Learn how to list API key credentials by expiration date using the ArcGIS API for Python.
API key credentials are used to authenticate requests to ArcGIS Location Platform, ArcGIS Online, and ArcGIS Enterprise. You can list API keys by expiration date to help you manage them.
This tutorial shows you how to list API keys in your portal by expiration date using the arcgis.gis
module in the ArcGIS API for Python.
Prerequisites
You need an ArcGIS Location Platform, ArcGIS Online or ArcGIS Enterprise account for this tutorial.
Steps
Set up your development environment
- Install the ArcGIS API for Python.
- Start your Jupyter Notebook environment locally.
List API keys by expiration date
-
Add the following
import
statements to your code.main.pyUse dark colors for code blocks from arcgis.gis import GIS from datetime import datetime, timezone
-
Establish a connection to the portal using the GIS() module.
main.pyUse dark colors for code blocks from arcgis.gis import GIS from datetime import datetime, timezone gis = GIS() ## Default portal: "https://arcgis.com/"
-
Call the
search
method using theitem
filter to return all API keys._type=" Application" main.pyUse dark colors for code blocks from arcgis.gis import GIS from datetime import datetime, timezone gis = GIS() ## Default portal: "https://arcgis.com/" items = gis.content.search(query="", item_type="Application", max_items=-1)
-
Use a
for
loop to sort the API keys by expiration date.main.pyUse dark colors for code blocks from arcgis.gis import GIS from datetime import datetime, timezone gis = GIS() ## Default portal: "https://arcgis.com/" items = gis.content.search(query="", item_type="Application", max_items=-1) key_list = [] for item in items: if "APIToken" in item.typeKeywords: app_item = gis.content.get(item.id) if app_item.apiToken1ExpirationDate != -1: ts = datetime.fromtimestamp(app_item.apiToken1ExpirationDate / 1000, timezone.utc).strftime('%Y-%m-%d %H:%M:%S') key_list.append((item.title, ts)) sorted_keys = sorted(key_list, key=lambda x: x[1])
-
Print the API keys sorted by expiration date.
main.pyUse dark colors for code blocks from arcgis.gis import GIS from datetime import datetime, timezone gis = GIS() ## Default portal: "https://arcgis.com/" items = gis.content.search(query="", item_type="Application", max_items=-1) key_list = [] for item in items: if "APIToken" in item.typeKeywords: app_item = gis.content.get(item.id) if app_item.apiToken1ExpirationDate != -1: ts = datetime.fromtimestamp(app_item.apiToken1ExpirationDate / 1000, timezone.utc).strftime('%Y-%m-%d %H:%M:%S') key_list.append((item.title, ts)) sorted_keys = sorted(key_list, key=lambda x: x[1]) for name, expiration in sorted_keys: print(f"Name: {name}, Expiration: {expiration}")
View the results
After running the code, you will see a list of API keys in your portal sorted by expiration date.
Name: All Location Services API Key (test), Expiration: 2024-09-25 08:38:08
Name: API Key (basemaps only), Expiration: 2024-09-25 08:44:56
Name: Anita_UC_Demo_Key, Expiration: 2024-10-01 19:16:15
Name: API key for map tile layer, Expiration: 2024-10-07 20:23:48
Name: test api key credentials, Expiration: 2024-10-30 20:16:35
Name: All privileges api key, Expiration: 2024-10-30 20:51:29
Name: roads codepen (test), Expiration: 2024-11-04 20:48:21
Name: try to invalidate this key, Expiration: 2024-11-07 18:05:45
Name: For codepen for blog, Expiration: 2024-11-27 23:11:25
Name: Demo Key, Expiration: 2024-12-26 18:12:54
Name: Al's API key for testing, Expiration: 2024-12-31 14:34:06
Name: Parcels key, Expiration: 2025-01-06 15:30:33
Name: Parcel_Key_Demo_Layer, Expiration: 2025-01-08 00:20:40
What's next
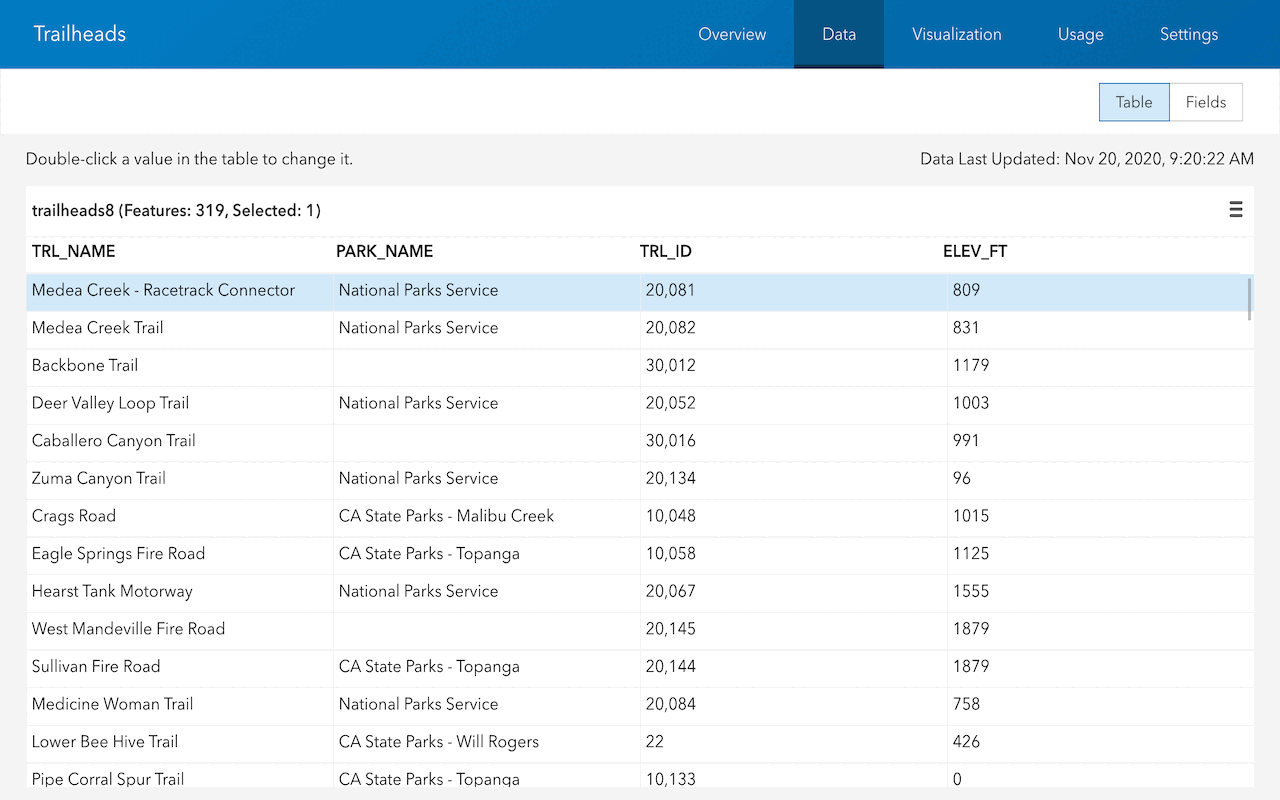
Copy a hosted layer item
Use the portal service to copy a hosted layer item in your portal.
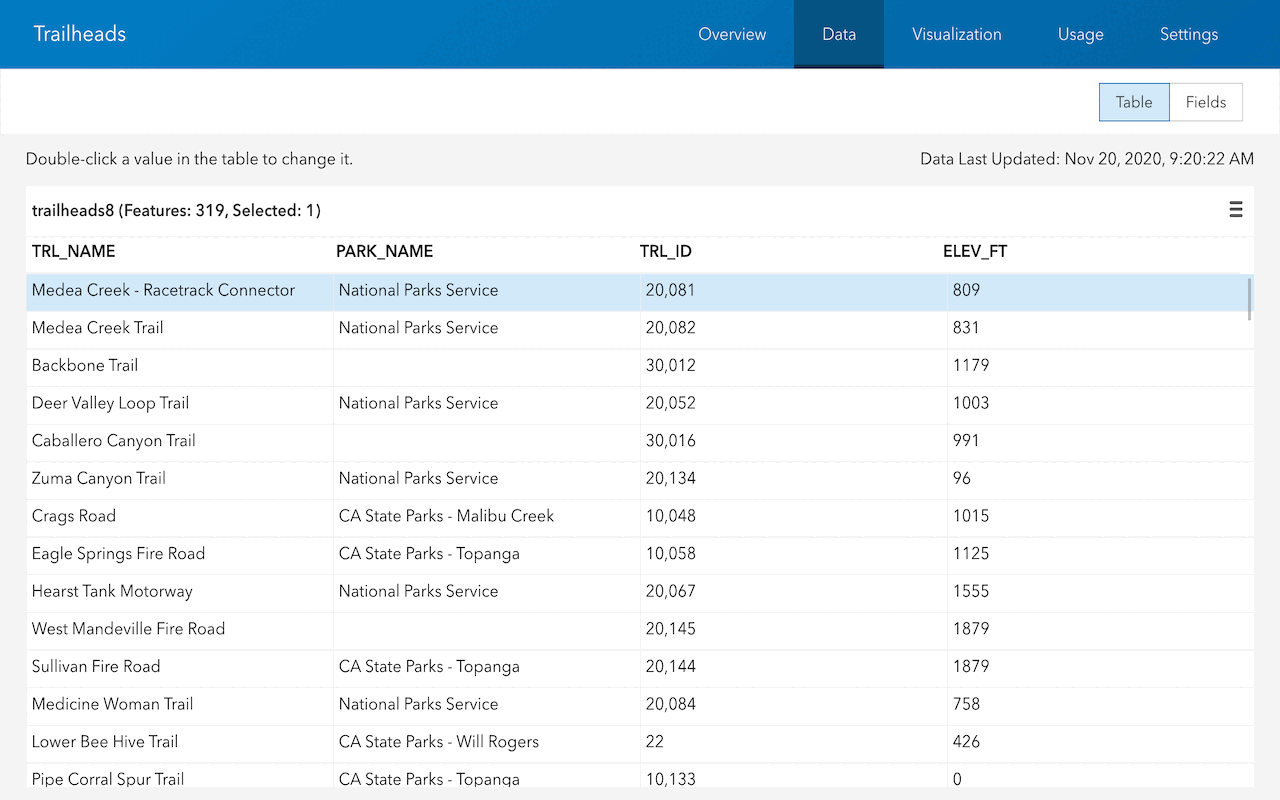
Set sharing level for an item
Use the portal service to set the sharing level for an item in your portal.
