Learn how to add features from a feature service to a scene.
Features are a type of geographic data that contain a geometry and attributes. They are typically hosted in an ArcGIS feature service. You can query a feature service to return features formatted as GeoJSON.
This tutorial explains how to get features from a feature service with ArcGIS REST JS, then display them in your CesiumJS application as a Geo
. The tutorial uses three different feature layers, containing points, lines, and polygons.
The three layers you will use are:
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a scene tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Item access
- Note: If you are using your own custom data layer for this tutorial, you need to grant the API key credentials access to the layer item. Learn more in Item access privileges.
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; Cesium.ArcGisMapService.defaultAccessToken = accessToken; const cesiumAccessToken = "YOUR_CESIUM_ACCESS_TOKEN"; Cesium.Ion.defaultAccessToken = cesiumAccessToken; const arcGisImagery = Cesium.ArcGisMapServerImageryProvider.fromBasemapType(Cesium.ArcGisBaseMapType.SATELLITE); const viewer = new Cesium.Viewer("cesiumContainer", { baseLayer: Cesium.ImageryLayer.fromProviderAsync(arcGisImagery), });
To learn about the other types of authentication available, go to Types of authentication.
Add references to ArcGIS REST JS
-
In the
<head>
element, reference thefeature-service
andrequest
packages from ArcGIS REST JS.Use dark colors for code blocks <script src="https://cesium.com/downloads/cesiumjs/releases/1.114/Build/Cesium/Cesium.js"></script> <link href="https://cesium.com/downloads/cesiumjs/releases/1.114/Build/Cesium/Widgets/widgets.css" rel="stylesheet"> <script src="https://unpkg.com/@esri/arcgis-rest-request@4.0.0/dist/bundled/request.umd.js"></script> <script src="https://unpkg.com/@esri/arcgis-rest-feature-service@4.0.0/dist/bundled/feature-service.umd.js"></script>
-
In the
<body>
, create anarcgis
using your access token to authenticate requests to the feature service.Rest.Api K e y Manager Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; Cesium.ArcGisMapService.defaultAccessToken = accessToken; const authentication = arcgisRest.ApiKeyManager.fromKey(accessToken);
Add point features
Point features are typically displayed on top of all other layers. Use arcgis
to query the Trailheads feature service and add features to the scene as GeoJSON.
-
Go to the Trailheads URL and browse the properties of the layer. Make note of the layer's Name, Type, and Fields.
-
In CodePen, define a variable to store the URL of the feature service.
Use dark colors for code blocks const pointLayerName = "Trailheads"; const pointLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+pointLayerName+"/FeatureServer/0";
-
Call the
arcgis
operation to make an authenticated request to the Trailheads feature service. Set theRest.query Features f
property togeojson
to format the response as GeoJSON.Use dark colors for code blocks const pointLayerName = "Trailheads"; const pointLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+pointLayerName+"/FeatureServer/0"; arcgisRest.queryFeatures({ url: pointLayerURL, authentication, f:"geojson" }).then((response) => { });
-
Add the service response service to the scene as a
Geo
. Set theJson Data Source clamp
property to clamp the features to terrain.To Ground Use dark colors for code blocks const pointLayerName = "Trailheads"; const pointLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+pointLayerName+"/FeatureServer/0"; arcgisRest.queryFeatures({ url: pointLayerURL, authentication, f:"geojson" }).then((response) => { const data = Cesium.GeoJsonDataSource.load(response,{ clampToGround:true }) viewer.dataSources.add(data); });
Add polyline features
Line features are typically displayed below points. Use arcgis
to query the Trails feature service and add features to the scene as GeoJSON.
-
Go to the Trails URL and browse the properties of the layer. Make note of the layer's Name, Type, and Fields.
-
In CodePen, define a variable to store the URL of the feature service.
Use dark colors for code blocks const lineLayerName = "Trails"; const lineLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+lineLayerName+"/FeatureServer/0";
-
Call the
arcgis
operation to make an authenticated request to the Trails feature service. Set theRest.query Features f
property togeojson
to format the response as GeoJSON.Use dark colors for code blocks const lineLayerName = "Trails"; const lineLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+lineLayerName+"/FeatureServer/0"; arcgisRest.queryFeatures({ url: lineLayerURL, authentication, f:"geojson" }).then((response) => { });
-
Add the service response service to your scene as a
Geo
. Set theJson Data Source clamp
property to clamp the features to terrain.To Ground Use dark colors for code blocks const lineLayerName = "Trails"; const lineLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+lineLayerName+"/FeatureServer/0"; arcgisRest.queryFeatures({ url: lineLayerURL, authentication, f:"geojson" }).then((response) => { const data = Cesium.GeoJsonDataSource.load(response,{ clampToGround:true }) viewer.dataSources.add(data); });
Add polygon features
Polygon features are typically displayed below lines and points. Use arcgis
to query the Parks and Open Spaces feature service and add features to the scene as GeoJSON.
-
Go to the Parks and Open Spaces URL and browse the properties of the layer. Make note of the layer's Name, Type, and Fields.
-
In CodePen, define a variable to store the URL of the feature service.
Use dark colors for code blocks const polygonLayerName = "Parks_and_Open_Space"; const polygonLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+polygonLayerName+"/FeatureServer/0";
-
Call the
arcgis
operation to make an authenticated request to the Parks and Open Spaces feature service. Set theRest.query Features f
property togeojson
to format the response as GeoJSON.Use dark colors for code blocks const polygonLayerName = "Parks_and_Open_Space"; const polygonLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+polygonLayerName+"/FeatureServer/0"; arcgisRest.queryFeatures({ url: polygonLayerURL, authentication, f:"geojson" }).then((response) => { });
-
Add the service response service to your scene as a
Geo
. Set theJson Data Source clamp
property to clamp the features to terrain.To Ground Use dark colors for code blocks const polygonLayerName = "Parks_and_Open_Space"; const polygonLayerURL = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/"+polygonLayerName+"/FeatureServer/0"; arcgisRest.queryFeatures({ url: polygonLayerURL, authentication, f:"geojson" }).then((response) => { const data = Cesium.GeoJsonDataSource.load(response,{ clampToGround:true }) viewer.dataSources.add(data); });
Run the app
In CodePen, run your code to display the map.
Your map should display the locations of trailheads, trails, and open spaces in the Santa Monica Mountains.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
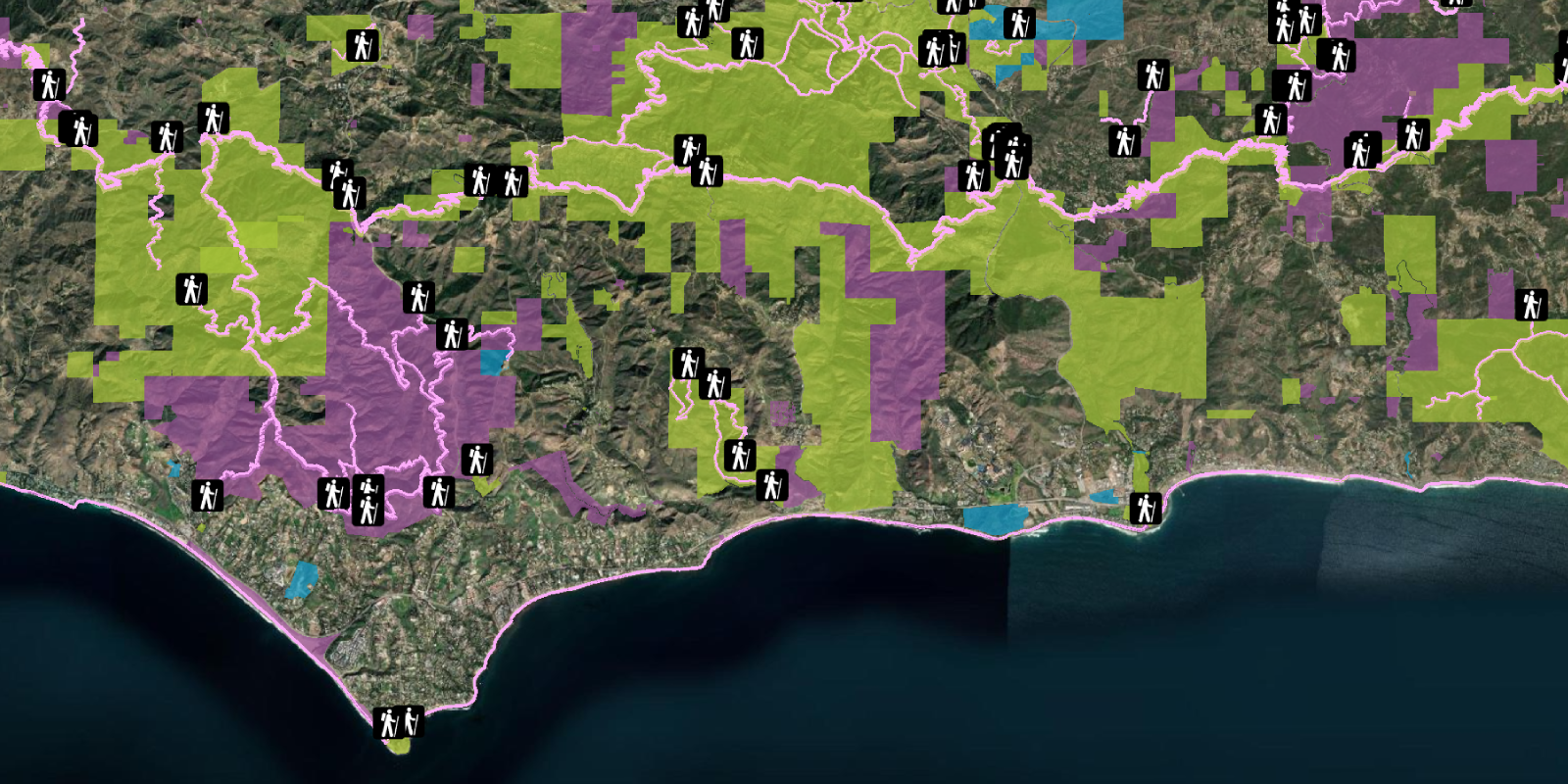
Style features
Use data-driven styling to apply symbol colors and styles to features.
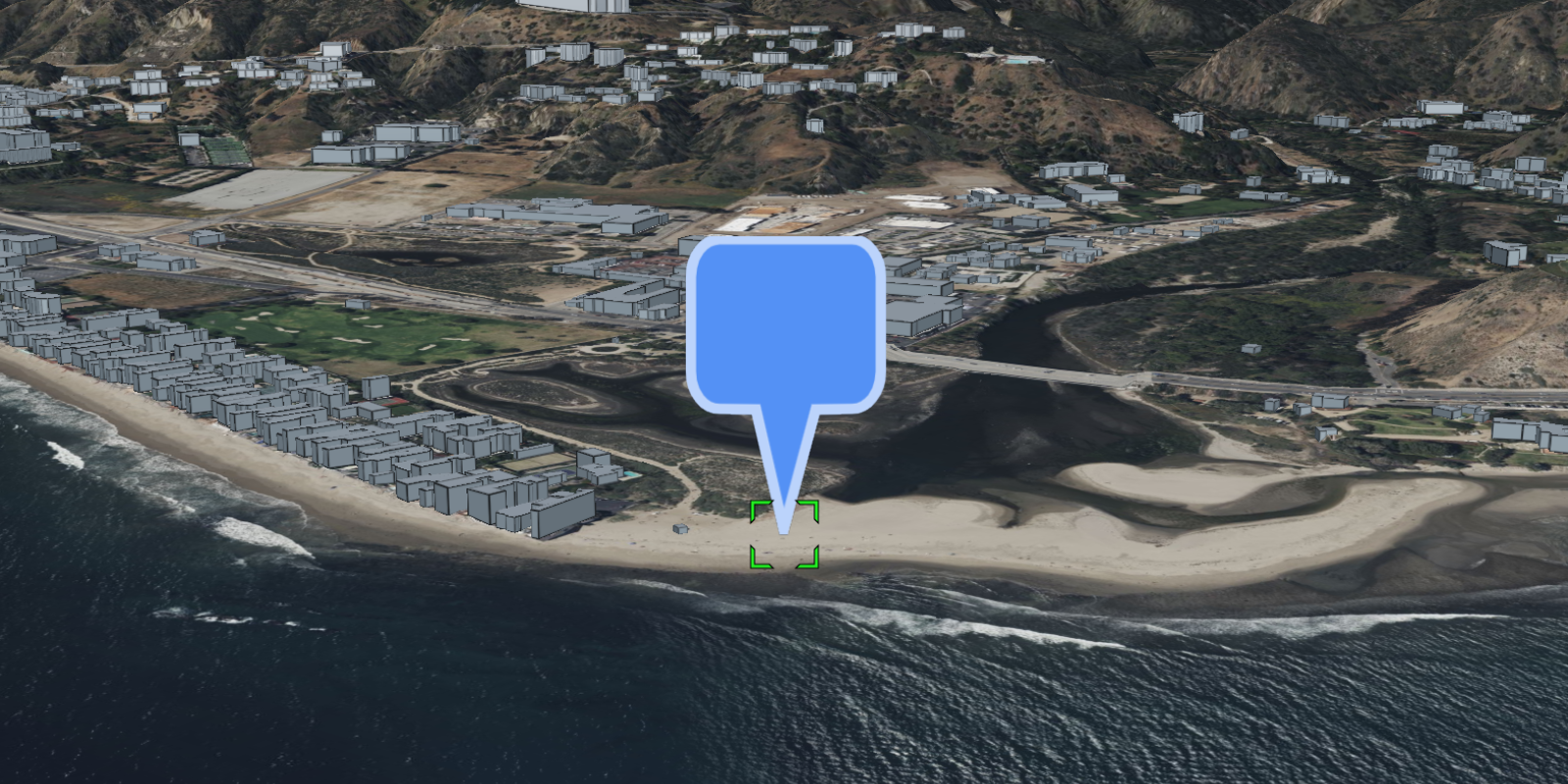
Display a pop-up (Feature)
Display feature attributes in a popup.
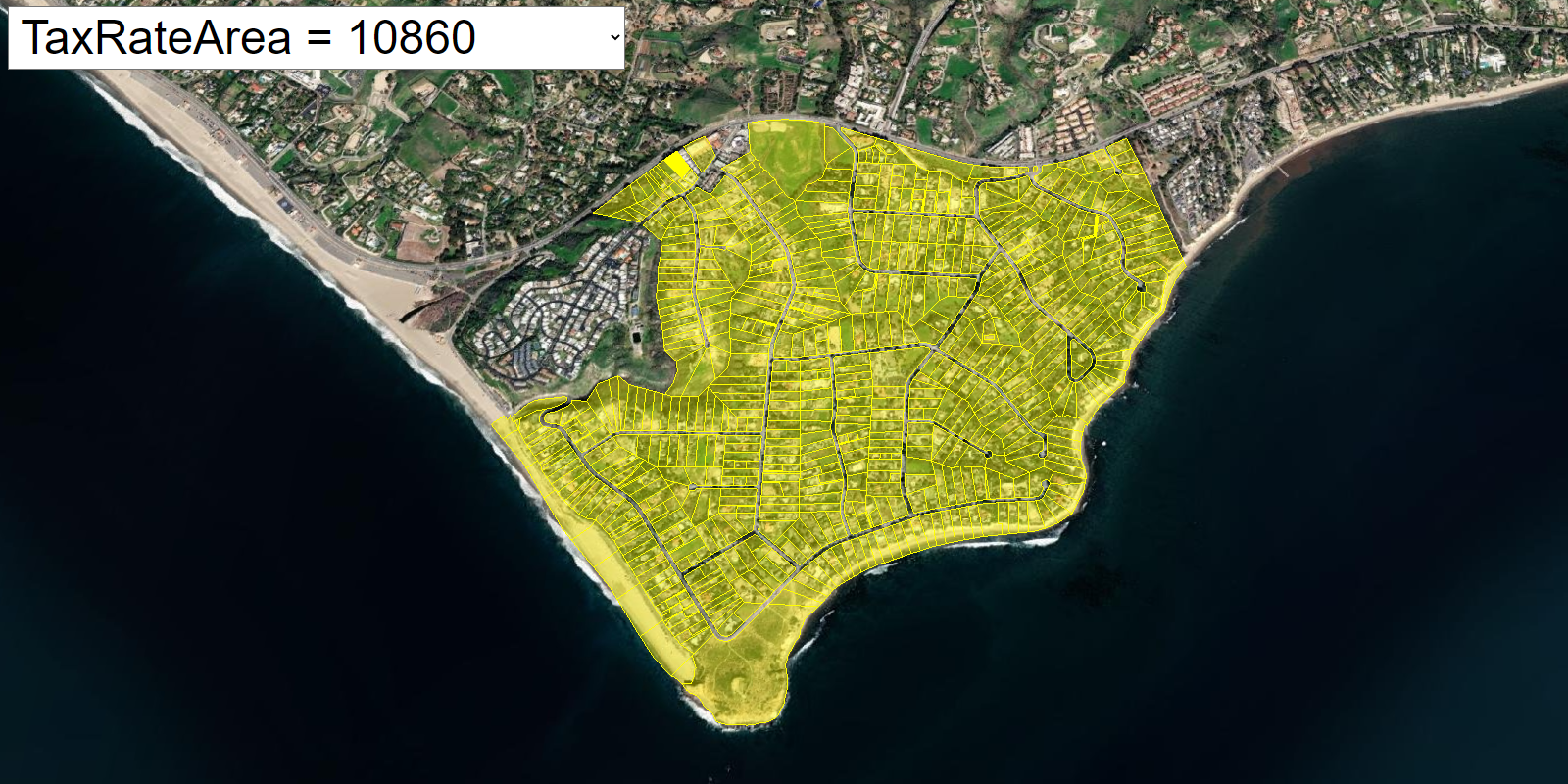
Query features (SQL)
Execute a SQL query to access polygon features from a feature service.
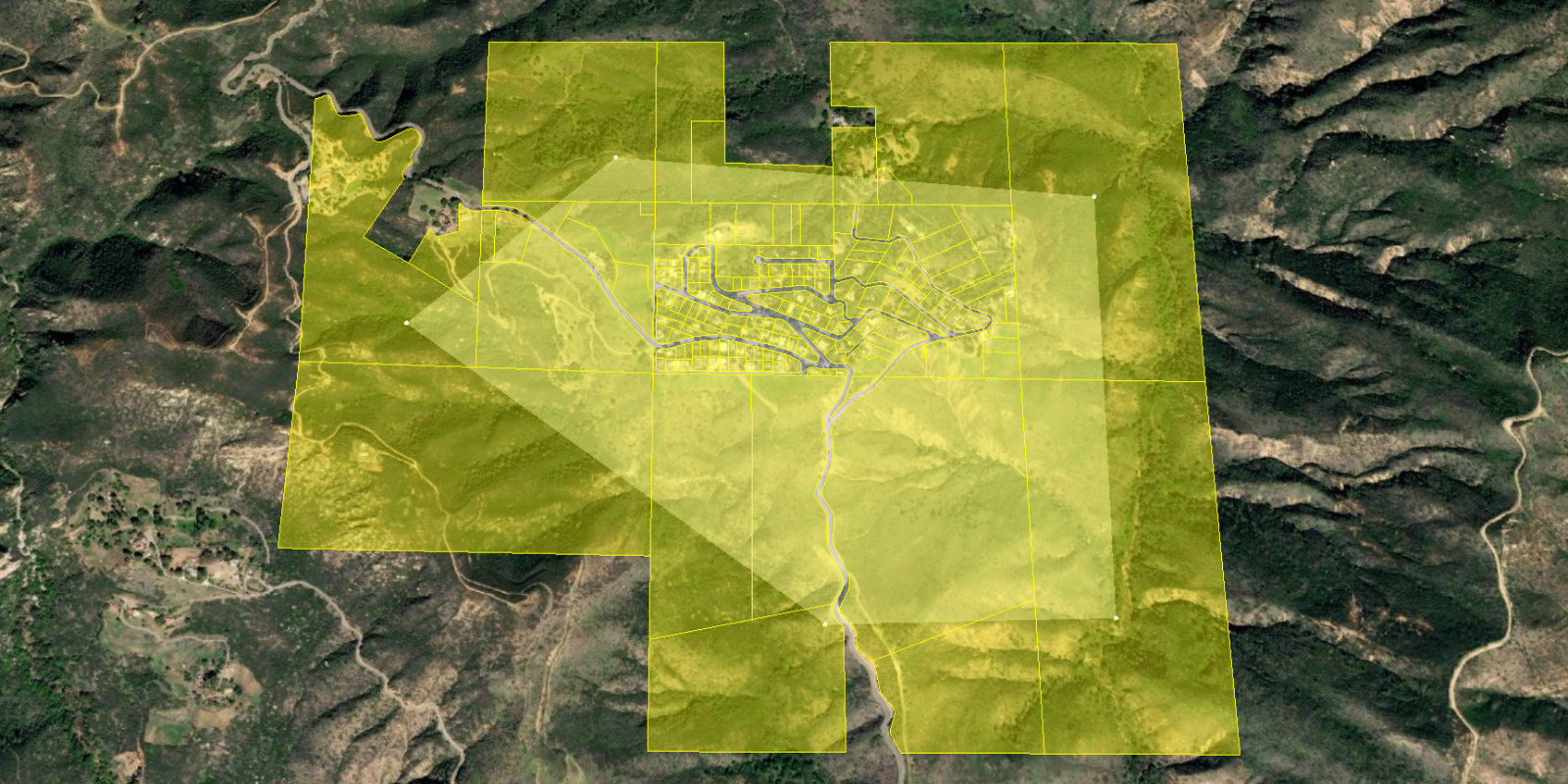
Query features (spatial)
Execute a spatial query to access polygon features from a feature service.