Learn how to create and display a map from a web map stored in ArcGIS.
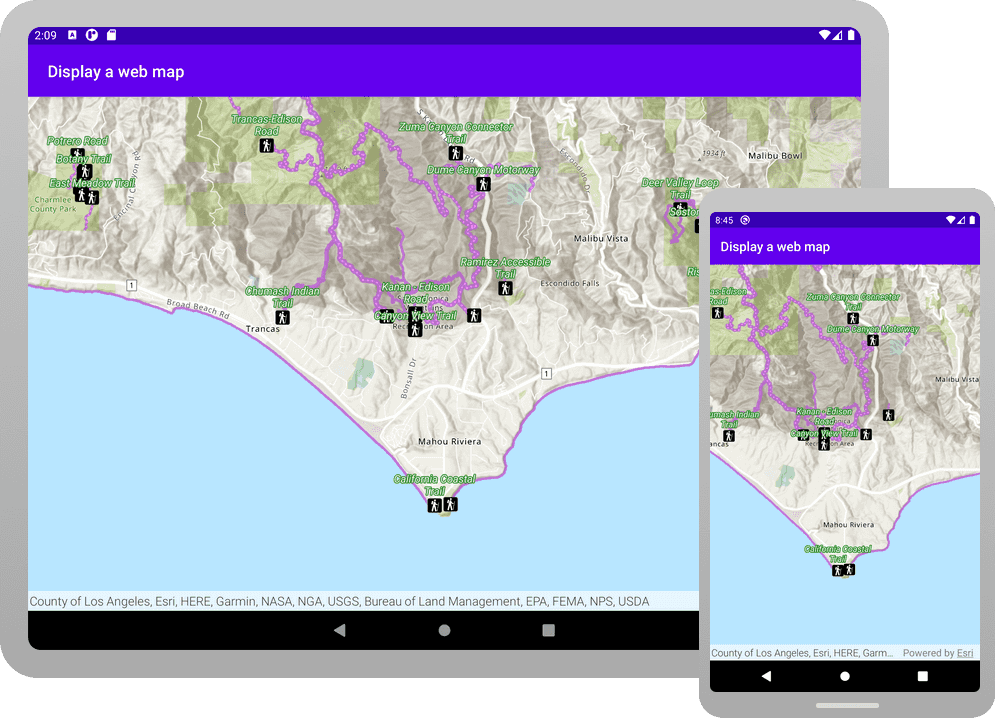
A web map contains the definition of a map, including properties such as the basemap, initial extent, layers, styles, pop-ups, and labels. You can author and save web maps using the Map Viewer or ArcGIS Pro and share them in ArcGIS. Each web map is stored as an item in ArcGIS Online or ArcGIS Enterprise, allowing you to access a web map from a portal using its item ID and display it in your app.
In this tutorial, you use a web map's item ID to display a map of trails, trailheads and parks in the Santa Monica Mountains . The web map is hosted in ArcGIS Online.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
A development and deployment environment that meets the system requirements.
-
An IDE for Android development in Kotlin.
Steps
Open an Android Studio project
-
To start this tutorial, complete the Display a map tutorial. Or download and unzip the Display a map solution in a new folder.
-
Modify the old project for use in this new tutorial. Expand More info for instructions.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android tool window, open app > res > values > strings.xml.
In the
<string name="app
element, change the text content to Display a web map._name" > strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Display a web map</string> </resources>
-
In the Android tool window, open Gradle Scripts > settings.gradle.kts.
Change the value of
root
to "Display a web map".Project.name settings.gradle.ktsUse dark colors for code blocks dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url = uri("https://esri.jfrog.io/artifactory/arcgis") } } } rootProject.name = "Display a web map" include(":app")
-
The UI theme composable in Display a map tutorial was
Display
. Rename the theme composable throughout the tutorial by refactoringA Map Theme Display
.A Map Theme In the Android tool window, open app > kotlin+java > com.exmple.app > ui.theme > Theme.kt.
Right-click the function name
Display
and select Refactor -> Rename. Replace the name withA Map Theme Display
.A Web Map Theme Theme.ktUse dark colors for code blocks Copy @Composable fun DisplayAMapTheme( darkTheme: Boolean = isSystemInDarkTheme(), // Dynamic color is available on Android 12+ dynamicColor: Boolean = true, content: @Composable () -> Unit ) { val colorScheme = when { dynamicColor && Build.VERSION.SDK_INT >= Build.VERSION_CODES.S -> { val context = LocalContext.current if (darkTheme) dynamicDarkColorScheme(context) else dynamicLightColorScheme(context) } darkTheme -> DarkColorScheme else -> LightColorScheme }
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
If you downloaded the solution, get an access token and set the API key in MainActivity.kt.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Android Studio: in the Android tool window, open app > java > com.example.app > MainActivity.
-
In the
set
function, find theApi Key() ApiKey.create()
call and paste your copied access token inside the double quotes, replacing YOUR_ACCESS_TOKEN.MainActivity.ktUse dark colors for code blocks Copy private fun setApiKey() { ArcGISEnvironment.apiKey = ApiKey.create("YOUR_ACCESS_TOKEN") }
-
Get the web map item ID
You can use ArcGIS tools to create and view web maps. Use the Map Viewer to identify the web map item ID. This item ID will be used later in the tutorial.
- Go to the LA Trails and Parks web map in the Map Viewer in ArcGIS Online. This web map displays trails, trailheads and parks in the Santa Monica Mountains.
- Make a note of the item ID at the end of the browser's URL. The item ID should be
41281c51f9de45edaf1c8ed44bb10e30
.
Add import statements
Replace the import statements in MainScreen.kt with the imports needed for this tutorial.
@file:OptIn(ExperimentalMaterial3Api::class)
package com.example.app.screens
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.runtime.Composable
import androidx.compose.runtime.remember
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.stringResource
import com.arcgismaps.mapping.ArcGISMap
import com.arcgismaps.mapping.PortalItem
import com.arcgismaps.portal.Portal
import com.arcgismaps.toolkit.geoviewcompose.MapView
import com.example.app.R
Display the web map
You can create a map from a web map using the web map's item ID. Use the PortalItem
class to access the web map, and the ArcGISMap
class to create and display a map from it.
-
Open the app > kotlin+java > com.example.app > MainActivity.kt. Delete the code inside
create
. These lines come from the Display a map tutorial and do not apply here.Map() MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { return ArcGISMap(BasemapStyle.ArcGISTopographic).apply { initialViewpoint = Viewpoint( latitude = 34.0270, longitude = -118.8050, scale = 72000.0 ) } }
-
Inside the empty
create
, create a newMap() Portal
referencing ArcGIS Online as theurl
parameter andPortal.
for theConnection. Anonymous connection
parameter.MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { val portal = Portal( url = "https://www.arcgis.com", connection = Portal.Connection.Anonymous ) }
-
Next, create a
PortalItem
for the web map, by passing theportal
and the web map's item ID as parameters.MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { val portal = Portal( url = "https://www.arcgis.com", connection = Portal.Connection.Anonymous ) val portalItem = PortalItem( portal = portal, itemId = "41281c51f9de45edaf1c8ed44bb10e30" ) }
-
Last, create an
ArcGISMap
usingportal
as the constructor parameter.Item MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { val portal = Portal( url = "https://www.arcgis.com", connection = Portal.Connection.Anonymous ) val portalItem = PortalItem( portal = portal, itemId = "41281c51f9de45edaf1c8ed44bb10e30" ) return ArcGISMap(portalItem) }
-
Click Run > Run > app to run the app.
You should see a map of trails, trailheads and parks in the Santa Monica Mountains . Drag, swipe, or pinch on the map view to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: