Learn how to apply renderers and label definitions to a feature layer based on attribute values.
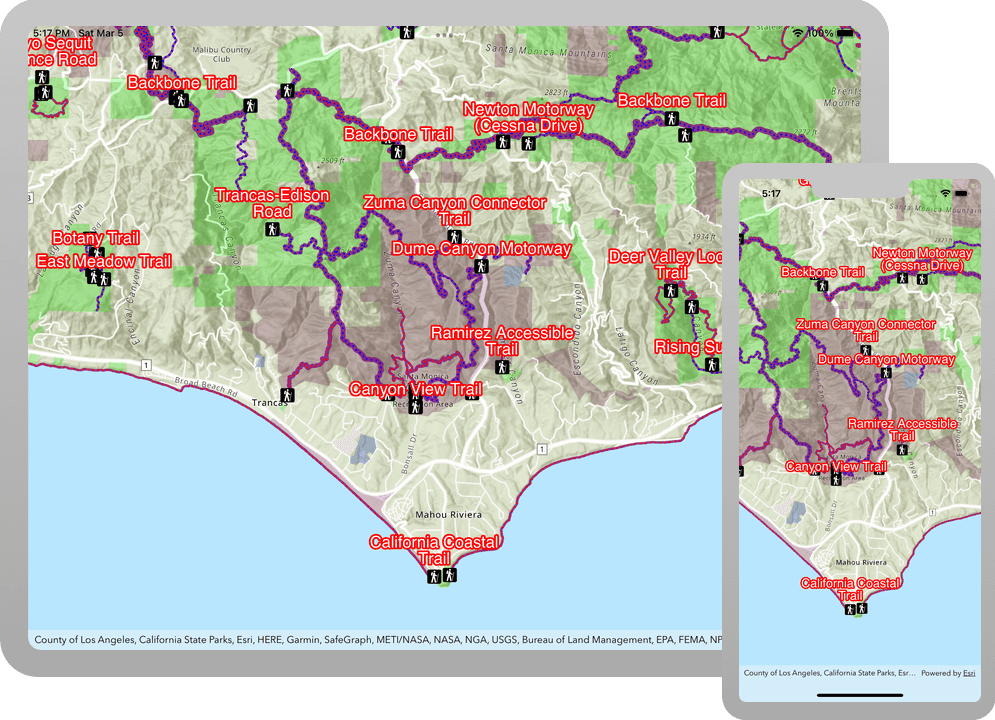
Applications can display feature layer data with different styles to enhance the visualization. The type of
AGSRenderer
you choose depends on your application. An
AGSSimpleRenderer
applies the same symbol to all features, an
AGSUniqueValueRenderer
applies a different symbol to each unique attribute value, and an
AGSClassBreaksRenderer
applies a symbol to a range of numeric values. Renderers are responsible for accessing the data and applying the appropriate symbol to each feature when the layer draws. You can also use a
AGSLabelDefinition
to show attribute information for features. Visit the Styles and data visualization documentation to learn more about styling layers.
You can also author, style and save web maps, web scenes, and layers as portal items and then add them to the map in your application. Visit the following tutorials to learn more about adding portal items.
In this tutorial, you will apply different renderers to enhance the visualization of three feature layers with data for the Santa Monica Mountains: Trailheads with a single symbol, Trails based on elevation change and bike use, and Parks and Open Spaces based on the type of park.
Prerequisites
The following are required for this tutorial:
- An ArcGIS account to access your API keys. If you don't have an account, sign up for free.
- Your system meets the system requirements.
Steps
Open the Xcode project
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.xcodeproj
file in Xcode. -
If you downloaded the solution project, set your API key.
An API Key enables access to services, web maps, and web scenes hosted in ArcGIS Online.
-
Go to your developer dashboard to get your API key. For these tutorials, use your default API key. It is scoped to include all of the services demonstrated in the tutorials.
- In Xcode, in the Project Navigator, click AppDelegate.swift.
- In the editor, set the
APIKey
property on theAGSArcGISRuntime
with your API key.Environment
AppDelegate.swiftUse dark colors for code blocks func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { // Note: it is not best practice to store API keys in source code. // The API key is referenced here for the convenience of this tutorial. AGSArcGISRuntimeEnvironment.apiKey = "YOUR_API_KEY" return true }
-
Add app configuration constants
Create a configuration file to specify constants that can be used by the app to connect to data and resources.
-
Add a new swift file named AppConfiguration.swift.
- In Xcode's app menu, select File > New > File....
- Select Swift File from the iOS tab's Source sub-menu.
- Name the file AppConfiguration and ensure your app's target is checked.
- Click Create.
-
Create four static
URL
s: three for accessing feature layers, and a fourth for accessing a static image for use in a picture marker symbol. You will use these resources in future steps.AppConfiguration.swiftUse dark colors for code blocks extension URL { static let parksAndOpenSpaces = URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space/FeatureServer/0")! static let trails = URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails/FeatureServer/0")! static let trailheads = URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads/FeatureServer/0")! static let trailheadImage = URL(string: "https://static.arcgis.com/images/Symbols/NPS/npsPictograph_0231b.png")! }
Create helper method to add feature layer to map
Add a helper method that creates a feature layer from a given URL
and adds it to the list of map's operational layers.
-
Open
View
in the Project Navigator. Add a new method namedController.swift add
.Feature Layer(url: URL) ViewController.swiftUse dark colors for code blocks private func addFeatureLayer(url: URL) -> AGSFeatureLayer { let serviceFeatureTable = AGSServiceFeatureTable(url: url) let featureLayer = AGSFeatureLayer(featureTable: serviceFeatureTable) mapView.map?.operationalLayers.add(featureLayer) return featureLayer }
Add a layer with a unique value renderer
Create a method to apply a different symbol for each type of park area to the Parks and Open Spaces feature layer.
-
Add a new method named
add
inOpen Space Layer() View
.Controller.swift AGSUniqueValue
assigns a symbol to a value or values. A unique value renderer uses a collection of unique values to assign the appropriate symbol for each feature it renderers.For this example, the renderer uses a feature's
TYPE
attribute value to apply the correct symbol.ViewController.swiftUse dark colors for code blocks private func addOpenSpaceLayer() { // Create a parks and open spaces feature layer, and add it to mapview. let featureLayer = addFeatureLayer(url: .parksAndOpenSpaces) // Create fill symbols. let purpleFillSymbol = AGSSimpleFillSymbol(style: .solid, color: .purple, outline: .none) let greenFillSymbol = AGSSimpleFillSymbol(style: .solid, color: .green, outline: .none) let blueFillSymbol = AGSSimpleFillSymbol(style: .solid, color: .blue, outline: .none) let redFillSymbol = AGSSimpleFillSymbol(style: .solid, color: .red, outline: .none) // Create a unique value for natural areas, regional open spaces, local parks & regional recreation parks. let naturalAreas = AGSUniqueValue(description: "Natural Areas", label: "Natural Areas", symbol: purpleFillSymbol, values: ["Natural Areas"]) let regionalOpenSpace = AGSUniqueValue(description: "Regional Open Space", label: "Regional Open Space", symbol: greenFillSymbol, values: ["Regional Open Space"]) let localPark = AGSUniqueValue(description: "Local Park", label: "Local Park", symbol: blueFillSymbol, values: ["Local Park"]) let regionalRecreationPark = AGSUniqueValue(description: "Regional Recreation Park", label: "Regional Recreation Park", symbol: redFillSymbol, values: ["Regional Recreation Park"]) // Create and assign a unique value renderer to the feature layer. let openSpacesUniqueValueRenderer = AGSUniqueValueRenderer(fieldNames: ["TYPE"], uniqueValues: [naturalAreas, regionalOpenSpace, localPark, regionalRecreationPark], defaultLabel: "Open Spaces", defaultSymbol: .none) featureLayer.renderer = openSpacesUniqueValueRenderer // Set the layer opacity to semi-transparent. featureLayer.opacity = 0.2 }
-
Update
view
to call the newD i d Load() add
method.Open Space Layer() ViewController.swiftUse dark colors for code blocks override func viewDidLoad() { super.viewDidLoad() setupMap() addOpenSpaceLayer() }
-
Press Command + R to run the app.
When the app opens, Parks and Open Spaces feature layer is added to the map. The map displays the different types of parks and open spaces with four unique symbols.
Add a layer with a class breaks renderer
Create a method to apply a different symbol for each of the five ranges of elevation gain to the Trails feature layer.
-
Add a new method named
add
.Trails Layer() An
AGSClassBreak
assigns a symbol to a range of values.For this example, the renderer uses each feature's
ELEV_
attribute value to classify it into a defined range (class break) and apply the corresponding symbol.GAIN ViewController.swiftUse dark colors for code blocks private func addTrailsLayer() { // Create a trails feature layer, and add it to mapview let featureLayer = addFeatureLayer(url: .trails) // Create simple line symbols. let firstClassSymbol = AGSSimpleLineSymbol(style: .solid, color: .purple, width: 3.0) let secondClassSymbol = AGSSimpleLineSymbol(style: .solid, color: .purple, width: 4.0) let thirdClassSymbol = AGSSimpleLineSymbol(style: .solid, color: .purple, width: 5.0) let fourthClassSymbol = AGSSimpleLineSymbol(style: .solid, color: .purple, width: 6.0) let fifthClassSymbol = AGSSimpleLineSymbol(style: .solid, color: .purple, width: 7.0) // Create 5 class breaks. let firstClassBreak = AGSClassBreak(description: "Under 500", label: "0 - 500", minValue: 0.0, maxValue: 500.0, symbol: firstClassSymbol) let secondClassBreak = AGSClassBreak(description: "501 to 1000", label: "501 - 1000", minValue: 501.0, maxValue: 1000.0, symbol: secondClassSymbol) let thirdClassBreak = AGSClassBreak(description: "1001 to 1500", label: "1001 - 1500", minValue: 1001.0, maxValue: 1500.0, symbol: thirdClassSymbol) let fourthClassBreak = AGSClassBreak(description: "1501 to 2000", label: "1501 - 2000", minValue: 1501.0, maxValue: 2000.0, symbol: fourthClassSymbol) let fifthClassBreak = AGSClassBreak(description: "2001 to 2300", label: "2001 - 2300", minValue: 2001.0, maxValue: 2300.0, symbol: fifthClassSymbol) let elevationBreaks = [firstClassBreak, secondClassBreak, thirdClassBreak, fourthClassBreak, fifthClassBreak] // Create and assign a class breaks renderer to the feature layer. let elevationClassBreaksRenderer = AGSClassBreaksRenderer(fieldName: "ELEV_GAIN", classBreaks: elevationBreaks) featureLayer.renderer = elevationClassBreaksRenderer // Set the layer opacity to semi-transparent. featureLayer.opacity = 0.75 }
-
Update
view
to call the newD i d Load() add
method.Trails Layer() ViewController.swiftUse dark colors for code blocks override func viewDidLoad() { super.viewDidLoad() setupMap() addOpenSpaceLayer() addTrailsLayer() }
-
Press Command + R to run the app.
When the app opens, the Trails feature layer is added to the map. The map displays trails with different symbols depending on trail elevation.
Add layers with definition expressions
You can use a definition expression to define a subset of features to display. Features that do not meet the expression criteria are not displayed by the layer. In the following steps, you will create two methods that use a definition expression to apply a symbol to a subset of features in the Trails feature layer.
AGSFeatureLayer.DefinitionExpression
uses a SQL expression to limit the features available for query and display. Your code will create two layers that each display a different subset of trails based on the value for the USE_
field. Trails that allow bikes will be symbolized with a blue symbol ("USE_
) and those that don't will be red ("USE_
). Another way to symbolize these features would be to create a
AGSUniqueValueRenderer
that applies a different symbol for these values.
-
Add a method with a definition expression to filter for trails that permit bikes.
ViewController.swiftUse dark colors for code blocks private func addBikeOnlyTrailsLayer() { // Create a trails feature layer, and add it to mapview. let featureLayer = addFeatureLayer(url: URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails/FeatureServer/0")!) // Write a definition expression to filter for trails that do permit the use of bikes. featureLayer.definitionExpression = "USE_BIKE = 'Yes'" // Create and assign a simple renderer to the feature layer. let bikeTrailSymbol = AGSSimpleLineSymbol(style: .dot, color: .blue, width: 2.0) let bikeTrailRenderer = AGSSimpleRenderer(symbol: bikeTrailSymbol) featureLayer.renderer = bikeTrailRenderer }
-
Add another method with a definition expression to filter for trails that don't allow bikes.
ViewController.swiftUse dark colors for code blocks private func addNoBikeTrailsLayer() { // Create a trails feature layer, and add it to mapview. let featureLayer = addFeatureLayer(url: URL(string: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails/FeatureServer/0")!) // Write a definition expression to filter for trails that don't permit the use of bikes. featureLayer.definitionExpression = "USE_BIKE = 'No'" // Create and assign a simple renderer to the feature layer. let noBikeTrailSymbol = AGSSimpleLineSymbol(style: .dot, color: .red, width: 2.0) let noBikeTrailRenderer = AGSSimpleRenderer(symbol: noBikeTrailSymbol) featureLayer.renderer = noBikeTrailRenderer }
-
Update
view
to call the newD i d Load() add
andBike Only Trails Layer() add
methods.No Bike Trails Layer() ViewController.swiftUse dark colors for code blocks override func viewDidLoad() { super.viewDidLoad() setupMap() addOpenSpaceLayer() addTrailsLayer() addNoBikeTrailsLayer() addBikeOnlyTrailsLayer() }
-
Press Command + R to run the app.
When the app opens, two Trails feature layers are added to the map. One shows where bikes are permitted and the other where they are prohibited.
Add a layer with a label definition
Create a method to style trailheads with hiker images and labels for the Trailheads feature layer.
-
Create a helper method named
make
to define a label defintion based on a specific attribute of the feature layerLabel Definition() TRL_
. Also define label placement and symbol.NAME ViewController.swiftUse dark colors for code blocks private func makeLabelDefinition() -> AGSLabelDefinition { let trailHeadsTextSymbol = AGSTextSymbol() trailHeadsTextSymbol.color = .white trailHeadsTextSymbol.size = 20.0 trailHeadsTextSymbol.haloColor = .red trailHeadsTextSymbol.haloWidth = 2.0 trailHeadsTextSymbol.fontFamily = "Noto Sans" trailHeadsTextSymbol.fontStyle = .italic trailHeadsTextSymbol.fontWeight = .normal // Make an arcade label expression. let expression = "$feature.TRL_NAME" let arcadeLabelExpression = AGSArcadeLabelExpression(arcadeString: expression) let labelDefinition = AGSLabelDefinition(labelExpression: arcadeLabelExpression, textSymbol: trailHeadsTextSymbol) labelDefinition.placement = .pointAboveCenter return labelDefinition }
-
Add a method named
add
.Trailheads Layer() Use an
AGSPictureMarkerSymbol
to draw a trailhead hiker image. Use theAGSLabelDefinition
to label each trailhead by its name.ViewController.swiftUse dark colors for code blocks private func addTrailheadsLayer() { let featureLayer = addFeatureLayer(url: .trailheads) let pictureMarkerSymbol = AGSPictureMarkerSymbol(url: .trailheadImage) pictureMarkerSymbol.height = 18.0 pictureMarkerSymbol.width = 18.0 let simpleRenderer = AGSSimpleRenderer(symbol: pictureMarkerSymbol) featureLayer.renderer = simpleRenderer featureLayer.labelsEnabled = true let trailHeadsDefinition = makeLabelDefinition() featureLayer.labelDefinitions.addObjects(from: [trailHeadsDefinition]) }
-
Update
view
to call the newD i d Load() add
method.Trailheads Layer() ViewController.swiftUse dark colors for code blocks override func viewDidLoad() { super.viewDidLoad() setupMap() addOpenSpaceLayer() addTrailsLayer() addNoBikeTrailsLayer() addBikeOnlyTrailsLayer() addTrailheadsLayer() }
-
Press Command + R to run the app.
If you are using the Xcode simulator your system must meet these minimum requirements: macOS Big Sur 11.3, Xcode 13, iOS 13. If you are using a physical device, then refer to the system requirements.
When the app opens, all the layers you've created and symbolized are displayed on the map.
- Parks and open spaces are displayed with four unique symbols
- Trails use different symbols (line widths) depending on trail elevation
- Trails are blue where bikes are permitted and red where they are prohibited
- Trailheads are displayed with a hiker icon and labels display each trail's name
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: