ArcGIS Online is a cloud-based, collaborative content management system for maps, apps, data, and other geographic content. ArcGIS Online and the developer dashboard provide tools that you can use to store and manage data for your applications. You can import existing data, create new datasets, or convert data to new types of data. All data is managed and accessed as a hosted layer, and each layer is powered by a data service.
ArcGIS Online
With ArcGIS Online you can do things like:
- Create web maps
- Web enable your data
- Control how your maps, data, and applications are shared
- Find relevant and useful basemaps, data, and configurable GIS resources
- Manage content and users in your organization
Once hosted, you can access your data as services from your ArcGIS Runtime applications. You can also use ArcGIS Runtime to create new content (items), search for users and groups, and manage sharing.
ArcGIS for organizations
To access all the capabilities of ArcGIS Online, your organization can purchase a subscription. With a subscription to ArcGIS Online, organizations can manage all of their geographic content in a secure, cloud-based Esri environment. Members of the organization can use maps to explore data, create and share maps and apps, and publish their data as hosted web layers. Administrators customize the website, invite and add members to the organization, and manage resources.
Learn more about ArcGIS for organizations at ArcGIS Online.
Portal items, users, and groups
ArcGIS Online is an information portal and is represented in the API by the
AGSPortal
class. This class is loadable.
When you sign in to ArcGIS Online with an organization account, you see a specialized view that your organization administrator has configured for you, giving you access to maps, content, and other services specific to your organization. You can also access all your own content and services.
Portal items
ArcGIS Online and ArcGIS Enterprise can store many types of content. See Supported items in the ArcGIS documentation for an exhaustive list. Perhaps the type most commonly used by apps is a web map—a JSON description of the data in a map, along with other display and behavioral properties. Other types include tile packages, feature collections, and services. Some types, such as globe documents and map templates, may only be relevant to desktop systems. Although you can access these types of data using ArcGIS Runtime API, you may not be able to use them on a mobile device.
The
AGSPortalItem
class represents an individual item stored on a portal. Each item includes the following:
- Metadata, such as a unique identifier (ID), title, summary, description, thumbnail image, and tags
- Binary or text-based data—for example, the JSON that defines a web map or a feature collection, or a URL pointing to a map service, or the binary data of a tile package or shapefile
The item ID is the most important metadata for a portal item. You can use the ID to access an item in the portal and work with it in your app (such as loading a web map from a portal item, for example).
Portal users
A registered user of a portal is represented in the API by the
AGSPortalUser
class. When you have signed in to a portal, you can get authentication information relating to the authenticated user from the portal class.
A user must authenticate to access secured resources. The authentication method you decide to implement might vary based upon the resources required by your application. Esri's preferred authentication methods are:
-
User authentication (OAuth 2.0): This method obtains user credentials and authentication and responds with an OAuth 2.0
access_
to the client app. The app uses thistoken token
in all subsequent requests to the platform. This is the recommended method, and is most commonly used with ArcGIS Online and ArcGIS Enterprise. -
API key: This unique identifier is used to authenticate a user, developer, or calling program to an API, although most typically used to authenticate a project rather than a human user.
For an overview of the ways to access secure services, see ArcGIS Security and Authentication.
Portal groups
Groups are a way to collaborate with other users who share a common interest. A user can create groups, join groups, and share items with those groups. Groups have titles, descriptions, thumbnails, and unique IDs to help users identify them. The sharing model within ArcGIS Online is based on groups. Groups are represented in the API by the
AGSPortalGroup
class.
Connect to public content
To connect to ArcGIS Online and access public content anonymously, you can begin by creating an
AGSPortal
instance (without authenticating with the portal).
self.portal = AGSPortal(url: URL(string: "https://www.arcgis.com")!, loginRequired: false)
self.portal.load() {[weak self] (error) in
if let error = error {
print(error)
}
// check the portal item loaded and print the modified date
if self?.portal.loadStatus == AGSLoadStatus.loaded {
if let portalName = self?.portal.portalInfo?.portalName {
print(portalName)
}
}
}
From here, you can access public content; for example, you can
- Display a web map.
- Access the data stored in a portal item.
- Search for content such as web maps, map services, map features, groups, and users.
Some organizations share content publicly and allow anonymous access to that content; connect to publicly accessible content from such organizations by specifying the organization URL.
For example:
self.portal = AGSPortal(url: URL(string: "https://anorganization.maps.arcgis.com")!, loginRequired: false)
Connect to secured content
Apps that target organization users who have an ArcGIS account should add a
AGSCredential
to the
AGSAuthenticationManager
to authenticate the user when creating the portal object.
The portal object will have access to all the secure content for which the user has access rights and can be used to find out more information about the user, such as the user's full name (instead of the account user name). Additionally, information about the organization such as the name, banner image, description, and so on, can be found as shown above. Apps often make use of this information when a user connects to a specific portal, to show the user organization branding and context.
Typically, the portal object with the authenticated user is cached and used throughout the app session, to provide the app with a view of a portal that is centered around a single user. When the app is restarted, the credential must be reinstated, or the user must repeat the authentication process.
self.portal = AGSPortal(url: URL(string: "https://www.arcgis.com")!, loginRequired: false)
self.portal.credential = AGSCredential(user: "theUser", password: "thePassword")
self.portal.load() {[weak self] (error) in
if let error = error {
print(error)
return
}
// check the portal item loaded and print the modified date
if self?.portal.loadStatus == AGSLoadStatus.loaded {
let fullName = self?.portal.user?.fullName
print(fullName!)
}
}
ArcGIS Enterprise
ArcGIS Enterprise provides you with the same core capabilities as ArcGIS Online, but it can be installed and hosted on your own premises, behind your firewall, for controlled distribution of content.
Learn more about ArcGIS Enterprise.
Connecting to an instance of ArcGIS Enterprise portal is done in a very similar way to connecting to ArcGIS Online and is represented in the API by the same class,
AGSPortal
. Use the URL to the Enterprise portal website, along with an appropriate credential valid on that portal, or no credential if accessing public content anonymously.
self.portal = AGSPortal(url: URL(string: "https://geoportal.mycompany.com")!, loginRequired: false)
self.portal.credential = AGSCredential(user: "username", password: "password")
Samples
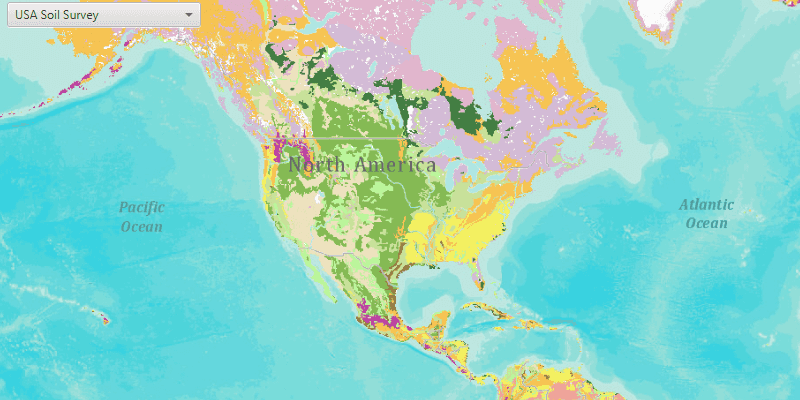
Open map (URL)
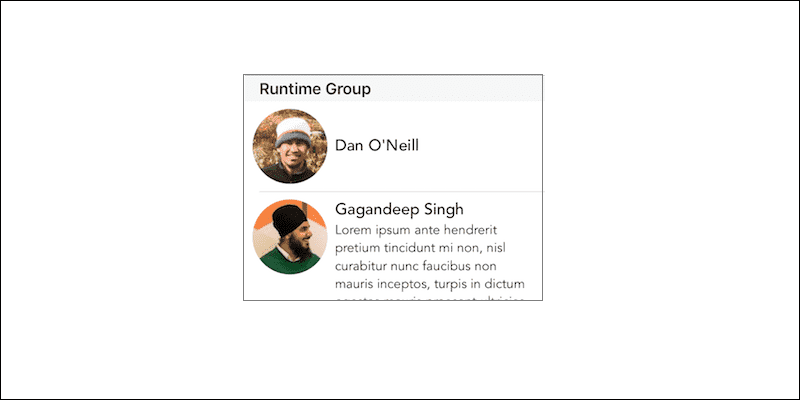
List portal group users
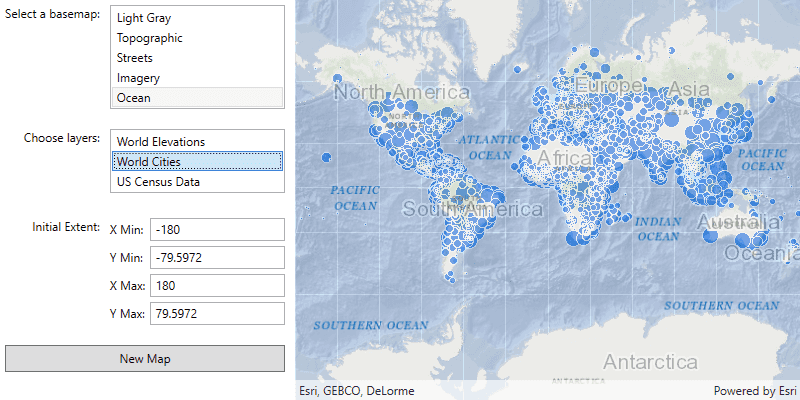
Create and save a map
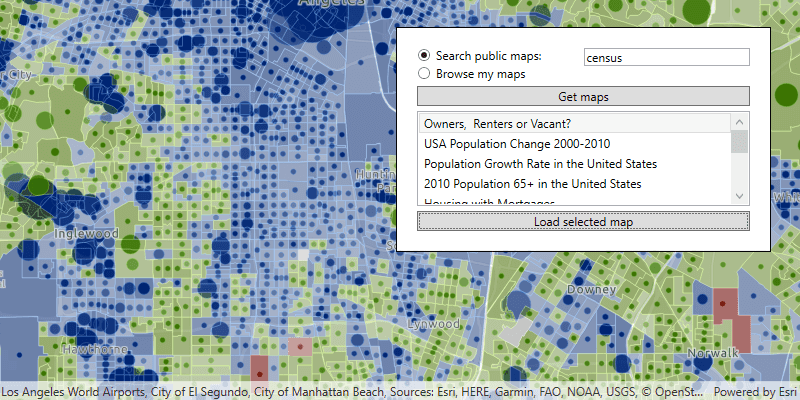
Search for web map
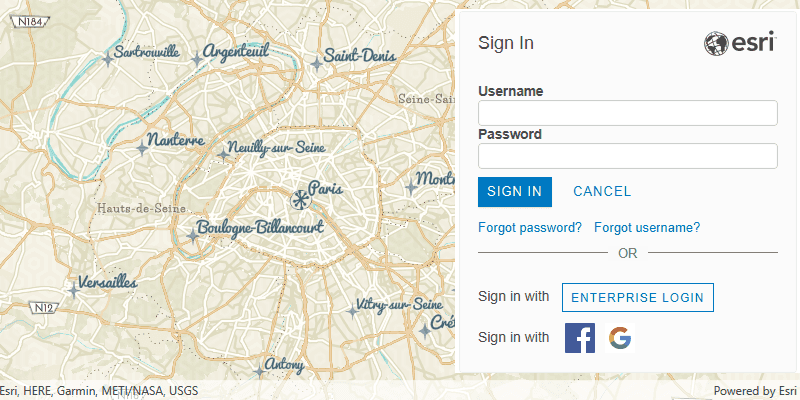
Authenticate with OAuth
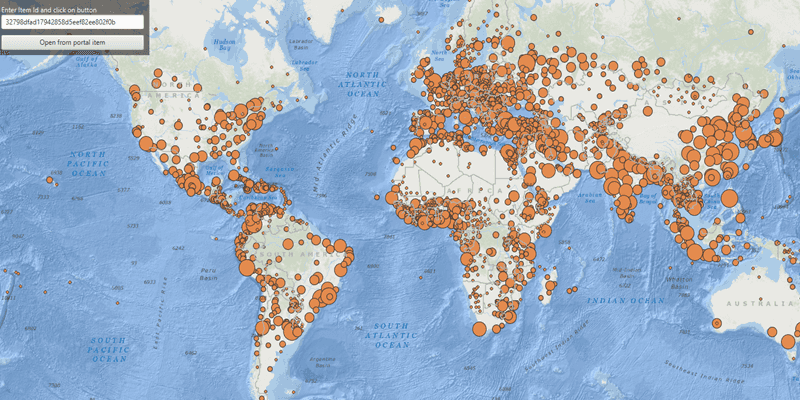
Feature collection layer (portal item)
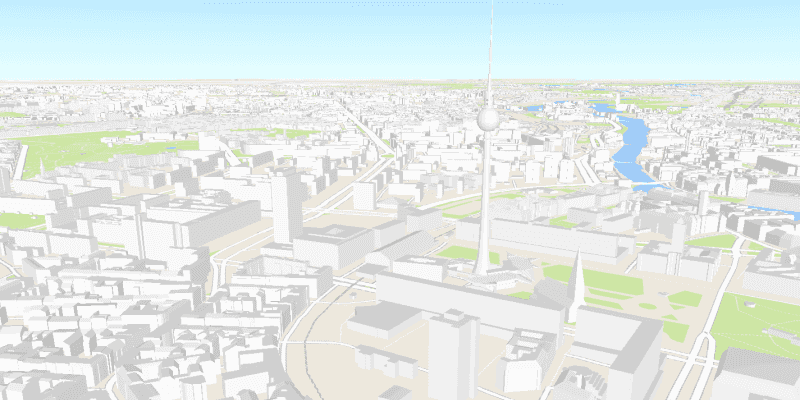
Open a scene (portal item)
Services
Feature service
Add, update, delete, and query feature data.
Vector tile service
Store and access vector tile data.
Image tile service
Store and access image tile data.
Tools
ArcGIS Online
Cloud-based GIS with tools to manage, analyze, and share hosted data.
Developer dashboard
Manage API keys, service usage, and data for your applications.
Map Viewer
Create, explore, and share web maps for 2D applications.
Scene Viewer
Create, explore, and share web scenes for 3D applications.