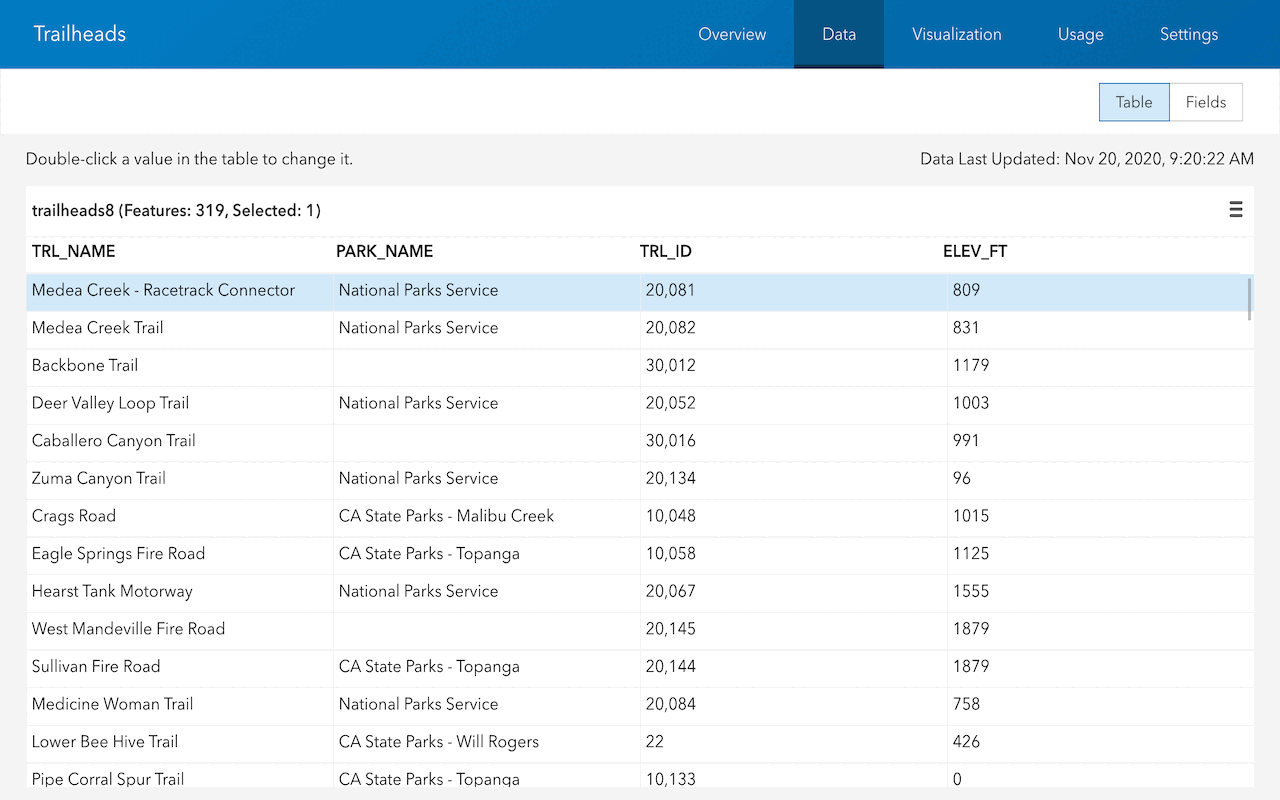
Create a new group and add members
Use the portal service to create a new group and add members in your portal.

List items scoped to an API key
Use ArcGIS API for Python to list items scoped to an API key in your portal.