A map is a container for layers. You use a map together with a map view to display layers of geographic data in 2D. Most applications contain a basemap layer to display geographic data with streets or satellite imagery, and the data is typically provided by the basemap styles service.
You can use the basemap styles service in your application to:
- Display different types of geographic data of the world for a map.
- Display default vector tile basemap styles such as streets navigation, light gray canvas, and OSM.
- Display custom basemap styles with your own colors, glyphs, and fonts.
- Display map tile (raster) basemap layers for satellite imagery and hillshade.
How to access the basemap styles service
To access vector basemap layers, you reference both the main Esri Leaflet plugin and the vector
plugin.
- Reference the Esri Leaflet and vector plugins.
- Define a basemap style enumeration.
- Instantiate the
vector
class.Basemap Layer - Set the basemap enumeration and API key.
Example
Display a basemap layer (v1)
This example loads and displays the ArcGIS:
vector tile style in the map. To see all of the basemap style enumerations, go to Basemap styles service in the Mapping APIs and location services guide.
<!-- Esri Leaflet -->
<script src="https://unpkg.com/esri-leaflet@3.0.12/dist/esri-leaflet.js"
integrity="sha512-oUArlxr7VpoY7f/dd3ZdUL7FGOvS79nXVVQhxlg6ij4Fhdc4QID43LUFRs7abwHNJ0EYWijiN5LP2ZRR2PY4hQ=="
crossorigin=""></script>
<!-- Vector basemap layer plugin -->
<script src="https://unpkg.com/esri-leaflet-vector@4.2.3/dist/esri-leaflet-vector.js"
integrity="sha512-7rLAors9em7cR3/583gZSvu1mxwPBUjWjdFJ000pc4Wpu+fq84lXF1l4dbG4ShiPQ4pSBUTb4e9xaO6xtMZIlA=="
crossorigin=""></script>
<script>
const apiKey = "YOUR_API_KEY";
const basemapEnum = "ArcGIS:Streets";
const map = L.map('map', {
minZoom: 2
}).setView([34.02,-118.805], 13);
L.esri.Vector.vectorBasemapLayer(basemapEnum, {
apiKey: apiKey
}).addTo(map);
</script>
Display a basemap layer (v2)
This example loads and displays the arcgis/streets
style from version 2 of the basemap styles service. To see all of the basemap style enumerations available in the v2 service, go to the Basemap styles service API Reference.
<!-- Esri Leaflet -->
<script src="https://unpkg.com/esri-leaflet@3.0.12/dist/esri-leaflet.js"
integrity="sha512-oUArlxr7VpoY7f/dd3ZdUL7FGOvS79nXVVQhxlg6ij4Fhdc4QID43LUFRs7abwHNJ0EYWijiN5LP2ZRR2PY4hQ=="
crossorigin=""></script>
<!-- Vector basemap layer plugin -->
<script src="https://unpkg.com/esri-leaflet-vector@4.2.3/dist/esri-leaflet-vector.js"
integrity="sha512-7rLAors9em7cR3/583gZSvu1mxwPBUjWjdFJ000pc4Wpu+fq84lXF1l4dbG4ShiPQ4pSBUTb4e9xaO6xtMZIlA=="
crossorigin=""></script>
<script>
const apiKey = "YOUR_API_KEY";
const basemapEnum = "arcgis/streets";
const map = L.map('map', {
minZoom: 2
}).setView([34.02,-118.805], 13);
L.esri.Vector.vectorBasemapLayer(basemapEnum, {
apiKey: apiKey,
version: 2
}).addTo(map);
</script>
Tutorials
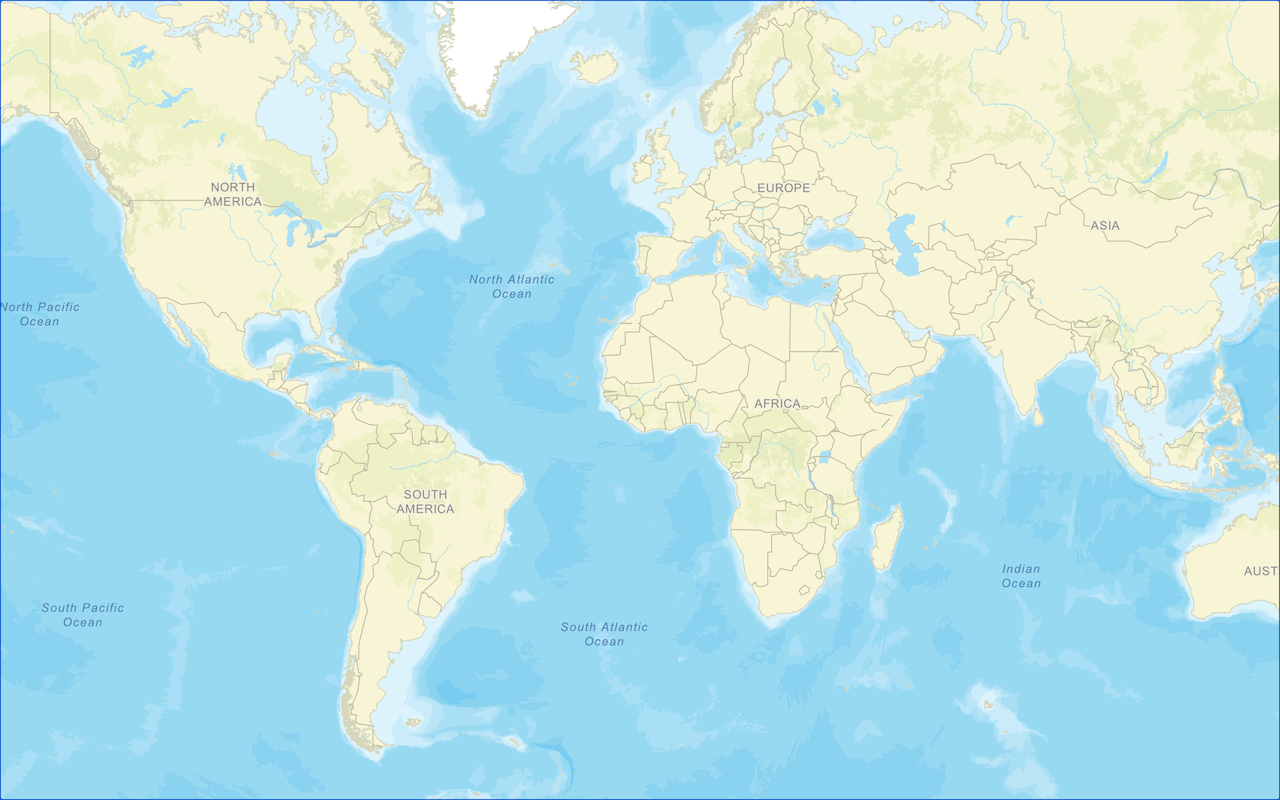
Display a map
Create and display a map with the basemap styles service.
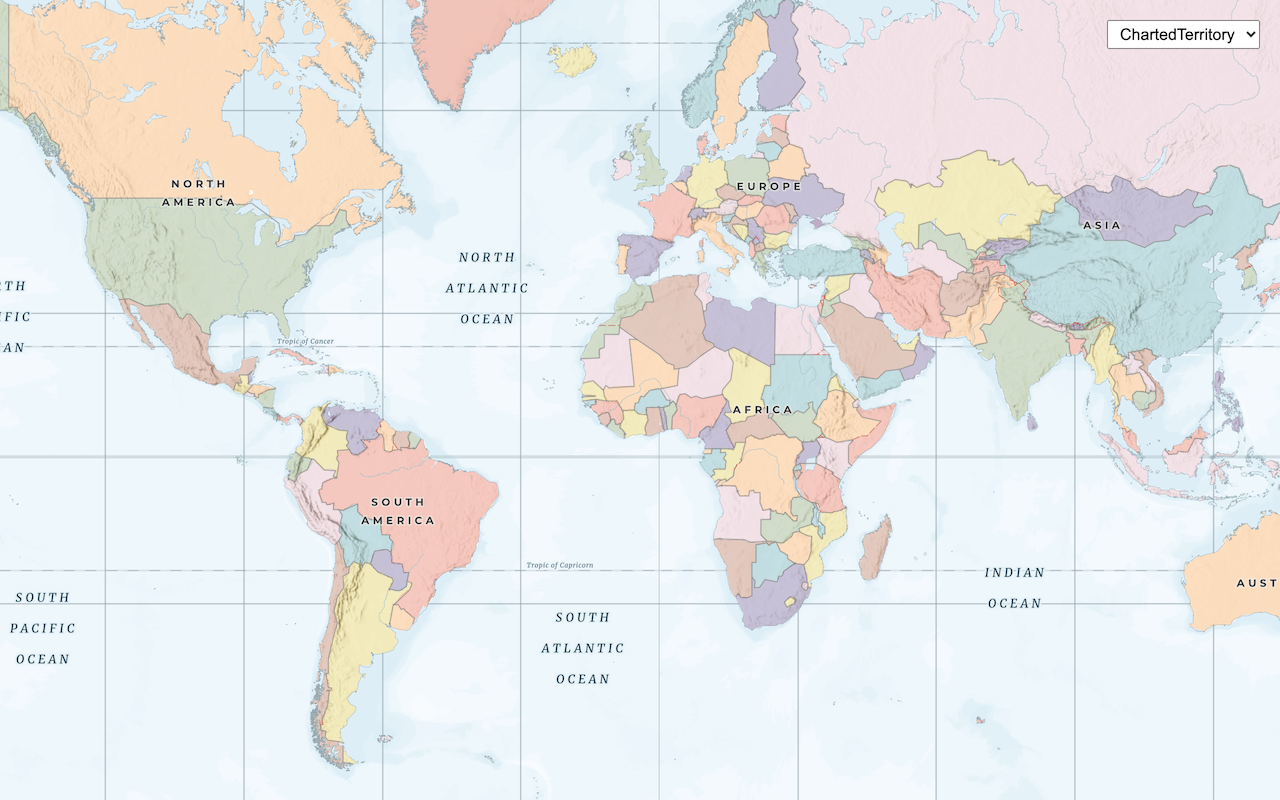
Change the basemap style
Switch a basemap style in a map.
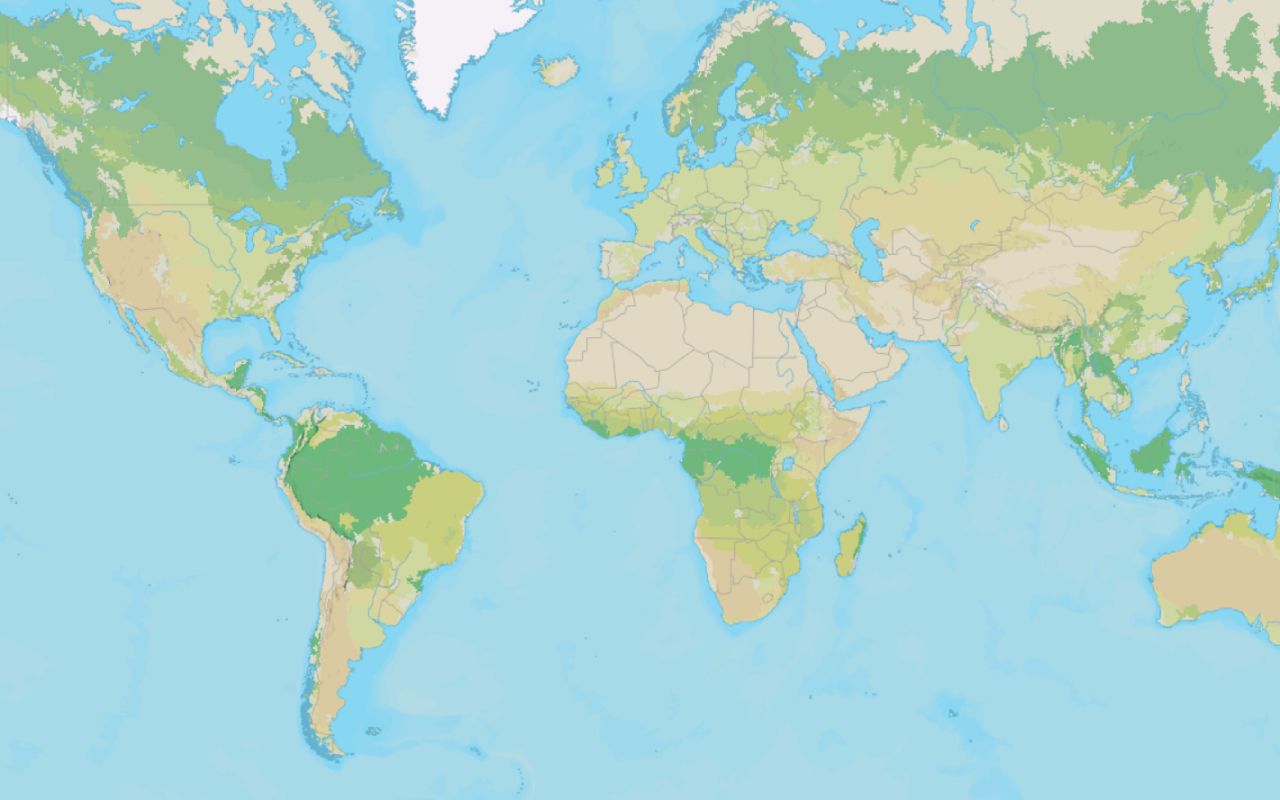
Change the basemap style (v2)
Switch a basemap style in a map using the basemap styles service v2.
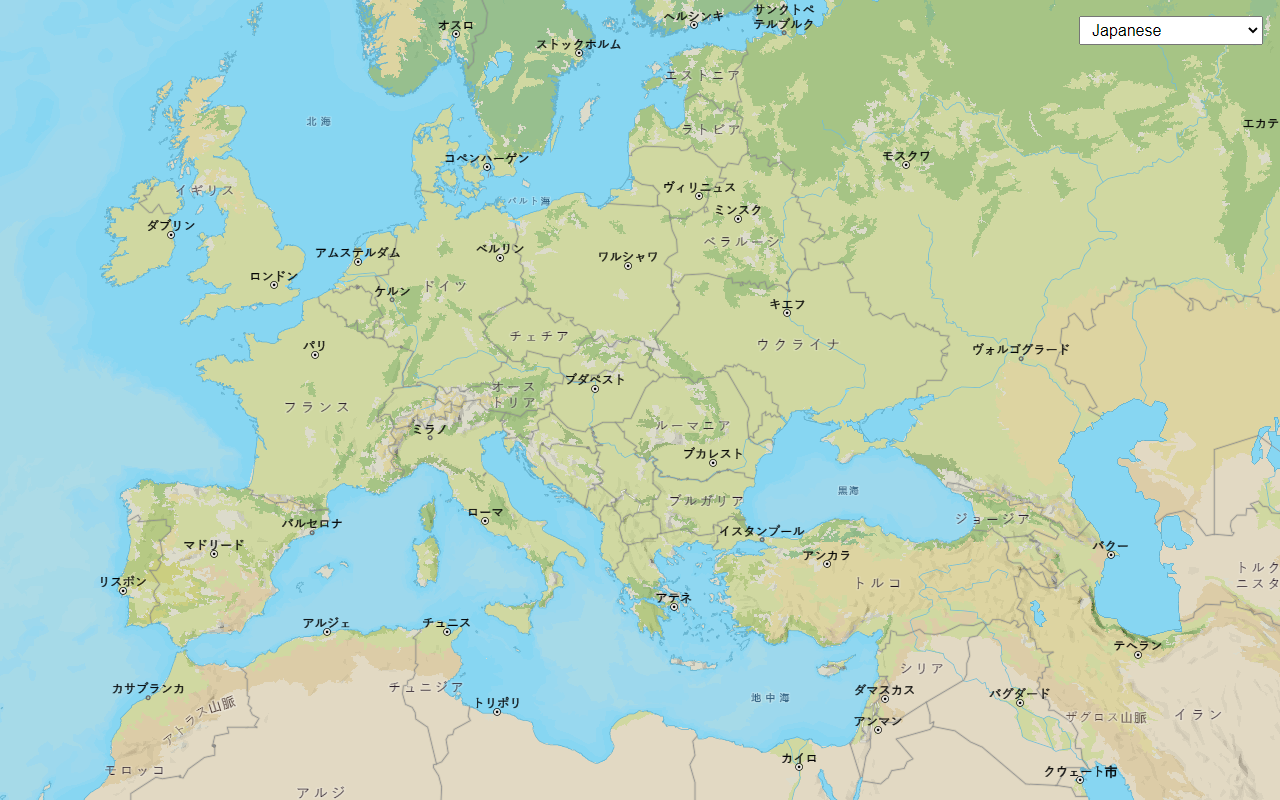
Change the place label language
Switch the language of place labels on a basemap.