Learn how to find an address or place using a search box and the geocoding service.
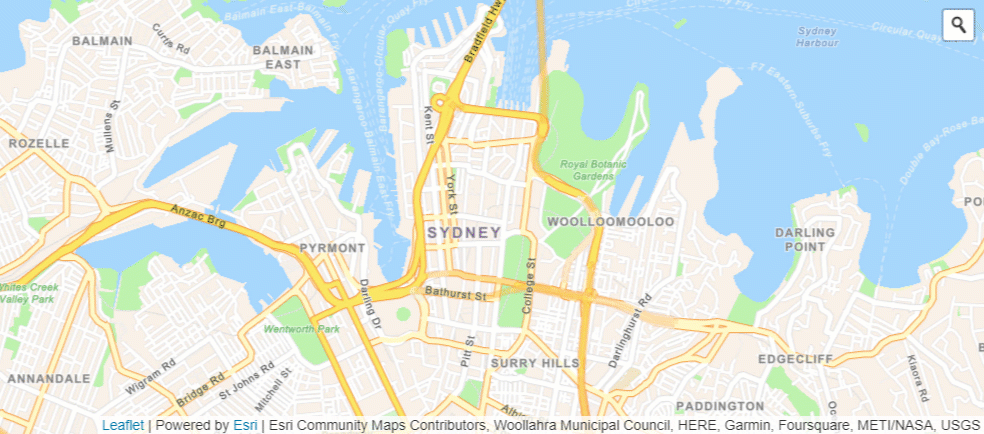
Find the location of addresses with the geocoding service.
Geocoding is the process of converting address or place text into a location. The geocoding service provides address and place geocoding as well as reverse geocoding.
Esri Leaflet provides a geocoder to access the geocoding service. In this tutorial, you use the Geosearch control to search for addresses and places.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
To learn about the other types of authentication available, go to Types of authentication.
Reference the geocoder
-
Reference the Esri Leaflet Geocoder plugin and its CSS stylesheet.
Use dark colors for code blocks <!-- Load Leaflet from CDN --> <link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" crossorigin="" /> <script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js" crossorigin=""></script> <!-- Load Esri Leaflet from CDN --> <script src="https://unpkg.com/esri-leaflet@3.0.12/dist/esri-leaflet.js"></script> <script src="https://unpkg.com/esri-leaflet-vector@4.2.3/dist/esri-leaflet-vector.js"></script> <!-- Load Esri Leaflet Geocoder from CDN --> <link rel="stylesheet" href="https://unpkg.com/esri-leaflet-geocoder@3.1.4/dist/esri-leaflet-geocoder.css"> <script src="https://unpkg.com/esri-leaflet-geocoder@3.1.4/dist/esri-leaflet-geocoder.js"></script>
Update the map
A navigation basemap layer is typically used in geocoding and routing applications. Update the basemap layer to use arcgis/navigation
.
-
Update the basemap style and change the map view to center on location
[151.2093, -33.8688]
, Sydney.Use dark colors for code blocks const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/navigation"; const map = L.map("map", { minZoom: 2 }) map.setView([-33.8688, 151.2093], 14); // Sydney L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
Add the Geosearch control
Use the Geosearch
control to access the geocoding service.
-
Add the control to the
topright
corner of the map. Createplaceholder
text and setuse
toM a p Bounds false
.Use dark colors for code blocks L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map); const searchControl = L.esri.Geocoding.geosearch({ position: "topright", placeholder: "Enter an address or place e.g. 1 York St", useMapBounds: false, }).addTo(map);
-
Set the
providers
toarcgis
in order to access the geocoding service. Set yourOnline Provider access
and theToken lat
andlng
so that the geocoder returns the geocoded results for Sydney first.Use dark colors for code blocks const searchControl = L.esri.Geocoding.geosearch({ position: "topright", placeholder: "Enter an address or place e.g. 1 York St", useMapBounds: false, providers: [ L.esri.Geocoding.arcgisOnlineProvider({ apikey: accessToken, nearby: { lat: -33.8688, lng: 151.2093 } }) ] }).addTo(map);
-
Run your app, and interact with the auto-complete enabled search control.
Display results
You can display the results
of the search using a Marker
and Popup
.
-
Add a
Layer
to the map to contain the geocoding results.Group Use dark colors for code blocks const searchControl = L.esri.Geocoding.geosearch({ position: "topright", placeholder: "Enter an address or place e.g. 1 York St", useMapBounds: false, providers: [ L.esri.Geocoding.arcgisOnlineProvider({ apikey: accessToken, nearby: { lat: -33.8688, lng: 151.2093 } }) ] }).addTo(map); const results = L.layerGroup().addTo(map);
-
Create an event handler to access the
data
from the search results. Call theclear
method to remove the previous data from theLayers Layer
.Group Use dark colors for code blocks const results = L.layerGroup().addTo(map); searchControl.on("results", (data) => { results.clearLayers(); });
-
Create a loop that adds the coordinates of a selected search results to a
Marker
.Use dark colors for code blocks const results = L.layerGroup().addTo(map); searchControl.on("results", (data) => { results.clearLayers(); for (let i = data.results.length - 1; i >= 0; i--) { const marker = L.marker(data.results[i].latlng); results.addLayer(marker); } });
-
Add a
lng
variable that stores the rounded search result coordinates. Append theL a t String bind
method to thePopup marker
to display the coordinates and address of the result.To learn more about using pop-ups with Esri Leaflet, go to the Display a pop-up tutorial.
Use dark colors for code blocks const results = L.layerGroup().addTo(map); searchControl.on("results", (data) => { results.clearLayers(); for (let i = data.results.length - 1; i >= 0; i--) { const marker = L.marker(data.results[i].latlng); const lngLatString = `${Math.round(data.results[i].latlng.lng * 100000) / 100000}, ${ Math.round(data.results[i].latlng.lat * 100000) / 100000 }`; marker.bindPopup(`<b>${lngLatString}</b><p>${data.results[i].properties.LongLabel}</p>`); results.addLayer(marker); marker.openPopup(); } });
Run the app
In CodePen, run your code to display the map.
Click on the geocoder control and enter an address or place such as "Starbucks". When you select a result, the map will zoom to its location.
What's next?
Learn how to use additional ArcGIS location services in these tutorials: