Learn how to find an address near a location with the geocoding service.
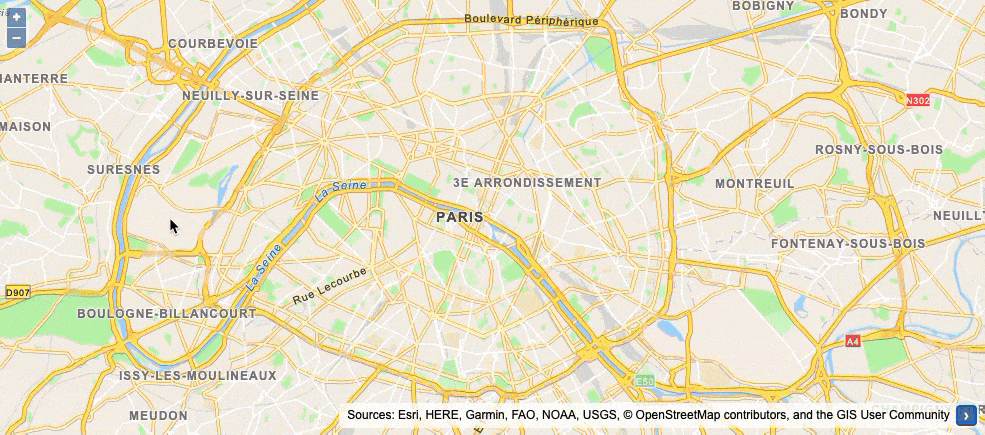
Reverse geocode coordinates to addresses with the geocoding service.
Reverse geocoding is the process of converting a location to an address or place. To reverse geocode, you use the Geocoding service and the reverse
operation. This operation requires an initial location and returns an address with attributes such as place name and location.
In this tutorial, you use the reverse
method of ArcGIS REST JS to reverse geocode and find the closest address to your clicked location on the map.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapId = "arcgis/streets"; const basemapURL = `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapId}?token=${accessToken}`; olms.apply(map, basemapURL);
To learn about the other types of authentication available, go to Types of authentication.
Add references
This tutorial uses ArcGIS REST JS for reverse geocoding, and the ol-popup library to display pop-ups.
-
In the
<head>
element, add references to the ArcGIS REST JS and ol-popup libraries.Use dark colors for code blocks <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/ol@v8.2.0/ol.css" type="text/css" /> <script src="https://cdn.jsdelivr.net/npm/ol@v8.2.0/dist/ol.js"></script> <script src="https://cdn.jsdelivr.net/npm/ol-mapbox-style@10.6.0/dist/olms.js" type="text/javascript"></script> <script src="https://unpkg.com/@esri/arcgis-rest-request@4.0.0/dist/bundled/request.umd.js"></script> <script src="https://unpkg.com/@esri/arcgis-rest-geocoding@4.0.0/dist/bundled/geocoding.umd.js"></script> <script src="https://unpkg.com/ol-popup@5.1.0/dist/ol-popup.js"></script> <link rel="stylesheet" href="https://unpkg.com/ol-popup@5.1.0/src/ol-popup.css" />
Update the map
A navigation basemap layer is typically used in geocoding and routing applications. Update the basemap layer to use arcgis/navigation
.
-
Update the basemap and the map initialization to center on location
[2.3522,48.8566]
, Paris.Use dark colors for code blocks <script> const accessToken = "YOUR_ACCESS_TOKEN"; const map = new ol.Map({ target: "map" }); const view = new ol.View({ center: ol.proj.fromLonLat([2.3522, 48.8566]), // Paris zoom: 12 }); map.setView(view); const basemapId = "arcgis/navigation"; olms.apply(map, `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapId}?token=${accessToken}`); </script>
Add a pop-up
Create a Popup
to display the results of the reverse geocode. It is a type of Overlay
so you add it to the map with map.add
.
-
Create a
Popup
and save it to apopup
variable. Add it to the map withmap.add
.Overlay Use dark colors for code blocks const view = new ol.View({ center: ol.proj.fromLonLat([2.3522, 48.8566]), // Paris zoom: 12 }); map.setView(view); const popup = new Popup(); map.addOverlay(popup);
Add click event handler
Before you call the reverse geocoding service, you need the location of the clicked point. Use ol.proj.transform
to convert this into a latitude and longitude.
-
Add a click handler to the map. Inside, convert the clicked coordinates from the event object into latitude and longitude.
Use dark colors for code blocks const popup = new Popup(); map.addOverlay(popup); map.on("click", (e) => { const coords = ol.proj.transform(e.coordinate, "EPSG:3857", "EPSG:4326"); });
Call reverse geocode service
Use the ArcGIS REST JS reverse
method to find an address closest to a point.
-
Inside the click handler, create a new
arcgis
to access the geocoding service. Call theRest.Api K e y Manager arcgis
method with the coordinates array.Rest.reverse Geocode Use dark colors for code blocks const coords = ol.proj.transform(e.coordinate, "EPSG:3857", "EPSG:4326"); const authentication = arcgisRest.ApiKeyManager.fromKey(accessToken); arcgisRest .reverseGeocode(coords, { authentication })
Display the result
The response from the reverse
operation contains two properties. address
is a structured object with fields such as street name and business name. location
contains the location of the returned address, which may differ from the coordinates you provided. Display these values in a pop-up. Use a catch
handler to check for errors accessing the service.
-
Add a
then
handler. Usepopup.show
to display the resulting address and coordinates.Use dark colors for code blocks arcgisRest .reverseGeocode(coords, { authentication }) .then((result) => { const message = `${result.address.LongLabel}<br>` + `${result.location.x.toLocaleString()}, ${result.location.y.toLocaleString()}`; popup.show(e.coordinate, message); })
-
Add a
catch
handler. In most cases, errors occur when the user clicks in the water, so simply hide the pop-up when this happens. Usepopup.hide()
to remove the pop-up and then write the error to the console.Use dark colors for code blocks popup.show(e.coordinate, message); }) .catch((error) => { popup.hide(); console.error(error); });
Run the app
In CodePen, run your code to display the map.
Click on the map to reverse geocode the clicked point and display a pop-up with the closest address and coordinates.
What's next?
Learn how to use additional ArcGIS location services in these tutorials: