaps.
In this tutorial, you use the Story
object to update the title and summary of a story and share it publicly.
Prerequisites
Steps
1. Install the ArcGIS API for Python
If you have not done this, you will need to install the ArcGIS API for Python library. You can do this by running the following command in your Python environment:
pip install arcgis
If you have Python 3.x.x installed, run the following command:
pip3 install arcgis
2. Authenticate and connect to ArcGIS Online
To access and customize your ArcGIS StoryMaps, you need to authenticate and connect to your ArcGIS Online account. You can do this by creating an instance of the GIS class and providing your login credentials:
from arcgis.gis import GIS
# Create a GIS object and authenticate
gis = GIS("https://www.arcgis.com", "username", "password")
3. Retrieve and modify a story
Once you are connected, you can retrieve a story by its ID or search for it using specific criteria. For example, to retrieve a story by its ID:
# Retrieve a story by its ID
storymap_id = "your_storymap_id"
storymap = gis.content.get(storymap_id)
4. Customize the story
You can customize various aspects of the story, such as its title, summary, cover image, layout, and content. Here is an example of updating the title and summary:
# Update the title and summary of the story
new_title = "New Title"
new_summary = "This is a customized story created using Python."
storymap.update(item_properties={'title': new_title, 'snippet': new_summary})
You can explore the item_
dictionary to see other available properties you can modify.
5. Save and publish the changes
After making the desired modifications, you need to save and publish the changes to apply them to the story:
# Save and publish the changes
storymap.save()
storymap.share(everyone=True) # Optionally, make the story public
You can adjust the sharing settings according to your requirements.
These are the basic instructions to get started with customizing ArcGIS StoryMaps using ArcGIS API for Python. Please refer to the Python API documentation for more detailed information and examples to help you further explore and utilize its capabilities.
Tutorials
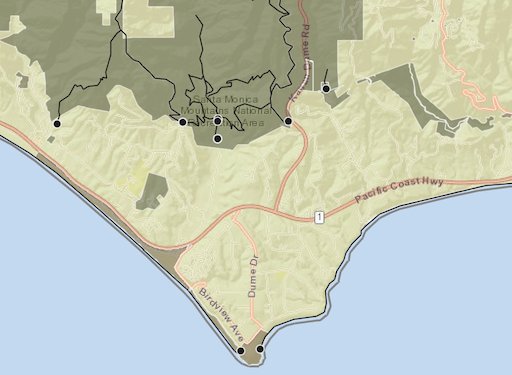
Create a web map
Use Map Viewer to create a web map for your application.
ArcGIS Online Map Viewer
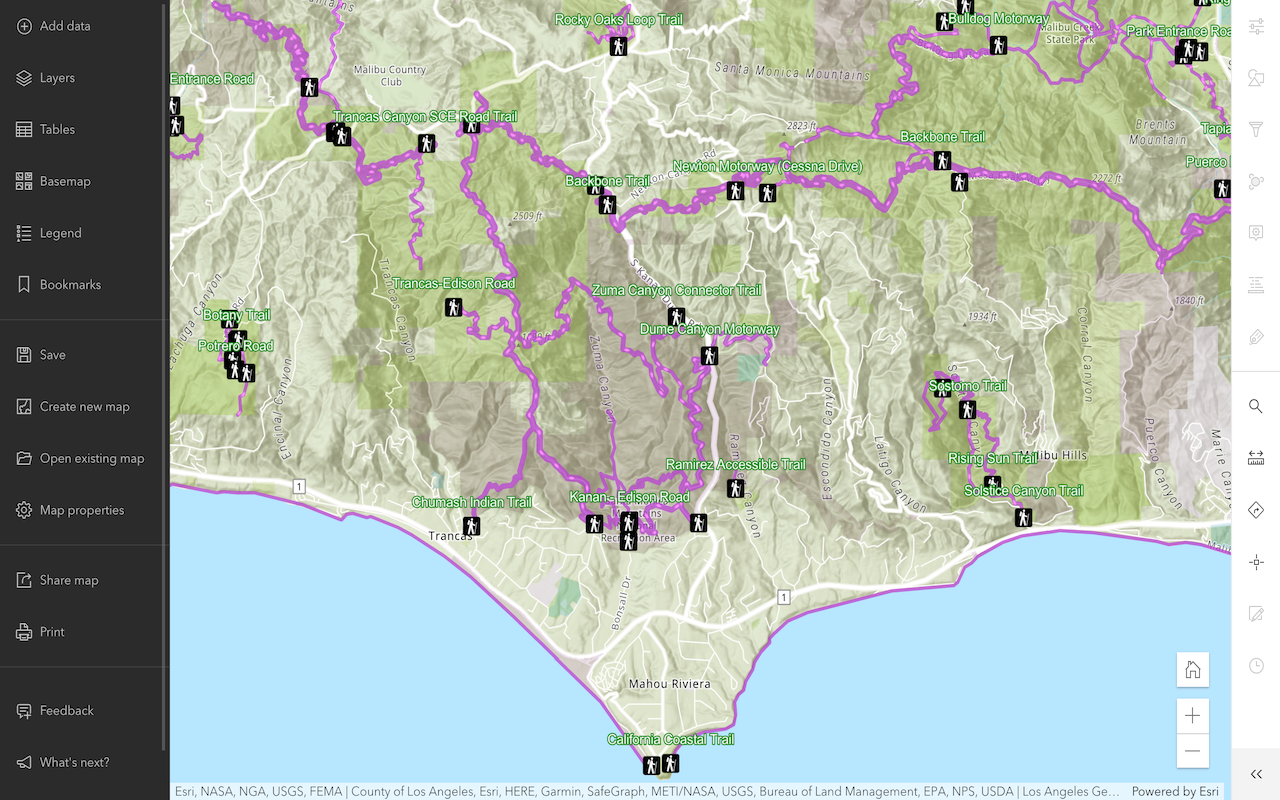
Style layers in a web map
Use Map Viewer to style layers in a web map.
ArcGIS Online Map Viewer
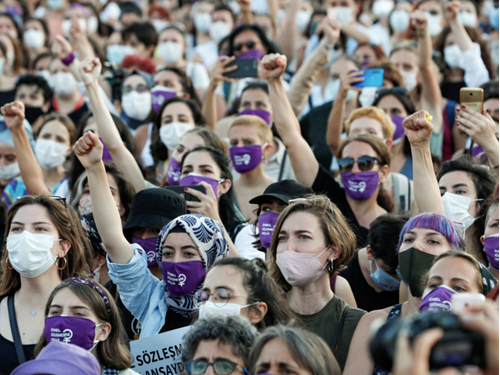
Customize a theme for impactful stories
Recreate an award-winning theme from the 2020 ArcGIS StoryMaps Competition to get to know the capabilities of the StoryMaps theme builder.
ArcGIS Online Location Platform dashboard
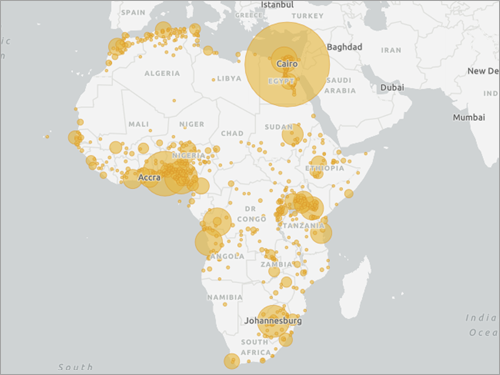
Build a sidecard in your story
Use a sidecar to allow others to access your mapped data one step at a time.
ArcGIS Online Location Platform dashboard