Learn how to find an address near a location with the geocoding service.
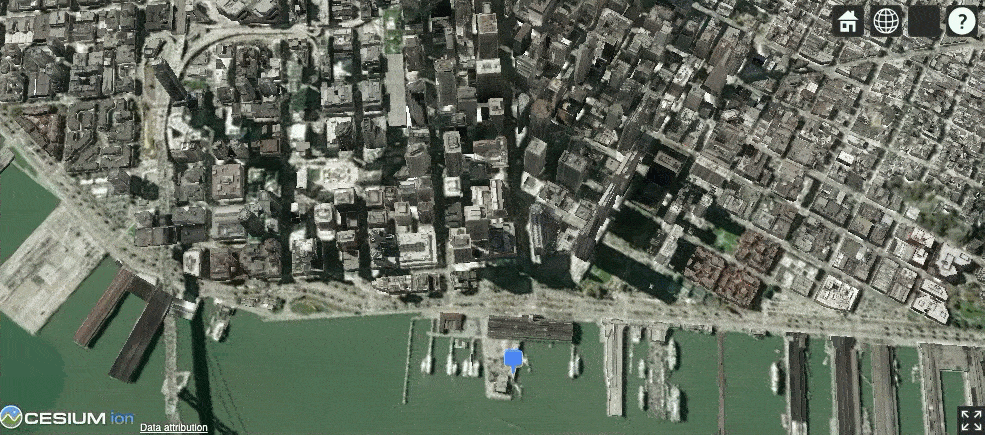
Reverse geocode coordinates to addresses with the geocoding service.
Reverse geocoding is the process of converting a location to an address or place. To reverse geocode, you use the geocoding service and the reverse
operation. This operation requires an initial location and returns an address with attributes such as place name and location.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; Cesium.ArcGisMapService.defaultAccessToken = accessToken; const cesiumAccessToken = "YOUR_CESIUM_ACCESS_TOKEN"; Cesium.Ion.defaultAccessToken = cesiumAccessToken; const arcGisImagery = Cesium.ArcGisMapServerImageryProvider.fromBasemapType(Cesium.ArcGisBaseMapType.SATELLITE); const viewer = new Cesium.Viewer("cesiumContainer", { baseLayer: Cesium.ImageryLayer.fromProviderAsync(arcGisImagery), });
To learn about the other types of authentication available, go to Types of authentication.
Code
<!DOCTYPE html>
<html lang="en">
<head>
<title>CesiumJS: Reverse Geocode</title>
<script src="https://cesium.com/downloads/cesiumjs/releases/1.114/Build/Cesium/Cesium.js"></script>
<link href="https://cesium.com/downloads/cesiumjs/releases/1.114/Build/Cesium/Widgets/widgets.css" rel="stylesheet">
<!-- Load ArcGIS REST JS from CDN -->
<script src="https://unpkg.com/@esri/arcgis-rest-request@4.0.0/dist/bundled/request.umd.js"></script>
<script src="https://unpkg.com/@esri/arcgis-rest-geocoding@4.0.0/dist/bundled/geocoding.umd.js"></script>
<style>
html,
body {
margin: 0px;
padding: 0px;
height: 100%;
}
#cesiumContainer {
height: 100%;
}
</style>
</head>
<body>
<div id="cesiumContainer"></div>
<script type="module">
const accessToken = "YOUR_ACCESS_TOKEN";
Cesium.ArcGisMapService.defaultAccessToken = accessToken;
const authentication = arcgisRest.ApiKeyManager.fromKey(accessToken);
const cesiumAccessToken = "YOUR_CESIUM_ACCESS_TOKEN";
Cesium.Ion.defaultAccessToken = cesiumAccessToken;
const arcGisImagery = Cesium.ArcGisMapServerImageryProvider.fromBasemapType(Cesium.ArcGisBaseMapType.SATELLITE, {
enablePickFeatures:false
});
const viewer = new Cesium.Viewer("cesiumContainer", {
baseLayer: Cesium.ImageryLayer.fromProviderAsync(arcGisImagery),
terrain: Cesium.Terrain.fromWorldTerrain(),
timeline: false,
animation: false,
geocoder:false
});
viewer.camera.setView({
destination: Cesium.Cartesian3.fromDegrees(-122.388805,37.797517,2000),
orientation: {
heading: Cesium.Math.toRadians(-130),
pitch: Cesium.Math.toRadians(-70.0),
}
});
const geoidService = await Cesium.ArcGISTiledElevationTerrainProvider.fromUrl("https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/EGM2008/ImageServer");
const i3sProvider = await Cesium.I3SDataProvider.fromUrl("https://tiles.arcgis.com/tiles/z2tnIkrLQ2BRzr6P/arcgis/rest/services/SanFrancisco_3DObjects_1_7/SceneServer",{
geoidTiledTerrainProvider: geoidService
});
viewer.scene.primitives.add(i3sProvider);
function onLeftClick(movement) {
viewer.entities.removeAll();
const pickedPosition = viewer.scene.pickPosition(movement.position);
const cartographic = Cesium.Cartographic.fromCartesian(pickedPosition);
const originLatLng = [Cesium.Math.toDegrees(cartographic.longitude),Cesium.Math.toDegrees(cartographic.latitude)]
findAddress(originLatLng);
}
viewer.screenSpaceEventHandler.setInputAction(onLeftClick,Cesium.ScreenSpaceEventType.LEFT_CLICK);
function findAddress(coordinates) {
arcgisRest
.reverseGeocode(coordinates, {
authentication
})
.then((response) => {
const pinBuilder = new Cesium.PinBuilder();
const location = Cesium.Cartesian3.fromDegrees(response.location.x,response.location.y);
const result = viewer.entities.add({
name:`${response.location.x.toLocaleString()}, ${response.location.y.toLocaleString()}`,
description:`${response.address.LongLabel}`,
position:location,
billboard: {
verticalOrigin: Cesium.VerticalOrigin.BOTTOM,
heightReference: Cesium.HeightReference.CLAMP_TO_GROUND,
scale:0.6,
image: pinBuilder.fromColor(Cesium.Color.fromCssColorString('#5491f5'),48).toDataURL(),
}
})
viewer.selectedEntity = result;
})
}
findAddress([-122.392, 37.796])
</script>
</body>
</html>
What's next?
Learn how to use additional ArcGIS location services in these tutorials: