You can use ArcGIS Maps SDKs for Native Apps to create real-time apps that consume a stream of data from a supported data source, such as an ArcGIS stream service or a custom DynamicEntityDataSource
. ArcGIS Maps SDK for Java abstracts much of the work required to connect to a stream, consume incoming data, and display it dynamically in your app. The real-world objects described by data in a stream are called dynamic entities.
Dynamic entities allow you to do things like:
- Track moving assets, such as vehicles in a delivery fleet, with updates to their current location, speed, estimated arrival, fuel level, and so on.
- Monitor stationary sensors, such as weather stations, with updates to attributes such as wind speed and direction, precipitation, and temperature.
- Get notified of new incidents, such as crime or accidents, and locate available responders in the area.
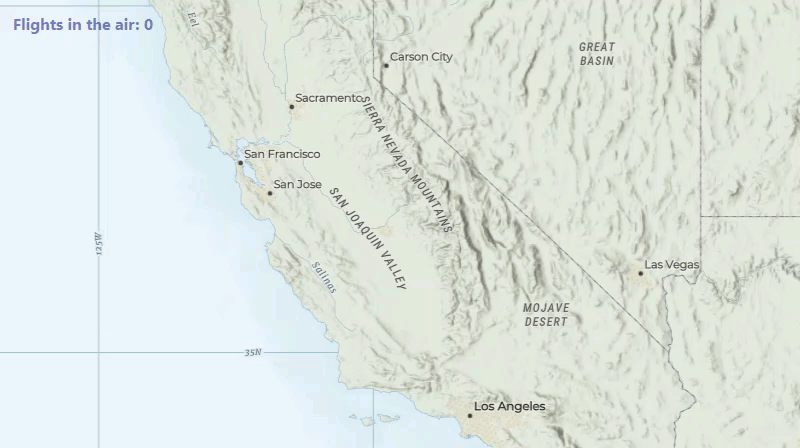
Dynamic entities and observations
Real-world objects that change over time are represented by the DynamicEntity
class. DynamicEntity
, like Graphic
and ArcGISFeature
, is a type of GeoElement
. This means it has a collection of attributes and a geometry to describe its location. Dynamic entities might be moving (like vehicles or people), stationary (like monitoring stations or gauges), or point-in-time events (such as crime or accident incidents).
Dynamic entities are updated with data coming from a stream. These updates are referred to as observations and are represented by the DynamicEntityObservation
class (also a type of GeoElement
). An observation is a static snapshot of the state of a dynamic entity at a given time. This snapshot includes the associated dynamic entity's location (geometry) as well as all its attributes. For example, an observation for a moving aircraft may include updates to attributes like speed, altitude, and heading in addition to its updated location. While observations remain static, a dynamic entity changes because it's essentially a pointer to the most recent observation. Observations can be identified in a map view or scene view.
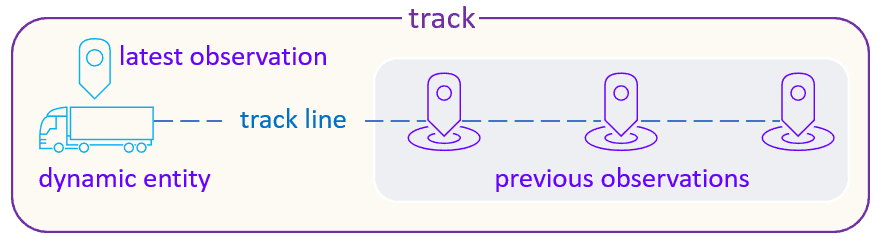
For any dynamic entity, you can get a collection of all its observations. Likewise, for any observation, you can find the dynamic entity to which it belongs. The collection of all observations for a given dynamic entity is often referred to as a track. Observations with the same track id belong to the same track and refer to the same dynamic entity. Tracks can be easily visualized with a track line connecting all observations of the same track.
Working with dynamic entities in your app involves the following broad steps:
Connect to a stream data source
In ArcGIS Maps SDK for Java, stream data sources are represented by the DynamicEntityDataSource
base class. This data source plays a central role in your real-time app by managing the connection to the underlying stream, managing the local data cache, and can be used to create a DynamicEntityLayer
for display in a map or scene.
The DynamicEntityDataSource
abstracts many of the details of working with the underlying stream. For example, it manages the connection to the stream, notifies of connection status changes, and automatically attempts to reconnect when needed. Members on DynamicEntityDataSource
can be used to purge all data from the local data cache, or define data management options for the local cache. It also notifies of incoming data updates and when data is purged (stale data is removed) from the DynamicEntityDataSource
.
Currently, ArcGIS Stream Service is the only data source that is directly supported out-of-the-box, but you can also create a custom dynamic entity data source from the DynamicEntityDataSource
base class to wrap any underlying stream. Your custom dynamic entity data sources can expose data from things like an RSS feed, a text file, a feature service, or a proprietary peer-to-peer feed.
At a minimum, a custom DynamicEntityDataSource
must provide logic for when the data source loads, when it connects to the underlying data feed, and when it disconnects from the feed. When your data source loads, you must provide basic metadata to identify the track ID field, define the attributes for the dynamic entities, and specify the spatial reference used to describe locations. See the Add custom dynamic entity data source sample for an example of a custom dynamic entity data source.
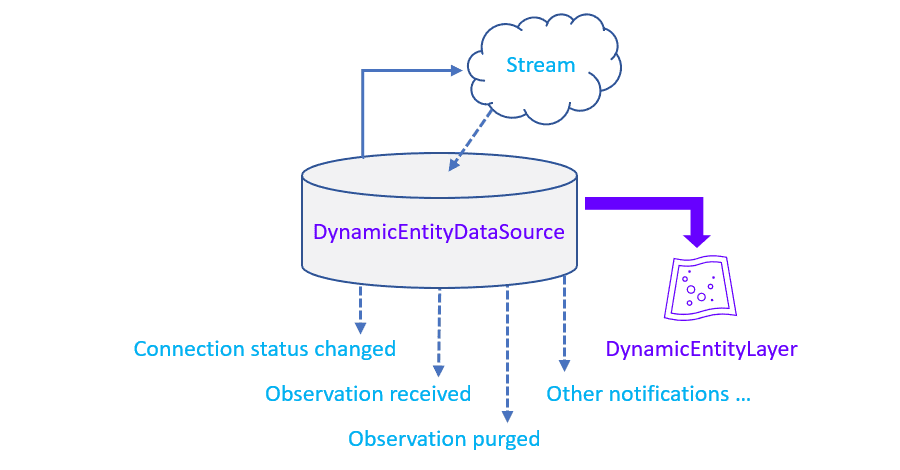
// Create an ArcGIS stream service using a stream service URL.
ArcGISStreamService streamService = new ArcGISStreamService(streamServiceUrl);
If you are using a dynamic entity layer, the stream service will automatically load and connect when it is added to the map. If you are using the dynamic entity data source without a dynamic entity layer, then you will need to explicitly load and connect the dynamic entity data source to begin recieving events/updates. Once the connection is successfully established, data will begin to flow from the stream.
// Connect the stream service to begin streaming dynamic entities and observations.
streamService.connectAsync();
Stream metadata
A DynamicEntityDataSource
may have metadata that describes the service. ArcGISStreamService
, for example, provides the ArcGISStreamServiceInfo
class that you can use to get information about the data provided by the stream. Once a ArcGISStreamService
is loaded, you can use its metadata to find things like:
- Fields: Field definitions for data in the stream
- Track ID field: The field that contains a unique ID for each track in a track-aware service
- Archive service URL: A service that contains the latest observations for dynamic entities provided by this stream
- Geometry type: The type of geometry returned (point, polyline, or polygon, for example)
ArcGIS stream service filter
For an ArcGISStreamService
, you can use an ArcGISStreamServiceFilter
to define which observations should be included based on spatial or attribute criteria (or both). For streams that deliver a lot of observations frequently, applying a filter can help keep the display uncluttered and the dynamic entity data source lean. If you're creating a custom dynamic entity data source, you can implement your own filtering logic.
// Add a filter for the data source to limit the amount of data received by the application.
ArcGISStreamServiceFilter streamServiceFilter = new ArcGISStreamServiceFilter();
streamServiceFilter.setGeometry(utahSandyEnvelope);
streamServiceFilter.setWhereClause("speed > 0");
streamService.setFilter(streamServiceFilter);
Purge options
Data received from the DynamicEntityDataSource
is stored in memory on the client and can grow quickly depending on the number of dynamic entities and frequency of updates. You can use the DynamicEntityDataSourcePurgeOptions
to control app data storage for the local cache. For example, you can define a limit on the number of observations stored either for the entire dynamic entity data source (maximum observations) or for each dynamic entity (maximum observations per track). By default, the maximum total number of observations stored is 100,000 (with no limit to the number of observations per track). You can also define the limit according to the age of observations, using the maximum duration purge option.
To clear all observations from the cache, use DynamicEntityDataSource.purgeAllAsync()
. This will clear all data from the local data store, but will not disconnect from the stream. Dynamic entities and observations will continue to be added to the dynamic entity data source as they come from the stream.
// Set a duration of five seconds for how long observation data is stored in the data source.
streamService.getPurgeOptions().setMaximumDuration(5);
// Keep no more than five observations per track.
streamService.getPurgeOptions().setMaximumObservationsPerTrack(5);
Display data coming from the stream
ArcGIS Maps SDK for Java provides a special type of layer for displaying dynamic entities, called DynamicEntityLayer
. This layer is created from a DynamicEntityDataSource
and manages the display of all dynamic entities and observations as they are added, purged, or updated. The renderer you define for the layer controls the display of dynamic entities and can be one of any of the available renderer types. You can define labels for dynamic entities as you would for other layers, but labels are not displayed for previous observations. See Symbols, renderers, and styles for more information about defining a renderer for a layer and Add labels for information about defining labels for geoelements.
Adding a DynamicEntityLayer
to a map or scene automatically loads and connects the associated DynamicEntityDataSource
.
Scene properties on the DynamicEntityLayer
are provided to define aspects of 3D display.
// Create a dynamic entity layer to display the data from the stream service.
DynamicEntityLayer dynamicEntityLayer = new DynamicEntityLayer(streamService);
// Set the renderer to the dynamic entity layer.
dynamicEntityLayer.setRenderer(entityRenderer);
dynamicEntityLayer.setLabelsEnabled(false);
// Add the dynamic entity layer to the map's operational layers.
map.getOperationalLayers().add(dynamicEntityLayer);
Track display properties
A dynamic entity layer's TrackDisplayProperties
allow you to control the display of previous observations by setting a maximum number of observations to show, displaying a line that connects them (track line), and applying any of the available renderers to the observations and/or track line. Note that the track line attributes collection includes only the entity ID attribute that uniquely identifies the track. This attribute can be used to display a symbol for each track using one of the attribute-based renderers.
The maximum observations defined for the track display properties applies strictly to the display. Don't confuse this property, used to control the display, with the maximum observations for the data source's purge options, used to limit the number of observations stored in the cache.
// Get the track display properties property value to control the display of previous observations
// and track lines.
TrackDisplayProperties layerTrackDisplayProperties = dynamicEntityLayer.getTrackDisplayProperties();
// Apply renderers for the previous observations and track lines.
layerTrackDisplayProperties.setPreviousObservationRenderer(previousObservationsRenderer);
layerTrackDisplayProperties.setTrackLineRenderer(trackLineRenderer);
// Configure checkboxes to control the visibility of track lines and previous observations.
trackLinesCheckBox.selectedProperty().bindBidirectional(
layerTrackDisplayProperties.showTrackLineProperty());
observationsCheckBox.selectedProperty().bindBidirectional(
layerTrackDisplayProperties.showPreviousObservationsProperty());
Select
DynamicEntityLayer
has methods for selecting or unselecting dynamic entities and observations in the display. When selecting a dynamic entity, the selection will move with the entity (as new observations come from the stream). Since observations are static, selected observations will appear like any static geoelement on the display.
// Select the new snow plow.
dynamicEntityLayer.selectDynamicEntity(observation.dynamicEntity());
// Unselect the snow plow in the layer.
dynamicEntityLayer.unselectDynamicEntity(observation.dynamicEntity());
Handle stream events
As information is delivered by the stream, the dynamic entity data source is continuously updated to reflect the dynamic entities and observations being added and removed (purged). While the DynamicEntityLayer
manages the display of the dynamic entities in the map or scene, you can also respond to notifications about dynamic entities being added or removed from the local data store.
The DynamicEntityDataSource
exposes the following notifications as it receives data from the stream.
dynamicEntityObservationReceivedEvent
—an observation is received from the stream.DynamicEntityObservationPurgedEvent
—an observation is purged from the local data source; for example, when the maximum observations or maximum observation duration has been surpassed.DynamicEntityReceivedEvent
—a new dynamic entity is received from the stream; for example, when the first observation for this dynamic entity is received.DynamicEntityPurgedEvent
—a dynamic entity is purged from the local data source; for example, when it no longer has any associated observations in the cache.
As a simple example, you could handle these events in order to increment or decrement a numeric variable to keep a count of the current number of entities in the data store.
// Handle new dynamic entities being added from the stream.
dynamicEntityDataSource.addDynamicEntityObservationReceivedListener(event -> {
dynamicEntity++;
});
// Handle dynamic entities being purged from the local data.
dynamicEntityDataSource.addDynamicEntityObservationPurgedListener(event -> {
dynamicEntity--;
});
You can also handle updates for a specific dynamic entity by handling its DynamicEntityChangedEvent
event. In the event handler, you can respond to observations as they are received or purged. You can also be notified when the entity itself is purged.
You can get notifications from connectionStatus
to respond to changes with the connection status. For example, you might want to display the connection status to the user. You might also want to clean up resources in your app or unsubscribe to notification events when the connection closes.
// Update the button text depending on the connection status value.
connectionButton.textProperty().bind(Bindings.createStringBinding(() -> {
if (streamService.getConnectionStatus() == ConnectionStatus.CONNECTED) {
return "Disconnect";
} else {
return "Connect";
}
}, streamService.connectionStatusProperty()));