Feature layers, graphics overlays, and map image layer sublayers in your ArcGIS Runtime app can be labeled using a combination of attribute values, text strings, and values calculated with an expression. You can determine how labels are positioned and prioritized, and how conflicts between overlapping labels are automatically and dynamically resolved.
For feature layers, graphics overlays, and map image sublayers, labeling is implemented using a collection of
AGSLabelDefinition
objects to define things like:
- What labels look like (font, size, color, angle, and so on).
- At which scales they should be visible.
- The text to display (perhaps using attribute values).
- Which geoelements should be labeled.
- How to handle overlaps and prioritize labels.
If you want to label everything in your layer or overlay the same way, you can define a single label definition. If you want to display different labels for different attribute values, you can add as many label definitions as you need to define distinct sets of geoelements for labeling.
Define labels
Label definitions establish the label text and how labels are rendered for a specified group of geoelements using attributes, which provide the label text as well as determine which label is applied to each geoelement. The map in the following image uses three label definitions: one with a large blue label for cities with populations over 1.5 million, smaller black labels for cities between 0.5 and 1.5 million, and a small gray label for cities under 0.5 million. For the label text, each definition uses the value from the name
attribute.
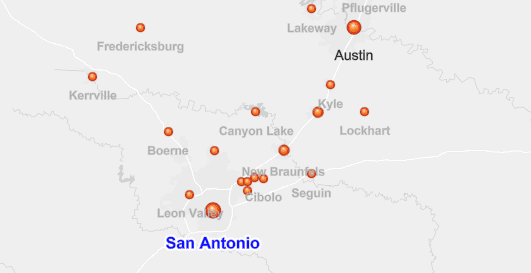
// Create a label definition with the appropriate symbol for small cities.
let expression = AGSArcadeLabelExpression(arcadeString: "return $feature.name;")
let labelDefSmall = AGSLabelDefinition(labelExpression: expression, textSymbol: textSymbolSmallCities)
labelDefSmall.whereClause = "[POPULATION] < 500000"
labelDefSmall.placement = AGSLabelingPlacement.pointBelowCenter
// Add the label definitions to the city layer’s label definitions collection.
citiesLayer.labelDefinitions.add(labelDefSmall)
// Make sure to enable labels for the layer so they appear.
citiesLayer.labelsEnabled = true
Each label's symbol, text, placement, overlap rules, and so on is represented with a
AGSLabelDefinition
and is constructed to conform to label properties defined in the Web map specification for labels. In order to provide a more accurate representation of labels created using ArcGIS Pro (for a mobile map package, for example), ArcGIS Runtime defines additional label properties that are not included in the web map specification. These properties provide additional control for things like the layout, orientation, and stacking of label text and label overlap behavior. See the
AGSLabelDefinition
class for details.
Most properties for a label definition are optional and will use a default value if not assigned explicitly. At a minimum, you must provide an expression that defines the label text and a symbol (with at least color, font size, and type) to display labels for a layer. Refer to the label properties section for a description of some common properties available for label definitions.
Label properties
The following list describes some common aspects of label display and behavior that you can control in a label definition. Relevant classes and members are shown in parentheses.
-
Which geoelements to label (
AGSLabelDefinition.whereClause
)—The geoelements labeled with the label definition are determined by evaluating an attribute expression. This expression is defined using SQL syntax, Arcade expressions are not supported for defining a label definition's where clause. If this expression is not defined, all elements are included in the definition. When using multiple label definitions, it's important that expressions uniquely assign geoelements for each definition. Creating multiple label definitions for a single layer is useful when you want to distinguish labels for certain types of elements. When labeling a cities layer, for example, you may want to distinguish capital cities from the others by using a larger font or different color. -
Label text (
AGSLabelDefinition.expression
,AGSSimpleLabelExpression
,AGSArcadeLabelExpression
,AGSWebmapLabelExpression
)—A label expression can be used to determine the text to display for each geoelement in the label definition. The label text can come from a combination of available attributes, text strings, and expressions. A label showing length in meters from values in feet from an attribute namedlength_
, for example, may use an Arcade expression like this:ft ($feature.length_
. A feature with aft * 0.3048) + ' meters' length_
value offt 343
would display a label of104.546 meters
. See the Label expressions section for more details and examples. -
Text symbol (
AGSLabelDefinition.textSymbol
,AGSTextSymbol
)—The font, size, color, angle, and so on, used to display labels in the definition. You can also provide a background color or halo for the text symbol. -
Display scale range (
AGSLabelDefinition.minScale
,AGSLabelDefinition.maxScale
)—The scale range, defined with a minimum and maximum scale denominator, at which labels in the definition will be displayed. A value of0
for one of the range values means that the end of the range is not restricted. -
Placement (
AGSLabelDefinition.placement
,AGSLabelingPlacement
)—Labels can be placed at a specified position relative to the elements they describe. There are different options for placement depending upon the geometry of the geoelements being labeled. Line features, for example, may have labels placed above the center of the line, below the center of the line, above the end point, and so on. The default is automatic. Depending upon the type of geometry, this means:- Above right for point labels
- Above along for line labels
- Always horizontal for polygon labels
-
Priority (
AGSLabelDefinition.priority
)—A label definition can be given a priority relative to other definitions in the layer. If labels from different definitions conflict in their placement, the label from the highest-priority definition will be displayed. Values indicate priority relative to other label definitions, with lower values indicating higher priority. If priority is not specified, a default prioritization is used based on geometry type: points (top priority), followed by lines, and then polygons. -
Label overlap (
AGSLabelDefinition.deconflictionStrategy
,AGSLabelDefinition.labelOverlapStrategy
,AGSLabelDeconflictionStrategy
,AGSLabelOverlapStrategy
)—Control label overlap with geoelements and other labels. -
Duplicate or repeating labels (
AGSLabelDefinition.removeDuplicatesStrategy
,AGSLabelDefinition.repeatStrategy
,AGSLabelDefinition.multipartStrategy
)—You can define preferences for removing duplicate labels within a specified radius as well as for repeating labels along lines at a constant interval. You can also control how geoelements with multipart geometry should be labeled.
Label display can also be affected by applying a reference scale to the map. Reference scale is the scale at which symbols and labels appear at their intended, true size. As you zoom in and out beyond the reference scale, symbols and text will increase or decrease in size relative to the reference scale to keep a consistent size on the map. If no reference scale is set, symbols remain a constant size on the screen and do not change size as you zoom in or out. Individual layers in a map can opt in or out of honoring the map's reference scale.
Label expressions
Depending upon your use case, you can define text for your labels using one of the following types of label expression classes:
-
AGSArcadeLabelExpression
—An expression that uses the ArcGIS Arcade expression language to define label text. -
AGSSimpleLabelExpression
—A simple label expression that uses the ArcGIS REST API labeling syntax. -
AGSWebmapLabelExpression
—A web map script to be read and evaluated by a web map expression interpreter.
Arcade expressions
Arcade is a simple, lightweight scripting language that can evaluate expressions at runtime. It was designed specifically for creating custom visualizations and labeling expressions in ArcGIS. Users can write, share, and execute custom expressions in ArcGIS Pro, ArcGIS Online, ArcGIS API for JavaScript, and ArcGIS Runtime.
You can access feature attributes using Arcade's $feature
global variable. For example, to label roads with a STREET_
field, you can refer to the value in that attribute with this syntax: $feature.STREET_
. Arcade also provides built-in functions for mathematical calculations, logical operations, and formatting that you can use in your expression. Consult the Arcade guide for details and usage examples.
When defining a label, the final line of the expression must evaluate to a string or a number.
The following Arcade expression reads the attribute values for wind speed and direction. It then uses conditional logic (When
function) to assign a cardinal direction based on azimuth. Another conditional function (IIf
) is used to return an empty string for wind speeds of 0
and a formatted label for all other values.
var DEG = $feature.WIND_DIRECT;
var SPEED = $feature.WIND_SPEED;
var DIR = When(SPEED == 0, '',
(DEG < 22.5 && DEG >= 0) || DEG > 337.5, 'N',
DEG >= 22.5 && DEG < 67.5, 'NE',
DEG >= 67.5 && DEG < 112.5, 'E',
DEG >= 112.5 && DEG < 157.5, 'SE',
DEG >= 157.5 && DEG < 202.5, 'S',
DEG >= 202.5 && DEG < 247.5, 'SW',
DEG >= 247.5 && DEG < 292.5, 'W',
DEG >= 292.5 && DEG < 337.5, 'NW', '');
return IIf(SPEED > 0, SPEED + ' mph ' + DIR, '');
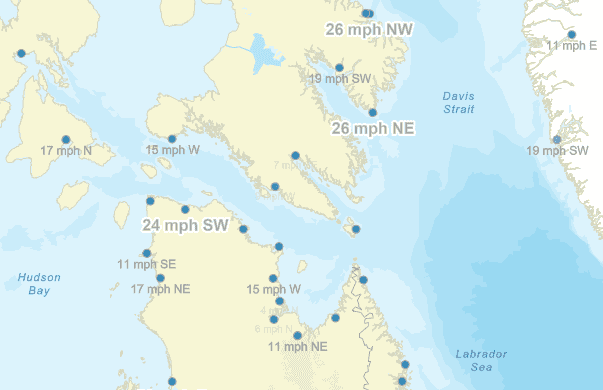
Simple expressions
Simple expressions use ArcGIS REST API syntax. You can use functions in the expression to provide basic formatting of values, such as ROUND()
, CONCAT
, and FORMATDATETIME()
. See JSON labeling objects for more information.
The simple expression below creates a label that combines static text, city name, and population. Note that the static text must be inside quotes.
[NAME] CONCAT NEWLINE CONCAT \"(Pop=\" CONCAT [POPULATION] CONCAT \")\"
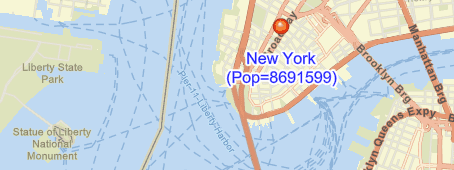
Web map expressions
A
AGSWebmapLabelExpression
expression provides a legal web map script to be read and evaluated by a web map expression interpreter.
A web map label expression that combines static text with an attribute value will look like this: State {State_
.
Note that unlike a simple or Arcade expression, quotes are not needed around the literal text.
Use text formatting tags
Text used for labels can contain some of the same formatting tags used by ArcGIS Pro. These tags are similar to HTML tags and are used to modify the appearance of the text. For the current release, ArcGIS Runtime supports the following tags:
<
—Font name, size, and scaling.FNT> <
—Color (defined with RGB or CMYK values).CLR> <
,BOL> <
—Bold / un-bold._ BOL> <
,ITA> <
—Italic / un-italic._ ITA> <
,UND> <
—Underline / un-underline._ UND>
These tags allow you to format parts of the text differently than what has been defined for the label's text symbol. The following expression, for example, displays the name
attribute with bold, underlined text. The rest of the text uses the unaltered text symbol previously defined for the label definition.
"return \"<BOL><UND>\" + $feature.name + \"</UND></BOL>\" + TextFormatting.NewLine +
\"(Population=\" + $feature.population + \")\";"
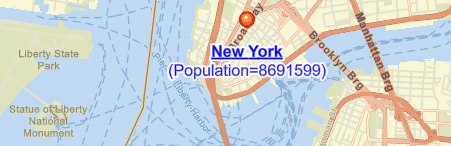
See the Text formatting tags topic in the ArcGIS Pro documentation for more information about these formatting tags and how they can be used with your labels.