Learn how to perform and display a viewshed analysis in a 3D scene.
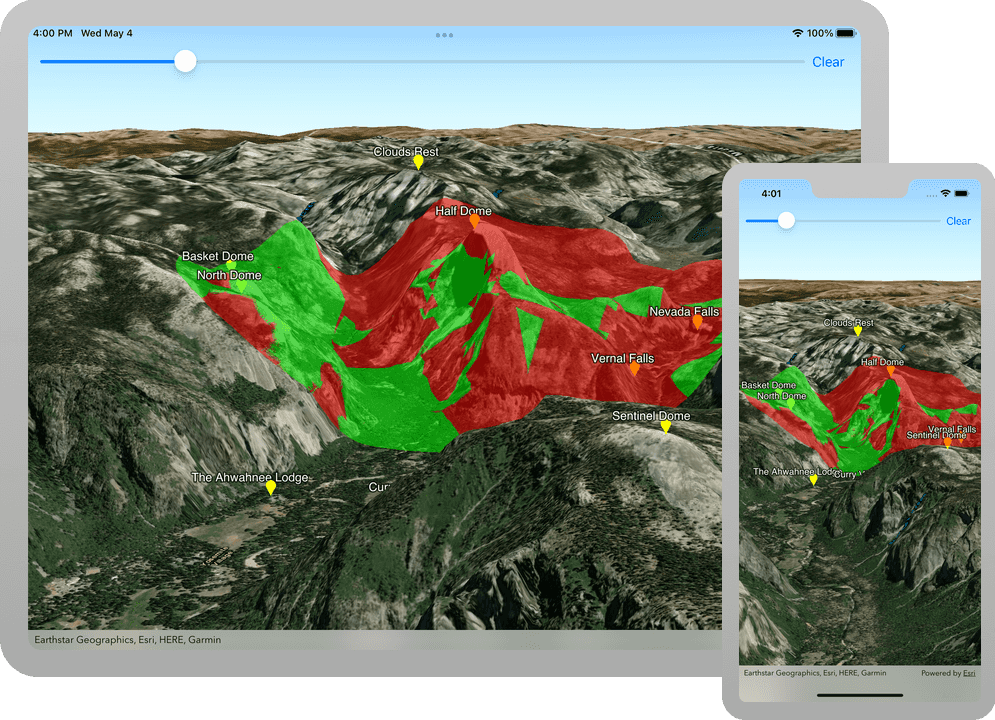
Viewshed analysis determines the visibility of terrain, buildings, and other 3D objects from an observer's location within a scene (using a specified field of view). The result indicates which areas are visible and which are obstructed when viewed from the observer's perspective.
In this tutorial, you will perform and display a viewshed analysis in a web scene. Your viewshed analysis will show visibility (visible or obstructed) and can be used to determine which hotspots in the Yosemite Valley are visible from a specified observer's perspective.
Prerequisites
The following are required for this tutorial:
- An ArcGIS account to access your API keys. If you don't have an account, sign up for free.
- Your system meets the system requirements.
Steps
Open the Xcode project
-
To start this tutorial, first complete the Display a web scene tutorial or download and unzip the solution.
-
Open the
.xcodeproj
file in Xcode. -
If you downloaded the solution project, set your API key.
An API Key enables access to services, web maps, and web scenes hosted in ArcGIS Online.
-
Go to your developer dashboard to get your API key. For these tutorials, use your default API key. It is scoped to include all of the services demonstrated in the tutorials.
- In Xcode, in the Project Navigator, click AppDelegate.swift.
- In the editor, set the
APIKey
property on theAGSArcGISRuntime
with your API key.Environment
AppDelegate.swiftUse dark colors for code blocks func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { // Note: it is not best practice to store API keys in source code. // The API key is referenced here for the convenience of this tutorial. AGSArcGISRuntimeEnvironment.apiKey = "YOUR_API_KEY" return true }
-
Get the web scene item ID
You can use ArcGIS tools to create and view web scenes. Use the Scene Viewer to identify the web scene item ID. This item ID will be used later in the tutorial.
- Go to the Yosemite Valley Hotspots web scene in the Scene Viewer in ArcGIS Online. This web scene displays terrain and hotspots in the Yosemite Valley.
- Make a note of the item ID at the end of the browser's URL. The item ID should be 7558ee942b2547019f66885c44d4f0b1
Update the scene
-
In Xcode, in the Project Navigator, click ViewController.swift.
-
In the editor, modify the
setup
method to create anScene() AGSScene
for the web scene. To do this, create a portal item providing the web scene's item ID and anAGSPortal
referencing ArcGIS Online.ViewController.swiftUse dark colors for code blocks Change line private func setupScene() { let scene: AGSScene = { let portal = AGSPortal.arcGISOnline(withLoginRequired: false) let item = AGSPortalItem(portal: portal, itemID: "7558ee942b2547019f66885c44d4f0b1") return AGSScene(item: item) }() sceneView.scene = scene }
Add a UI to control the viewshed analysis
To control the viewshed analysis, some UI elements are required.
-
Create a private
UISlider
namedslider
. The slider changes the viewshed's maximum distance by expanding or contracting the size of the observer's field of view. Themaximum
andValue minimum
properties define the range of values the user can select to calculate the viewshed. You set an action on the slider using a selector for a method that will be added in a later step.Value ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. private lazy var slider: UISlider = { let slider = UISlider() slider.maximumValue = 12_000 slider.minimumValue = 1_000 slider.value = 3_000 slider.addTarget(self, action: #selector(sliderValueDidChange), for: .allTouchEvents) return slider }()
-
Define a private method named
setup
and assign theUI() slider
as well as a"Clear"
button to the navigation bar. The clear button removes the viewshed from the sceneYou set an action on the clear button using a selector for a method that will be added in a later step.ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. private lazy var slider: UISlider = { let slider = UISlider() slider.maximumValue = 12_000 slider.minimumValue = 1_000 slider.value = 3_000 slider.addTarget(self, action: #selector(sliderValueDidChange), for: .allTouchEvents) return slider }() private func setupUI() { navigationItem.titleView = slider navigationItem.rightBarButtonItem = UIBarButtonItem( title: "Clear", style: .plain, target: self, action: #selector(clearScene) ) }
-
Define a method named
slider
and assign it theValue D i d Change(_ : ) @objc
keyword. This method updates the viewshed'smax
to a value specified by the slider. TheDistance @objc
method keyword exposes the method to Objective-C, a necessary step for using theUISlider
API.ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. private func setupUI() { navigationItem.titleView = slider navigationItem.rightBarButtonItem = UIBarButtonItem( title: "Clear", style: .plain, target: self, action: #selector(clearScene) ) } @objc func sliderValueDidChange(_ sender: UISlider) { viewshed.maxDistance = Double(sender.value) }
-
Define a method named
clear
and assign it theScene(_ : ) @objc
keyword. This method hides the viewshed from the scene view. The@objc
method keyword exposes the method to Objective-C, a necessary step for using theUIBar
API.Button Item ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. @objc func sliderValueDidChange(_ sender: UISlider) { viewshed.maxDistance = Double(sender.value) } @objc func clearScene(_ sender: UIBarButtonItem) { clearViewshed() }
-
In
view
, call the methodD i d Load() setup
.UI() ViewController.swiftUse dark colors for code blocks Add line. override func viewDidLoad() { super.viewDidLoad() setupScene() setupUI() }
-
In Xcode, in the Project Navigator, click Main.storyboard.
-
In the editor, select
View
. In the menu bar, click Editor > Embed In > Navigation Controller.Controller Embedding
View
within a Navigation Controller places a navigation bar at the top ofController View
. The navigation bar contains the UI elements.Controller -
To verify that your UI is set up properly, press <Command+R> to run the app. You should see a slider and a "Clear" button contained by the navigation bar and the app should load the Yosemite Valley Hotspots web scene.
Create a viewshed analysis
Visual analyses are used to help you make sense of complex 3D data contained by a scene. Use a AGSLocation
to perform and display a viewshed analysis using a 3D point to define the observer's location.
-
In Xcode, in the Project Navigator, click ViewController.swift.
-
In the editor, create a private lazy
AGSLocation
namedViewshed viewshed
. Upon launch, the viewshed should not be visible.ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. private lazy var viewshed: AGSLocationViewshed = { let viewshed = AGSLocationViewshed( location: AGSPointMake3D(0, 0, 0, 0, .wgs84()), heading: 0, pitch: 90, horizontalAngle: 360, verticalAngle: 180, minDistance: 10, maxDistance: Double(slider.value) ) viewshed.isVisible = false return viewshed }()
-
Define a private method named
setup
to add the viewshed to the scene.Analysis Overlay() The viewshed analysis is added to a scene view using an analysis overlay. An analysis overlay is a container for analyses. It can be used to display visual analyses in a scene view. You can add more than one analysis overlay and they are displayed on top of all other layers.
ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. private lazy var viewshed: AGSLocationViewshed = { let viewshed = AGSLocationViewshed( location: AGSPointMake3D(0, 0, 0, 0, .wgs84()), heading: 0, pitch: 90, horizontalAngle: 360, verticalAngle: 180, minDistance: 10, maxDistance: Double(slider.value) ) viewshed.isVisible = false return viewshed }() private func setupAnalysisOverlay() { let analysisOverlay = AGSAnalysisOverlay() sceneView.analysisOverlays.add(analysisOverlay) analysisOverlay.analyses.add(viewshed) }
-
Define a private method named
set
that receives a point as a parameter. This method is used to set the location of the viewshed and make it visible, if it is not visible already.Viewshed Location(point: ) ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. private func setupAnalysisOverlay() { let analysisOverlay = AGSAnalysisOverlay() sceneView.analysisOverlays.add(analysisOverlay) analysisOverlay.analyses.add(viewshed) } private func setViewshedLocation(point: AGSPoint) { viewshed.location = point if !viewshed.isVisible { viewshed.isVisible = true } }
-
Define a private method named
clear
. This method is used to hide the viewshed.Viewshed() ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. private func setViewshedLocation(point: AGSPoint) { viewshed.location = point if !viewshed.isVisible { viewshed.isVisible = true } } private func clearViewshed() { viewshed.isVisible = false }
-
In
view
, callD i d Load() setup
.Analysis Overlay() ViewController.swiftUse dark colors for code blocks Add line. override func viewDidLoad() { super.viewDidLoad() setupScene() setupUI() setupAnalysisOverlay() }
Display the viewshed analysis with touch events
Touch events determine where to place the observer for the viewshed analysis. A user will long-press and drag to reveal and move the observer's location.
-
Extend
View
to conform to theController AGSGeo
protocol and include the four long-press geoview touch delegate methods.View Touch Delegate ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. extension ViewController: AGSGeoViewTouchDelegate { func geoView(_ geoView: AGSGeoView, didLongPressAtScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { } func geoView(_ geoView: AGSGeoView, didMoveLongPressToScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { } func geoView(_ geoView: AGSGeoView, didEndLongPressAtScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { } func geoViewDidCancelLongPress(_ geoView: AGSGeoView) { } }
-
When the user begins, moves, and ends a long-press touch event, call
set
. Using all three touch delegate methods together allows the user to reveal the observer location with a long-press touch event and move the observer by dragging their finger around the screen.Viewshed Location(point: ) ViewController.swiftUse dark colors for code blocks Add line. Add line. Add line. extension ViewController: AGSGeoViewTouchDelegate { func geoView(_ geoView: AGSGeoView, didLongPressAtScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { setViewshedLocation(point: mapPoint) } func geoView(_ geoView: AGSGeoView, didMoveLongPressToScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { setViewshedLocation(point: mapPoint) } func geoView(_ geoView: AGSGeoView, didEndLongPressAtScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { setViewshedLocation(point: mapPoint) } func geoViewDidCancelLongPress(_ geoView: AGSGeoView) { } }
-
When the user cancels the long-press touch event, call
clear
.Viewshed() This may happen, for instance, when you have the magnifier visible and attempt to take a screenshot using the home/lock button combination.
ViewController.swiftUse dark colors for code blocks Add line. extension ViewController: AGSGeoViewTouchDelegate { func geoView(_ geoView: AGSGeoView, didLongPressAtScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { setViewshedLocation(point: mapPoint) } func geoView(_ geoView: AGSGeoView, didMoveLongPressToScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { setViewshedLocation(point: mapPoint) } func geoView(_ geoView: AGSGeoView, didEndLongPressAtScreenPoint screenPoint: CGPoint, mapPoint: AGSPoint) { setViewshedLocation(point: mapPoint) } func geoViewDidCancelLongPress(_ geoView: AGSGeoView) { clearViewshed() } }
-
In the
setup
method, assignScene() View
toController scene
.View.touch Delegate
This step bridges scene
user touch interactions with View
via the AGSGeo
protocol.
private func setupScene() {
let scene: AGSScene = {
let portal = AGSPortal.arcGISOnline(withLoginRequired: false)
let item = AGSPortalItem(portal: portal, itemID: "7558ee942b2547019f66885c44d4f0b1")
return AGSScene(item: item)
}()
sceneView.scene = scene
sceneView.touchDelegate = self
}
Run the app
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Big Sur 11.3, Xcode 13, iOS 13. If you are using a physical device, then refer to the system requirements.
You should see a scene of hotspots in the Yosemite Valley. Long-press and drag to display and move a viewshed analysis to explore the visibility of terrain from various locations.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: