This sample demonstrates how to generate a default popupTemplate and labels for point clusters enabled in a MapView. To generate a popupTemplate, call the getTemplates() method in the esri/smartMapping/popup/clusters module. Use the getLabelSchemes() method in esri/smartMapping/labels/clusters to generate suggested default labeling
for the clusters along with a suggested cluster
.
async function generateClusterConfig(layer) {
// generates default popupTemplate
const popupTemplate = await clusterPopupCreator
.getTemplates({ layer })
.then((popupTemplateResponse) => popupTemplateResponse.primaryTemplate.value);
// generates default labelingInfo
const { labelingInfo, clusterMinSize } = await clusterLabelCreator
.getLabelSchemes({ layer, view })
.then((labelSchemes) => labelSchemes.primaryScheme);
return {
type: "cluster",
popupTemplate,
labelingInfo,
clusterMinSize,
maxScale: 50000
};
}
Clustering is enabled via the featureReduction property of the FeatureLayer.
layer
.when()
.then(generateClusterConfig)
.then((featureReduction) => {
layer.featureReduction = featureReduction;
Since a layer's featureReduction property is independent of its renderer, the symbol and popup
of the cluster graphics can be used to summarize features comprising the cluster. See Clustering styles and configurations for more information about the various ways clusters can summarize the points they represent.
The layer in this sample visualizes places of worship with a UniqueValueRenderer. When clustering is enabled, each cluster is assigned the symbol of the most common uniqueValueInfo among the features in the cluster.
Display all points | Display clustered features |
---|---|
![]() |
![]() |
You can reference the predominant value of a cluster in the popup
. For layers with a Unique
, the summary field name follows this format: {cluster_
. In this case, the renderer visualizes unique values in the religion
field, so the aggregate field name to reference in the popup
is {cluster_
.
This sample also demonstrates how you can explore and filter a layer by category with clustering enabled the same way you would on a non-clustered layer. When a filter is applied to the layer view of a clustered layer, the clusters will recompute client-side and only display information complying with the filter.
filterSelect.addEventListener("change", (event) => {
const newValue = event.target.value;
const whereClause = newValue ? "religion = '" + newValue + "'" : null;
layerView.filter = {
where: whereClause
};
// close popup for former cluster that no longer displays
view.closePopup();
});
Related samples and resources
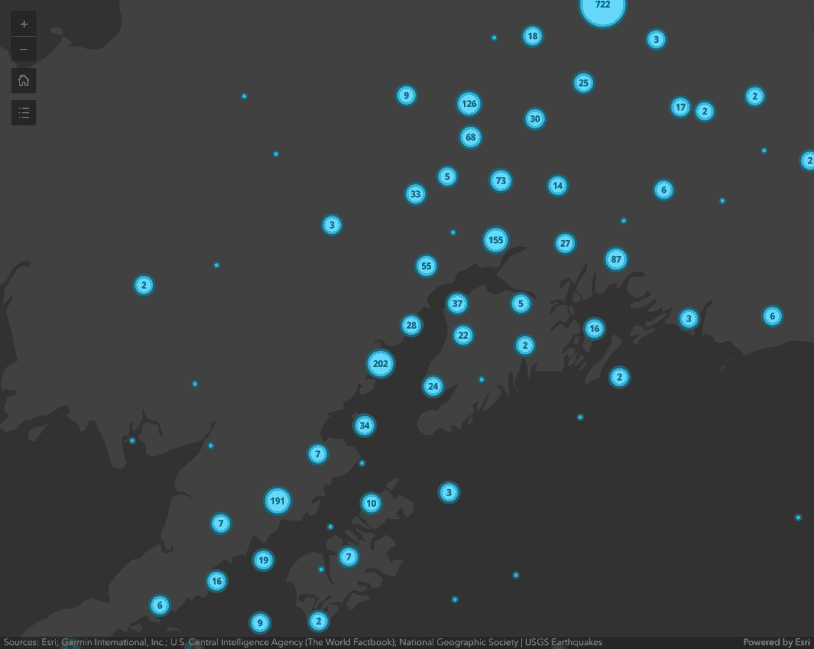
Intro to clustering
Intro to clustering
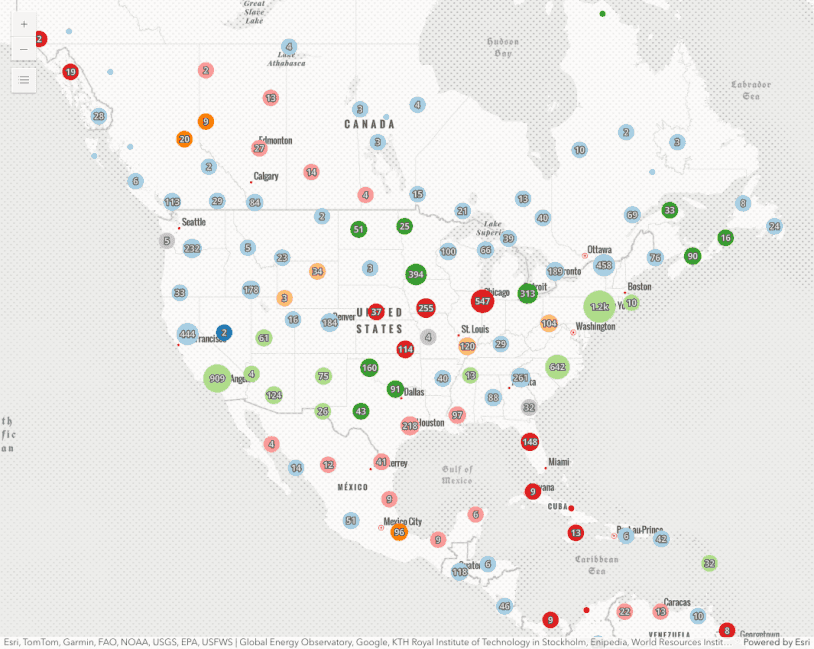
Clustering - filter popup features
This sample demonstrates how to filter clustered features within a cluster's popup.
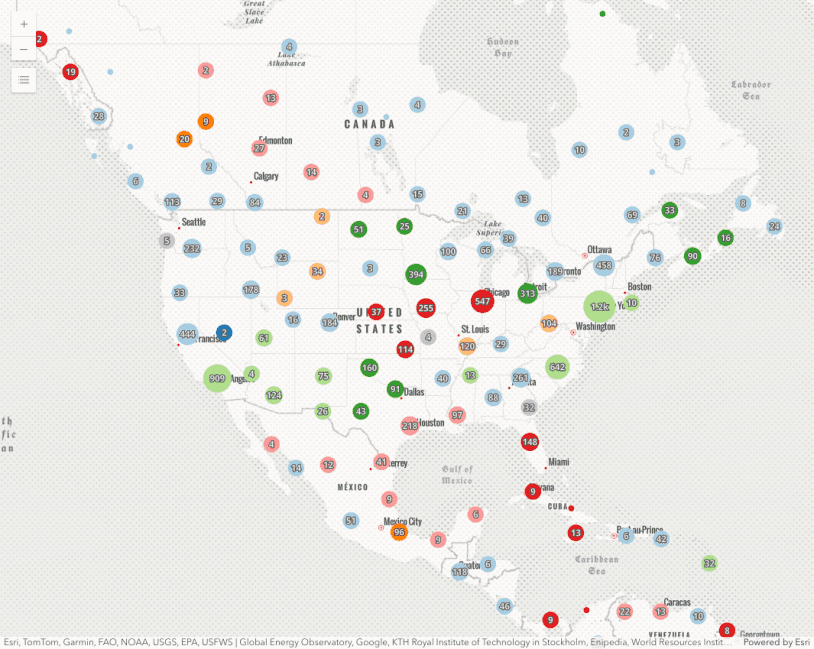
Clustering - query clusters
Clustering - query clusters
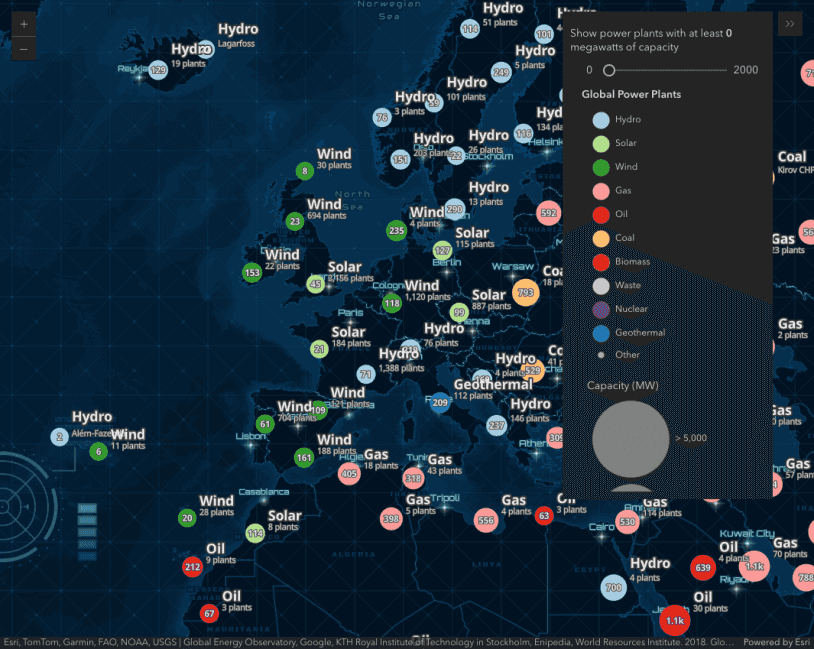
Clustering - advanced configuration
Clustering - advanced configuration
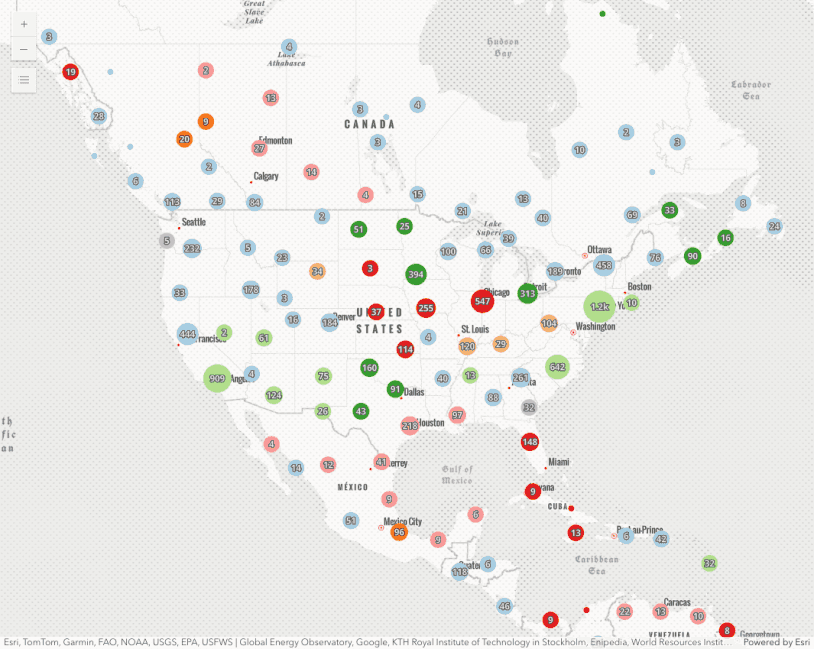
Popup charts for point clusters
This sample demonstrates how to summarize clustered features using charts within a cluster's popup.
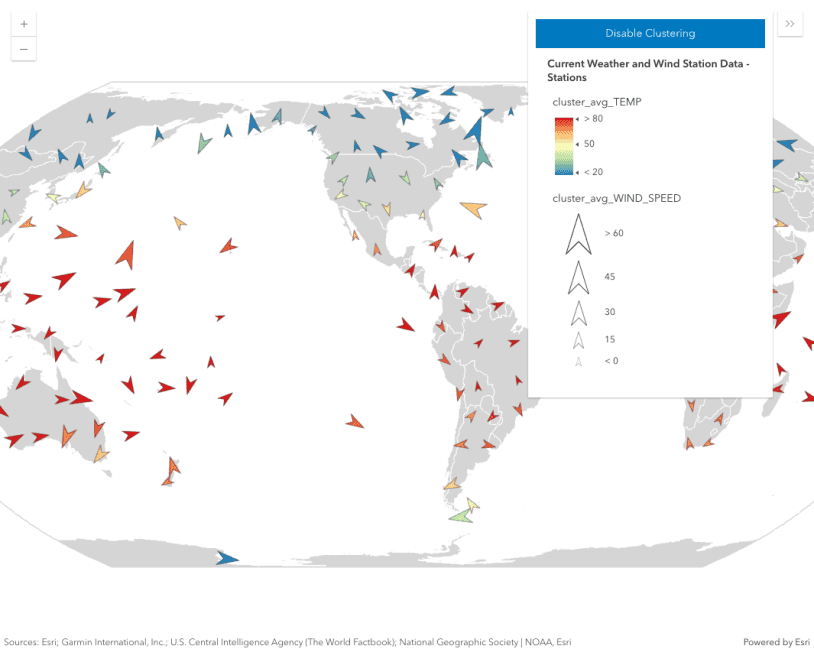
Point clustering with visual variables
Point clustering with visual variables
FeatureReductionCluster
Read the API Reference for more information.