Learn how to use the portal service to create a new group in your portal, and add members to the group.
Prerequisites
You need the following for this tutorial:
- Account: An ArcGIS Location Platform, ArcGIS Online, or ArcGIS Enterprise account.
- Authentication: A basic understanding of API key authentication or User authentication.
Steps
Get the portal URL
To access a portal, you need an ArcGIS account which is associated with an organization that allows you to store and manage your content.
- In a web browser, sign in to your portal with your ArcGIS account.
- Identify the portal URL from the navigation bar. The base URL should be one of the following:
- ArcGIS Location Platform:
https
://www.arcgis.com/sharing/rest - ArcGIS Online:
https
://www.arcgis.com/sharing/rest - ArcGIS Enterprise:
https
://{machine.domain.com}/{webadaptor}/rest
- ArcGIS Location Platform:
Implement authentication
You need an access token with the correct privileges for in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
-
Privileges
- Admin privileges > Content > View all
- Admin privileges > Groups
-
Create a new group and add members
-
Add the following import statement to your code.
main.pyUse dark colors for code blocks from arcgis.gis import GIS
-
Establish a connection to the portal using the
GI
module.S() main.pyUse dark colors for code blocks from arcgis.gis import GIS # Authenticate with your ArcGIS Online account gis = GIS("https://www.arcgis.com", "your_username", "your_password")
-
Create a dictionary of the new group and add it using the
create
method._from _dict main.pyUse dark colors for code blocks from arcgis.gis import GIS # Authenticate with your ArcGIS Online account gis = GIS("https://www.arcgis.com", "your_username", "your_password") # Create a group group_properties = { "title": "Example Group", "description": "This is an example group", "tags": "example, group, arcgis python", "snippet": "Example group", "access": "public" # Can be 'private', 'org', or 'public' } group = gis.groups.create_from_dict(group_properties) print(f"Group created: {group.title}")
-
Add existing members to the new group using the
add
method._users main.pyUse dark colors for code blocks from arcgis.gis import GIS # Authenticate with your ArcGIS Online account gis = GIS("https://www.arcgis.com", "your_username", "your_password") # Create a group group_properties = { "title": "Example Group", "description": "This is an example group", "tags": "example, group, arcgis python", "snippet": "Example group", "access": "public" # Can be 'private', 'org', or 'public' } group = gis.groups.create_from_dict(group_properties) print(f"Group created: {group.title}") # Add an existing member to the group usernames_to_add = ["member_username1", "member_username2"] result = group.add_users(usernames_to_add)
-
Verify members were added to the group.
main.pyUse dark colors for code blocks from arcgis.gis import GIS # Authenticate with your ArcGIS Online account gis = GIS("https://www.arcgis.com", "your_username", "your_password") # Create a group group_properties = { "title": "Example Group", "description": "This is an example group", "tags": "example, group, arcgis python", "snippet": "Example group", "access": "public" # Can be 'private', 'org', or 'public' } group = gis.groups.create_from_dict(group_properties) print(f"Group created: {group.title}") # Add an existing member to the group usernames_to_add = ["member_username1", "member_username2"] result = group.add_users(usernames_to_add) if 'notAdded' in result and result['notAdded']: print(f"Failed to add user(s): {result['notAdded']}") else: print(f"Users added successfully to the group {group.title}")
View the results
If successful, your code will print an output like this to verify that the operation was successful:
Group created: Example Group
Users added successfully to the group Example Group
What's next
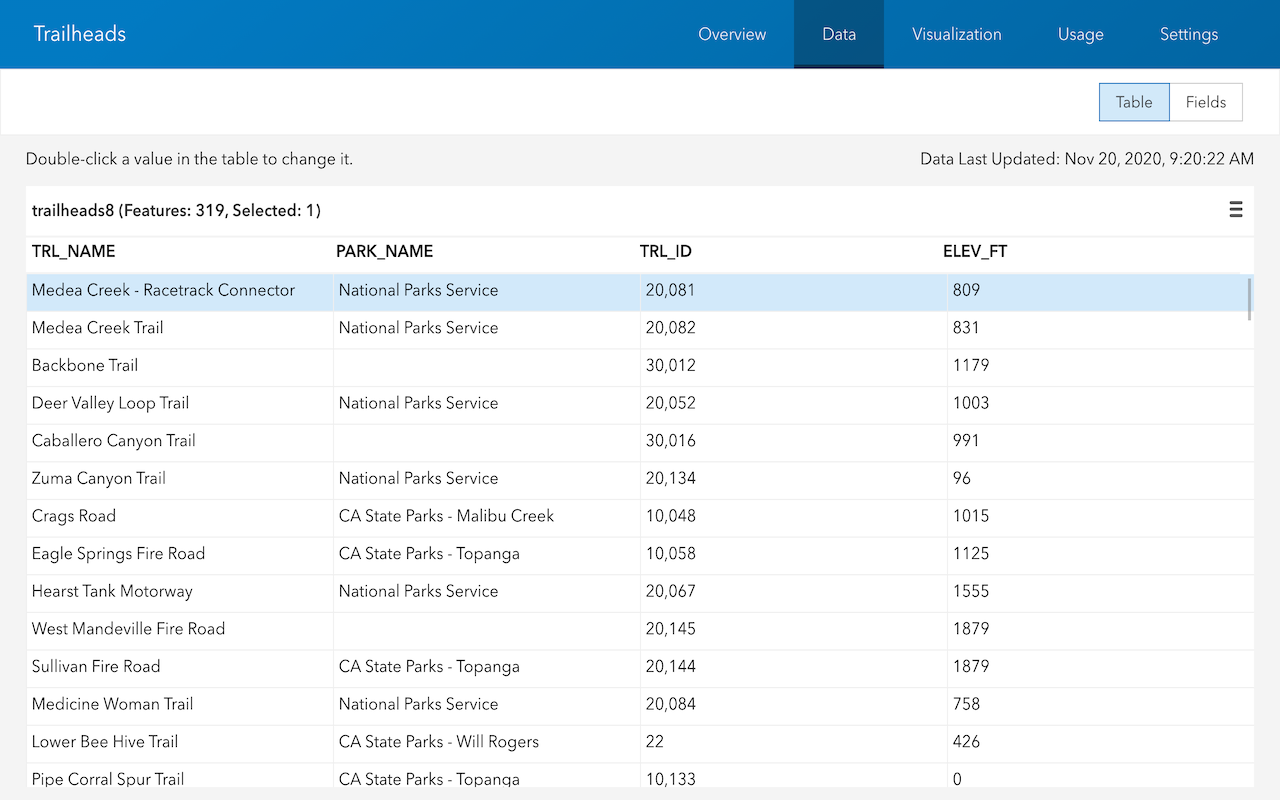
Sign in and access your portal
Use the portal service to sign in and access your portal.
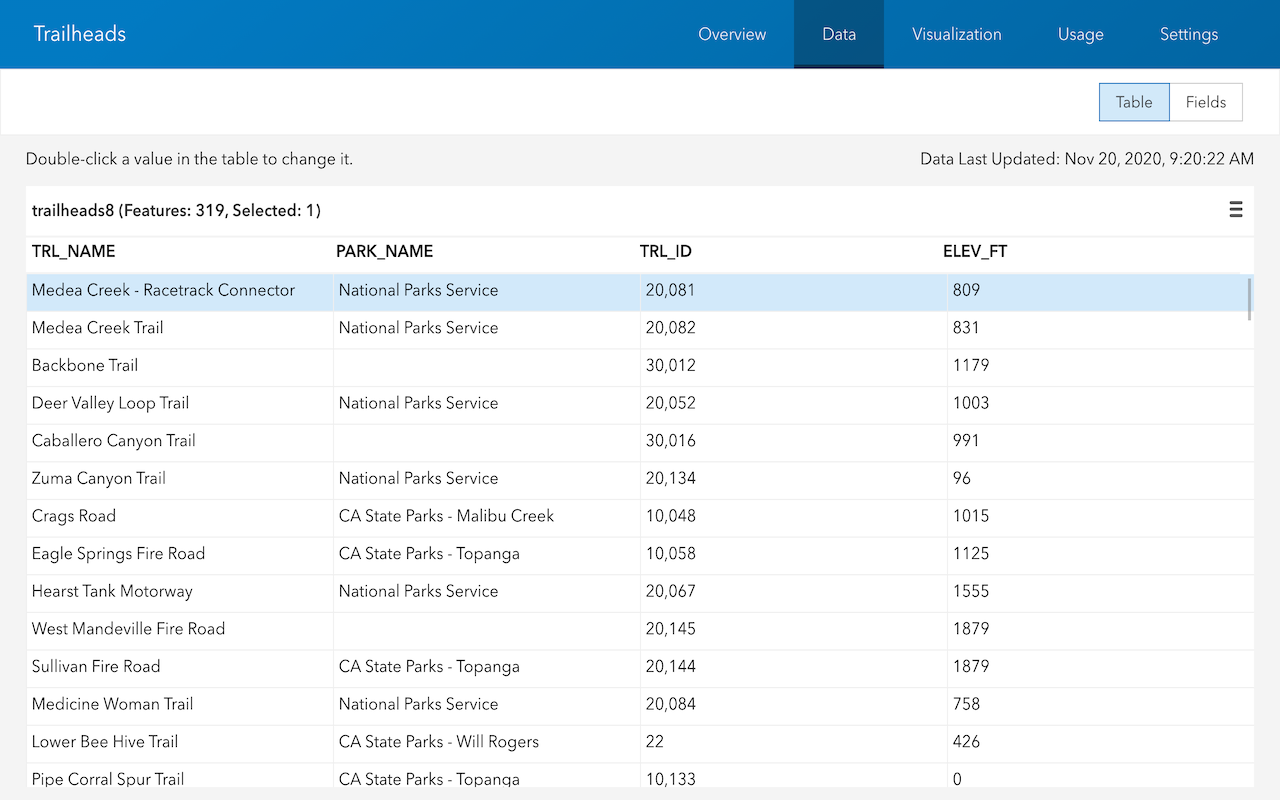
List items in your portal
Use the portal service to list items in your portal.
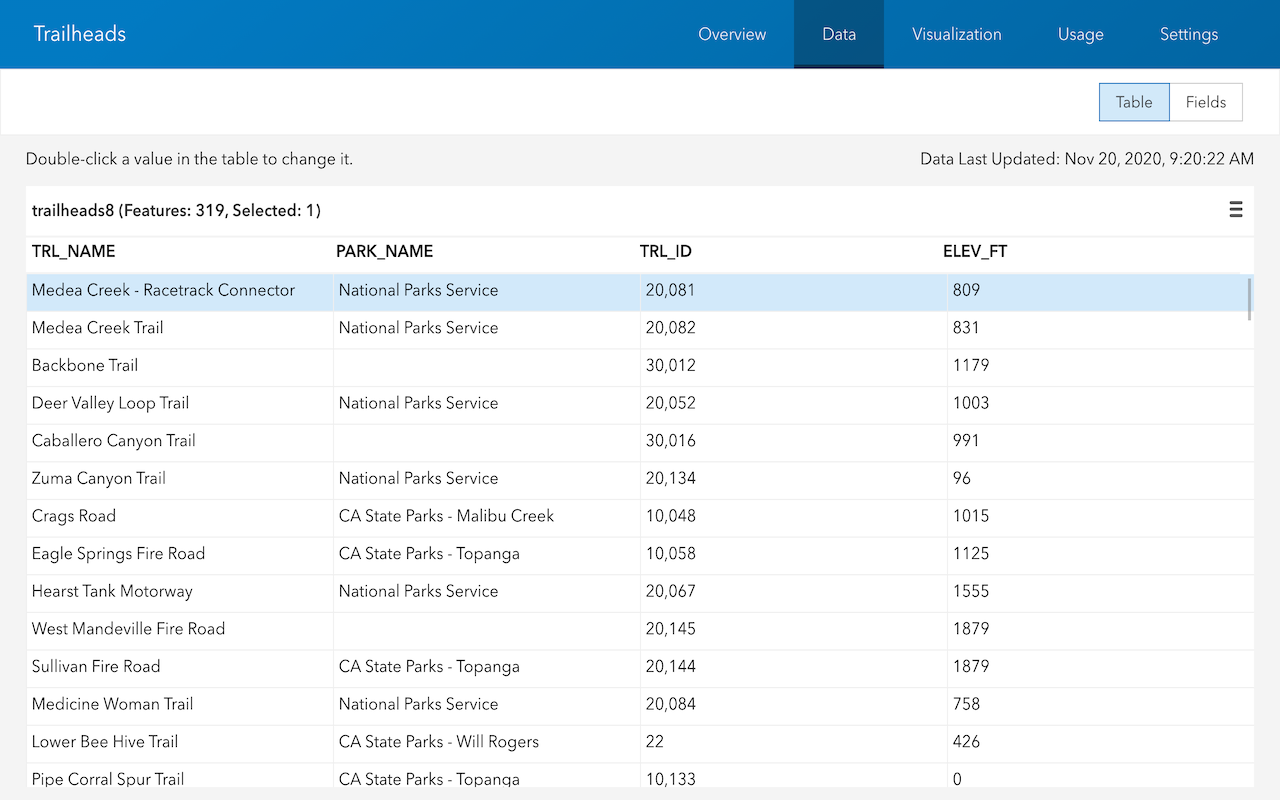
Set sharing level for an item
Use the portal service to set the sharing level for an item in your portal.