Learn how to search for coffee shops, gas stations, restaurants and other nearby places with the geocoding service.
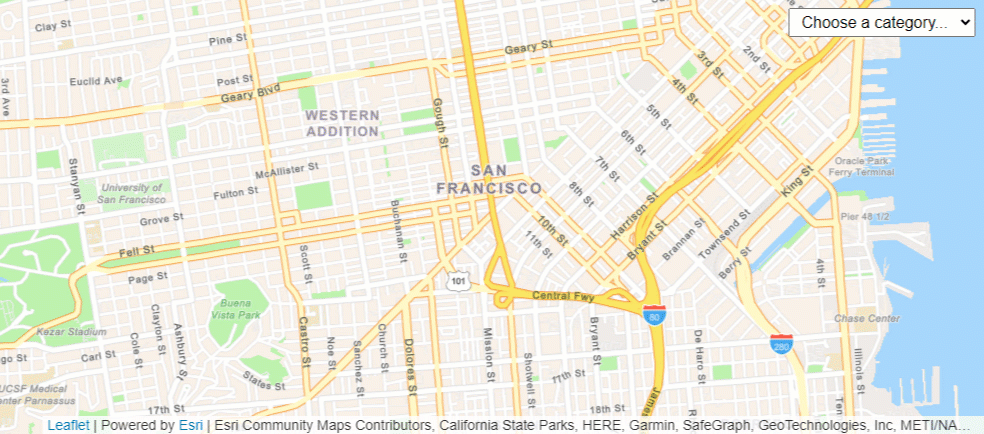
Place addresses for businesses by category with the geocoding service.
Place finding is the process of searching for a place name or POI to find its address and location. You can use the geocoding service to find places such as coffee shops, gas stations, or restaurants for any geographic location around the world. You can search for places by name or by using categories. You can search near a location or you can search globally.
Esri Leaflet provides a built in geocoder to access the geocoding service. In this tutorial, you use the geocode
operation to find places by place category.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
To learn about the other types of authentication available, go to Types of authentication.
Reference the geocoder
-
Reference the Esri Leaflet Geocoder plugin.
Use dark colors for code blocks <!-- Load Leaflet from CDN --> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" crossorigin="" /> <script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js" crossorigin=""></script> <!-- Load Esri Leaflet from CDN --> <script src="https://unpkg.com/esri-leaflet@3.0.12/dist/esri-leaflet.js"></script> <script src="https://unpkg.com/esri-leaflet-vector@4.2.3/dist/esri-leaflet-vector.js"></script> <!-- Load Esri Leaflet Geocoder from CDN --> <script src="https://unpkg.com/esri-leaflet-geocoder@3.1.4/dist/esri-leaflet-geocoder.js"></script>
Update the map
A navigation basemap layer is typically used in geocoding and routing applications. Update the basemap layer to use arcgis/navigation
.
-
Update the basemap style and change the map view to center on location
[151.2093, -33.8688]
, Sydney.Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/navigation"; const map = L.map("map", { minZoom: 2 }) map.setView([37.7749, -122.4194], 14); // San Francisco L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
Create a place category selector
You filter place search results by providing a location and category. Places can be filtered by categories such as coffee shops, gas stations, and hotels. Create a selector to provide a list of several categories from which to choose.
-
Extend the
Control
class to create aPlaces
dropdown. Add HTML and CSS to customize its appearance.Select Use dark colors for code blocks L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map); L.Control.PlacesSelect = L.Control.extend({ onAdd: function (map) { const select = L.DomUtil.create("select", ""); select.setAttribute("id", "optionsSelect"); select.setAttribute("style", "font-size: 16px;padding:4px 8px;"); return select; }, onRemove: function (map) { // Nothing to do here } });
-
Create a list of
place
that be can used to make a selection.Categories Use dark colors for code blocks L.Control.PlacesSelect = L.Control.extend({ onAdd: function (map) { const placeCategories = [ ["", "Choose a category..."], ["Coffee shop", "Coffee shop"], ["Gas station", "Gas station"], ["Food", "Food"], ["Hotel", "Hotel"], ["Parks and Outdoors", "Parks and Outdoors"] ]; const select = L.DomUtil.create("select", ""); select.setAttribute("id", "optionsSelect"); select.setAttribute("style", "font-size: 16px;padding:4px 8px;"); return select; }, onRemove: function (map) { // Nothing to do here } });
-
Add each place category as an
option
in theselect
element.Use dark colors for code blocks L.Control.PlacesSelect = L.Control.extend({ onAdd: function (map) { const placeCategories = [ ["", "Choose a category..."], ["Coffee shop", "Coffee shop"], ["Gas station", "Gas station"], ["Food", "Food"], ["Hotel", "Hotel"], ["Parks and Outdoors", "Parks and Outdoors"] ]; const select = L.DomUtil.create("select", ""); select.setAttribute("id", "optionsSelect"); select.setAttribute("style", "font-size: 16px;padding:4px 8px;"); placeCategories.forEach((category) => { let option = L.DomUtil.create("option"); option.value = category[0]; option.innerHTML = category[1]; select.appendChild(option); }); return select; }, onRemove: function (map) { // Nothing to do here } });
-
Add the category selector to the
topright
corner of the map.Use dark colors for code blocks onRemove: function (map) { // Nothing to do here } }); L.control.placesSelect = function (opts) { return new L.Control.PlacesSelect(opts); }; L.control.placesSelect({ position: "topright" }).addTo(map);
-
Run your app and interact with the category selector, choosing between different options.
Search for place addresses
To find place addresses, you use the geocode
operation. Performing a local search based on a category requires a location from which to search and a category name. The operation calls the geocoding service and returns place candidates with a name, address and location information.
-
Create a function called
show
that takes a category selection as a parameter.Places Use dark colors for code blocks L.control.placesSelect({ position: "topright" }).addTo(map); function showPlaces(category) { }
-
Call the
geocode
operation and set youraccess
. Pass the category, and set the search location to the center of the map.Token Use dark colors for code blocks function showPlaces(category) { L.esri.Geocoding .geocode({ apikey: accessToken }) .category(category) .nearby(map.getCenter(), 10) }
-
Execute the request using
run
and handle any errors within the callback function.Use dark colors for code blocks function showPlaces(category) { L.esri.Geocoding .geocode({ apikey: accessToken }) .category(category) .nearby(map.getCenter(), 10) .run(function (error, response) { if (error) { return; } }); }
Add a change event handler
Use an event handler to call the show
function when the category is changed.
-
Add a
change
event handler to theselect
element. Inside, call theshow
function with the selected category as its parameter.Places Use dark colors for code blocks L.control.placesSelect({ position: "topright" }).addTo(map); function showPlaces(category) { L.esri.Geocoding .geocode({ apikey: accessToken }) .category(category) .nearby(map.getCenter(), 10) } const select = document.getElementById("optionsSelect"); select.addEventListener("change", () => { if (select.value !== "") { showPlaces(select.value); } });
Display results
You can display the results
of the search with a Marker
and Popup
.
-
Add a
Layer
to the map to contain the results.Group Use dark colors for code blocks L.control.placesSelect({ position: "topright" }).addTo(map); const layerGroup = L.layerGroup().addTo(map); function showPlaces(category) { L.esri.Geocoding .geocode({ apikey: accessToken }) .category(category) .nearby(map.getCenter(), 10) .run(function (error, response) { if (error) { return; } }); }
-
In the
show
function, call thePlaces clear
method to remove the previous results from theLayers layer
.Group Use dark colors for code blocks L.control.placesSelect({ position: "topright" }).addTo(map); const layerGroup = L.layerGroup().addTo(map); function showPlaces(category) { L.esri.Geocoding .geocode({ apikey: accessToken }) .category(category) .nearby(map.getCenter(), 10) .run(function (error, response) { if (error) { return; } layerGroup.clearLayers(); }); }
-
Iterate through each search result to create a
Marker
and add the markers to thelayer
. Call theGroup bind
method to display the place names and addresses in a popup.Popup Use dark colors for code blocks function showPlaces(category) { L.esri.Geocoding .geocode({ apikey: accessToken }) .category(category) .nearby(map.getCenter(), 10) .run(function (error, response) { if (error) { return; } layerGroup.clearLayers(); response.results.forEach((searchResult) => { L.marker(searchResult.latlng) .addTo(layerGroup) .bindPopup(`<b>${searchResult.properties.PlaceName}</b></br>${searchResult.properties.Place_addr}`); }); }); }
Run the app
In CodePen, run your code to display the map.
When you choose a place category, you should see the places returned with pins on the map. Click each pin to view a pop-up with the place information.
What's next?
Learn how to use additional ArcGIS location services in these tutorials: