Learn how to display feature attributes in a pop-up.
A pop-up, also known as a "popup", is a visual element that displays information about a feature when it is clicked. You typically style and configure a pop-up using HTML and CSS for each layer in a map. Pop-ups can display attribute values, calculated values, or rich content such as images, charts, or videos.
In this tutorial, you create an interactive pop-up for the Trailheads feature layer in the Santa Monica Mountains. When a feature is clicked, a pop-up is displayed containing the name of the trail and the service that manages it. You use the ol-popup library to achieve this.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Item access
- Note: If you are using your own custom data layer for this tutorial, you need to grant the API key credentials access to the layer item. Learn more in Item access privileges.
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapId = "arcgis/streets"; const basemapURL = `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapId}?token=${accessToken}`; olms.apply(map, basemapURL);
To learn about the other types of authentication available, go to Types of authentication.
Add the pop-up library
OpenLayers does not include a pop-up component. You can use the third-party library ol-popup to simplify adding pop-ups to your map.
-
In the
<head>
element, add references to the ol-popup library.Use dark colors for code blocks <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/ol@v8.2.0/ol.css" type="text/css" /> <script src="https://cdn.jsdelivr.net/npm/ol@v8.2.0/dist/ol.js"></script> <script src="https://cdn.jsdelivr.net/npm/ol-mapbox-style@10.6.0/dist/olms.js" type="text/javascript"></script> <script src="https://unpkg.com/ol-popup@5.1.0/dist/ol-popup.js"></script> <link rel="stylesheet" href="https://unpkg.com/ol-popup@5.1.0/src/ol-popup.css" />
Add the trailheads layer
Use a Vector
layer with a Vector
source to display the trailheads as GeoJSON.
For more information about adding feature layers using GeoJSON, see the Add a feature layer as GeoJSON tutorial.
-
Before the
olms
initialization, create a aVector
layer. Give it aVector
source with a GeoJSON feature format to load and display the trailheads feature layer. Save it to atrailheads
variable.Layer Use dark colors for code blocks const basemapId = "arcgis/streets"; const trailheadsLayer = new ol.layer.Vector({ source: new ol.source.Vector({ format: new ol.format.GeoJSON(), url: `https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads/FeatureServer/0/query?where=1%3D1&outFields=*&returnGeometry=true&f=pgeojson` }) });
-
Add a load handler to the
olms
initialization. Inside, Usemap.add
to add this layer to the map.Layer Use dark colors for code blocks olms.apply(map, `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapId}?token=${accessToken}`) .then(function (map) { map.addLayer(trailheadsLayer); });
Initialize the pop-up
A Popup
is a type of Overlay
. Use map.add
to add it to the map.
-
Create a new
Popup
and save it to apopup
variable. Add it to the map withmap.add
.Overlay Use dark colors for code blocks .then(function (map) { map.addLayer(trailheadsLayer); }); const popup = new Popup(); map.addOverlay(popup);
Display a pop-up on click
A pop-up can display any HTML content. Use a click
event handler to detect the click, then use map.get
to find any trailhead features at the clicked point. You pass a function as a layer
to do so.
If the returned array contains at least one feature, you can use Feature.get
to extract the trail name (TRL_
) and park name (PARK_
). You then use popup.show
to update the pop-up position and content.
-
Add a
click
event handler. Inside, useget
to get an array of trailhead features clicked on.Features At Pixel Use dark colors for code blocks const popup = new Popup(); map.addOverlay(popup); map.on("click", (event) => { let trailName, parkName; const trailheads = map.getFeaturesAtPixel(event.pixel, { layerFilter: (layer) => layer === trailheadsLayer }); });
-
If there is a trail name to show, use
popup.show
to set the position and content of the pop-up. Otherwise, usepopup.hide
to hide it.Use dark colors for code blocks const trailheads = map.getFeaturesAtPixel(event.pixel, { layerFilter: (layer) => layer === trailheadsLayer }); if (trailheads.length > 0) { const trailName = trailheads[0].get("TRL_NAME"); const parkName = trailheads[0].get("PARK_NAME"); popup.show(event.coordinate, `<b>${trailName}</b></br>${parkName}`); } else { popup.hide(); }
Run the app
In CodePen, run your code to display the map.
The map view should display the Trailheads feature layer. When you click a feature, the name of the trailhead and its park name is displayed in a pop-up.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
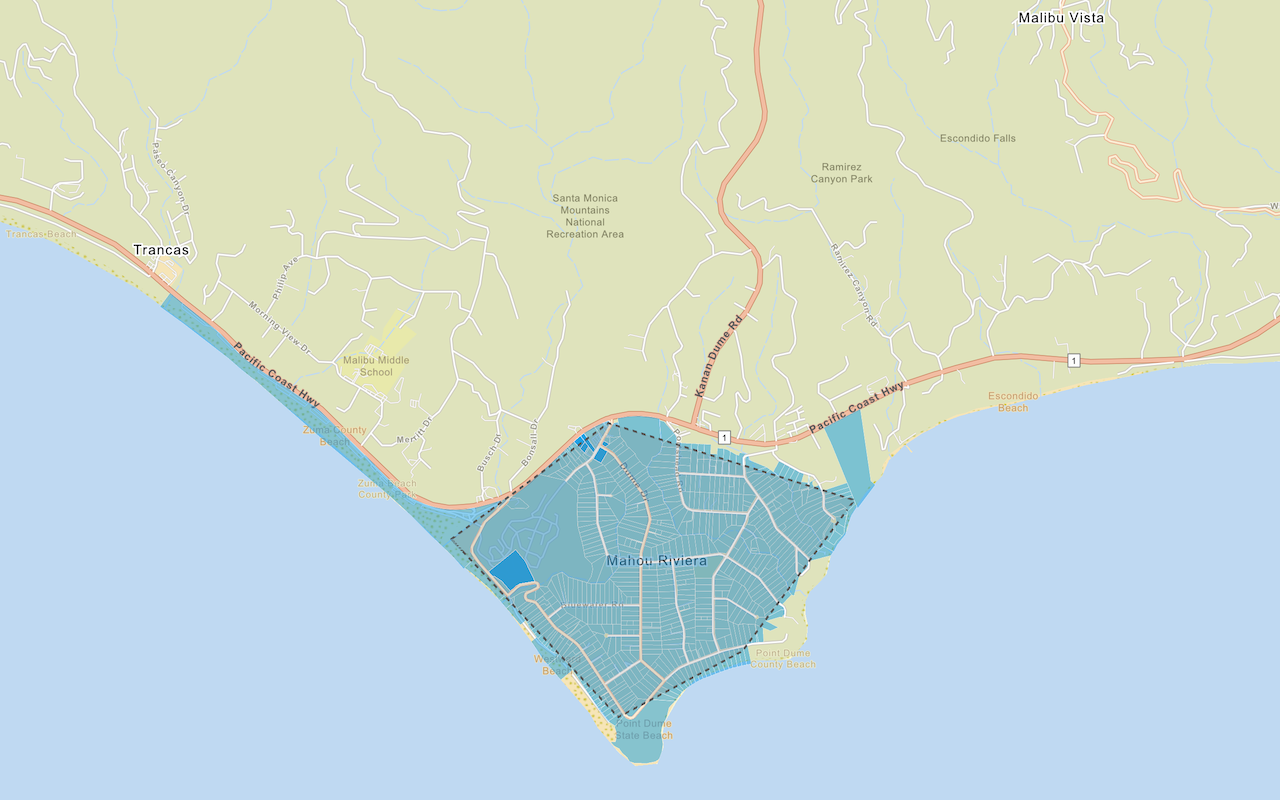
Query a feature layer (spatial)
Execute a spatial query to access polygon features from a feature service.
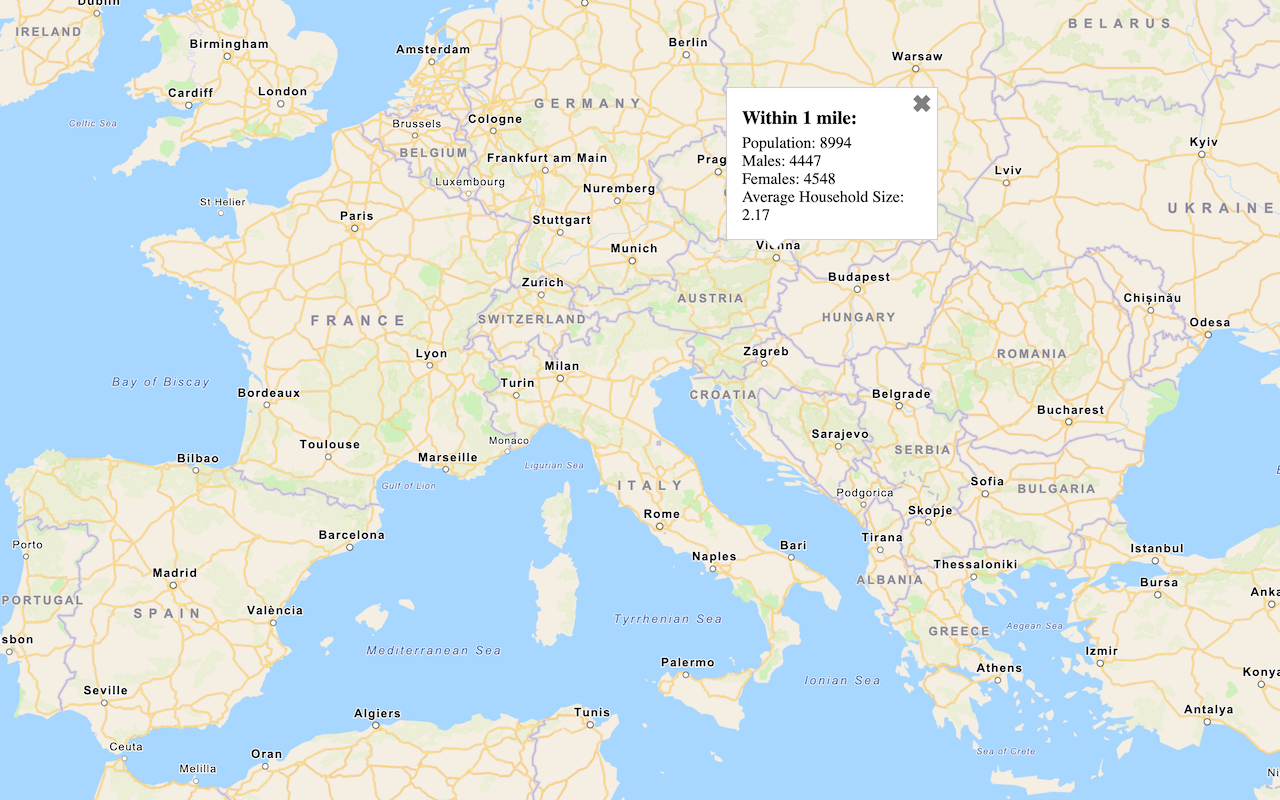
Get global data
Query demographic information for locations around the world with the GeoEnrichment service.
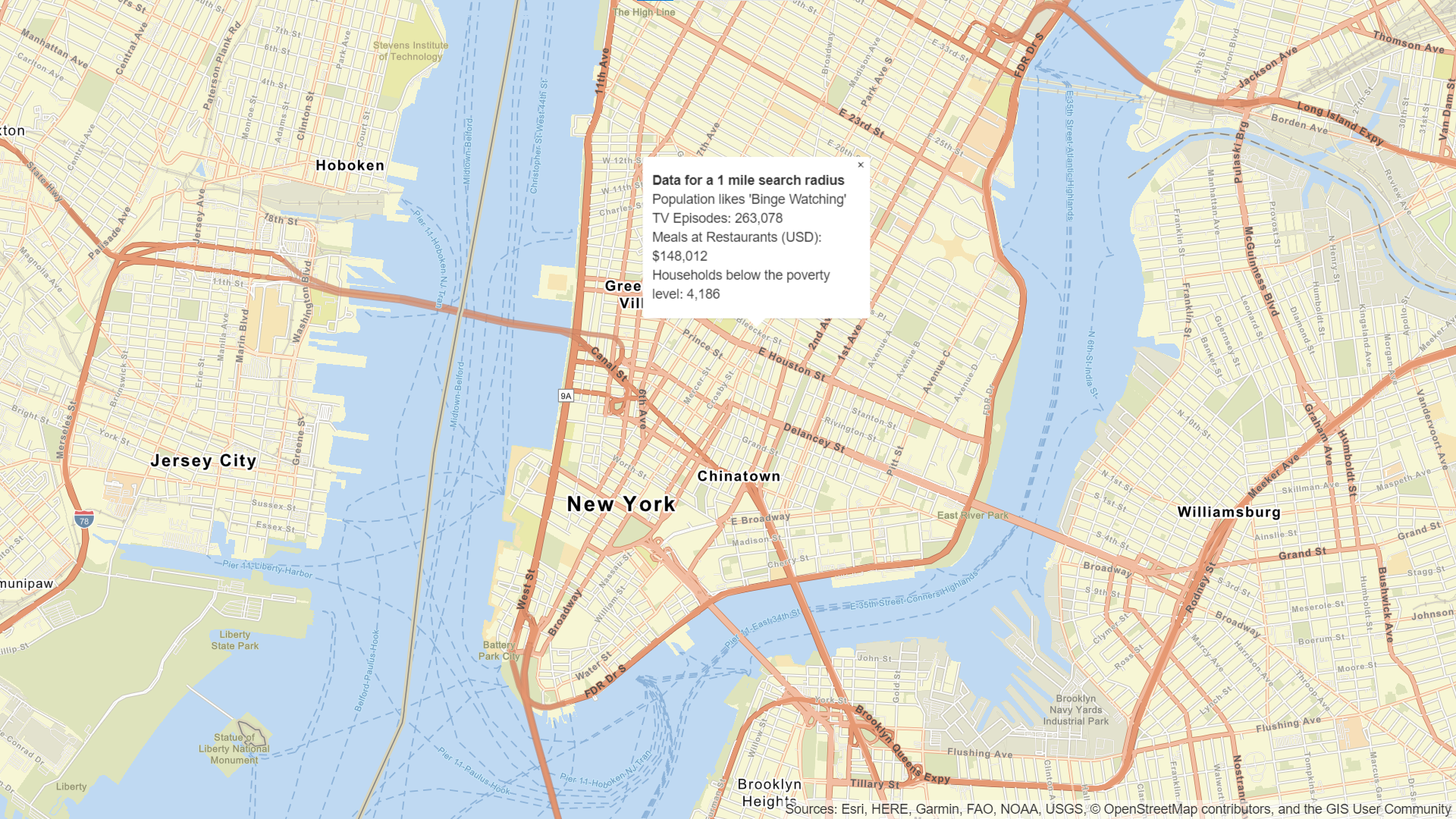
Get local data
Query regional facts, spending trends, and psychographics with the GeoEnrichment service.
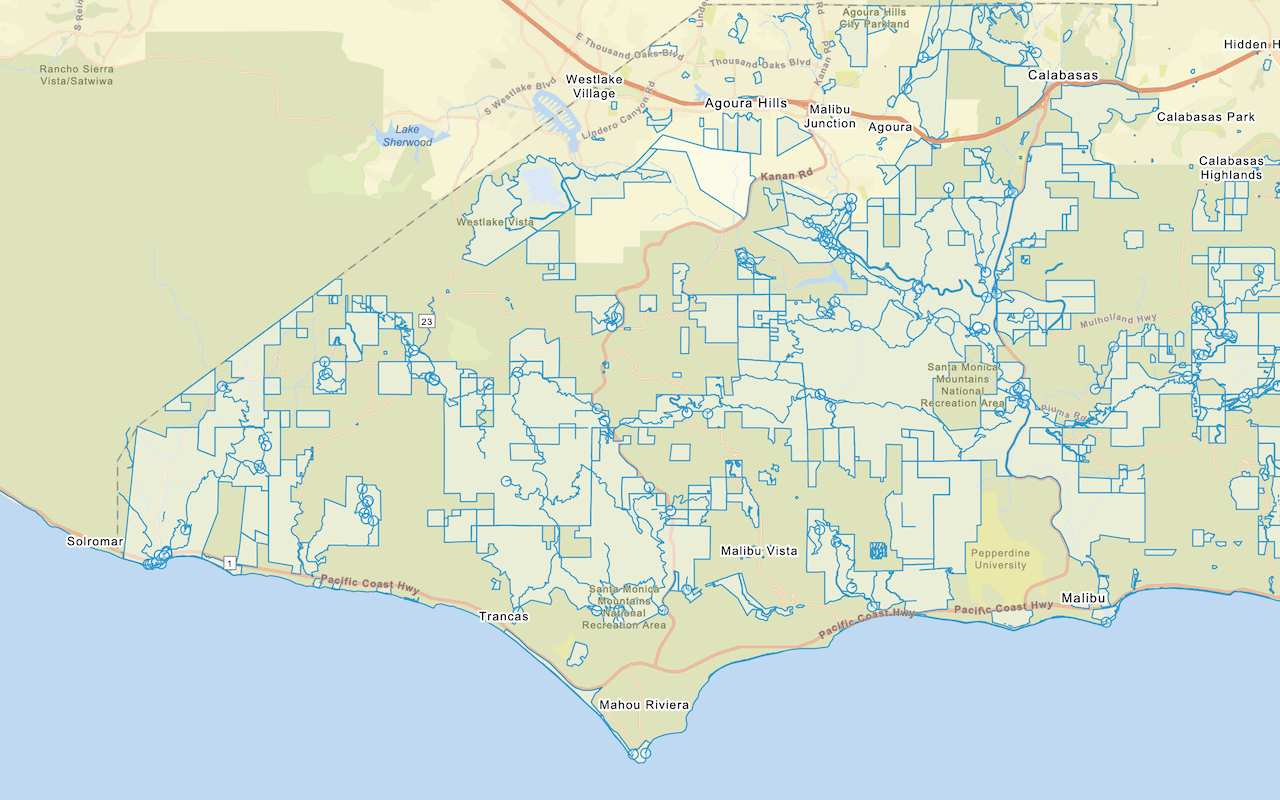
Add a feature layer
Add features from feature layers to a map.