Learn how to add features from feature layers to a map.
A feature layer is a dataset in a hosted feature service. Each feature layer contains features with a single geometry type (point, line, or polygon), and a set of attributes. You can access and display features by making query requests to the feature service and displaying them in a map.
In this tutorial, you access and display three different hosted feature layers.
The feature layers are:
Prerequisites
You need an ArcGIS Developer or ArcGIS Online account to access the developer dashboard and create an API key.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Set the API key
To access location services, you need an API key or OAuth 2.0 access token. To learn how to create and scope your key, visit the Create an API key tutorial.
-
Go to your dashboard to get an API key. The API key must be scoped to access the services used in this tutorial.
-
In CodePen, update
api
to use your key.Key Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const apiKey = "YOUR_API_KEY"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { apiKey: apiKey }).addTo(map);
Add a point feature layer
Point features are typically displayed in a feature layer on top of all other layers. Use the Feature
class to reference the Trailheads URL and add features to the map.
-
Go to the Trailheads URL and browse the properties of the layer. Make note of the Name, Type, Drawing Info, and Fields properties.
-
In CodePen, create a
Feature
and set theLayer url
property.Use dark colors for code blocks L.esri.Vector.vectorBasemapLayer(basemapEnum, { apiKey: apiKey }).addTo(map); var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" });
-
Add the
trailheads
layer to the map.Use dark colors for code blocks L.esri.Vector.vectorBasemapLayer(basemapEnum, { apiKey: apiKey }).addTo(map); var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" }); trailheads.addTo(map);
-
Run the app to view the Trailheads layer in the map.
Add a line feature layer
Line features are typically displayed in a feature layer below points. Use the Feature
class to reference the Trails URL and add features to the map.
-
Go to the Trails URL and browse the properties of the layer. Make note of the Name, Type, Drawing Info, and Fields properties.
-
In CodePen, create a
Feature
and set theLayer url
property.Use dark colors for code blocks var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" }); trailheads.addTo(map); var trails = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0" });
-
Add the
trails
layer to the map.Use dark colors for code blocks var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" }); trailheads.addTo(map); var trails = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0" }); trails.addTo(map);
-
Run the app to view the Trails layer in the map.
Add a polygon feature layer
Polygon features are typically displayed in a feature layer below lines. Use the Feature
class to reference the Parks and Open Spaces URL and add features to the map.
-
Go to the Parks and Open Spaces URL and browse the properties of the layer. Make note of the Name, Type, Drawing Info, and Fields properties.
-
In CodePen, create a
Feature
and set theLayer url
property.Use dark colors for code blocks var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" }); trailheads.addTo(map); var trails = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0" }); trails.addTo(map); var parks = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0" });
-
Add the
parks
layer to the map.Use dark colors for code blocks var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" }); trailheads.addTo(map); var trails = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0" }); trails.addTo(map); var parks = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0" }); parks.addTo(map);
Run the app
In CodePen, run your code to display the map.
Your map should display the locations of trailheads, trails, and open spaces in the Santa Monica Mountains.
The map view should display all three feature layers in the map. The map view draws the map in following order:
- Topographic basemap layer
- Parks and Open Spaces (polygons)
- Trails (lines)
- Trailheads (points)
It is important to add feature layers in the correct order so that features are displayed correctly (not overlapping) and so you can interact with the features.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
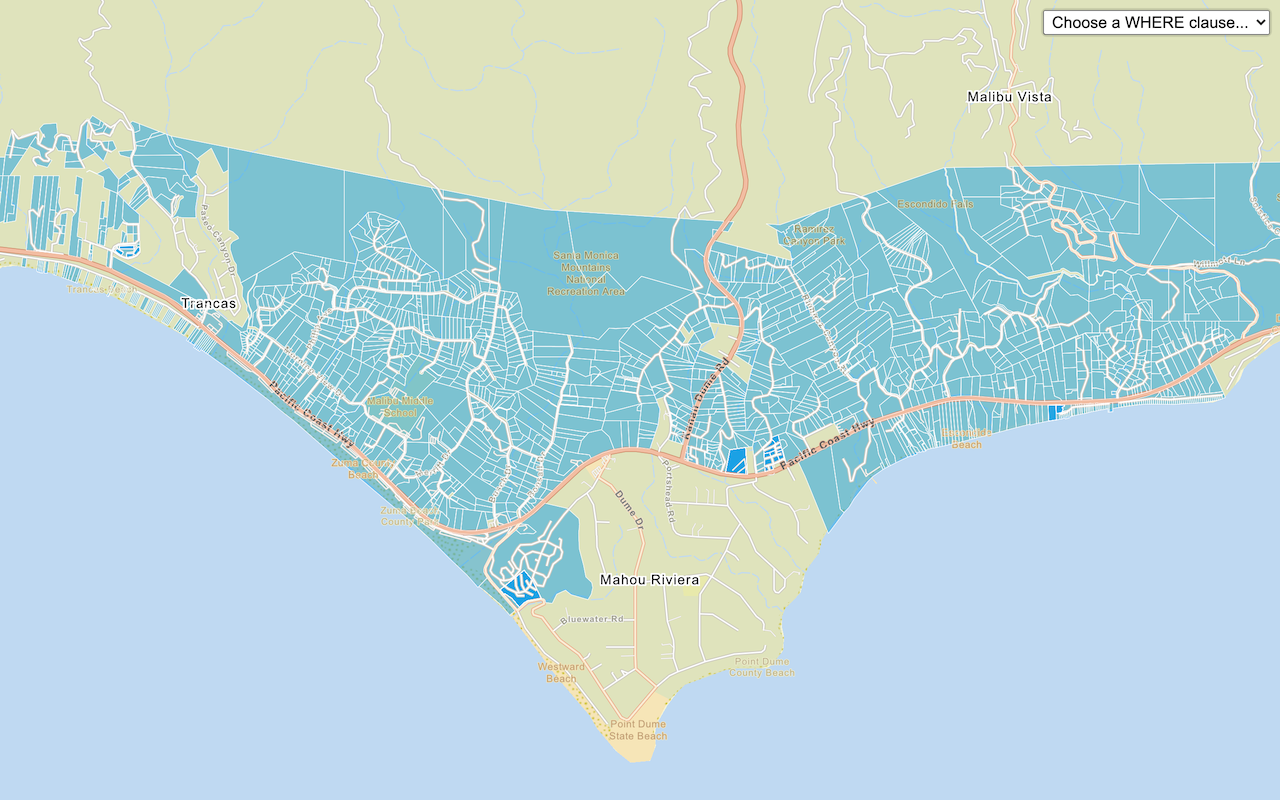
Query a feature layer (SQL)
Execute a SQL query to access polygon features from a feature layer.
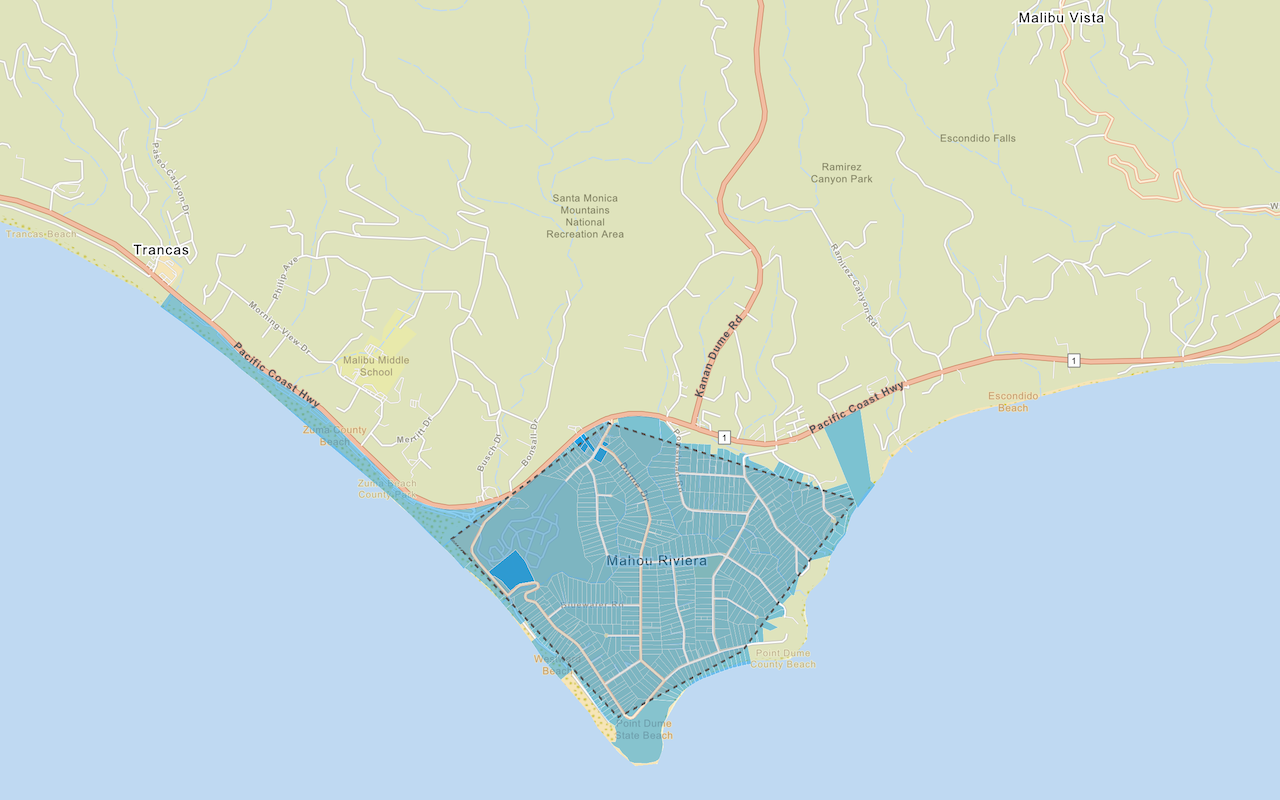
Query a feature layer (spatial)
Execute a spatial query to access polygon features from a feature service.
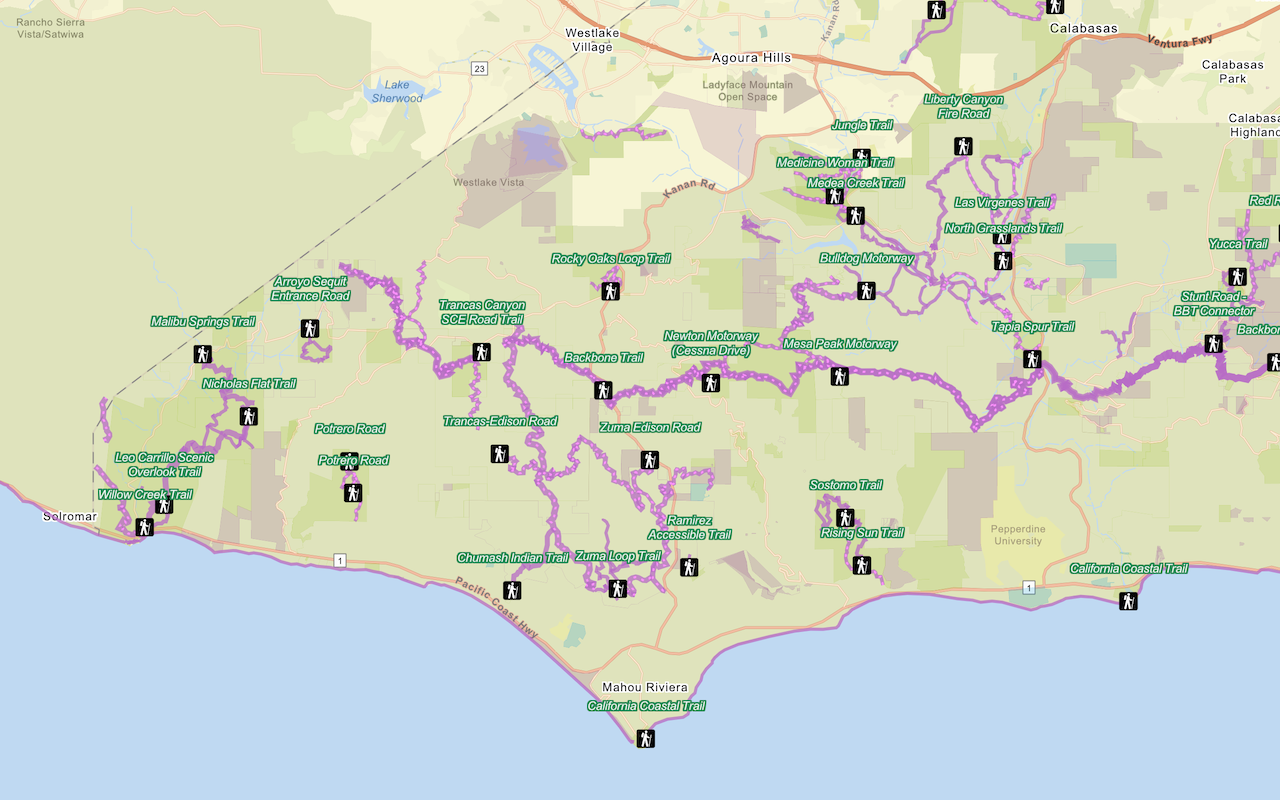
Style a feature layer
Use data-driven styling to apply symbol colors and styles to feature layers.
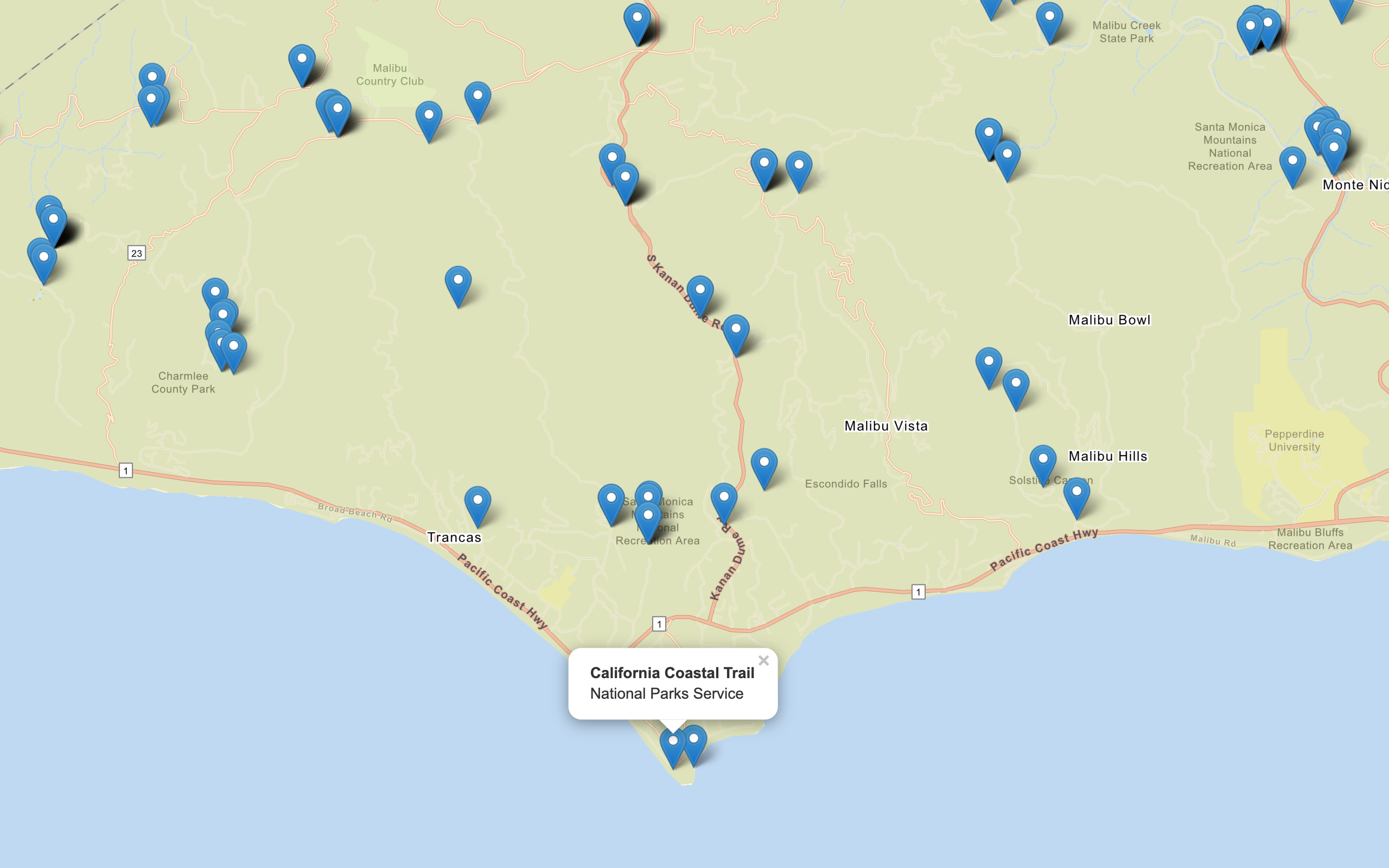
Display a pop-up
Display feature attributes in a popup.