Learn how to display a map with a basemap layer using OpenLayers.
A map contains layers of geographic data. A map contains a basemap layer and, optionally, one or more data layers. You can display a specific area of a map by using a map view and setting the location and zoom level.
In this tutorial you will use OpenLayers to display a map of the Santa Monica Mountains in California using the topographic basemap layer.
This tutorial is the starting point for the other OpenLayers tutorials.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- Go to CodePen to create a new pen for your application.
Add HTML
Define an HTML page to create a map that is the full width and height of the browser window.
-
In CodePen > HTML, add HTML and CSS to create a page with a div element called
map
.Use the HTML to create a page with a map. Use the
map
div to display the map. Use the CSS to make the map full width and height.The
<!DOCTYPE html>
tag is not required in CodePen. If you are using a different editor or running the page on a local server, be sure to add this tag to the top of your HTML page.Use dark colors for code blocks <html> <head> <meta charset="utf-8" /> <meta name="viewport" content="initial-scale=1, maximum-scale=1, user-scalable=no" /> <title>OpenLayers Tutorials: Display a map</title> <style> html, body, #map { padding: 0; margin: 0; height: 100%; width: 100%; font-family: Arial, Helvetica, sans-serif; font-size: 14px; color: #323232; } </style> </head> <body> <div id="map"></div> </body> </html>
Load the libraries
You will need add <script>
and <link>
tags to load the JavaScript and CSS files for OpenLayers and ol-mapbox-style
.
-
In the
<head>
element, add references to the OpenLayers CSS and JavaScript library.Use dark colors for code blocks <style> html, body, #map { padding: 0; margin: 0; height: 100%; width: 100%; font-family: Arial, Helvetica, sans-serif; font-size: 14px; color: #323232; } </style> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/ol@v8.2.0/ol.css" type="text/css" /> <script src="https://cdn.jsdelivr.net/npm/ol@v8.2.0/dist/ol.js"></script>
-
Add a reference to the
ol-mapbox-style
library, which is required to load and style a vector tile layer using a vector tile style in OpenLayers.The additional library
ol-mapbox-style
is provided by the developers of OpenLayers. It used to display ArcGIS basemap styles. For more information, see the FAQ.Use dark colors for code blocks <script src="https://cdn.jsdelivr.net/npm/ol-mapbox-style@10.6.0/dist/olms.js"></script>
Create a map and view
Use the Map
and View
classes to create an OpenLayers map.
The OpenLayers Map
class displays the contents of the map and provides a user interface to interact with it. It supports clicking, zooming, panning, rotating, and tilting the perspective of the map. It also lets you interact with the visible contents of the map data, for example finding features at the mouse cursor. You can also use it to modify the data displayed by adding new sources or changing layer properties. OpenLayers takes care of automatically re-rendering as needed in response to changes made to layers.
For further details, see the OpenLayers documentation.
-
Add a
<script>
element in the<body>
element.Use dark colors for code blocks </head> <body> <div id="map"></div> <script> </script>
-
Use the
Map
class to create the map with options to control its display and behavior. Set thetarget
property to"map"
, which is the id of the div element.Use dark colors for code blocks <script> const map = new ol.Map({ target: "map" }); </script>
-
Create a
View
, and useset
to apply it to the map. To center the map view, set theView center
property to-118.80500,34.02700
and thezoom
property to13
.Use dark colors for code blocks <script> const map = new ol.Map({ target: "map" }); map.setView( new ol.View({ center: ol.proj.fromLonLat([-118.805, 34.027]), zoom: 12 }) ); </script>
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
- Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Privileges
- Copy the API key access token as it will be used in the next step.
To learn about other ways to get an access token, go to Types of authentication.
Add the basemap layer
OpenLayers does not directly support a vector basemap or a vector style file, so you will use the openlayers-mapbox-style
(olms
) JavaScript library to load a Mapbox style from the Basemap styles service and render it with OpenLayers.
The Mapbox style is JSON file that contains a reference to the vector tile layers used in the style, and visual styling rules to apply to one or more data layers in those tiles.
-
Store your API key as a variable. You will need to include this API key whenever you invoke ArcGIS services.
Use dark colors for code blocks map.setView( new ol.View({ center: ol.proj.fromLonLat([-118.805, 34.027]), zoom: 12 }) ); const accessToken = "YOUR_ACCESS_TOKEN";
-
Create a
basemap
variable and set it toI d arcgis/streets
.Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapId = "arcgis/streets";
-
Construct the basemap URL based on
basemap
and your API key.I d Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapId = "arcgis/streets"; const basemapURL = `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapId}?token=${accessToken}`;
-
Use
olms
to apply the basemap style to your map. Theolms
function takes two inputs, themap
element and the URL for a Mapbox basemap style file.Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapId = "arcgis/streets"; const basemapURL = `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/${basemapId}?token=${accessToken}`; olms.apply(map, basemapURL);
Run the app
In CodePen, run your code to display the map.
