Learn how to search for coffee shops, gas stations, restaurants and other nearby places with the geocoding service.
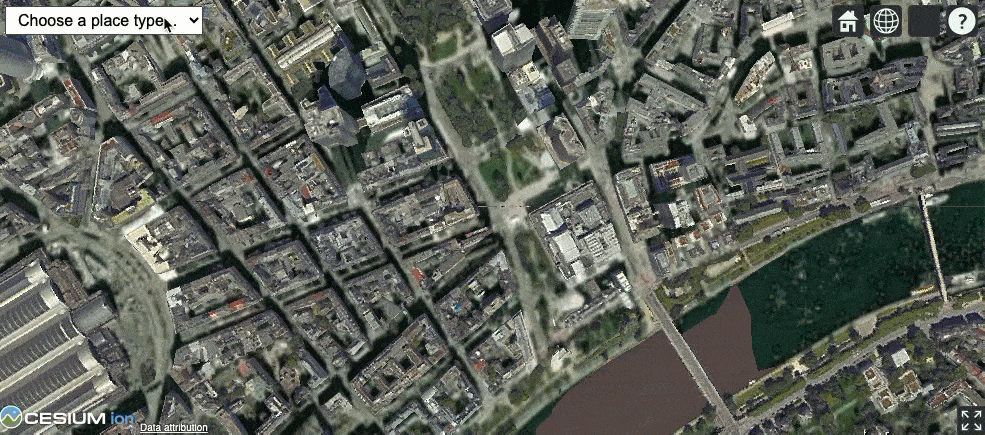
Place addresses for businesses by category with the geocoding service.
Place finding is the process of searching for a place name or POI to find its address and location. You can use the geocoding service to find places such as coffee shops, gas stations, or restaurants for any geographic location around the world. You can search for places by name or by using categories. You can search near a location or you can search globally.
In this tutorial, you use ArcGIS REST JS to access the geocoding service and find places by place category.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; Cesium.ArcGisMapService.defaultAccessToken = accessToken; const cesiumAccessToken = "YOUR_CESIUM_ACCESS_TOKEN"; Cesium.Ion.defaultAccessToken = cesiumAccessToken; const arcGisImagery = Cesium.ArcGisMapServerImageryProvider.fromBasemapType(Cesium.ArcGisBaseMapType.SATELLITE); const viewer = new Cesium.Viewer("cesiumContainer", { baseLayer: Cesium.ImageryLayer.fromProviderAsync(arcGisImagery), });
To learn about the other types of authentication available, go to Types of authentication.
Code
<!DOCTYPE html>
<html lang="en">
<head>
<title>CesiumJS: Find places</title>
<script src="https://cesium.com/downloads/cesiumjs/releases/1.114/Build/Cesium/Cesium.js"></script>
<link href="https://cesium.com/downloads/cesiumjs/releases/1.114/Build/Cesium/Widgets/widgets.css" rel="stylesheet">
<!-- Load ArcGIS REST JS from CDN -->
<script src="https://unpkg.com/@esri/arcgis-rest-request@4.0.0/dist/bundled/request.umd.js"></script>
<script src="https://unpkg.com/@esri/arcgis-rest-geocoding@4.0.0/dist/bundled/geocoding.umd.js"></script>
<style>
html, body, #cesiumContainer {
width:100%;
height:100%;
padding:0px;
margin:0px;
}
#places-select {
left: 8px;
top: 8px;
position: absolute;
font-size: 16px;
padding: 4px 8px;
}
</style>
</head>
<body>
<div id="cesiumContainer"></div>
<select id="places-select">
<option value="">Choose a place type...</option>
<option value="Coffee shop" selected>Coffee shops</option>
<option value="Gas station">Gas stations</option>
<option value="Food">Food</option>
<option value="Hotel">Hotels</option>
<option value="Parks and Outdoors">Parks and Outdoors</option>
</select>
<script type="module">
const accessToken = "YOUR_ACCESS_TOKEN";
Cesium.ArcGisMapService.defaultAccessToken = accessToken;
const authentication = arcgisRest.ApiKeyManager.fromKey(accessToken);
const cesiumAccessToken = "YOUR_CESIUM_ACCESS_TOKEN";
Cesium.Ion.defaultAccessToken = cesiumAccessToken;
const arcGisImagery = Cesium.ArcGisMapServerImageryProvider.fromBasemapType(Cesium.ArcGisBaseMapType.SATELLITE, {
enablePickFeatures:false
});
const viewer = new Cesium.Viewer("cesiumContainer", {
baseLayer: Cesium.ImageryLayer.fromProviderAsync(arcGisImagery),
terrain: Cesium.Terrain.fromWorldTerrain(),
timeline: false,
animation: false,
geocoder:false
});
viewer.camera.setView({
destination : Cesium.Cartesian3.fromDegrees(8.6724, 50.1085, 1500)
});
const geoidService = await Cesium.ArcGISTiledElevationTerrainProvider.fromUrl("https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/EGM2008/ImageServer");
const i3sProvider = await Cesium.I3SDataProvider.fromUrl("https://tiles.arcgis.com/tiles/cFEFS0EWrhfDeVw9/arcgis/rest/services/Buildings_Frankfurt_2021/SceneServer", {
geoidTiledTerrainProvider: geoidService,
token: accessToken
})
viewer.scene.primitives.add(i3sProvider);
function showPlaces() {
viewer.dataSources.removeAll()
const category = document.getElementById("places-select").value;
const cartographic = Cesium.Cartographic.fromCartesian(viewer.camera.position);
const center = [Cesium.Math.toDegrees(cartographic.longitude),Cesium.Math.toDegrees(cartographic.latitude)]
arcgisRest
.geocode({
authentication,
outFields: "Place_addr,PlaceName", // attributes to be returned
params: {
category,
location: center.join(','),
maxLocations: 25
}
})
.then((response) => {
const json = response.geoJson
Cesium.GeoJsonDataSource.load(response.geoJson, {
markerColor: Cesium.Color.ROYALBLUE,
markerSize:48,
clampToGround:true
}).then((data)=>{
viewer.dataSources.add(data)
console.log(data)
viewer.selectedEntity = data.entities.values[0]
})
})
}
document.getElementById("places-select").addEventListener("change", showPlaces);
showPlaces()
</script>
</body>
</html>
What's next?
Learn how to use additional ArcGIS location services in these tutorials: