Learn how to perform a text-based search to find places within a bounding box.
A bounding box search finds places within an extent using the places service. An extent typically represents the visible area of a map. To perform a bounding box search, you use the places package from ArcGIS REST JS. With the results of the search, you can make another request to the service and return place attributes such as street address and telephone number.
In this tutorial, you use ArcGIS REST JS to perform a bounding box search based on the visible extent on the map and return details about each place. It includes starter code that uses Calcite components to create a basic search interface.
Prerequisites
Steps
Get the starter code
- To get started, . It contains the Calcite Components necessary to create this application, as well as a blank Leaflet map.
Set the API key
To access location services, you need an API key or OAuth 2.0 access token. To learn how to create and scope your key, visit the Create an API key tutorial.
-
Go to your dashboard to get an API key. The API key must be scoped to access the services used in this tutorial.
-
In CodePen, update
api
to use your key.Key Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const apiKey = "YOUR_API_KEY"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { apiKey: apiKey }).addTo(map);
Reference ArcGIS REST JS
-
Reference the
places
andrequest
packages from ArcGIS REST JS.Use dark colors for code blocks <!-- Load Leaflet from CDN --> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" crossorigin="" /> <script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js" crossorigin=""></script> <!-- Load Esri Leaflet from CDN --> <script src="https://unpkg.com/esri-leaflet@3.0.12/dist/esri-leaflet.js"></script> <script src="https://unpkg.com/esri-leaflet-vector@4.2.3/dist/esri-leaflet-vector.js"></script> <!-- Calcite components --> <script type="module" src="https://js.arcgis.com/calcite-components/1.0.5/calcite.esm.js"></script> <link rel="stylesheet" type="text/css" href="https://js.arcgis.com/calcite-components/1.0.5/calcite.css" /> <!-- ArcGIS REST JS: request and places --> <script src="https://unpkg.com/@esri/arcgis-rest-request@4.0.0/dist/bundled/request.umd.js"></script> <script src="https://unpkg.com/@esri/arcgis-rest-places@1.0.0/dist/bundled/places.umd.js"></script>
-
Create a REST JS
Api
using your API key.K e y Manager Use dark colors for code blocks const apiKey = "YOUR_API_KEY"; const authentication = arcgisRest.ApiKeyManager.fromKey(apiKey); const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { apiKey: apiKey }).addTo(map);
Add event listeners
The provided for this tutorial includes a basic user interface with a text input and category buttons. Add event listeners to this interface to make requests to the places service on when they are clicked.
-
Create a
show
function to make requests to the places service.Places Use dark colors for code blocks const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { apiKey: apiKey }).addTo(map); const layerGroup = L.layerGroup().addTo(map); function showPlaces(text) { };
-
Add an event listener to the search button that calls
show
on click.Places Use dark colors for code blocks const control = document.getElementById("place-control"); const input = document.getElementById("search-input") const placeKeywords = ["Restaurants", "Hotels", "Museums", "ATMs", "Breweries"]; document.getElementById("search-button").addEventListener("click", e => { showPlaces(input.value) }) placeKeywords.forEach(category => { const categoryButton = L.DomUtil.create("calcite-button","category-button"); categoryButton.setAttribute("round",true); categoryButton.setAttribute("scale","s"); categoryButton.setAttribute("kind","inverse") categoryButton.innerHTML = category; categoryButton.id = category; control.appendChild(categoryButton); })
-
Add an event listener to each category button that calls
show
on click.Places Use dark colors for code blocks placeKeywords.forEach(category => { const categoryButton = L.DomUtil.create("calcite-button","category-button"); categoryButton.setAttribute("round",true); categoryButton.setAttribute("scale","s"); categoryButton.setAttribute("kind","inverse") categoryButton.innerHTML = category; categoryButton.id = category; control.appendChild(categoryButton); categoryButton.addEventListener("click", e => { input.value = category; showPlaces(category) }) })
Find places in the map bounds
-
Calculate the current visible extent of the Leaflet map with
map.get
. Access the top right and bottom left corners.Bounds() Use dark colors for code blocks function showPlaces(text) { const bounds = map.getBounds() const topRight = bounds.getNorthEast(); const bottomLeft = bounds.getSouthWest(); };
-
Use the ArcGIS REST JS
find
operation to make a request to the places service. Set thePlaces Within Extent search
parameter to the input text and pass the current map bounding box to theText xmin
,xmax
,ymin
, andymax
parameters.Use dark colors for code blocks function showPlaces(text) { const bounds = map.getBounds() const topRight = bounds.getNorthEast(); const bottomLeft = bounds.getSouthWest(); arcgisRest.findPlacesWithinExtent({ xmin: bottomLeft.lng, ymin: bottomLeft.lat, xmax: topRight.lng, ymax: topRight.lat, searchText: text, authentication }) };
Display results
The response from the places service will contain a list of place results. Each result will include the x/y coordinates, name, category, and unique ID associated with a place.
-
Add a new
Layer
to your map to display service results. When a new places request is made, clear theGroup Layer
.Group Use dark colors for code blocks const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { apiKey: apiKey }).addTo(map); const layerGroup = L.layerGroup().addTo(map); function showPlaces(text) { layerGroup.clearLayers(); const bounds = map.getBounds() const topRight = bounds.getNorthEast(); const bottomLeft = bounds.getSouthWest(); arcgisRest.findPlacesWithinExtent({ xmin: bottomLeft.lng, ymin: bottomLeft.lat, xmax: topRight.lng, ymax: topRight.lat, searchText: text, authentication }) };
-
Access the service results. For each result, add a Leaflet
Marker
to your map.Use dark colors for code blocks function showPlaces(text) { layerGroup.clearLayers(); const bounds = map.getBounds() const topRight = bounds.getNorthEast(); const bottomLeft = bounds.getSouthWest(); arcgisRest.findPlacesWithinExtent({ xmin: bottomLeft.lng, ymin: bottomLeft.lat, xmax: topRight.lng, ymax: topRight.lat, searchText: text, authentication }) .then((response)=>{ response.results.forEach((result)=>{ const marker = L.marker([result.location.y, result.location.x]) .addTo(layerGroup); }); }); };
-
Run the app. When you click a category button or search for a phrase, the map should display a set of points representing place results.
Display a popup
To view more information about each place result, bind a popup to each marker that displays more information about the place.
-
Use
bind
to bind a popup to each result marker. Create the popup contents dynamically using a new function. The current Leaflet marker is automatically passed as the first function parameter.Popup Use dark colors for code blocks .then((response)=>{ response.results.forEach((result)=>{ const marker = L.marker([result.location.y, result.location.x]) .addTo(layerGroup); marker.bindPopup(getDetails, { minWidth:200 }); }); }); }; function getDetails(place){ const popup = document.createElement('div'); return popup };
-
Set the
id
of each marker to the uniqueplace
associated with the POI.I d Use dark colors for code blocks .then((response)=>{ response.results.forEach((result)=>{ const marker = L.marker([result.location.y, result.location.x]) .addTo(layerGroup); marker.id = result.placeId; marker.bindPopup(getDetails, { minWidth:200 }); }); }); }; function getDetails(place){ const popup = document.createElement('div'); return popup };
Get place address and phone number
You can access more information about a place using the unique place
associated with it. Perform a subsequent request to the places service to get the street address and phone number of a clicked POI.
-
Use the ArcGIS REST JS
get
function to get detailed information about a specific place. Pass thePlace Details place
associated with the current marker, and set theI d requested
parameter to return theFields street
andAddress telephone
properties.Use dark colors for code blocks function getDetails(place){ const popup = document.createElement('div'); arcgisRest.getPlaceDetails(({ placeId: place.id, authentication, requestedFields: ["name","address:streetAddress", "contactInfo:telephone"] })) return popup };
-
Access the service response. Display the results in your popup if they are available.
Use dark colors for code blocks function getDetails(place){ const popup = document.createElement('div'); arcgisRest.getPlaceDetails(({ placeId: place.id, authentication, requestedFields: ["name","address:streetAddress", "contactInfo:telephone"] })) .then((result)=>{ let popupContents = `<b>${result.placeDetails.name}</b><br>`; if (result.placeDetails.address.streetAddress) popupContents += `${result.placeDetails.address.streetAddress}<br>`; if (result.placeDetails.contactInfo.telephone) popupContents += `${result.placeDetails.contactInfo.telephone}`; popup.innerHTML = popupContents; }); return popup };
Run the app
In CodePen, run your code to display the application. The app should display a map with a search control. Upon clicking a button or entering a phrase, place results should appear on the map. Clicking a result will submit another service request to get the place address and phone number.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
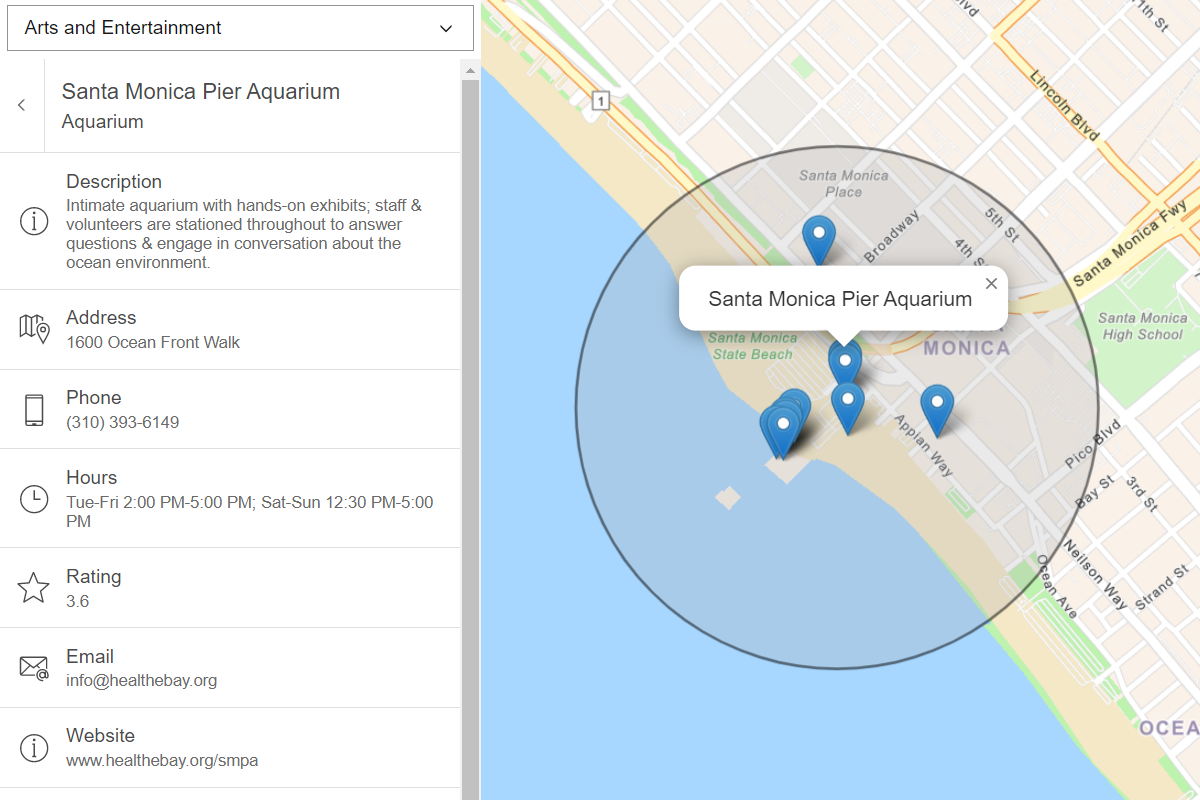
Find nearby places and details
Find points of interest near a location and get detailed information about them
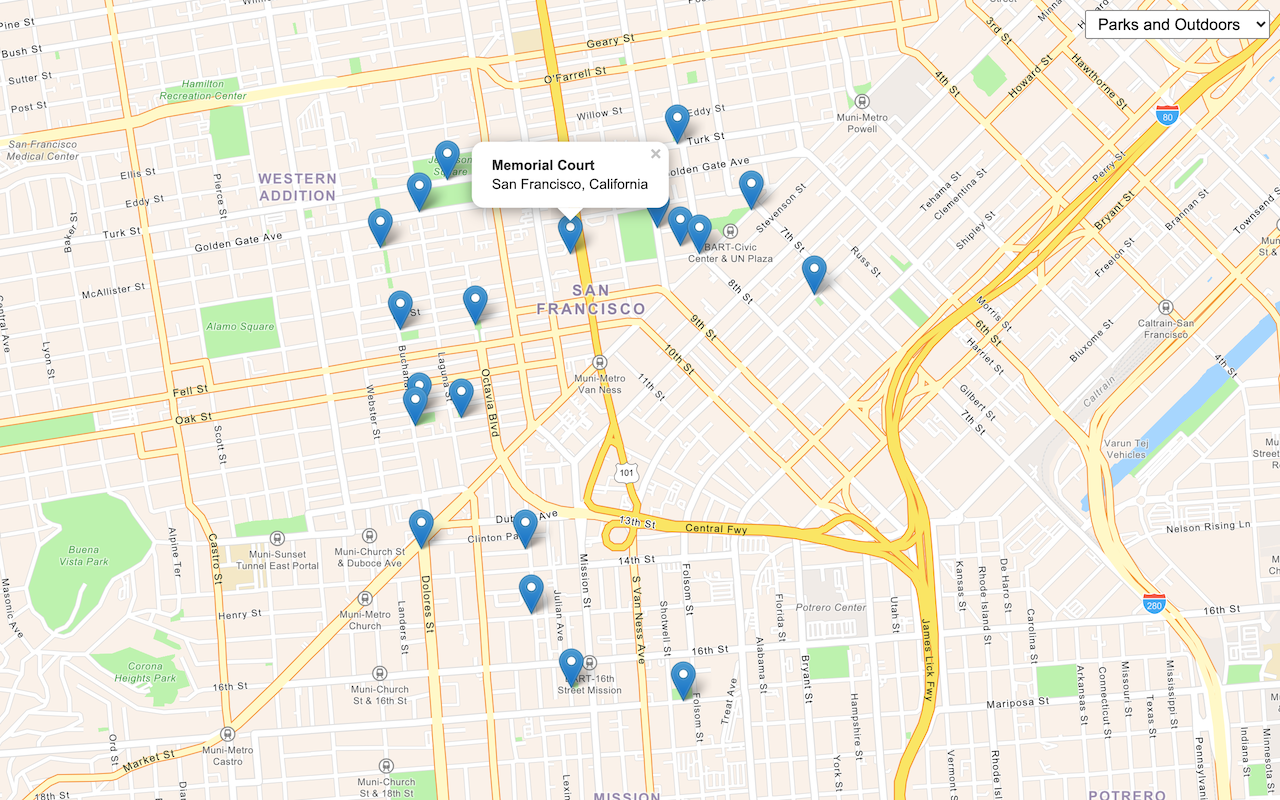
Find place addresses
Search for coffee shops, gas stations, restaurants and other nearby places with the Geocoding service.
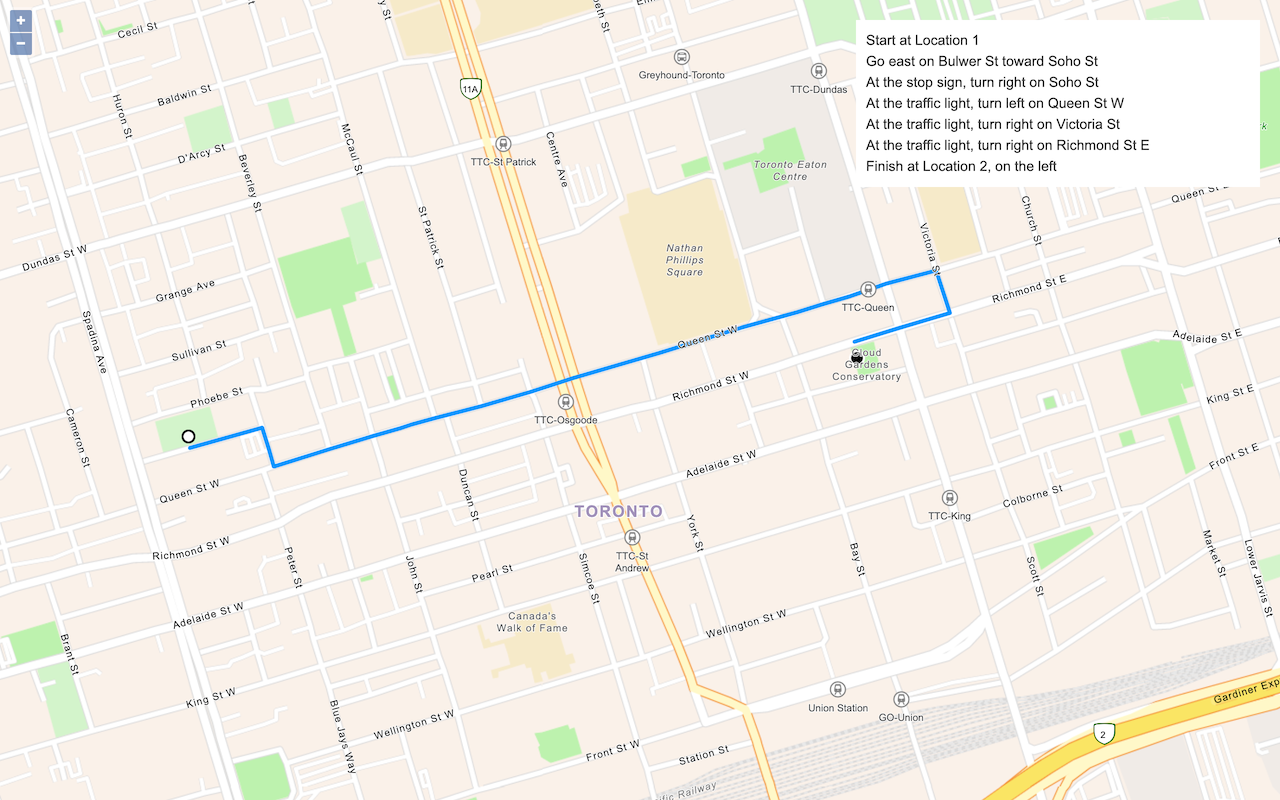
Find a route and directions
Find a route and directions with the route service.