Maps and scenes provide interactive displays of geographic data that enable you to visualize and explore patterns, answer questions, and share insight. They can be opened, edited, and shared across the ArcGIS system and beyond. Maps are designed for interaction in two dimensions (2D) and scenes for interaction in three dimensions (3D). See Scenes (3D) in this guide for more information about working with scenes.
You can use a map to:
- Display a basemap layer such as streets or satellite imagery.
- Access and display data layers based on files or services, including data you have authored.
- Provide context for temporary points, lines, polygons, or text displayed as graphics.
- Inspect data layers and display information from attributes.
- Measure distance and explore spatial relationships between geometries.
- Save a collection of layers as a web map to be shared across ArcGIS.
How a map works
A map works together with a map view to display geographic content in two dimensions. A map contains a collection of layers including multiple data layers from online or local sources, and a basemap layer that gives geographic context. A map can also contain datasets that enable searches for addresses or place names, networks for solving routes, utility networks for tracing the flow of services such as water and electricity, and non-spatial tables. Maps can be created, displayed, edited, and saved using ArcGIS Runtime. Because the format is based on the ArcGIS web map standard, these maps can be shared uniformly across the ArcGIS system.
For offline workflows (when you don't have network connectivity), you can open a map stored in a mobile map package. Mobile map packages can be created using ArcGIS Pro, ArcGIS Enterprise, or ArcGIS Online. See Offline maps, scenes, and data for more information about implementing offline workflows with ArcGIS Runtime.
Map
A map contains a collection of layers displayed in the order in which they were added, with the most recently added layer being displayed above existing layers. You can use the map to change the display order of layers as well as to control which layers are visible, and expose this functionality with user interface controls such as lists, check boxes, or switches. A map also contains a collection of bookmarks, which, similar to a web browser bookmark, allow you to quickly navigate to a predefined area of the map.
You can open an existing map or create one entirely with code. To create a map, you typically first add a basemap layer and then one or more data layers.
AGSArcGISRuntimeEnvironment.apiKey = "YOUR_API_KEY"
let map = AGSMap(
basemapStyle: .arcGISNavigation
)
You can also open a map stored in a portal (such as ArcGIS Online) using its URL.
let map = AGSMap(url: URL(string:"https://www.arcgis.com/home/item.html?id=acc027394bc84c2fb04d1ed317aac674")!)
You can define the initial extent at which the map will display by setting the
AGSMap.initialViewpoint
property.
Layer
Each layer in a map references geographic data, either from an online service or from a local dataset. There are different types of of layers that can be added to a map, each designed to display a particular type of data. Some layers display images, such as satellite imagery or aerial photography, while others are composed of a collection of features to represent real-world entities using point, line, or polygon geometries. In addition to geometry, features have attributes that provide details about the entity it represents.
The
AGSLayer
class is the base class for all types of layers used in ArcGIS Runtime. The type of layer you create depends on the type of data you want to display. For example, to display feature data you can create an
AGSFeatureLayer
that references an online service (such as a feature service) or a supported local dataset.
AGSArcGISRuntimeEnvironment.apiKey = "YOUR-API-KEY"
let map = AGSMap(
basemapStyle: .arcGISTopographic
)
let trailheadsLayer = AGSFeatureLayer(featureTable:
AGSServiceFeatureTable(url: URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0")!)
)
let trailsLayer = AGSFeatureLayer(featureTable:
AGSServiceFeatureTable(url: URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0")!)
)
let openSpacesLayer = AGSFeatureLayer(featureTable:
AGSServiceFeatureTable(url: URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0")!)
)
map.operationalLayers.addObjects(from: [openSpacesLayer, trailsLayer, trailheadsLayer])
MapView
A map view is a user-interface control that displays a single map in your app. It contains built-in functionality that allows the user to explore the map by zooming in and out, recentering the map display, or getting additional information about elements in the map. It also manages graphics in one or more collections of graphics overlays. The graphics managed by the map view always display above all layers displayed in the map.
A map view control also allows you to:
- Access data for data layers and graphics.
- Display the current location as a point on the map.
- Identify and select features at a specified location.
- Export an image of the current display.
- Rotate the map display.
- Apply a time extent to filter the display of features.
- Filter layer data using attribute and spatial criteria.
Display a map by adding it to a
AGSMapView
control. Changes you make to the map, such as adding, removing, or reordering layers, will immediately be reflected in the map view display. The
AGSMap.initialViewpoint
property will determine the area of the map shown when the map loads. You can also use
AGSMapView.setViewpoint()
and
AGSMapView.setViewpointRotation()
to programmatically change the map area or orientation shown in the display.
@IBOutlet weak var mapView: AGSMapView!
...
mapView.map = map
mapView.setViewpoint(
AGSViewpoint(
latitude: 34.027,
longitude: -118.805,
scale: 72223.819286
)
)
Examples
Create and display a map
You can display a basic map by creating one with a basemap layer, setting its initial extent, and adding it to a map view. To learn how to add additional data, see the Layers topic.
Steps
- Create a new
AGSMap
, passing aAGSBasemapStyle
into the constructor. - Optionally, add one or more data layers to the map.
- Assign the map to an
AGSMapView
control in your app. - Set the map view
AGSViewpoint
to focus on a specified area of the map.
class ViewController: UIViewController {
@IBOutlet weak var mapView: AGSMapView!
private func setupMap() {
AGSArcGISRuntimeEnvironment.apiKey = "YOUR_API_KEY"
let map = AGSMap(
basemapStyle: .arcGISNavigation
)
mapView.map = map
mapView.setViewpoint(
AGSViewpoint(
latitude: 34.027,
longitude: -118.805,
scale: 72223.819286
)
)
}
override func viewDidLoad() {
super.viewDidLoad()
setupMap()
}
}
You can also create and save a web map using ArcGIS Pro or the ArcGIS Online map viewer and open it in your ArcGIS Runtime app.
Display a web map
You can open a web map saved as an item in a portal, such as ArcGIS Online, using its item ID or URL. A portal item can be secured to only allow access for authorized users, in which case you must provide credentials for access. To learn how to access secured content, see Security and authentication.
Steps
- Create an
AGSPortal
object to connect to the portal that hosts a web map you want to load. If you don't provide a portal URL, the connection defaults to ArcGIS Online. - Create an
AGSPortalItem
object using the item ID for the item that stores the web map. - Create a new map, passing the portal item into the constructor.
- Assign the map to an
AGSMapView
control in your app.
AGSArcGISRuntimeEnvironment.apiKey = "YOUR_API_KEY"
let portal = AGSPortal.arcGISOnline(withLoginRequired: false)
let itemID = "41281c51f9de45edaf1c8ed44bb10e30"
let portalItem = AGSPortalItem(portal: portal, itemID: itemID)
let map = AGSMap(item: portalItem)
mapView.map = map
Display a mobile map
This example displays a map from a mobile map package. You can create your own mobile map (or scene) packages using ArcGIS Pro or download existing ones from ArcGIS Online. The Yellowstone mobile map package, for example, shows points of interest in Yellowstone National Park.
Steps
- Create an
AGSMobileMapPackage
using the path to a local .mmpk file. - Call
AGSMobileMapPackage.loadWithCompletion:()
to load the package. - When the package loads, get the first map from the package using the
AGSMobileMapPackage.maps
property. - Display the map in an
AGSMapView
.
AGSArcGISRuntimeEnvironment.apiKey = "YOUR_API_KEY"
//initialize map package
self.mapPackage = AGSMobileMapPackage(name: "Yellowstone")
//load map package
self.mapPackage.load
let map = self.mapPackage.maps.first
//assign the first map from the map package to the map view
self.mapView.map = map
Use API keys
An API key can be used to authorize access to ArcGIS Online services and resources from your app, as well as to monitor access to those services. You can use a single API key for all requests made by your app, or assign individual keys for classes that expose an API key property. Refer to API keys in the security and authentication topic for details.
Tutorials
Samples
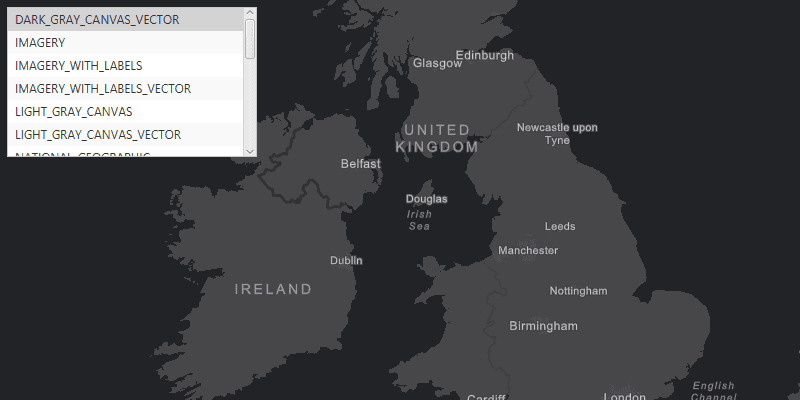
Change basemap
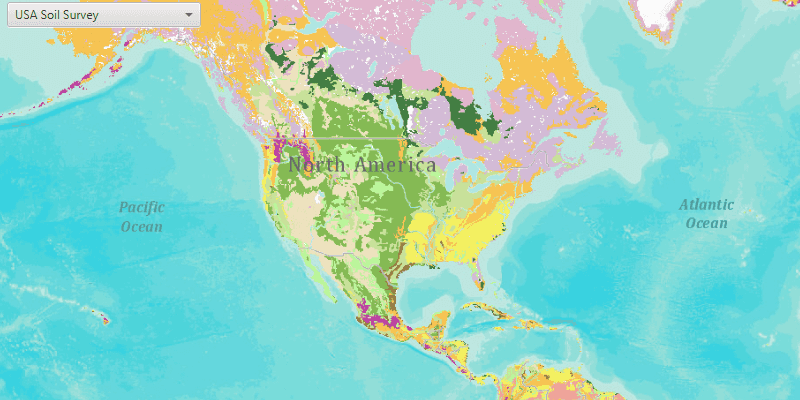
Open map (URL)
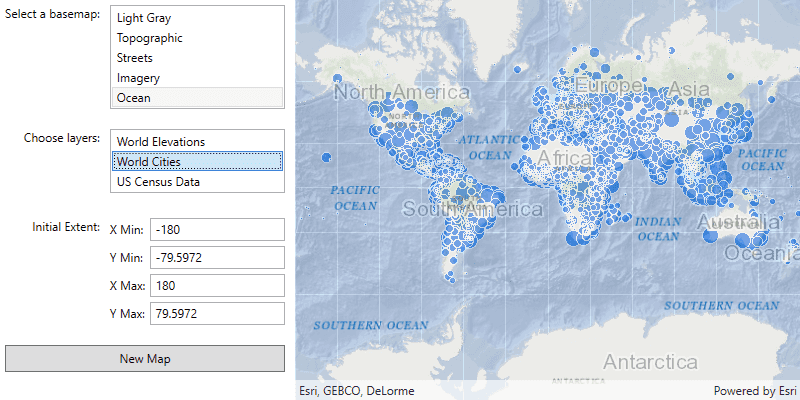
Create and save a map
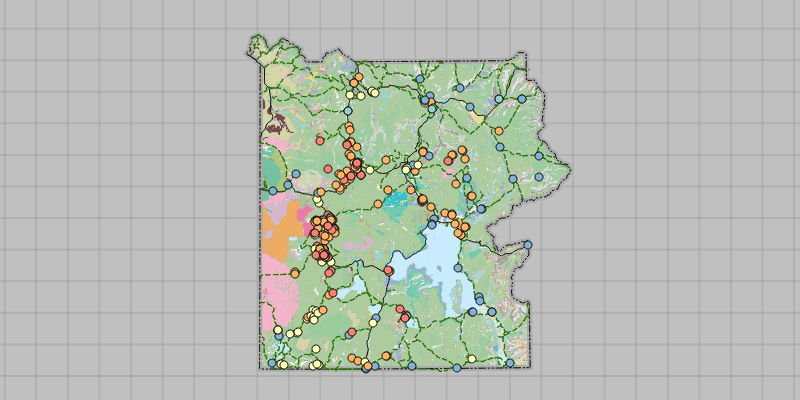
Open mobile map package
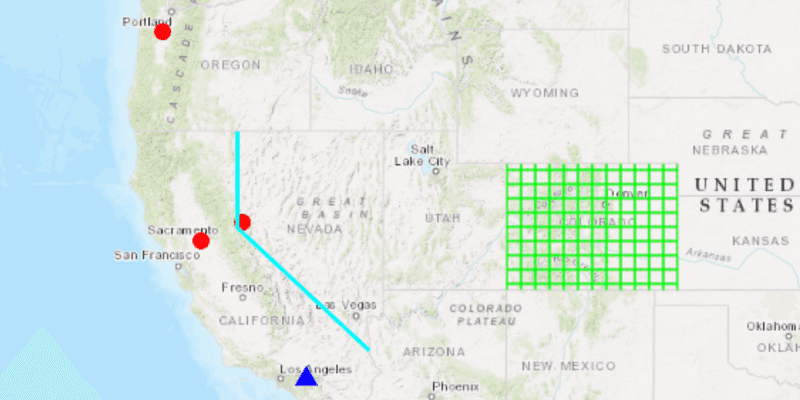
Create geometries
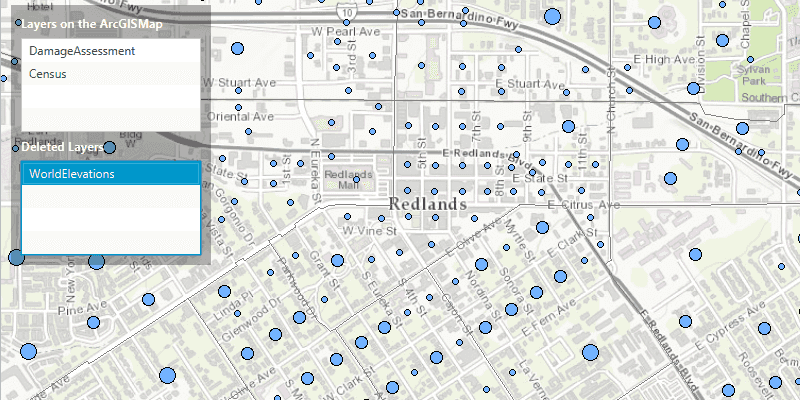