TRK_EntryExitPoints takes a track column and a geometry column and returns an array of struct representing the points at which the track enters and exits the geometry. The geometry type can be linestring or polygon.
Each element of the array contains two structs:
entry
—This struct contains information about where the track enters the geometry. It includes the following fields:point
—The geometry of the entry point.time
—The timestamp of the entry point formatted asH
.H- M M- S S hh :mm :ss.s track
—A boolean value._endpoint True
if the entry point is the starting or ending point of the track.
exit
—This struct contains information about where the track exits the geometry. It includes the following fields:point
—The geometry of the exit point.time
—The timestamp of the exit point formatted asH
.H- M M- S S hh :mm :ss.s track
—A boolean value._endpoint True
if the exit point is the starting or ending point of the track.
If the track crosses the geometry multiple times, each entry and exit point pair is included as a separate element in the array.
If the track and geometry columns are in different spatial references, the function automatically transforms the geometry into the spatial reference of the track. The spatial reference of the point geometry in the output would be the same as the track.
Tracks are linestrings that represent the change in an entity's location over time. Each vertex in the linestring has a timestamp (stored as the M-value) and the vertices are ordered sequentially.
For more information on using tracks in GeoAnalytics Engine, see the core concept topic on tracks.
Function | Syntax |
---|---|
Python | entry |
SQL | TRK |
Scala | entry |
For more details, go to the GeoAnalytics Engine API reference for entry_exit_points.
Python and SQL Examples
from geoanalytics.sql import functions as ST
from geoanalytics.tracks import functions as TRK
from pyspark.sql import functions as F
data = [(1, "LINESTRING M (-117.27 34.05 1633455010, -117.22 33.91 1633456062, -116.96 33.64 1633457132)",
"POLYGON((-117.22 33.91, -117.22 33.75, -117.05 33.91, -117.22 33.91))"),
(2, "LINESTRING M (-116.89 33.96 1633575895, -116.71 34.01 1633576982, -116.66 34.08 1633577061)",
"POLYGON((-116.80 34.05, -116.62 34.05, -116.72 33.98, -116.80 33.98, -116.80 34.05))"),
(3, "LINESTRING M (-116.24 33.88 1633575234, -116.33 34.02 1633576336)",
"POLYGON((-116.38 34.05, -116.20 34.05, -116.20 33.85, -116.38 33.85,-116.38 34.05))"),]
df = spark.createDataFrame(data, ["id", "trk_wkt", "poly_wkt"]) \
.withColumn("track", ST.line_from_text("trk_wkt", 4326)) \
.withColumn("polygon", ST.poly_from_text("poly_wkt", 4326))
result = df.select("id", F.explode(TRK.entry_exit_points("track", "polygon")).alias("entry_exit_points"))
ax = df.st.plot("track", edgecolor="lightgrey", linewidths=10, zorder=0, figsize=(15, 8))
df.st.plot("polygon", ax=ax, facecolor='lightblue', edgecolor="lightblue", alpha=0.5)
result.st.plot("entry_exit_points.entry.point", ax=ax, s=75, facecolor="green", zorder=2)
result.st.plot("entry_exit_points.exit.point", ax=ax, s=75, facecolor="red", zorder=2)
ax.legend(['Input track', 'Entry point','Exit point'], loc='lower right', fontsize='x-large')
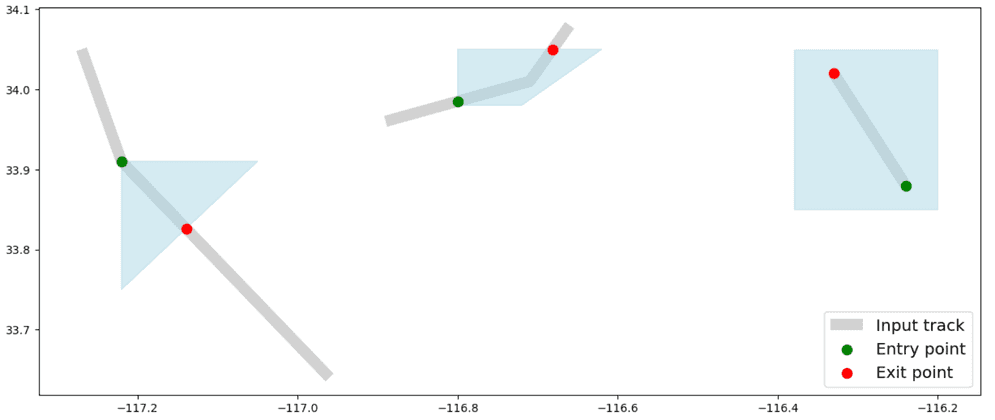
Scala Example
import com.esri.geoanalytics.sql.{functions => ST}
import com.esri.geoanalytics.sql.{trackFunctions => TRK}
import org.apache.spark.sql.{functions => F}
case class WKTRow(id: Int, lineWkt: String, polyWkt: String)
val data = Seq(WKTRow(1, "LINESTRING M (-117.27 34.05 1633455010, -117.22 33.91 1633456062, -116.96 33.64 1633457132)",
"POLYGON((-117.22 33.91, -117.22 33.75, -117.05 33.91, -117.22 33.91))"),
WKTRow(2, "LINESTRING M (-116.89 33.96 1633575895, -116.71 34.01 1633576982, -116.66 34.08 1633577061)",
"POLYGON((-116.80 34.05, -116.62 34.05, -116.72 33.98, -116.80 33.98, -116.80 34.05))"),
WKTRow(3, "LINESTRING M (-116.24 33.88 1633575234, -116.33 34.02 1633576336)",
"POLYGON((-116.38 34.05, -116.20 34.05, -116.20 33.85, -116.38 33.85,-116.38 34.05))"))
val df = spark.createDataFrame(data)
.withColumn("track", ST.lineFromText($"lineWkt", F.lit(4326)))
.withColumn("polygon", ST.polyFromText($"polyWkt", F.lit(4326)))
.withColumn("entry_exit_points", TRK.entryExitPoints($"track", $"polygon"))
.select("id", "entry_exit_points")
df.show(truncate = false)
df.printSchema()
+---+--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------+
|id |entry_exit_points |
+---+--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------+
|1 |[{{{"x":-117.22,"y":33.91,"m":1.633456062e9}, 2021-10-05 10:47:42, false}, {{"x":-117.13917714285714,"y":33.82606857142857,"m":1.633456394617143e9}, 2021-10-05 10:53:14.617142, false}}] |
|2 |[{{{"x":-116.80000000001343,"y":33.98499999999254,"m":1.6335764384999998e9}, 2021-10-06 20:13:58.499999, false}, {{"x":-116.68142857140938,"y":34.050000000013426,"m":1.633577027142857e9}, 2021-10-06 20:23:47.142857, false}}]|
|3 |[{{{"x":-116.24,"y":33.88,"m":1.633575234e9}, 2021-10-06 19:53:54, true}, {{"x":-116.33,"y":34.02,"m":1.633576336e9}, 2021-10-06 20:12:16, true}}] |
+---+--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------+
root
|-- id: integer (nullable = false)
|-- entry_exit_points: array (nullable = true)
| |-- element: struct (containsNull = true)
| | |-- entry: struct (nullable = false)
| | | |-- point: point (nullable = false)
| | | |-- time: timestamp (nullable = false)
| | | |-- track_endpoint: boolean (nullable = false)
| | |-- exit: struct (nullable = false)
| | | |-- point: point (nullable = false)
| | | |-- time: timestamp (nullable = false)
| | | |-- track_endpoint: boolean (nullable = false)
Version table
Release | Notes |
---|---|
1.5.0 | Python, SQL, and Scala functions introduced |