Learn how to use Esri Leaflet and the basemap styles service to display a map.
You can display a map with Esri Leaflet by using a vector tile basemap layer from the basemap styles service. A vector tile basemap layer contains the styles, layers, font glyphs, and icons to render the layers.
In this tutorial, you display a map of the Santa Monica Mountains using the streets basemap layer from the Basemap styles service.
This code is used as the starting point for the other Esri Leaflet tutorials.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- Go to CodePen to create a new pen for your application.
Add HTML
Define an HTML page to create a map that is the full width and height of the browser window.
-
In CodePen > HTML, add HTML and CSS to create a page with a div element called
map
.Use the HTML to create a page with a map. Use the
map
div to display the map. Use the CSS to make the map full width and height.The
<!DOCTYPE html>
tag is not required in CodePen. If you are using a different editor or running the page on a local server, be sure to add this tag to the top of your HTML page.Use dark colors for code blocks <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no" /> <title>Esri Leaflet Tutorials: Display a map</title> <style> body { margin: 0; padding: 0; } #map { position: absolute; top: 0; bottom: 0; right: 0; left: 0; font-family: Arial, Helvetica, sans-serif; font-size: 14px; color: #323232; } </style> </head> <body> <div id="map"></div> </body> </html>
Add script references
To access vector basemap layers, you reference both the esri-leaflet
and the esri-leaflet-vector
plugins in addition to theleaflet
libraries.
-
Add
<script>
and<link>
tags to reference the libraries.Use dark colors for code blocks <head> <meta charset="utf-8" /> <meta name="viewport" content="initial-scale=1,maximum-scale=1,user-scalable=no" /> <title>Esri Leaflet Tutorials: Display a map</title> <!-- Load Leaflet from CDN --> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" crossorigin="" /> <script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js" crossorigin=""></script> <!-- Load Esri Leaflet from CDN --> <script src="https://unpkg.com/esri-leaflet@3.0.12/dist/esri-leaflet.js"></script> <script src="https://unpkg.com/esri-leaflet-vector@4.2.3/dist/esri-leaflet-vector.js"></script>
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
- Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Privileges
- Copy the API key access token as it will be used in the next step.
To learn about other ways to get an access token, go to Types of authentication.
Create a map
Use a map
to add a map to the div
element with the basemap you specify. To find the list of default basemap styles, go to Basemap styles service.
-
Add
<script>
elements in the HTML<body>
. Create amap
with themin
set toZoom 2
.Use dark colors for code blocks <body> <div id="map"></div> <script> const map = L.map("map", { minZoom: 2 }) </script> </body>
-
Call the
set
method to center the map on the coordinatesView 34.02, -118.805
and to set the zoom level to13
.Use dark colors for code blocks <script> const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); </script>
-
Create an
access
variable to store your access token. ReplaceToken YOUR_
with the access token you previously copied from your API key credentials.ACCESS_ TOKEN Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const accessToken = "YOUR_ACCESS_TOKEN"; </script>
-
Create a
basemap
variable to store the basemap identifier,Enum ArcGIS:
.Streets Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/streets"; </script>
-
Instantiate the
vector
class and set theBasemap Layer basemap
andEnum access
before adding the layer to theToken map
.Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
Run the app
In CodePen, run your code to display the map. The map should display a street basemap layer centered on Point Dume in California.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
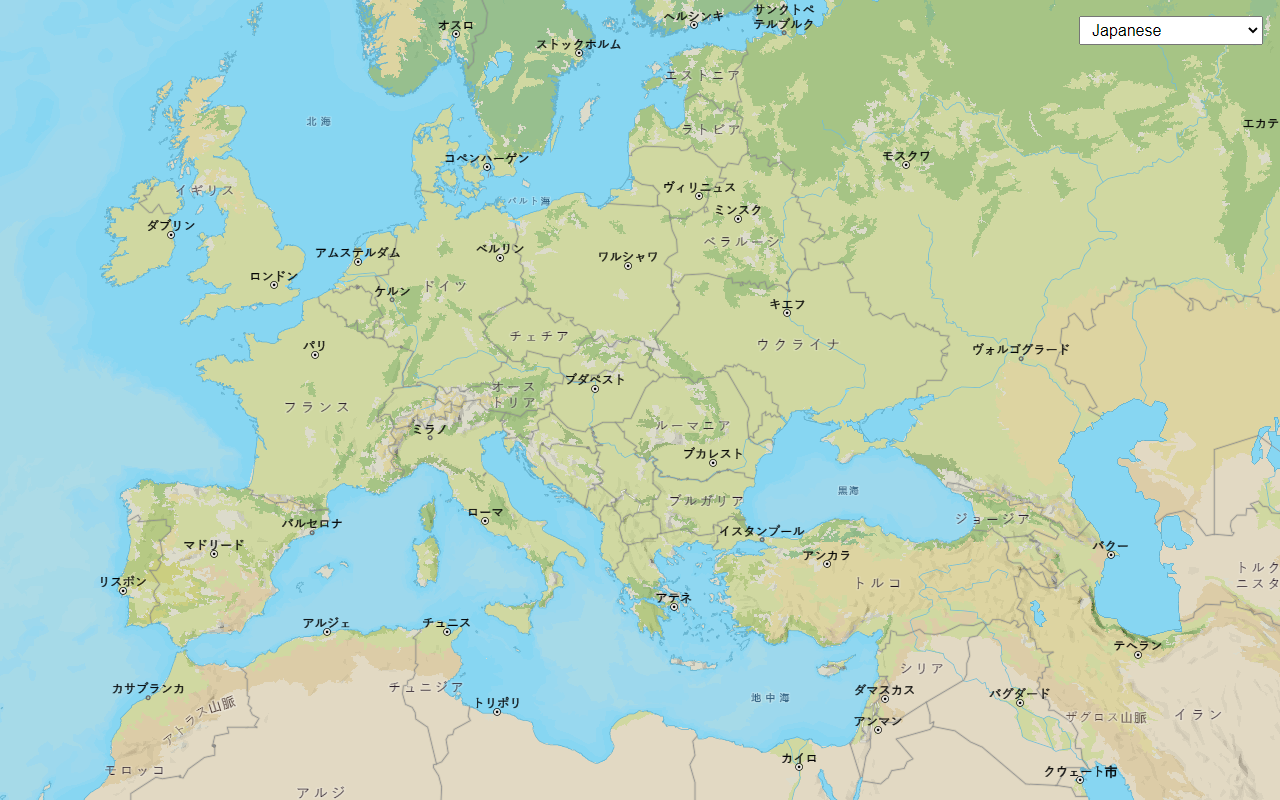
Change the place label language
Switch the language of place labels on a basemap.
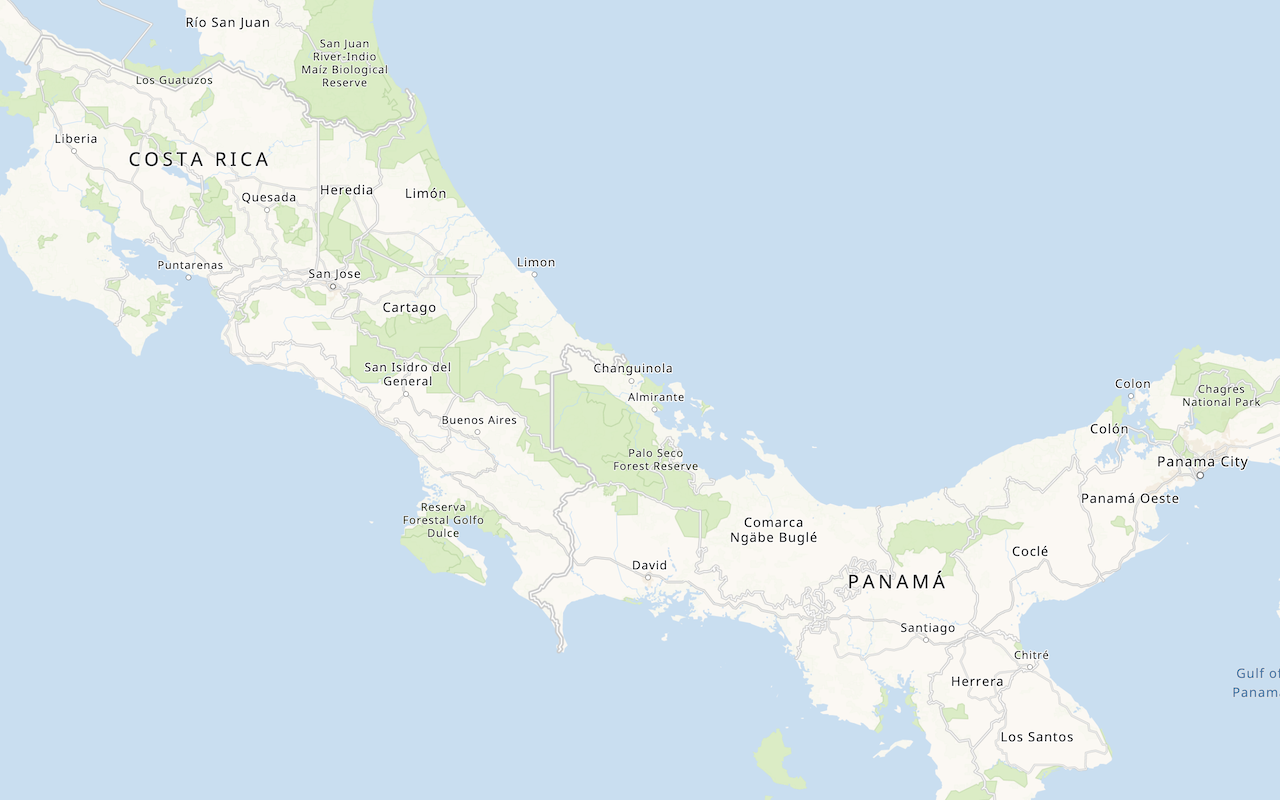
Display a custom basemap style
Add a styled vector basemap layer to a map.
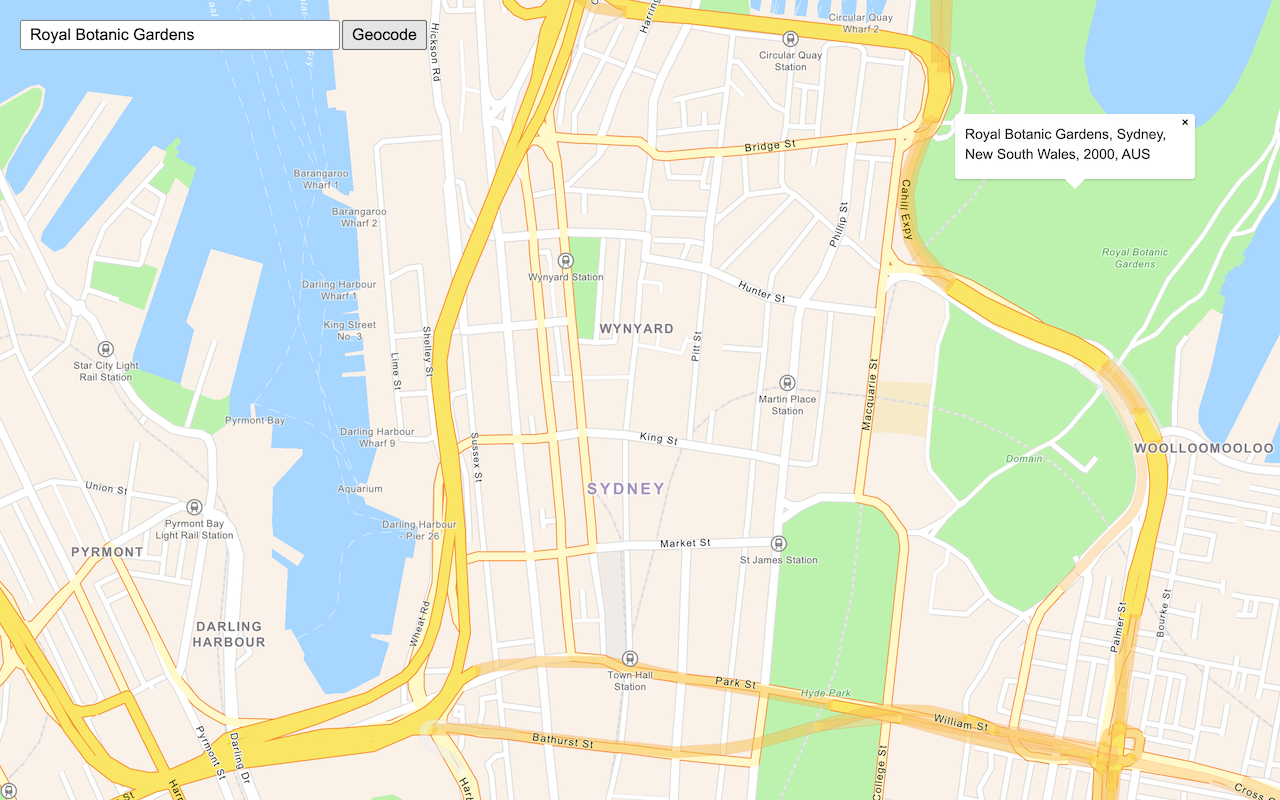
Search for an address
Find an address or place using a search box and the geocoding service.
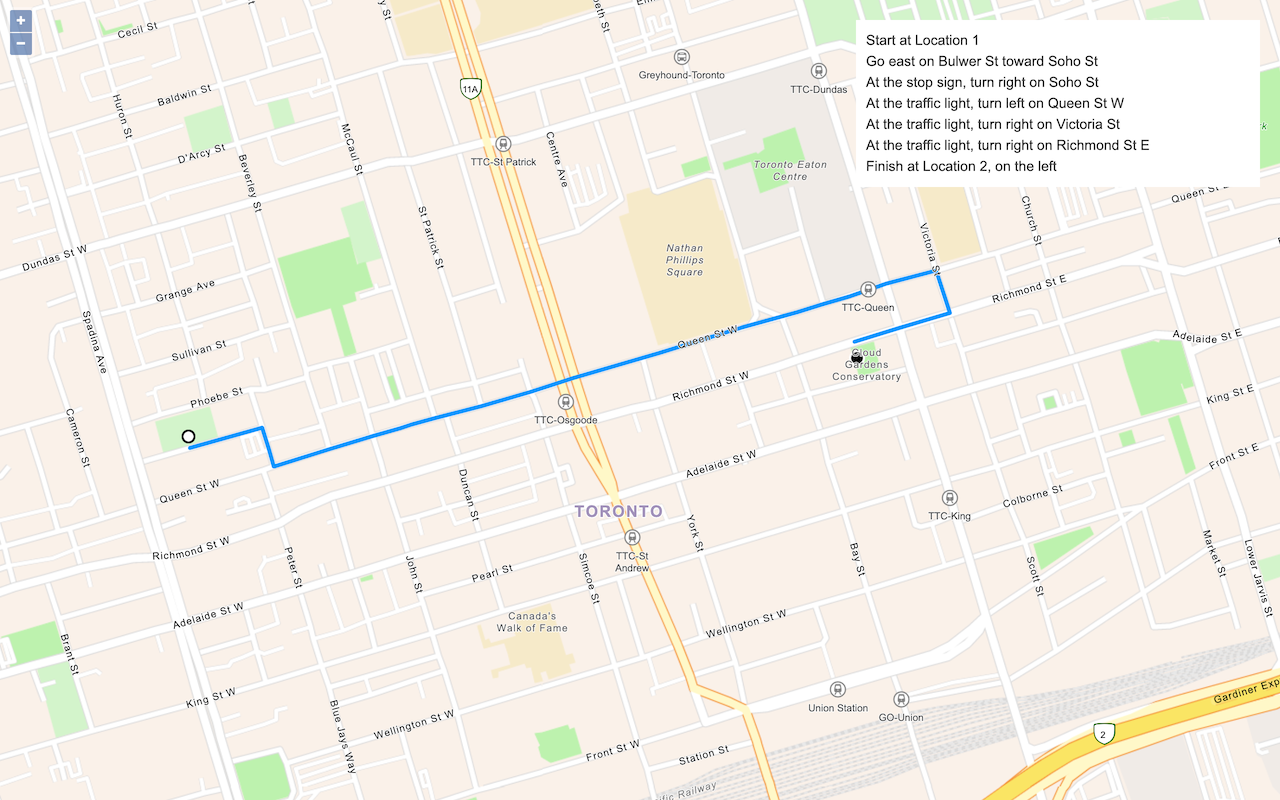
Find a route and directions
Find a route and directions with the route service.