Learn how to display feature attributes in a pop-up.
A pop-up, also known as a "popup", is a visual element that displays information about a feature when it is clicked. You typically style and configure a pop-up using HTML and CSS for each layer in a map. Pop-ups can display attribute values, calculated values, or rich content such as images, charts, or videos.
In this tutorial, you create an interactive pop-up for the Trailheads feature layer in the Santa Monica Mountains. When a feature is clicked, a pop-up is displayed containing the name of the trail and the service that manages it.
Prerequisites
You need an ArcGIS Location Platform or ArcGIS Online account.
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the resources used in this tutorial.
-
Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Item access
- Note: If you are using your own custom data layer for this tutorial, you need to grant the API key credentials access to the layer item. Learn more in Item access privileges.
- Privileges
-
Copy the API key access token to your clipboard when prompted.
-
In CodePen, update the
access
variable to use your access token.Token Use dark colors for code blocks const map = L.map("map", { minZoom: 2 }) map.setView([34.02, -118.805], 13); const accessToken = "YOUR_ACCESS_TOKEN"; const basemapEnum = "arcgis/streets"; L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map);
To learn about the other types of authentication available, go to Types of authentication.
Add the trailheads layer
Use the Feature
class to display the Trailheads layer on your map.
For more information about adding feature layers, go to the Add a feature layer tutorial.
-
Create a
Feature
and set theLayer url
property to access the feature service.Use dark colors for code blocks L.esri.Vector.vectorBasemapLayer(basemapEnum, { token: accessToken }).addTo(map); var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" }) .addTo(map);
Display a pop-up
Use the bind
method to display the attribute information of the trailheads layer.
-
Append the
bind
method to thePopup trailheads
layer and pass in afunction
to return the attribute information of the layer.Use dark colors for code blocks var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" }) .addTo(map); trailheads.bindPopup(function (layer) { });
-
Set the pop-up contents to display information about the trail and park name.
Use dark colors for code blocks var trailheads = L.esri .featureLayer({ url: "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0" }) .addTo(map); trailheads.bindPopup(function (layer) { return L.Util.template("<b>{TRL_NAME}</b><br>{PARK_NAME}</br>", layer.feature.properties); });
Run the app
In CodePen, run your code to display the map.
The map view should display the Trailheads feature layer. When you click a feature, the name of the trailhead and its park name is displayed in a pop-up.
What's next?
Learn how to use additional ArcGIS location services in these tutorials:
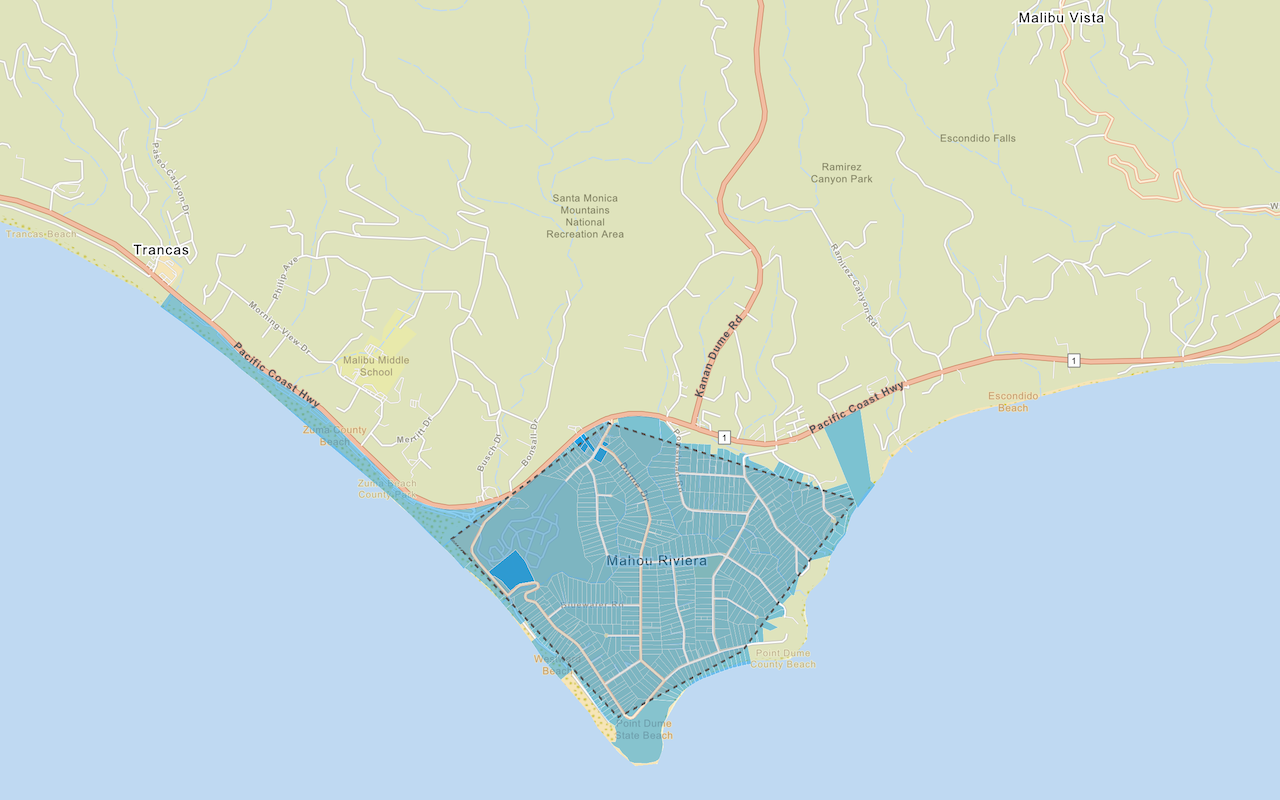
Query a feature layer (spatial)
Execute a spatial query to access polygon features from a feature service.
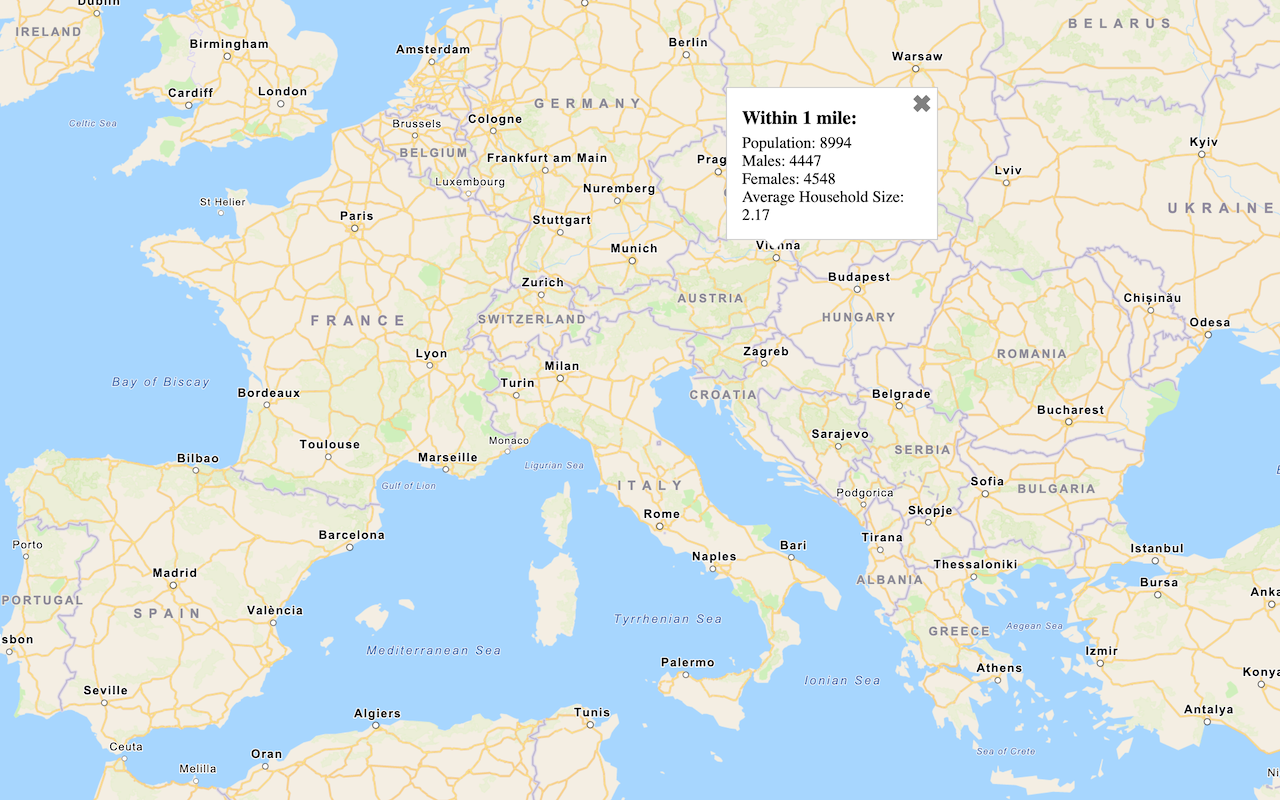
Get global data
Query demographic information for locations around the world with the GeoEnrichment service.
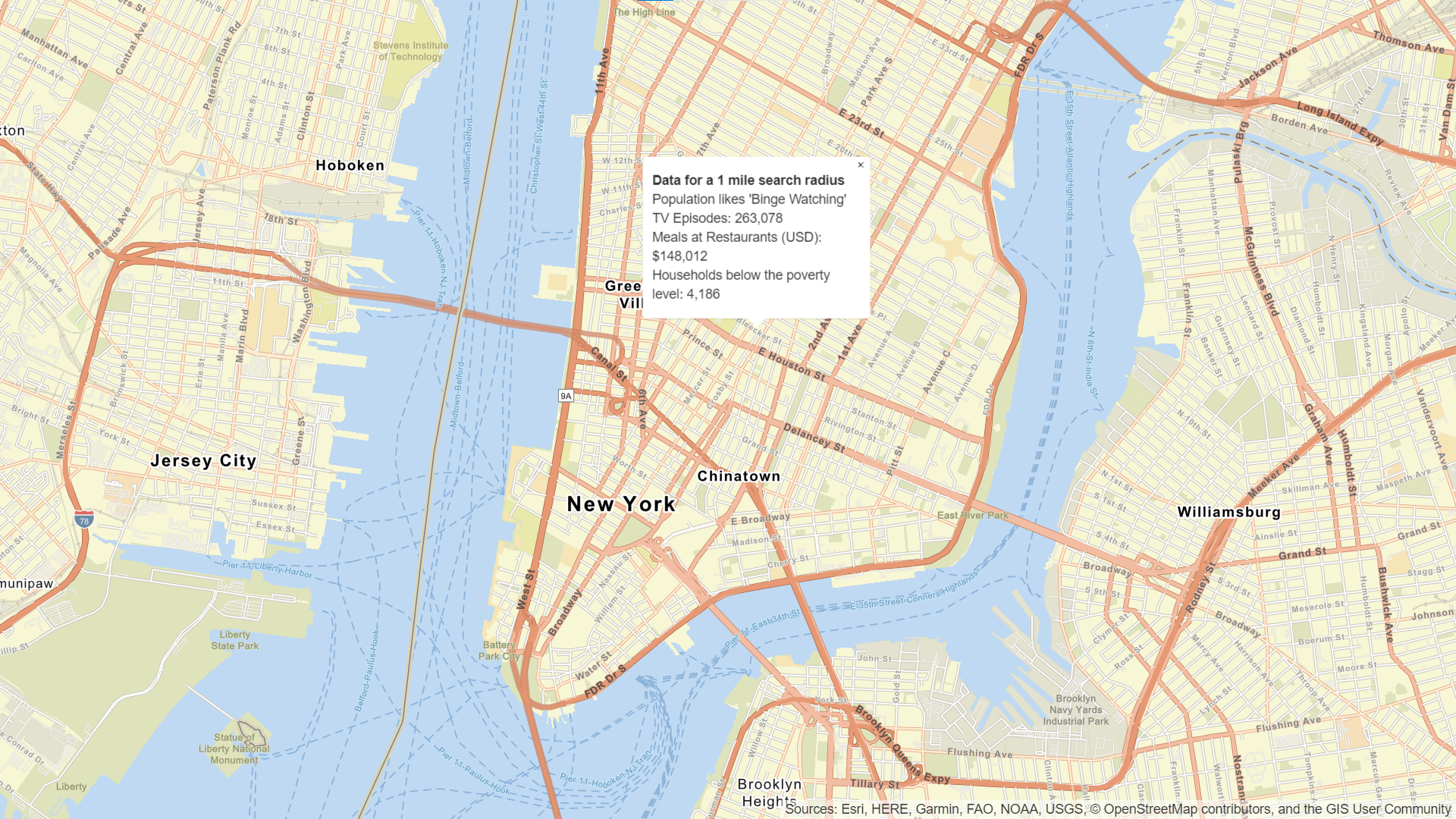
Get local data
Query regional facts, spending trends, and psychographics with the GeoEnrichment service.
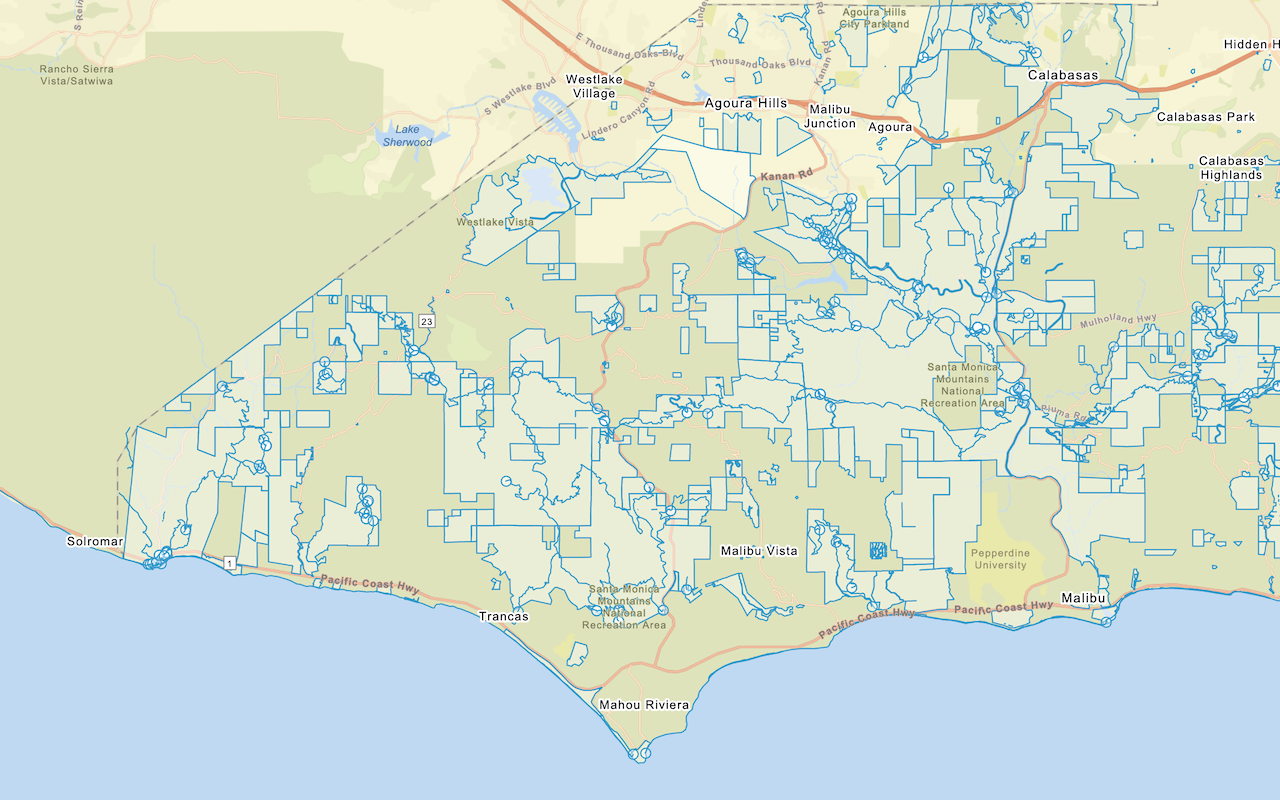
Add a feature layer
Add features from feature layers to a map.