Learn how to use a URL to access and display a feature layer in a map.
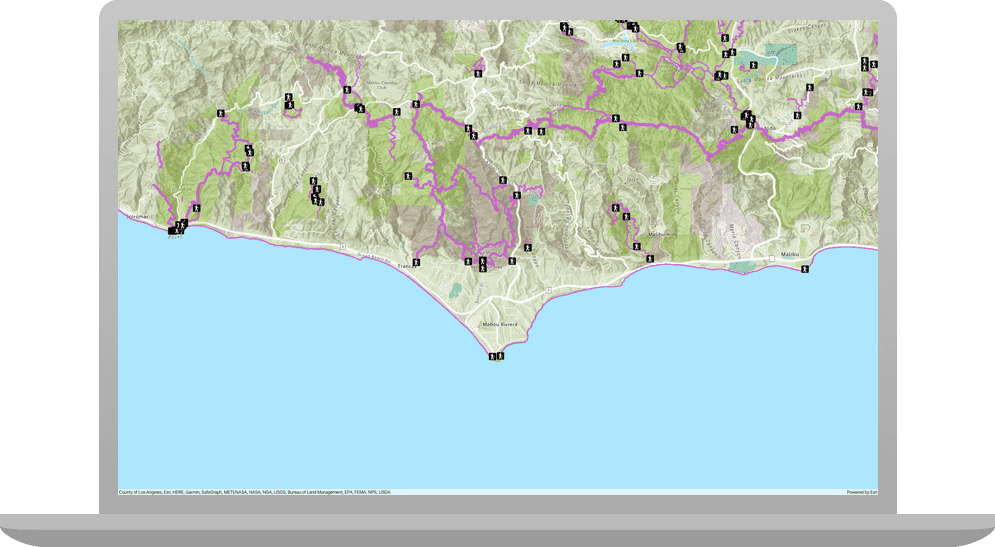
A feature layer is a dataset in a feature service hosted in ArcGIS. Each feature layer contains features with a single geometry type (point, line, or polygon), and a set of attributes. You can use feature layers to store, access, and manage large amounts of geographic data for your applications. You can access features from a feature layer using its underlying feature table.
In this tutorial, you use URLs to access and display three different feature layers hosted in ArcGIS Online:
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Confirm that your system meets the minimum system requirements.
-
An IDE for Java.
Steps
Open a Java project with Gradle
-
To start this tutorial, complete the Display a map tutorial, or download and unzip the Display a map solution into a new folder.
-
Open the build.gradle file as a project in IntelliJ IDEA.
-
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In IntelliJ IDEA's Project tool window, open src/main/java/com.example.app and double-click App.
-
In the
start()
method, set the API key property on theArcGISRuntimeEnvironment
with your access token. Replace YOUR_ACCESS_TOKEN with your copied access token. Be sure to surround your access token with double quotes as it is a string.App.javaUse dark colors for code blocks ArcGISRuntimeEnvironment.setApiKey("YOUR_ACCESS_TOKEN");
-
Add import statements
Add import statements to reference the API classes.
-
In the IntelliJ IDEA's Project tool window, open src/main/java/com.example.app and double-click App.
-
Add the following imports above the existing imports.
App.javaUse dark colors for code blocks Add line. Add line. import com.esri.arcgisruntime.data.ServiceFeatureTable; import com.esri.arcgisruntime.layers.FeatureLayer; import com.esri.arcgisruntime.ArcGISRuntimeEnvironment; import com.esri.arcgisruntime.mapping.ArcGISMap; import com.esri.arcgisruntime.mapping.BasemapStyle; import com.esri.arcgisruntime.mapping.Viewpoint; import com.esri.arcgisruntime.mapping.view.MapView;
-
In the
start()
life-cycle method, change the title that will appear on the application window toAdd a feature layer
.App.javaUse dark colors for code blocks @Override public void start(Stage stage) { // set the title and size of the stage and show it stage.setTitle("Add a feature layer tutorial"); stage.setWidth(800); stage.setHeight(700); stage.show();
Create service feature tables to reference feature service data
To display three new data layers (also known as operational layers) on top of the current basemap, you will create ServiceFeatureTable
objects using URLs to reference datasets hosted in ArcGIS Online.
-
Open a browser and navigate to the URL for Parks and Open Spaces to view metadata about the layer. To display the layer in your app, you only need the URL.
The service page provides information such as the geometry type, the geographic extent, the minimum and maximum scale at which features are visible, and the attributes (fields) it contains. You can preview the layer by clicking on Map Viewer in the "View In:" list at the top of the page.
-
In the
start()
method, create threeServiceFeatureTable
objects, using a string URL in each to reference the datasets. You will add: Trailheads (points), Trails (lines), and Parks and Open Spaces (polygons).A
ServiceFeatureTable
is effectively an in-memory database of the features from the service.App.javaUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. // set the map on the map view mapView.setMap(map); mapView.setViewpoint(new Viewpoint(34.02700, -118.80543, 144447.638572)); String parksUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0"; String trailsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0"; String trailHeadsUrl = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0"; ServiceFeatureTable parksServiceFeatureTable = new ServiceFeatureTable(parksUrl); ServiceFeatureTable trailsServiceFeatureTable = new ServiceFeatureTable(trailsUrl); ServiceFeatureTable trailHeadsServiceFeatureTable = new ServiceFeatureTable(trailHeadsUrl);
Create feature layers to display the hosted data
You will create three new FeatureLayer
objects to display the hosted layers above the basemap.
-
Create three new
FeatureLayer
objects using the service feature tables and add them to the map as data (operational) layers.Data layers are displayed in the order in which they are added. Polygon layers should be added before layers with lines or points if there's a chance the polygon symbols will obscure features beneath.
A
FeatureLayer
is simply a reference to a feature service and a fast and easy way to add data to a map. It is accessed via a URL which specifies the endpoint. By default, the API will try to load all of the features that fit into the current view.App.javaUse dark colors for code blocks Add line. Add line. Add line. ServiceFeatureTable parksServiceFeatureTable = new ServiceFeatureTable(parksUrl); ServiceFeatureTable trailsServiceFeatureTable = new ServiceFeatureTable(trailsUrl); ServiceFeatureTable trailHeadsServiceFeatureTable = new ServiceFeatureTable(trailHeadsUrl); map.getOperationalLayers().add(new FeatureLayer(parksServiceFeatureTable)); map.getOperationalLayers().add(new FeatureLayer(trailsServiceFeatureTable)); map.getOperationalLayers().add(new FeatureLayer(trailHeadsServiceFeatureTable));
-
Run the app. Ensure to run the app as a Gradle task and not as an application in your IDE. In the Gradle tool window, under Tasks > application, double-click run.
You should see point, line, and polygon features (representing trailheads, trails, and parks) draw on the map for an area in the Santa Monica Mountains in southern California.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: