What is a unique types style?
A unique types style assigns distinct symbols to unique data values returned from a field or expression in a layer. You can use this style to visualize what something represents, such as:
- Show points of interest on a map (e.g. schools, parks, places of worship)
- Represent categories of data (e.g. regions, types)
- Visualize ordinal data (e.g. high, medium, and low)
How a unique types style works
This style is configured with a Unique Value Renderer. This renderer requires the following:
- A reference to a data value either from a field name, or an Arcade expression.
- A list of unique value infos that match a unique symbol with an expected value returned from the field or expression.
Examples
Categorical data
This example demonstrates how to visualize categories using a string attribute value. The app visualizes freeways based on their classification: interstate, state highway, or U.S. highway.
- Create a Unique Value Renderer.
- Reference the field name containing the classification values.
- Create unique value info objects and assign a symbol to each expected value.
- You can optionally add a default symbol to represent all features that don't have a matching value.
const hwyRenderer = {
type: "unique-value",
legendOptions: {
title: "Freeway type"
},
field: "RTTYP",
uniqueValueInfos: [{
value: "S",
label: "State highway",
symbol: {
type: "simple-line",
color: "#e6d800",
width: "6px",
style: "solid"
}
}, {
value: "I",
label: "Interstate",
symbol: {
type: "simple-line",
color: "#e60049",
width: "6px",
style: "solid"
}
}, {
value: "U",
label: "US Highway",
symbol: {
type: "simple-line",
color: "#9b19f5",
width: "6px",
style: "solid"
}
}]
};
Points of interest (3D)
This example demonstrates how to visualize points of interest (POI) in a 3D scene. This demo shows how to create POI symbols with a PictureMarkerSymbol. You can also use web styles to create the same visualization.
- Create a unique value renderer.
- Reference the field name containing the location type (e.g.
museum
,park
, etc.). - Create unique value info objects and assign a symbol to each expected value.
// Expand the code above to view how each marker
// symbol is created in getUniqueValueSymbol()
const pointsRenderer = {
type: "unique-value",
field: "Type",
uniqueValueInfos: [
{
value: "Museum",
symbol: getUniqueValueSymbol("https://developers.arcgis.com/javascript/latest/sample-code/visualization-point-styles/live/Museum.png", "#D13470")
},
{
value: "Restaurant",
symbol: getUniqueValueSymbol("https://developers.arcgis.com/javascript/latest/sample-code/visualization-point-styles/live/Restaurant.png", "#F97C5A")
},
{
value: "Church",
symbol: getUniqueValueSymbol("https://developers.arcgis.com/javascript/latest/sample-code/visualization-point-styles/live/Church.png", "#884614")
},
{
value: "Hotel",
symbol: getUniqueValueSymbol("https://developers.arcgis.com/javascript/latest/sample-code/visualization-point-styles/live/Hotel.png", "#56B2D6")
},
{
value: "Park",
symbol: getUniqueValueSymbol("https://developers.arcgis.com/javascript/latest/sample-code/visualization-point-styles/live/Park.png", "#40C2B4")
}
]
};
Ordinal data
This example demonstrates how to create ordinal categories from a numeric field attribute using an Arcade expression. This app classifies highways by low, medium, or high truck traffic.
- Create a unique value renderer.
- Write an Arcade expression to classify the data into ordinal categories: low, medium, and high. Then reference it in the
value
property. See the snippet below.Expression - Create unique value info objects and assign a symbol to each expected value.
- You can optionally add a default symbol to represent all features that don't have a matching value.
var traffic = $feature.AADT;
When(
traffic > 80000, "High",
traffic > 20000, "Medium",
"Low"
);
const renderer = {
type: "unique-value",
valueExpression: `
var traffic = $feature.AADT;
When(
traffic > 80000, "High",
traffic > 20000, "Medium",
"Low"
);
`,
valueExpressionTitle: "Traffic volume",
uniqueValueInfos: [{
value: "High",
symbol: {
type: "simple-line",
color: "#810f7c",
width: "6px",
style: "solid"
}
}, {
value: "Medium",
symbol: {
type: "simple-line",
color: "#8c96c6",
width: "3px",
style: "solid"
}
}, {
value: "Low",
symbol: {
type: "simple-line",
color: "#9d978b",
width: "1px",
style: "solid"
}
}]
};
Categorical data (3D)
This example visualizes buildings based on building type: residential, commercial or mixed use. A unique value renderer assigns a color to each building based on the building's usage attribute.
Steps
- Create different symbols for each building type.
- Assign the symbols to a unique value renderer.
- Assign the renderer to the scene layer.
const typeRenderer = {
type: "unique-value",
legendOptions: {
title: "Building Type"
},
defaultSymbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#FFB55A", colorMixMode: "replace" }
}
]
},
defaultLabel: "Others",
field: "landuse",
uniqueValueInfos: [
{
value: "MIPS",
symbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#FD7F6F", colorMixMode: "replace" }
}
]
},
label: "Office"
},
{
value: "RESIDENT",
symbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#7EB0D5", colorMixMode: "replace" }
}
]
},
label: "Residential"
},
{
value: "MIXRES",
symbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#BD7EBE", colorMixMode: "replace" }
}
]
},
label: "Mixed use"
},
{
value: "MIXED",
symbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#B2E061", colorMixMode: "replace" }
}
]
},
label: "Mixed use without residential"
}
]
};
Related samples and resources
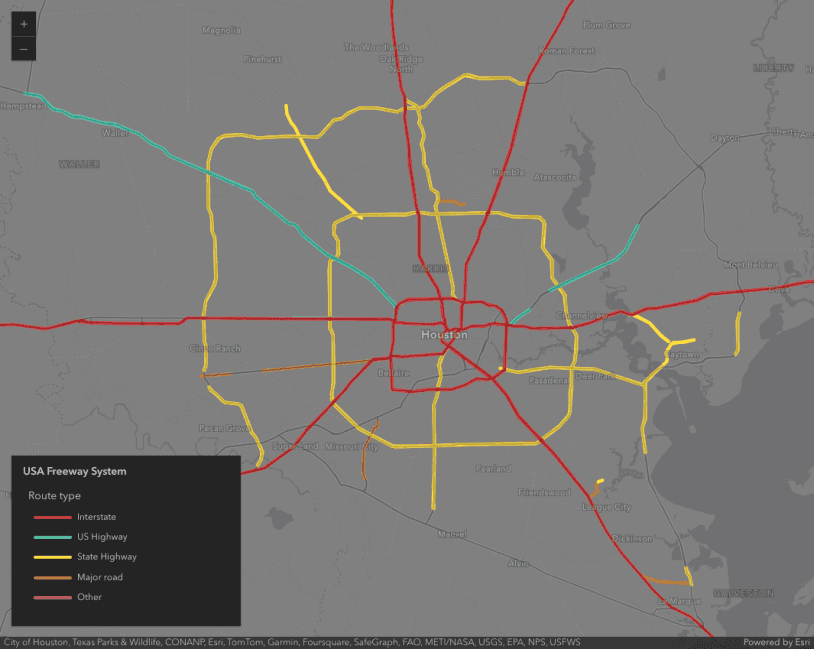
Visualize features by type
Visualize features by type
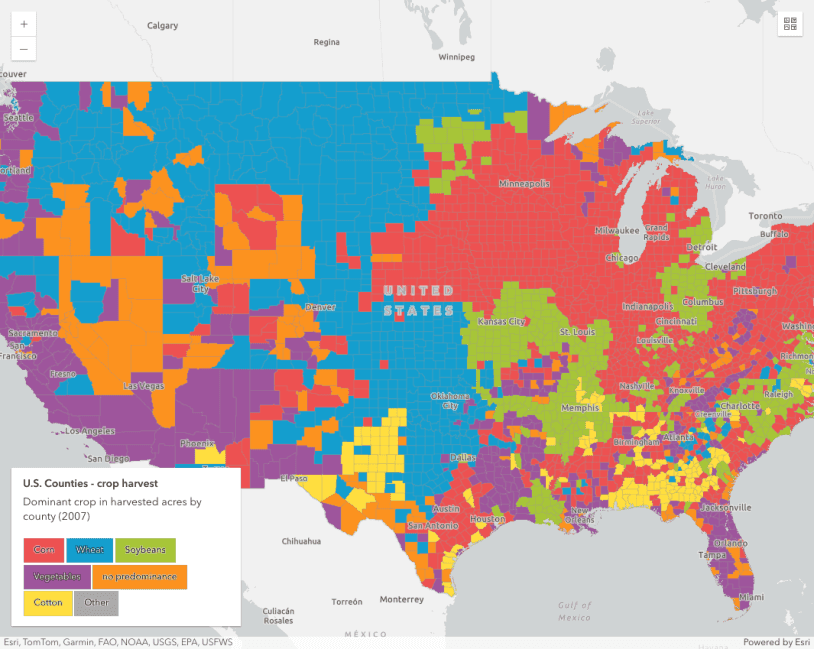
Generate data-driven visualization of unique values
Generate data-driven visualization of unique values
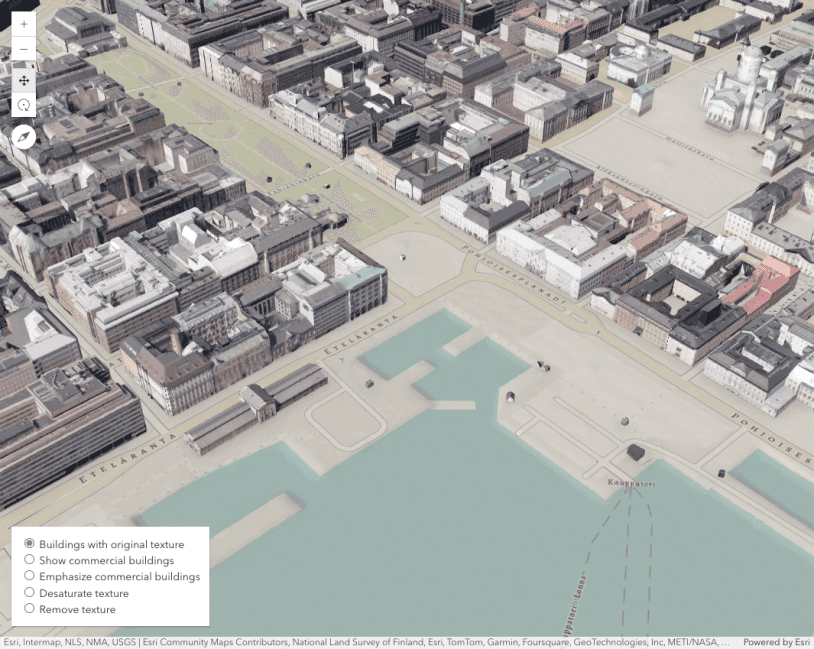
Coloring options for textured buildings
Coloring options for textured buildings
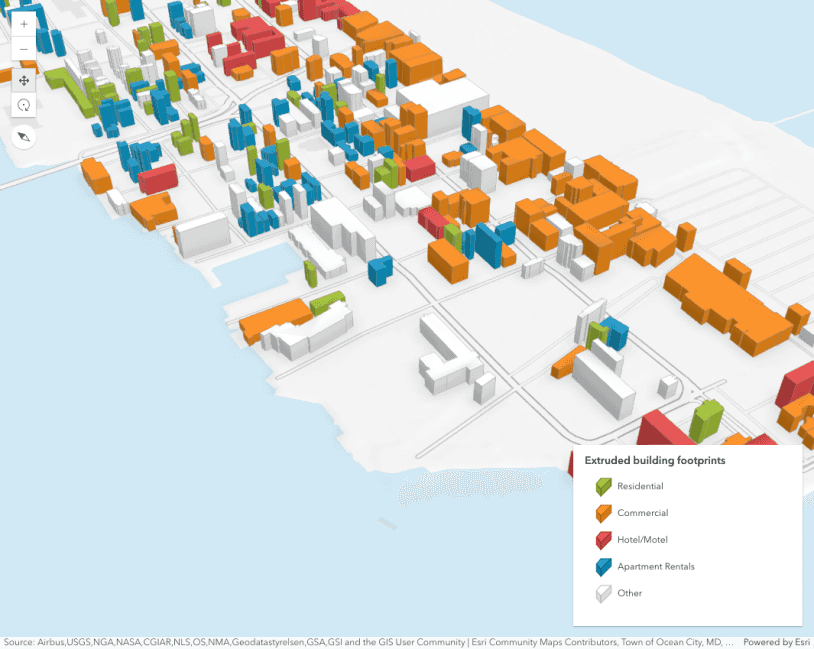
Extrude building footprints based on real world heights
Extrude building footprints based on real world heights
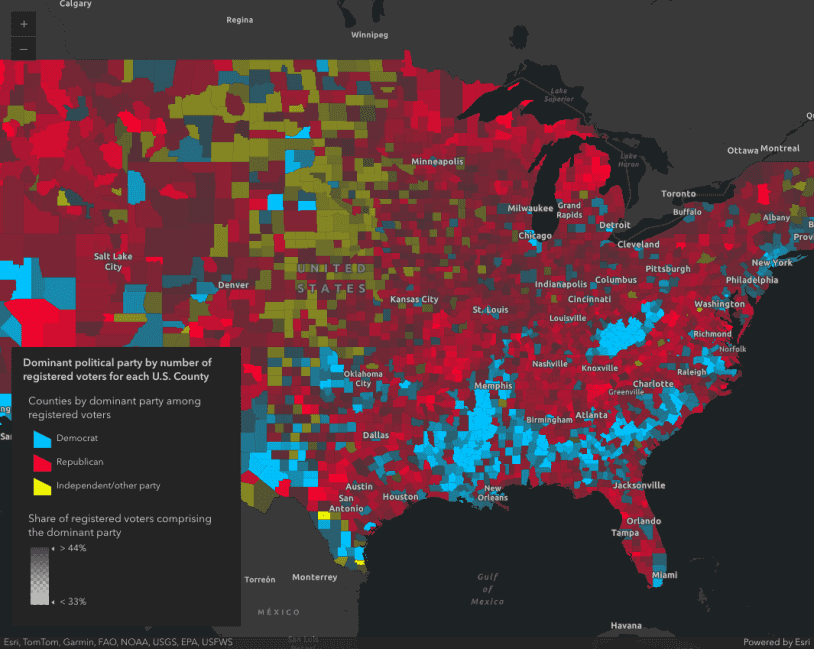
Create a custom visualization using Arcade
Create a custom visualization using Arcade
API support
2D | 3D | Arcade | Points | Lines | Polygons | Mesh | |
---|---|---|---|---|---|---|---|
Unique types | |||||||
Class breaks | |||||||
Visual variables | 1 | ||||||
Time | |||||||
Multivariate | |||||||
Predominance | |||||||
Dot density | |||||||
Charts | |||||||
Relationship | |||||||
Smart Mapping | 2 | 3 | 3 | 3 |
- 1. Color only
- 2. Size variable creators only supported for points
- 3. Size variable creators not supported in 3D