The map should display the topographic basemap layer for an area of the Santa Monica Mountains in California. Pan and zoom to explore the map.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
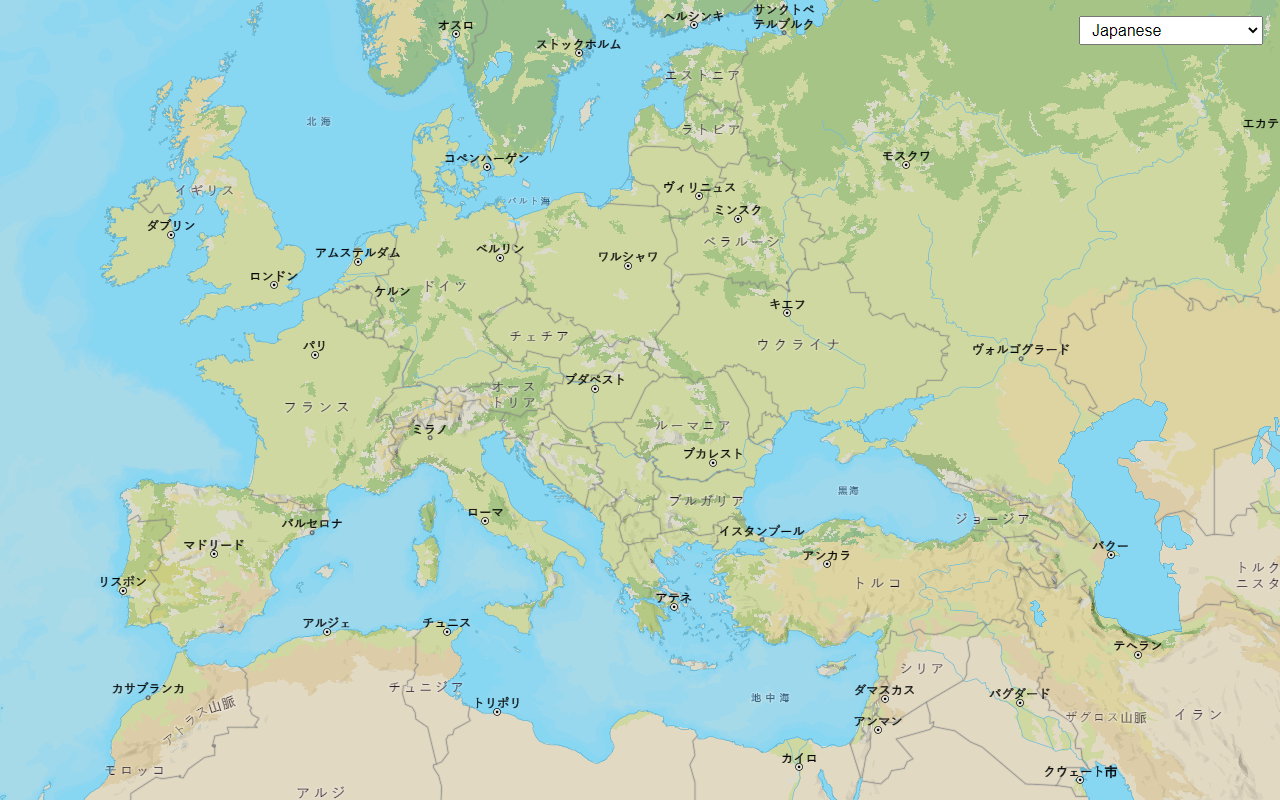
Change the place label language
Switch the language of place labels on a basemap.
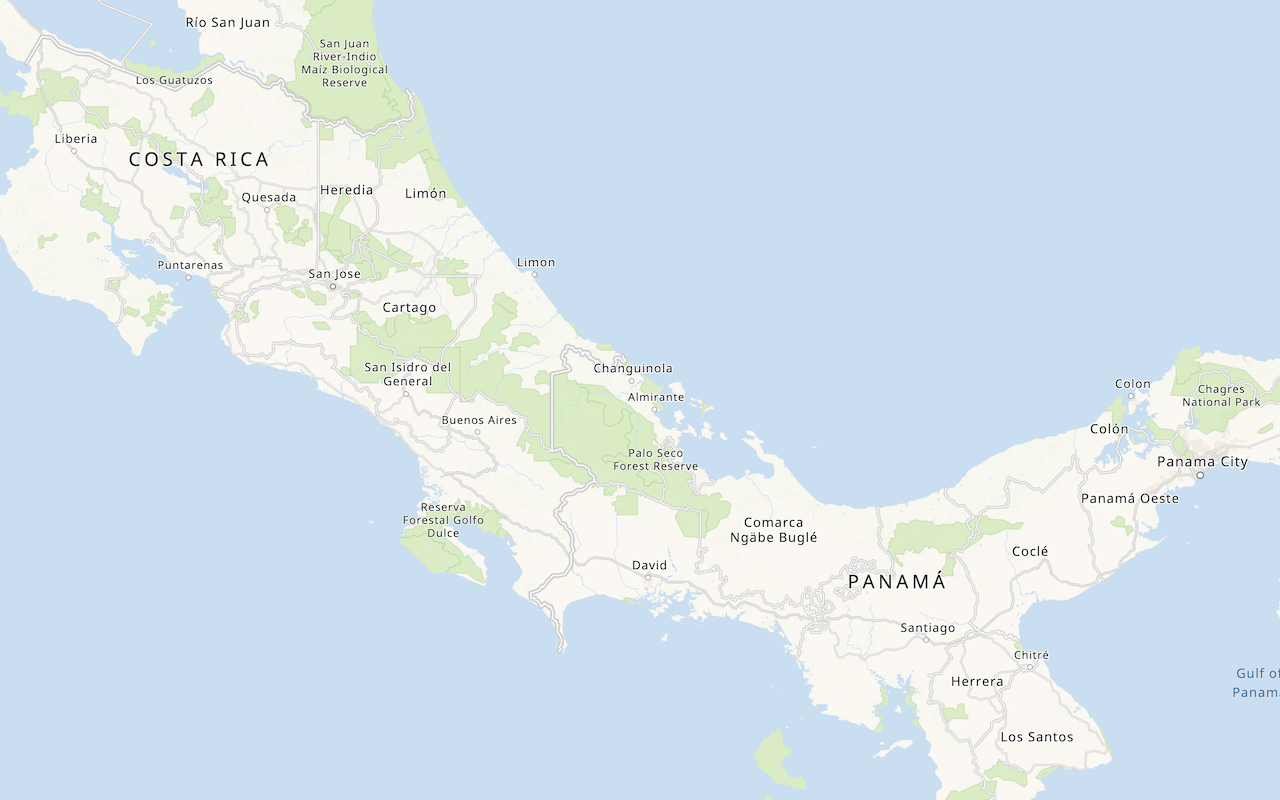
Display a custom basemap style
Add a styled vector basemap layer to a map.
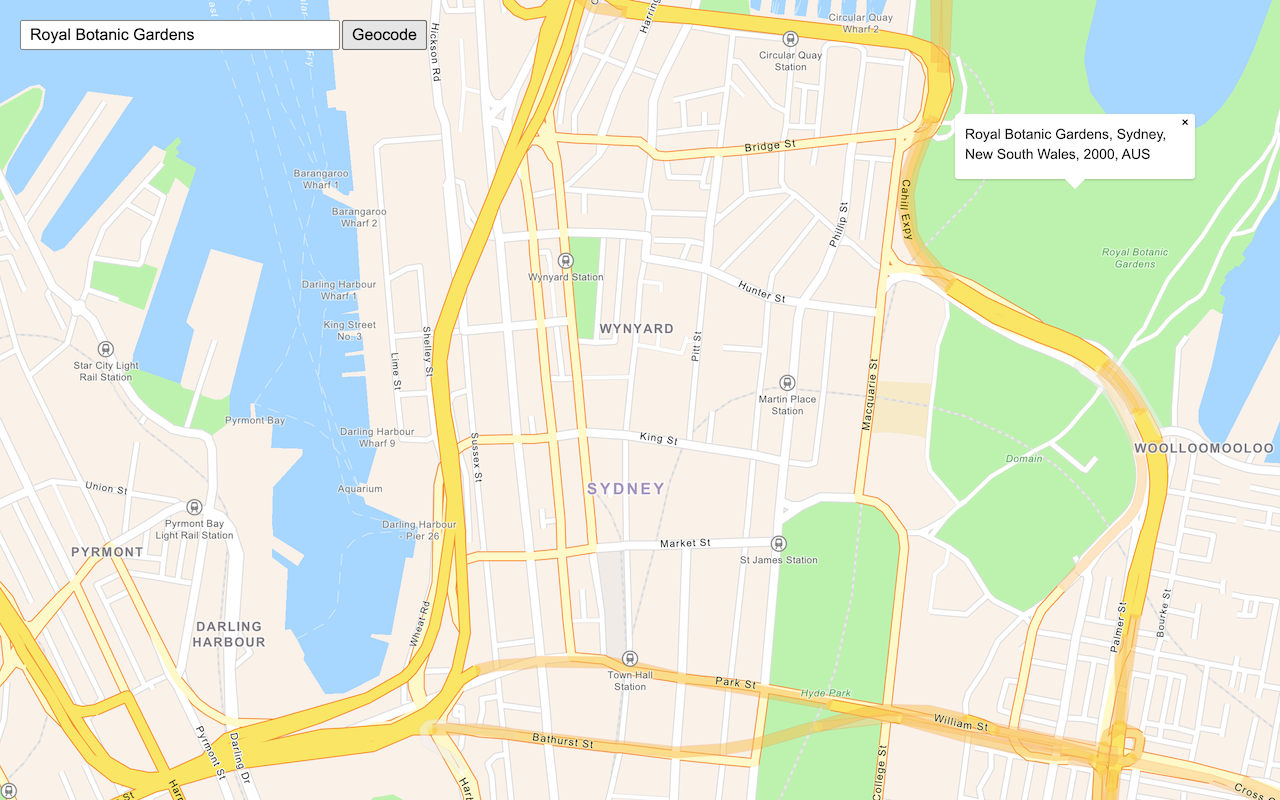
Search for an address
Find an address or place using a search box and the geocoding service.
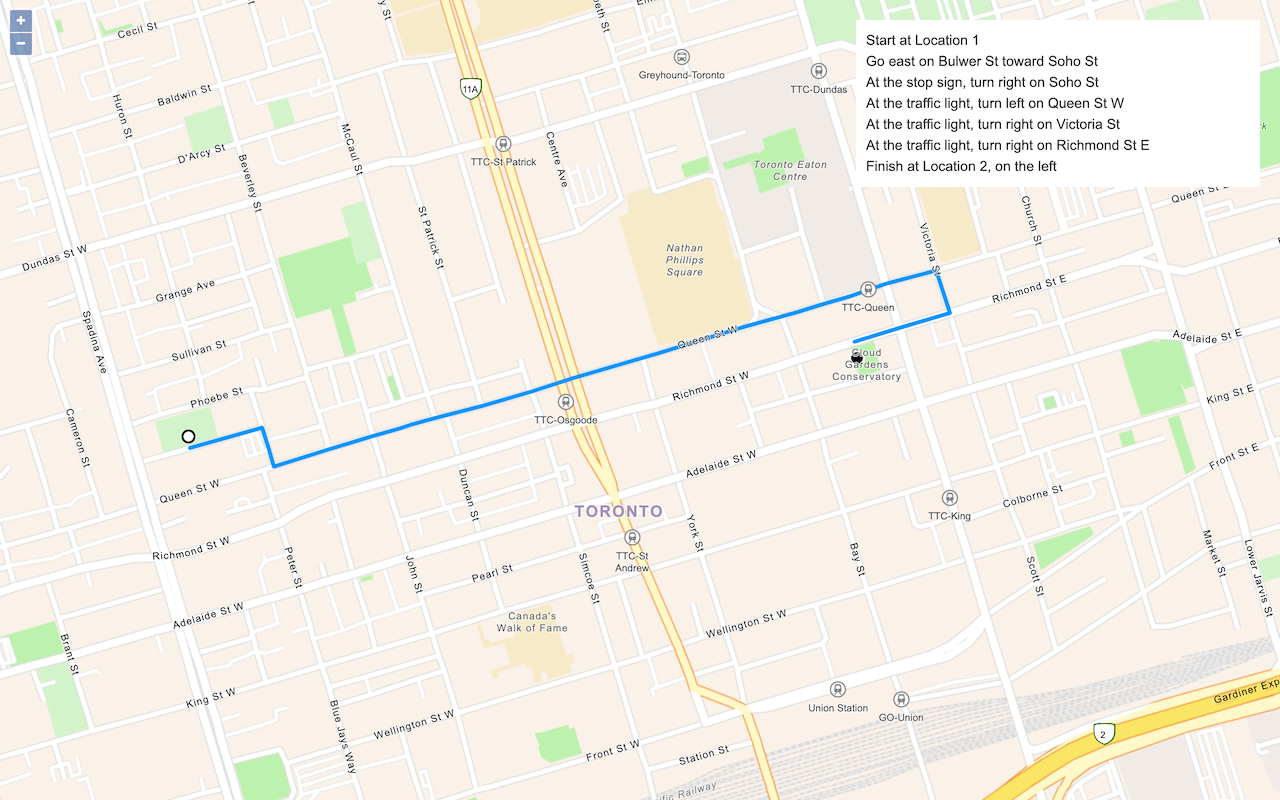
Find a route and directions
Find a route and directions with the route service.