Learn how to create and display a map from a web map stored in ArcGIS.
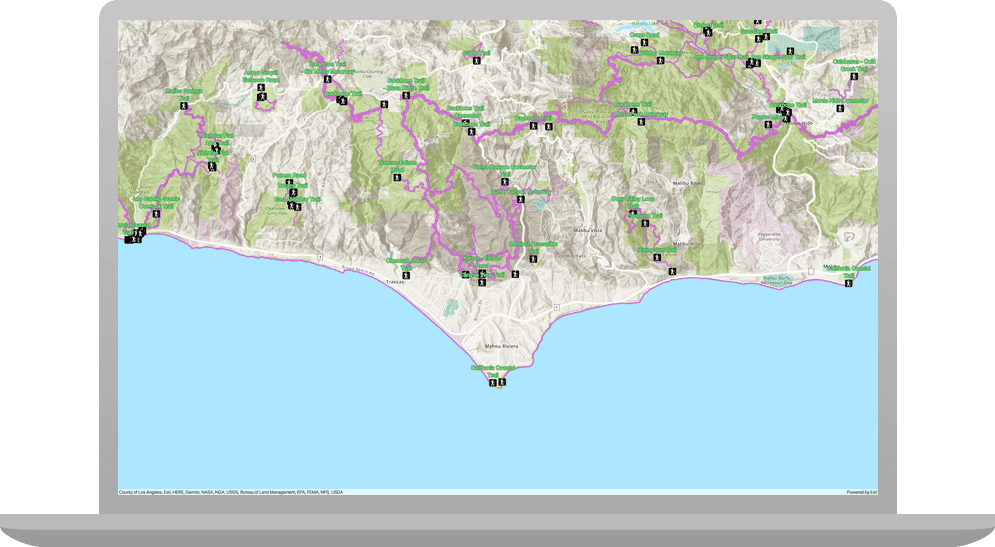
A web map contains the definition of a map, including properties such as the basemap, initial extent, layers, styles, pop-ups, and labels. You can author and save web maps using the Map Viewer or ArcGIS Pro and share them in ArcGIS. Each web map is stored as an item in ArcGIS Online or ArcGIS Enterprise, allowing you to access a web map from a portal using its item ID and display it in your app.
In this tutorial, you use a web map's item ID to display a map of trails, trailheads and parks in the Santa Monica Mountains . The web map is hosted in ArcGIS Online.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Confirm that your system meets the minimum system requirements.
-
An IDE for Java.
Steps
Open a Java project with Gradle
-
To start this tutorial, complete the Display a map tutorial, or download and unzip the Display a map solution into a new folder.
-
Open the build.gradle file as a project in IntelliJ IDEA.
-
If you downloaded the solution, get an access token and set the API key.
An API key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token using your ArcGIS Location Platform or ArcGIS Online account. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In IntelliJ IDEA's Project tool window, open src/main/java/com.example.app and double-click App.
-
In the
start()
method, set the API key property on theArcGISRuntimeEnvironment
with your access token. Replace YOUR_ACCESS_TOKEN with your copied access token. Be sure to surround your access token with double quotes as it is a string.App.javaUse dark colors for code blocks ArcGISRuntimeEnvironment.setApiKey("YOUR_ACCESS_TOKEN");
-
Prepare files before coding the app
Modify the files from the Display a map
tutorial so that they can be used in this tutorial: you will add and remove imports, change the application title, and remove unnecessary code.
-
In IntelliJ IDEA's Project tool window, open src/main/java/com.example.app and double-click App.
-
Add the following imports above the existing imports:
App.javaUse dark colors for code blocks 18 19 20 21Add line. Add line. import com.esri.arcgisruntime.portal.Portal; import com.esri.arcgisruntime.portal.PortalItem; import com.esri.arcgisruntime.ArcGISRuntimeEnvironment; import com.esri.arcgisruntime.mapping.ArcGISMap; import com.esri.arcgisruntime.mapping.view.MapView;
-
Remove the following imports:
App.javaUse dark colors for code blocks Remove line Remove line import com.esri.arcgisruntime.mapping.BasemapStyle; import com.esri.arcgisruntime.mapping.Viewpoint;
-
In the
start()
life-cycle method, change the title that will appear on the application window toDisplay a web map tutorial
.App.javaUse dark colors for code blocks @Override public void start(Stage stage) { // set the title and size of the stage and show it stage.setTitle("Display a web map tutorial"); stage.setWidth(800); stage.setHeight(700); stage.show();
-
In
start()
method, delete the code for creating a map from a basemap and setting a viewpoint. The web map defines the characteristics of the map, so you don't have to set them in your app.App.javaUse dark colors for code blocks ArcGISMap map = new ArcGISMap(BasemapStyle.ARCGIS_TOPOGRAPHIC); // set the map on the map view mapView.setMap(map); mapView.setViewpoint(new Viewpoint(34.02700, -118.80543, 144447.638572));
Get the web map item ID
You can use ArcGIS tools to create and view web maps. Use the Map Viewer to identify the web map item ID. This item ID will be used later in the tutorial.
- Go to the LA Trails and Parks web map in the Map Viewer in ArcGIS Online. This web map displays trails, trailheads and parks in the Santa Monica Mountains.
- Make a note of the item ID at the end of the browser's URL. The item ID should be
41281c51f9de45edaf1c8ed44bb10e30
Display the web map
You can create a map from a web map using the web map's item ID. Use the PortalItem
class to access the web map, and the ArcGISMap
class to create and display a map from it.
-
In the
start()
method, create a newPortal(java.lang.String,boolean)
referencing ArcGIS Online as theportal
parameter andUrl false
for thelogin
parameter.Required App.javaUse dark colors for code blocks 54 55 56 57 59Add line. // create a map view to display the map and add it to the stack pane mapView = new MapView(); stackPane.getChildren().add(mapView); Portal portal = new Portal("https://www.arcgis.com", false);
-
Create a
PortalItem
for the web map, by passing theportal
and the web map's item ID as parameters. -
Create an
ArcGISMap(com.esri.arcgisruntime.portal.PortalItem)
using theportal
as the constructor parameter.Item App.javaUse dark colors for code blocks 58 59Add line. Add line. Add line. Portal portal = new Portal("https://www.arcgis.com", false); String itemId = "41281c51f9de45edaf1c8ed44bb10e30"; PortalItem portalItem = new PortalItem(portal, itemId); ArcGISMap map = new ArcGISMap(portalItem);
-
Use the existing code to display the new
ArcGISMap
on themap
withView MapView.setMap()
.App.javaUse dark colors for code blocks Copy 54 55 56 57 58 59 60 61 62 63// create a map view to display the map and add it to the stack pane mapView = new MapView(); stackPane.getChildren().add(mapView); Portal portal = new Portal("https://www.arcgis.com", false); String itemId = "41281c51f9de45edaf1c8ed44bb10e30"; PortalItem portalItem = new PortalItem(portal, itemId); ArcGISMap map = new ArcGISMap(portalItem); mapView.setMap(map);
-
Run the app. Ensure to run the app as a Gradle task and not as an application in your IDE. In the Gradle tool window, under Tasks > application, double-click run.
You should see a map of trails, trailheads and parks in the Santa Monica Mountains . Click, drag, and scroll the mouse wheel on the map view to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: