Learn how to find an address or place using a search box and the geocoding service.
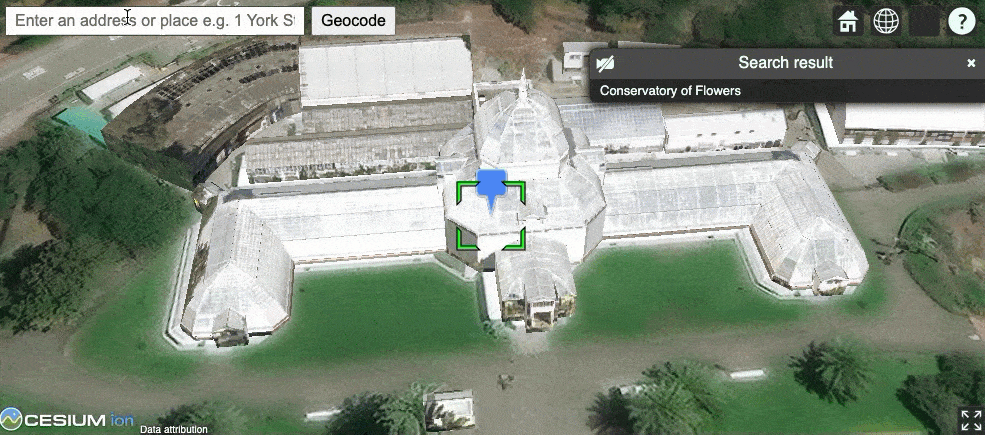
Find the location of addresses with the geocoding service.
Geocoding is the process of converting address or place text to a location. The geocoding service provides address and place geocoding and reverse geocoding.
In this tutorial, you use ArcGIS REST JS to access the geocoding service. You use a simple input control to accept text and a button to execute a search for an address or place. When an address or place is located, a pop-up will appear with the name, location, and address, and the view will pan to it.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a scene tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; Cesium.ArcGisMapService.defaultAccessToken = accessToken; const cesiumAccessToken = "YOUR_CESIUM_ACCESS_TOKEN"; Cesium.Ion.defaultAccessToken = cesiumAccessToken; const arcGisImagery = Cesium.ArcGisMapServerImageryProvider.fromBasemapType(Cesium.ArcGisBaseMapType.SATELLITE); const viewer = new Cesium.Viewer("cesiumContainer", { baseLayer: Cesium.ImageryLayer.fromProviderAsync(arcGisImagery), });
To learn about the other types of authentication available, go to Types of authentication.
Add references to ArcGIS REST JS
-
In the
<head>
element, reference thegeocoding
andrequest
packages from ArcGIS REST JS.Use dark colors for code blocks <script src="https://cesium.com/downloads/cesiumjs/releases/1.114/Build/Cesium/Cesium.js"></script> <link href="https://cesium.com/downloads/cesiumjs/releases/1.114/Build/Cesium/Widgets/widgets.css" rel="stylesheet"> <script src="https://unpkg.com/@esri/arcgis-rest-request@4.0.0/dist/bundled/request.umd.js"></script> <script src="https://unpkg.com/@esri/arcgis-rest-geocoding@4.0.0/dist/bundled/geocoding.umd.js"></script>
Update the scene
CesiumJS supports both 3D models and geocoding. Update the scene to display 3D buildings so that you can search for them with the geocoding service.
-
Update the scene view to center on location
[-122.4117, 37.769, 5000]
in San Francisco, CA.Use dark colors for code blocks viewer.camera.setView({ destination: Cesium.Cartesian3.fromDegrees(-122.4117, 37.769, 5000), orientation: { pitch: Cesium.Math.toRadians(-70) } })
-
Add the San Francisco 3D Buildings scene layer to visualize the city of San Francisco.
Use dark colors for code blocks viewer.camera.setView({ destination: Cesium.Cartesian3.fromDegrees(-122.4117, 37.769, 5000), orientation: { pitch: Cesium.Math.toRadians(-70) } }) const geoidService = await Cesium.ArcGISTiledElevationTerrainProvider.fromUrl("https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/EGM2008/ImageServer"); const i3sProvider = await Cesium.I3SDataProvider.fromUrl("https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/SanFrancisco_3DObjects_1_7/SceneServer",{ geoidTiledTerrainProvider: geoidService }); viewer.scene.primitives.add(i3sProvider);
Create geocoder controls
To trigger the geocode, users will type an address then click a button. Create an HTML <input>
control to accept user input and a <button>
control to initiate the query.
-
In the
<body>
, add a<div>
containing a text input element and a button element.Use dark colors for code blocks <body> <div id="cesiumContainer"></div> <div class="search"> <input id="geocode-input" type="text" placeholder="Enter an address or place e.g. 1 York St" size="50" /> <button id="geocode-button">Geocode</button> </div> <script type="module">
-
In the
<style>
section, style the controls so that they appear in the top left corner of the app.Use dark colors for code blocks <style> html, body, #cesiumContainer { width:100%; height:100%; padding:0px; margin:0px; } .search { position: absolute; top: 8px; left: 8px; } #geocode-input, #geocode-button { font-size: 16px; margin: 0 2px 0 0; padding: 4px 8px; } #geocode-input { width: 300px; } </style>
Call the geocoding service
When a user clicks the Geocode button, call arcgis
to geocode the user's search query.
arcgis
uses the geocoding service to perform a find
operation. To learn more about the operation, go to the REST API documentation.
-
Create a new
arcgis
using your access token to authenticate requests to the geocoding service.Rest.Api K e y Manager Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; Cesium.ArcGisMapService.defaultAccessToken = accessToken; const authentication = arcgisRest.ApiKeyManager.fromKey(accessToken);
-
Add an event listener to the geocoding button. When the button is clicked, call a new function called
find
with the user's input as a parameter.Address Use dark colors for code blocks const geoidService = await Cesium.ArcGISTiledElevationTerrainProvider.fromUrl("https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/EGM2008/ImageServer"); const i3sProvider = await Cesium.I3SDataProvider.fromUrl("https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/SanFrancisco_3DObjects_1_7/SceneServer",{ geoidTiledTerrainProvider: geoidService }); viewer.scene.primitives.add(i3sProvider); function findAddress(query) { } document.getElementById("geocode-button").addEventListener("click", () => { viewer.entities.removeAll(); const query = document.getElementById("geocode-input").value; findAddress(query); });
-
In the
find
function, callAddress arcgis
and pass theRest.geocode authentication
object. Set thesingle
parameter to the search query. Set theLine location
parameter to the camera's current position to prioritize search results near the current extent.Use dark colors for code blocks function findAddress(query) { const cameraPos = Cesium.Cartographic.fromCartesian(viewer.camera.position); const center = [Cesium.Math.toDegrees(cameraPos.longitude),Cesium.Math.toDegrees(cameraPos.latitude)] arcgisRest .geocode({ singleLine: query, authentication, params: { outFields: "*", location: center.join(",") } }) }
-
Access the service response to obtain query results. Alert the user if no results are returned.
Use dark colors for code blocks arcgisRest .geocode({ singleLine: query, authentication, params: { outFields: "*", location: center.join(",") } }) .then((response) => { const result = response.candidates[0]; if (!result === 0) { alert("That query didn't match any geocoding results."); return; } })
-
Check for issues in accessing the geocoding service (such as a network disruption or authentication problems) and alert the user.
Use dark colors for code blocks arcgisRest .geocode({ singleLine: query, authentication, params: { outFields: "*", location: center.join(",") } }) .then((response) => { const result = response.candidates[0]; if (!result === 0) { alert("That query didn't match any geocoding results."); return; } }) .catch((error) => { alert("There was a problem using the geocoder. See the console for details."); console.error(error); });
Display results
If the query is successful, the candidates
property of the response will contain a list of one of more address candidates. To display the top candidate, create a new entity and add it to your scene.
-
Add a new
Entity
to the viewer at the coordinates of the result. Set thename
anddescription
of the entity to display information about the result candidate.Use dark colors for code blocks .then((response) => { const result = response.candidates[0]; if (!result === 0) { alert("That query didn't match any geocoding results."); return; } const location = Cesium.Cartesian3.fromDegrees(result.location.x,result.location.y); const candidate = viewer.entities.add({ name:'Search result', description:`${result.address}`, position:location, }) })
-
Set the
billboard
property of your entity to display a pin on the map. Use aPin
to set theBuilder image
property of the pin.Use dark colors for code blocks const pinBuilder = new Cesium.PinBuilder() const location = Cesium.Cartesian3.fromDegrees(result.location.x,result.location.y); const candidate = viewer.entities.add({ name:'Search result', description:`${result.address}`, position:location, billboard: { verticalOrigin: Cesium.VerticalOrigin.BOTTOM, heightReference: Cesium.HeightReference.CLAMP_TO_GROUND, image: pinBuilder.fromColor(Cesium.Color.fromCssColorString('#5491f5'),48).toDataURL() } })
-
Select and track the result entity in order to display a pop-up and center the camera on the result.
Use dark colors for code blocks billboard: { verticalOrigin: Cesium.VerticalOrigin.BOTTOM, heightReference: Cesium.HeightReference.CLAMP_TO_GROUND, image: pinBuilder.fromColor(Cesium.Color.fromCssColorString('#5491f5'),48).toDataURL() } }) viewer.selectedEntity = candidate; viewer.trackedEntity = candidate;
Run the app
In CodePen, run your code to display the map.
In the input box, type "Ferry building San Francisco" and then click the Geocode button to find its location. If a location is found, the map will zoom to it and display a pop-up with the address.
What's next?
Learn how to use additional ArcGIS location services in these tutorials: