Learn how to execute a SQL query to access polygon features in a feature layer.
A feature layer can contain a large number of features stored in ArcGIS. To access a subset of the features, you can execute a SQL or spatial query, or both at the same time. You can also return the attributes, geometry, or both attributes and geometry for each record. SQL and spatial queries are useful when a feature layer is very large and you just want to access a subset of the data.
In this tutorial, you perform server-side SQL queries to return a subset of the features from the LA County Parcel feature layer. The feature layer contains over 2.4 million features. The resulting features are displayed as graphics on the map. A pop-up is also used to display feature attributes.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Item access
- Note: If you are using your own custom data layer for this tutorial, you need to grant the API key credentials access to the layer item. Learn more in Item access privileges.
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
To learn about the other types of authentication available, go to Types of authentication.
Create a SQL selector
ArcGIS feature layers support a standard SQL query where clause. Use a drop-down select
element to provide a list of SQL queries for the LA County Parcels feature layer.
-
Create a
Query
using theControl L.Control
class. Create an ono
function that contains each where clause. The selector containing the where clauses will not be removed from the map.n Add Use dark colors for code blocks L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map); L.Control.QueryControl = L.Control.extend({ onAdd: function (map) { const whereClauses = [ "Choose a WHERE clause...", "UseType = 'Residential'", "UseType = 'Government'", "UseType = 'Irrigated Farm'", "TaxRateArea = 10853", "TaxRateArea = 10860", "TaxRateArea = 08637", "Roll_LandValue > 1000000", "Roll_LandValue < 1000000" ]; }, onRemove: function (map) { // Nothing to do here } });
-
Create a
select
dropdown to display thewhere
.Clauses Use dark colors for code blocks L.Control.QueryControl = L.Control.extend({ onAdd: function (map) { const whereClauses = [ "Choose a WHERE clause...", "UseType = 'Residential'", "UseType = 'Government'", "UseType = 'Irrigated Farm'", "TaxRateArea = 10853", "TaxRateArea = 10860", "TaxRateArea = 08637", "Roll_LandValue > 1000000", "Roll_LandValue < 1000000" ]; const select = L.DomUtil.create("select", ""); select.setAttribute("id", "whereClauseSelect"); select.setAttribute("style", "font-size: 16px;padding:4px 8px;"); whereClauses.forEach(function (whereClause) { let option = L.DomUtil.create("option"); option.innerHTML = whereClause; select.appendChild(option); }); return select; }, onRemove: function (map) { // Nothing to do here } });
-
Add the
Query
to the map.Control Use dark colors for code blocks onRemove: function (map) { // Nothing to do here } }); L.control.queryControl = function (opts) { return new L.Control.QueryControl(opts); }; L.control .queryControl({ position: "topright" }) .addTo(map);
Add the parcel layer
Use the Feature
class to access the LA County Parcel feature layer. Since you are performing a server-side query, you can hide the features until a SQL query is selected.
-
Create a
Feature
and set theLayer url
with the LA County Parcel service URL.Use dark colors for code blocks L.control .queryControl({ position: "topright" }) .addTo(map); const parcels = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/LA_County_Parcels/FeatureServer/0", simplifyFactor: 0.5, precision: 4, }) .addTo(map);
-
Hide all features in the layer using a layer definition.
Use dark colors for code blocks const parcels = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/LA_County_Parcels/FeatureServer/0", simplifyFactor: 0.5, precision: 4, where: "1 = 0" }) .addTo(map);
Execute the query
The parcels feature layer layer definition changes when you choose an option from the selector. You can access the chosen where
clause with the value
property of the select
element.
The maximum number of features returned by a query for hosted feature layers is 2000. To return more, you need to detect the request exceeded the maximum feature amount with exceeded
, and then use the result
parameter to make multiple requests with the appropriate offset values. To learn more, visit the REST services documentation.
-
Add a
change
event handler to theselect
element. Inside, useset
to change the layer definition.Where Use dark colors for code blocks const parcels = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/LA_County_Parcels/FeatureServer/0", simplifyFactor: 0.5, precision: 4, where: "1 = 0" }) .addTo(map); const select = document.getElementById("whereClauseSelect"); select.addEventListener("change", () => { if (select.value !== "") { parcels.setWhere(select.value); } });
-
Create a pop-up using the
bind
method. Set its contents dynamically based on the currently clicked feature.Popup Use dark colors for code blocks const select = document.getElementById("whereClauseSelect"); select.addEventListener("change", () => { if (select.value !== "") { parcels.setWhere(select.value); } }); parcels.bindPopup(function (layer) { return L.Util.template("<b>Parcel {APN}</b>" + "Type: {UseType} <br>" + "Tax Rate City: {TaxRateCity}", layer.feature.properties); }); </script>
Run the app
In CodePen, run your code to display the map.
When the map displays, you should be able to use the query selector to display parcels. Click on a parcel to show a pop-up with the feature's attributes.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
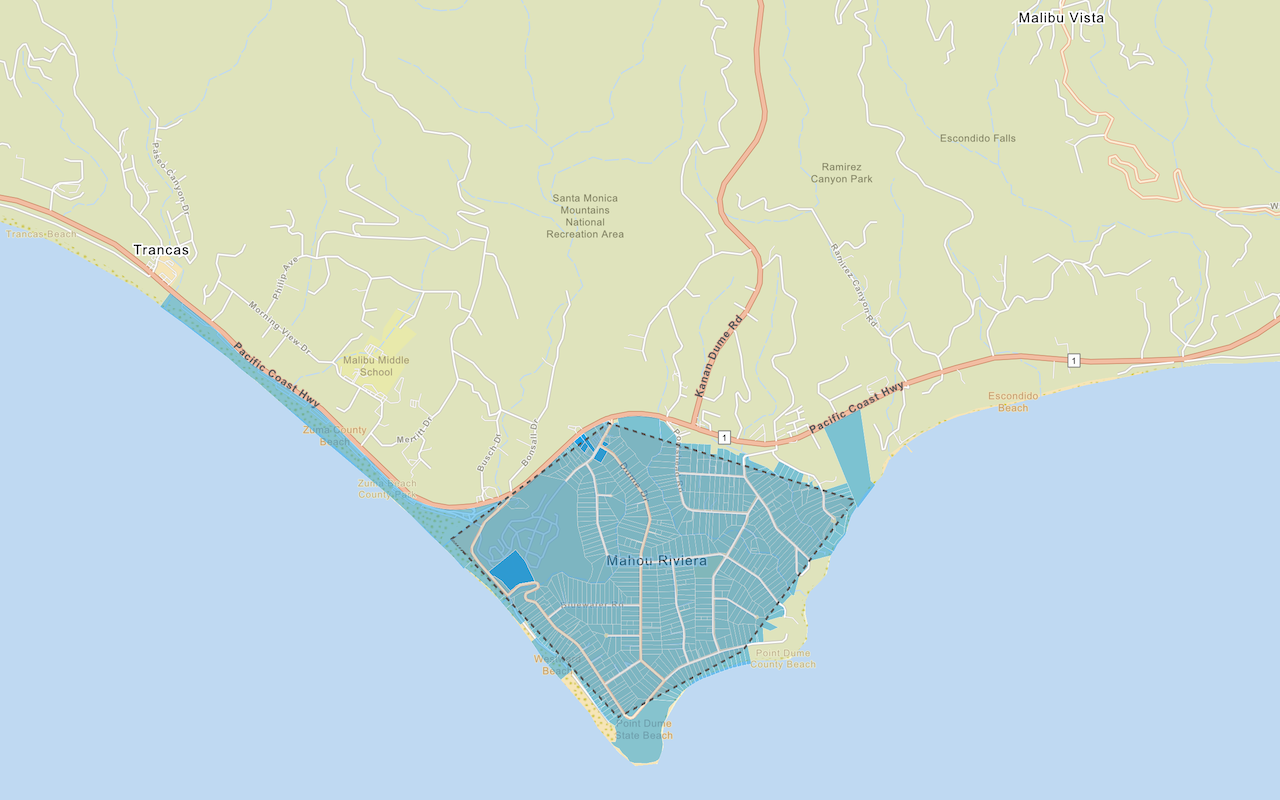
Query a feature layer (spatial)
Execute a spatial query to access polygon features from a feature service.
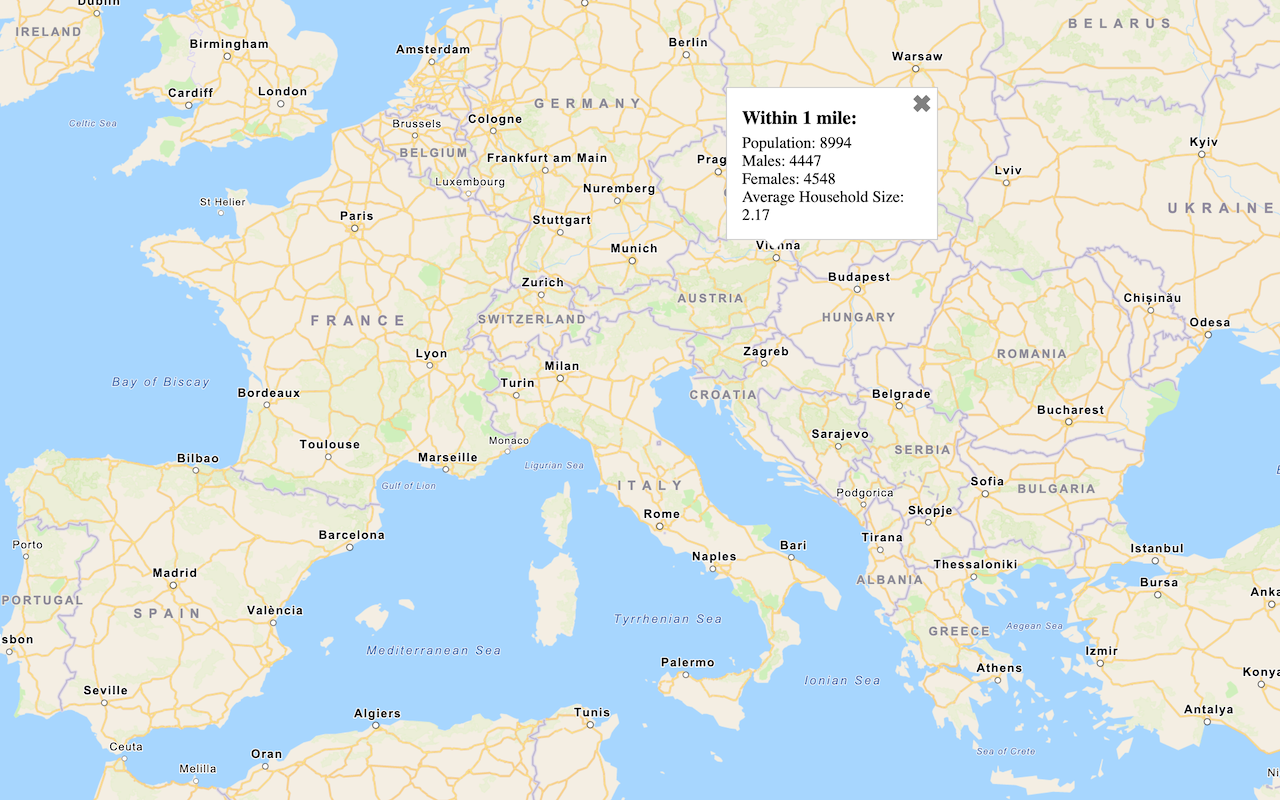
Get global data
Query demographic information for locations around the world with the GeoEnrichment service.
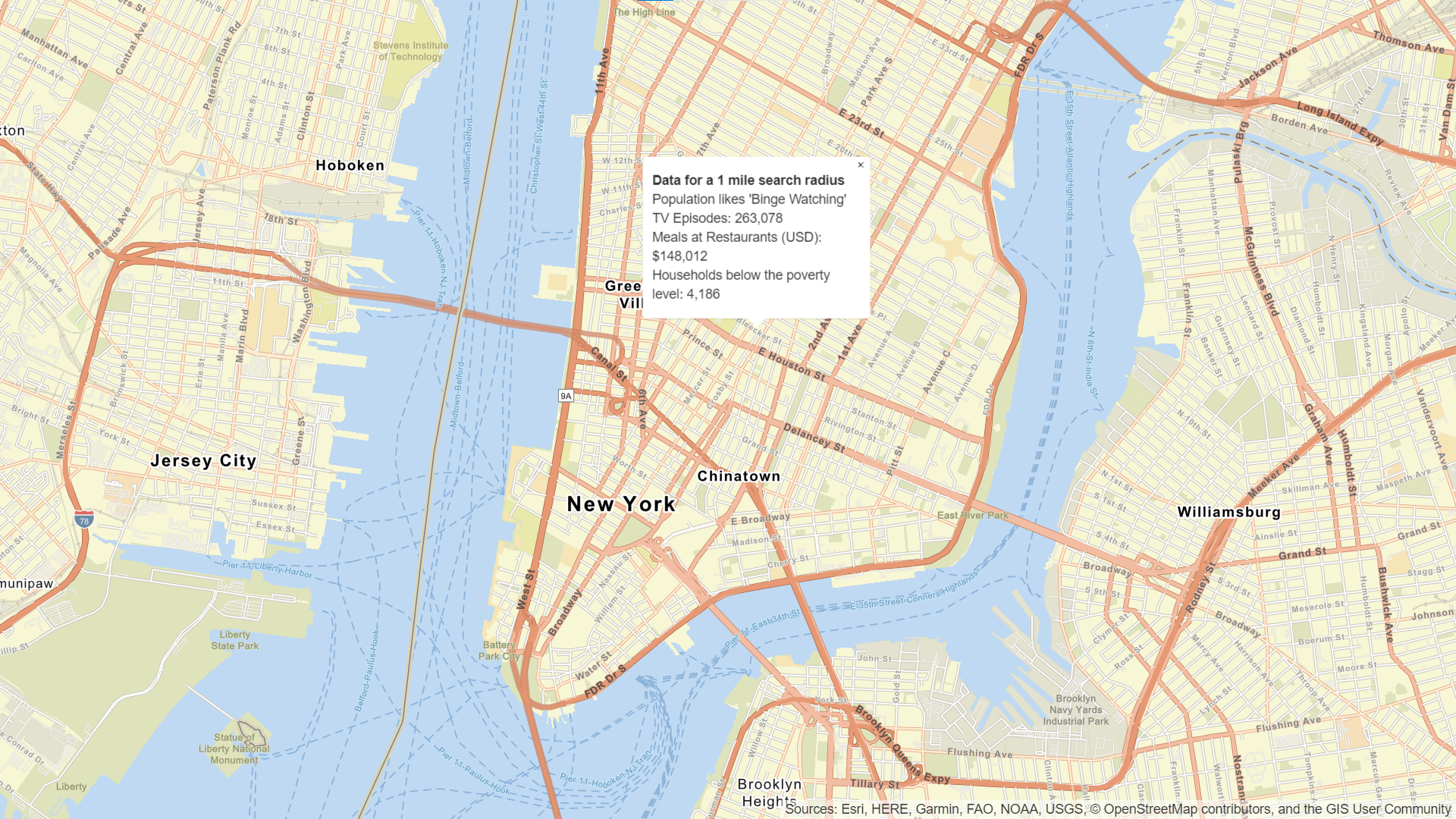
Get local data
Query regional facts, spending trends, and psychographics with the GeoEnrichment service.
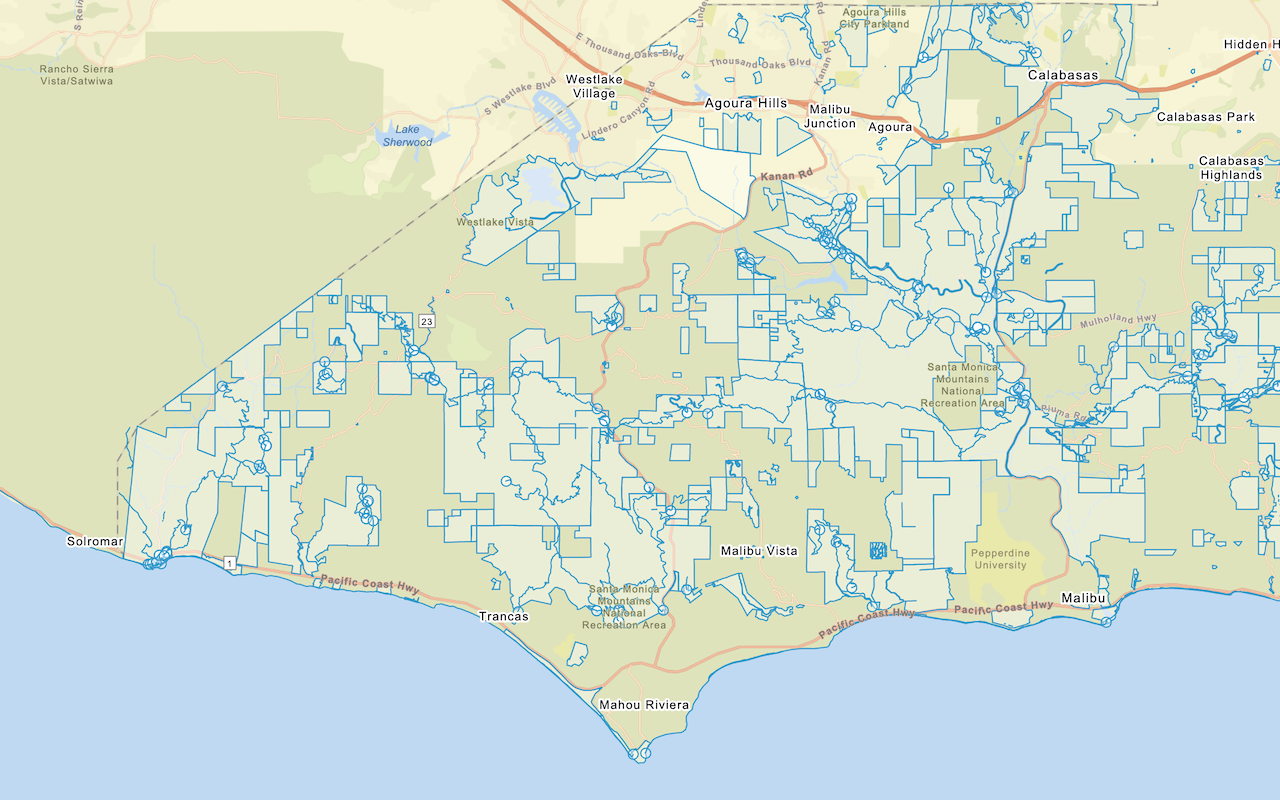
Add a feature layer
Add features from feature layers to a map.
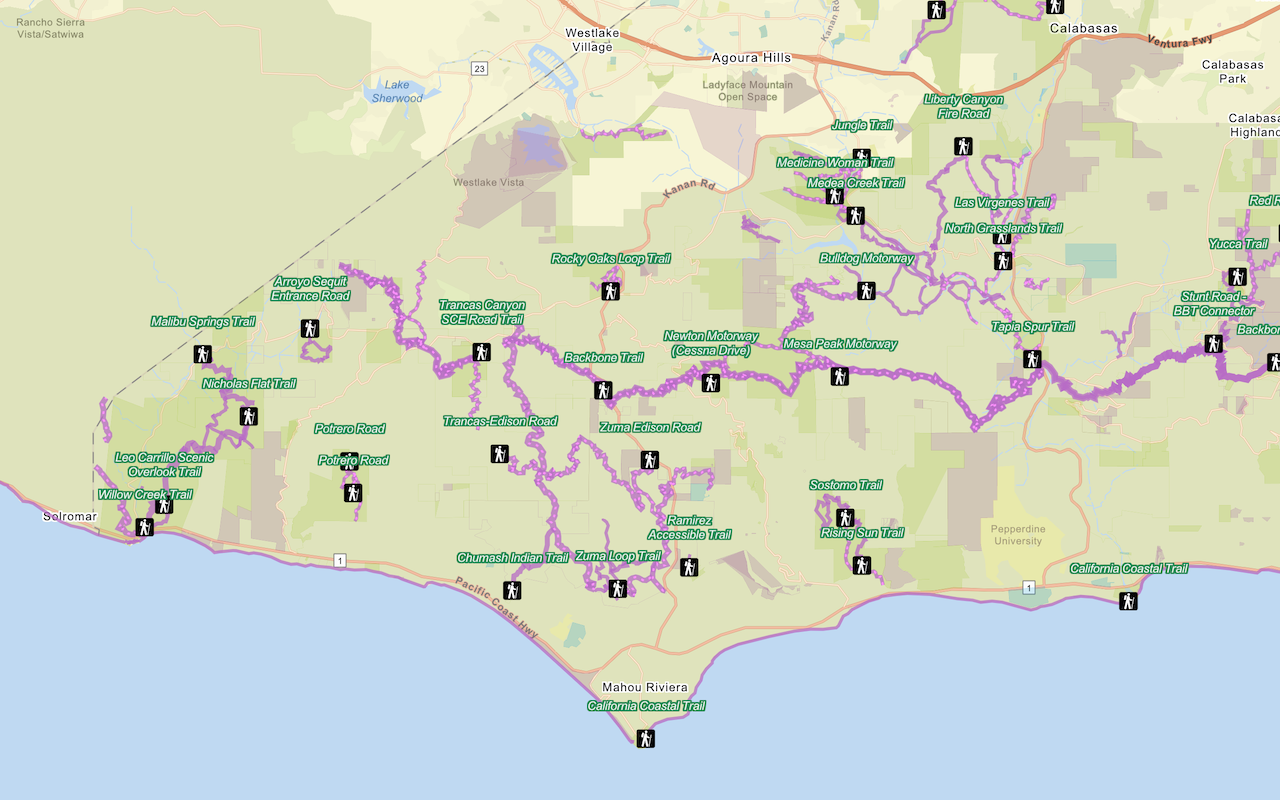
Style a feature layer
Use data-driven styling to apply symbol colors and styles to feature layers.