One of the difficulties with displaying a round earth in two dimensions is that, unlike a 3D representation that provides a continuous surface, a 2D representation must have a start and an end. While the earth must be divided along a line of longitude to "flatten" it for display in two dimensions (usually at 180º east/west longitude), a map in your ArcGIS Maps SDK for Java app can still wraparound the edges to provide a continuous display when panning east or west.
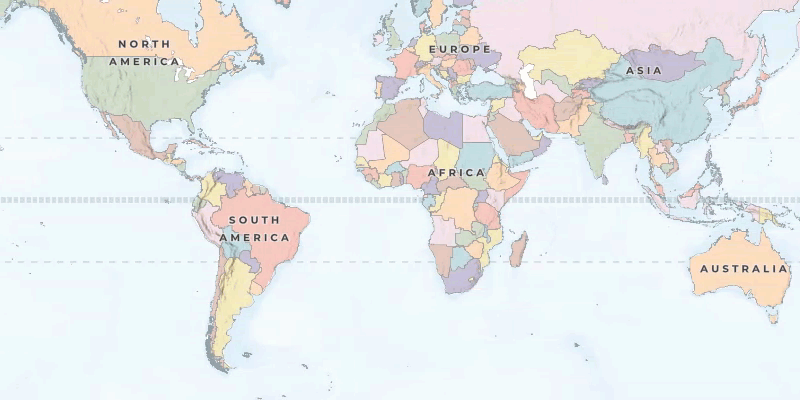
Enable or disable wraparound
By default, a map view attempts to wrap your map for a continuous experience as the user pans east and west. To disable wraparound behavior for a map view (or to re-enable it), you can set the wraparound mode to the appropriate value. Wraparound can only be applied to a map view if all the following requirements are met.
- The map's spatial reference covers the world. It's common for the full extent of a wraparound map to cover the world, but it is not required.
- The map's spatial reference supports panning horizontally over the antimeridian, indicated by a
true
value for theSpatialReference.isPannable()
property. Pannable spatial references include WGS 84 (WKID=4326) and Web Mercator (WKID=102113, 102100, or 3857). All tiled layers in the map must also use one of these spatial references. Dynamic layers, however, can be in any spatial reference because they are capable of reprojecting their data. - Dynamic layers in the map are based on services from ArcGIS Server 10.0 or higher. Earlier versions of the REST API do not support well-known text (WKT) values for spatial reference, which is required for making dynamic map services support wraparound.
If any of these requirements are not met, attempting to enable wraparound will fail silently (without alerting you through an error message).
The following example toggles the current wraparound mode for the map view. Wraparound behavior is disabled if it is currently enabled. If it is disabled, an attempt is made to enable wraparound if it is supported.
// Toggle the wraparound setting for the map view.
if (mapView.getWrapAroundMode() == WrapAroundMode.ENABLE_WHEN_SUPPORTED) {
//Disable wraparound if currently enabled.
mapView.setWrapAroundMode(WrapAroundMode.DISABLED);
} else {
// If it's disabled, attempt to enable wraparound mode.
mapView.setWrapAroundMode(WrapAroundMode.ENABLE_WHEN_SUPPORTED);
// Check if wraparound is now enabled.
if (!mapView.isWrapAroundEnabled()) {
// If wraparound is not now enabled, it is not supported.
new Alert(Alert.AlertType.ERROR, "Unable to enable wrap around");
}
}
Coordinates in wraparound mode
To better understand map navigation with wraparound, visualize a map as being composed of frames. The initial full extent of a wraparound-enabled map is frame 0. Assuming the map is in the WGS 84 coordinate system, frame 0 has X coordinates between -180° (west) and +180º (east) longitude.
Adjacent to this frame to the east is frame 1, which extends hypothetically between +180º and +540º longitude. If you pan the map eastwards until you pass 180º, you will be viewing frame 1. Similarly, adjacent to frame 0 to the west is frame -1, which extends hypothetically between -180º and -540º longitude. The series of frames continues infinitely in both directions.
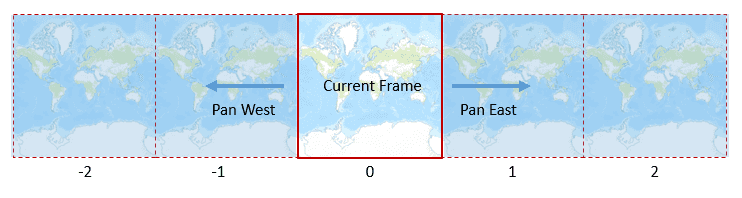
Longitude values (X coordinates) returned from a map may be real: in the range of -180º and +180º (within frame 0 in other words) or they may be hypothetical: less than -180º or greater than +180º (outside of frame 0). Here are some geometries that may contain hypothetical coordinates if wraparound is enabled:
- The map's bounding geometry or center point, returned as properties of the
Viewpoint
- Point locations on the map, converted from screen coordinates to map coordinates
- Geometries drawn on the display by the user (using
GeometryEditor
, for example)
Normalize geometries
The process of converting a geometry to contain only real coordinate values is called normalization. Geometries that contain hypothetical values are not acceptable as inputs to spatial queries, projection, geocoding services, routing services, or for storage in a geodatabase. Rather than trying to determine if a complex shape contains hypothetical coordinate values, it's best practice to always normalize geometry returned from the map when wraparound is enabled.
You can normalize geometries using GeometryEngine.normalizeCentralMeridian()
.
// If wraparound is enabled, normalize the geometry (in case all or part is outside of frame 0).
if (mapView.isWrapAroundEnabled()) {
extent = GeometryEngine.normalizeCentralMeridian(mapView.getVisibleArea());
}