Learn how to implement user authentication to access a secure ArcGIS service with OAuth credentials.
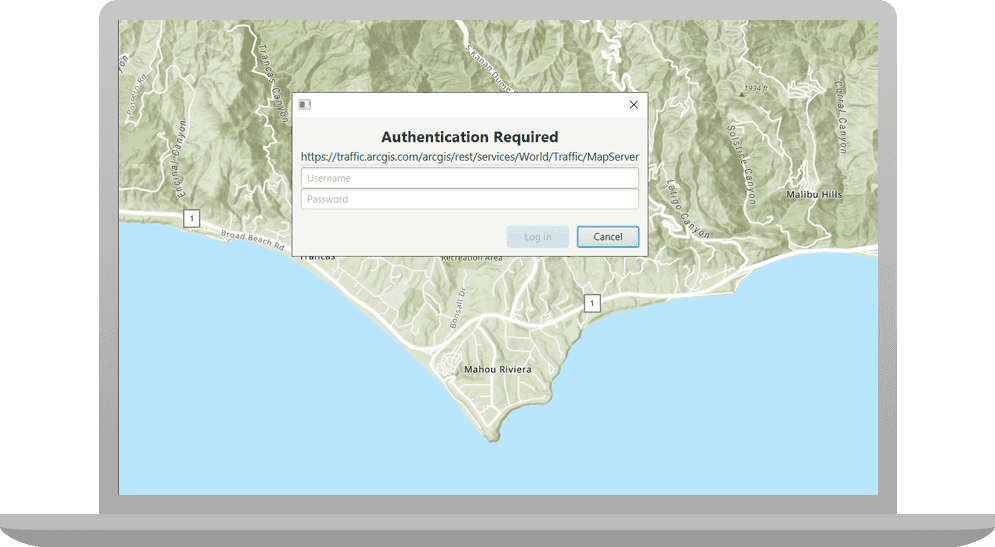
You can use different types of authentication to access secured ArcGIS services. To implement OAuth credentials for user authentication, you can use your ArcGIS account to register an app with your portal and get a Client ID, and then configure your app to redirect users to login with their credentials when the service or content is accessed. This is known as user authentication. If the app uses premium ArcGIS Online services that consume credits, for example, the app user's account will be charged.
In this tutorial, you will build an app that implements user authentication using OAuth credentials so users can sign in and be authenticated through ArcGIS Online to access the ArcGIS World Traffic service.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Confirm that your system meets the minimum system requirements.
-
An IDE for Java.
Steps
Create OAuth credentials
OAuth credentials are required to implement user authentication. These credentials are created as an Application item in your organization's portal.
-
Sign in to your portal.
-
Click Content > My content > New item and select Developer credentials.
-
In the Create developer credentials window, select OAuth 2.0 credentials radio button and click Next.
-
Add a Redirect URL to your OAuth credentials:
my-app:
. The remaining properties, Referrer URLs, Application environment and URL, can remain with their default values. Click Next.//auth -
For Privileges, click Next. Privileges are not required for this tutorial.
-
Click Skip to move past Grant item access as it is not required for this tutorial.
-
Provide a Title of your choice. Optionally, stipulate a Folder to store your Application item, add Tags, and add a Summary. Click Next.
-
Review your settings and go back to correct any errors. When you are ready, click Create. When the application item is created,
Client ID
,Client Secret
, andTemporary Token
values will also be generated. You will be redirected to the Application item's Overview page.
Client ID
and Redirect URL
when implementing OAuth in your app's code. The Client ID
is found on the Application item's Overview page, while the Redirect URL
is found on the Settings page.The Client ID
uniquely identifies your app on the authenticating server. If the server cannot find an app with the provided Client ID, it will not proceed with authentication.
The Redirect URL
is used to identify a response from the authenticating server when the system returns control back to your app after an OAuth login. You can configure several redirect URLs in your application definition and can remove or edit them. It's important to make sure the redirect URL used in your app's code matches a redirect URL configured for the application.
Open a Java project with Gradle
-
To start this tutorial, complete the Display a map tutorial, or download and unzip the Display a map solution into a new folder.
-
Open the build.gradle file as a project in IntelliJ IDEA.
-
Delete the code that sets your API Key. Since your app will be using OAuth, you will not need an API Key.
App.javaUse dark colors for code blocks Remove line ArcGISRuntimeEnvironment.setApiKey("YOUR_ACCESS_TOKEN");
Prepare files before coding the app
Modify the files from the Display a map tutorial so they can be used in this tutorial: you will add imports, change the application title, and modify the view point's scale.
-
In IntelliJ IDEA, locate the Project tool window, open src/main/java/com.example.app, and double-click App. Add the following imports, replacing those from the
Display a map
tutorial.-
In the main menu of IntelliJ IDEA, click View > Tool Windows > Project. The Project Tool window displays, with a vertical tab on the left that says Project.
-
Inside the Project tool window, your view name should also be Project. If the view name is something else (such as Packages or Project Files), click on the leftmost control in the title bar of the Project tool window, and select Project from the drop-down list.
App.javaUse dark colors for code blocks package com.example.app; import com.esri.arcgisruntime.ArcGISRuntimeEnvironment; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.StackPane; import javafx.stage.Stage; import com.esri.arcgisruntime.layers.ArcGISMapImageLayer; import com.esri.arcgisruntime.mapping.ArcGISMap; import com.esri.arcgisruntime.mapping.BasemapStyle; import com.esri.arcgisruntime.mapping.Viewpoint; import com.esri.arcgisruntime.mapping.view.MapView; import com.esri.arcgisruntime.security.AuthenticationManager; import com.esri.arcgisruntime.security.DefaultAuthenticationChallengeHandler; import com.esri.arcgisruntime.security.OAuthConfiguration; public class App extends Application {
-
-
In the
start()
life cycle method, change the title that will appear on the application window toAccess services with OAuth 2.0
. In addition, add thetry
statement, which will be closed later on in the tutorial.App.javaUse dark colors for code blocks @Override public void start(Stage stage) { try { // set title, size, and add scene to stage stage.setTitle("Access services with OAuth 2.0"); stage.setWidth(800); stage.setHeight(700); stage.show();
-
Change the scale of the map's initial viewpoint to 72000. This scale will make the secured layer visible without zooming in.
App.javaUse dark colors for code blocks ArcGISMap map = new ArcGISMap(BasemapStyle.ARCGIS_TOPOGRAPHIC); // set the map on the map view mapView.setMap(map); mapView.setViewpoint(new Viewpoint(34.02700, -118.80543, 72000.0 ));
Implement user authentication using OAuth 2.0
This API abstracts some of the details for user authentication using OAuth credentials in your app. You can use classes such as AuthenticationManager
to request, store, and manage credentials for secure resources.
Add code to set up the AuthenticationManager
, which launches a small browser window titled Authentication Required. The user must enter log-in credentials before proceeding.
-
In the
start()
method, after the line that callsmap
, create anView.set Viewpoint() OAuthConfiguration
.App.javaUse dark colors for code blocks mapView.setViewpoint(new Viewpoint(34.02700, -118.80543, 72000.0 )); // set up an OAuth config with URL to portal, a Client ID and a Redirect URL OAuthConfiguration oAuthConfiguration = new OAuthConfiguration("YOUR-ORGANIZATION-URL", "YOUR-APP-CLIENT-ID", "YOUR-APP-REDIRECT-URL");
The
OAuth
constructor takes three parameters:Configuration -
"YOUR-ORGANIZATION-URL"
: the URL for your organization's portal associated with the ArcGIS Location Platform or ArcGIS Online account you signed in with during Create OAuth credentials above. -
"YOUR-APP-CLIENT-ID"
: theClient ID
from your Application item's Overview tab in your portal. -
"YOUR-APP-REDIRECT-URL"
: theRedirect URL
from your Application item's Settings tab in your portal.
-
-
Create a
Default
and set it on theAuthentication Challenge Handler AuthenticationManager
. Then add theo
to theAuth Configuration Authentication
.Manager App.javaUse dark colors for code blocks mapView.setViewpoint(new Viewpoint(34.02700, -118.80543, 72000.0 )); // set up an OAuth config with URL to portal, a Client ID and a Redirect URL OAuthConfiguration oAuthConfiguration = new OAuthConfiguration("YOUR-ORGANIZATION-URL", "YOUR-APP-CLIENT-ID", "YOUR-APP-REDIRECT-URL"); // set up the authentication manager to handle authentication challenges DefaultAuthenticationChallengeHandler defaultAuthenticationChallengeHandler = new DefaultAuthenticationChallengeHandler(); AuthenticationManager.setAuthenticationChallengeHandler(defaultAuthenticationChallengeHandler); // add the OAuth configuration AuthenticationManager.addOAuthConfiguration(oAuthConfiguration);
-
Create a file named
style.css
to specify the visual display of the Authentication Required browser window.-
In the Project tool window, open src > main and right-click the main folder. In the context menu, click New > Directory and click resources under Gradle Source Sets. Hit Enter to create the folder.
-
In the Project tool window, right-click the resources folder. In the context menu, click New > File and name the file style.css.
-
Copy the code displayed below and paste it into the
style.css
file.style.cssUse dark colors for code blocks .panel-region .label { -fx-text-fill: white; } .label { -fx-text-fill: black; } .slider .axis { -fx-tick-label-fill: white; } .range-slider .axis { -fx-tick-label-fill: white; } .panel-region .check-box { -fx-text-fill: white; } .panel-region .radio-button { -fx-text-fill: white; } .color-picker .color-picker-label { -fx-text-fill: black; }
-
Add a traffic layer
You will add a layer to display the ArcGIS World Traffic service, a dynamic map service that presents historical and near real-time traffic information for different regions in the world. This is a secure service and requires an ArcGIS Online organizational subscription.
ArcGIS World Traffic service data is updated every five minutes to provide traffic speed and traffic incident visualization and identification.
Traffic speeds are displayed as a percentage of free-flow speeds, which is frequently the speed limit or how fast cars tend to travel when unencumbered by other vehicles. The streets are color coded as follows:
- Green (fast): 85 - 100% of free flow speeds
- Yellow (moderate): 65 - 85%
- Orange (slow); 45 - 65%
- Red (stop and go): 0 - 45%
-
Create an
ArcGISMapImageLayer
to display the traffic service. Then add the layer to the map's collection of data layers (operational layers). In addition, you'll add thecatch
statement that corresponds to thetry
statement added earlier in the tutorial.App.javaUse dark colors for code blocks // set up an OAuth config with URL to portal, a Client ID and a Redirect URL OAuthConfiguration oAuthConfiguration = new OAuthConfiguration("YOUR-ORGANIZATION-URL", "YOUR-APP-CLIENT-ID", "YOUR-APP-REDIRECT-URL"); // set up the authentication manager to handle authentication challenges DefaultAuthenticationChallengeHandler defaultAuthenticationChallengeHandler = new DefaultAuthenticationChallengeHandler(); AuthenticationManager.setAuthenticationChallengeHandler(defaultAuthenticationChallengeHandler); // add the OAuth configuration AuthenticationManager.addOAuthConfiguration(oAuthConfiguration); ArcGISMapImageLayer trafficLayer = new ArcGISMapImageLayer("https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"); map.getOperationalLayers().add(trafficLayer); } catch (Exception e) { // on any error, display the stack trace. e.printStackTrace(); } }
-
Run the app. Ensure to run the app as a Gradle task and not as an application in your IDE. In the Gradle tool window, under Tasks > application, double-click run.
You should initially see the map with the topographic basemap layer centered on the Santa Monica Mountains in California. You will then be prompted for an ArcGIS Online username and password. After you authenticate successfully with ArcGIS Online, the traffic layer will appear in the map.
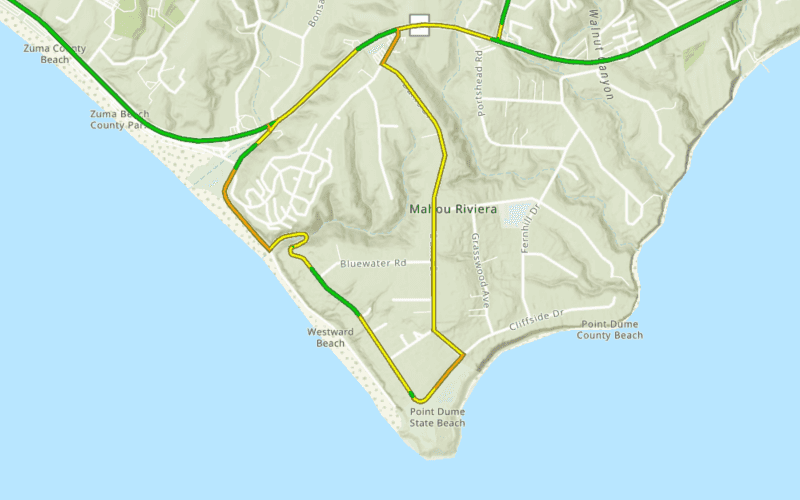