Learn how to download and display an offline map for a user-defined geographical area of a web map.
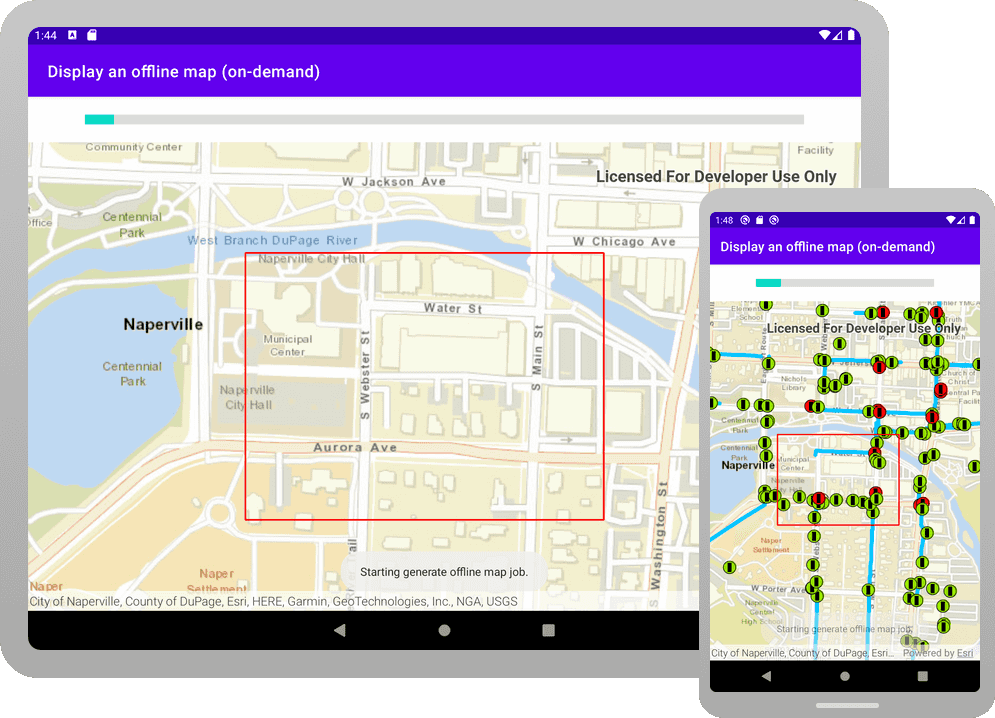
Offline maps allow users to continue working when network connectivity is poor or lost. If a web map is enabled for offline use, a user can request that ArcGIS generates an offline map for a specified geographic area of interest.
In this tutorial, you will download an offline map for an area of interest from the web map of the stormwater network within Naperville, IL, USA . You can then use this offline map without a network connection.
The following are required for this tutorial:
- An ArcGIS account to access API keys. If you don't have an account, sign up for free.
- A development and deployment environment that meets the system requirements.
- An IDE for Android development in Kotlin.
Steps
Open an Android Studio project
-
To start this tutorial, complete the Display a map tutorial. Or download and unzip the Display a map solution in a new folder.
-
Modify the old project for use in this new tutorial. Expand More info for instructions.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android tool window, open app > res > values > strings.xml.
In the
<string name="app_
element, change the text content to Display an offline map (on-demand).name"> strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Display an offline map (on-demand)</string> </resources>
-
In the Android tool window, open Gradle Scripts > settings.gradle.kts.
Change the value of
root
to "Display an offline map (on-demand)".Project.name settings.gradle.ktsUse dark colors for code blocks dependencyResolutionManagement { @Suppress("UnstableApiUsage") repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) @Suppress("UnstableApiUsage") repositories { google() mavenCentral() maven { url = uri("https://esri.jfrog.io/artifactory/arcgis") } } } rootProject.name = "Display an offline map (on-demand)" include(":app")
-
The UI theme composable in Display a map tutorial was
Display
. Rename the theme composable throughout the tutorial by refactoringAMap Theme Display
.AMap Theme In the Android tool window, open app > kotlin+java > com.exmple.app > ui.theme > Theme.kt.
Right-click the function name
Display
and select Refactor -> Rename. Replace the name withAMap Theme Display
.An Offline M a p On Demand Theme Theme.ktUse dark colors for code blocks Copy @Composable fun DisplayAMapTheme( darkTheme: Boolean = isSystemInDarkTheme(), // Dynamic color is available on Android 12+ dynamicColor: Boolean = true, content: @Composable () -> Unit ) { val colorScheme = when { dynamicColor && Build.VERSION.SDK_INT >= Build.VERSION_CODES.S -> { val context = LocalContext.current if (darkTheme) dynamicDarkColorScheme(context) else dynamicLightColorScheme(context) } darkTheme -> DarkColorScheme else -> LightColorScheme }
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
Set your API key in MainActivity.kt.
An API Key enables access to services, web maps, and web scenes hosted in ArcGIS Online.
-
In Android Studio: in the Android tool window, open app > java > com.example.app > MainActivity.
-
In the
set
function, find theA p i Key() ApiKey.create()
call and paste your API key inside the quotes, replacing YOUR_API_KEY.MainActivity.ktUse dark colors for code blocks Copy private fun setApiKey() { ArcGISEnvironment.apiKey = ApiKey.create("YOUR_API_KEY") }
-
Add import statements and some Compose variables
-
In the Android tool window, open app > kotlin+java > com.example.app > screens > MainScreen.kt. Replace the import statements with the imports needed for this tutorial.
MainScreen.ktUse dark colors for code blocks @file:OptIn(ExperimentalMaterial3Api::class) package com.example.app.screens import android.content.Context import android.widget.Toast import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxSize import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.foundation.layout.padding import androidx.compose.material3.ExperimentalMaterial3Api import androidx.compose.material3.LinearProgressIndicator import androidx.compose.material3.Scaffold import androidx.compose.material3.Text import androidx.compose.material3.TopAppBar import androidx.compose.runtime.Composable import androidx.compose.runtime.LaunchedEffect import androidx.compose.runtime.mutableIntStateOf import androidx.compose.runtime.mutableStateOf import androidx.compose.runtime.remember import androidx.compose.runtime.rememberCoroutineScope import androidx.compose.ui.Modifier import androidx.compose.ui.platform.LocalContext import androidx.compose.ui.res.stringResource import com.arcgismaps.Color import com.arcgismaps.geometry.Envelope import com.arcgismaps.geometry.SpatialReference import com.arcgismaps.mapping.ArcGISMap import com.arcgismaps.mapping.PortalItem import com.arcgismaps.mapping.symbology.SimpleFillSymbol import com.arcgismaps.mapping.symbology.SimpleFillSymbolStyle import com.arcgismaps.mapping.symbology.SimpleLineSymbol import com.arcgismaps.mapping.symbology.SimpleLineSymbolStyle import com.arcgismaps.mapping.view.Graphic import com.arcgismaps.mapping.view.GraphicsOverlay import com.arcgismaps.portal.Portal import com.arcgismaps.tasks.offlinemaptask.GenerateOfflineMapParameters import com.arcgismaps.tasks.offlinemaptask.OfflineMapTask import com.arcgismaps.toolkit.geoviewcompose.MapView import com.example.app.R import kotlinx.coroutines.CoroutineScope import kotlinx.coroutines.launch import java.util.Calendar
-
In the
Main
composable, create two variables:Screen context
to hold the local context of your app andcoroutine
to which you assignScope remember
.Coroutine Scope() MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { val context = LocalContext.current val coroutineScope = rememberCoroutineScope() var map = remember { createMap() } Scaffold( topBar = { TopAppBar(title = { Text(text = stringResource(id = R.string.app_name)) }) } ) { MapView( modifier = Modifier.fillMaxSize(), arcGISMap = map, ) } }
Get the web map item ID
You can use ArcGIS tools to create and view web maps. Use the Map Viewer to identify the web map item ID. This item ID will be used later in the tutorial.
- Go to the Naperville water network in the Map Viewer in ArcGIS Online. This web map displays stormwater network within Naperville, IL, USA .
- Make a note of the item ID at the end of the browser's URL. The item ID should be:
acc027394bc84c2fb04d1ed317aac674
Define a progress bar
-
In
Main
, add two variables used in displaying a progress bar.Screen Add a
remember
variable namedcurrent
. Inside theProgress remember
, callmutable
. The remembered value is an integer indicating the percent completion of an ongoing operation.I n t State Of(0) Then add a
remember
variable namedshow
. Inside theProgress Bar remember
block, callmutable
. The remembered value is Boolean indicating whether the progress bar is currently visible or not.State Of(false) MainActivity.ktUse dark colors for code blocks @Composable fun MainScreen() { val context = LocalContext.current val coroutineScope = rememberCoroutineScope() val currentProgress = remember { mutableIntStateOf(0) } val showProgressBar = remember { mutableStateOf(false) } var map = remember { createMap() } Scaffold( topBar = { TopAppBar(title = { Text(text = stringResource(id = R.string.app_name)) }) } ) { MapView( modifier = Modifier.fillMaxSize(), arcGISMap = map, ) } }
-
Inside the
Scaffold
block, find theMapView
from the Display a map tutorial and replace it with a call of theColumn
composable.A
Column
allows you to display the progress bar at the top of the screen and the map view below it. Inside theColumn
block, add aLinear
and then add back theProgress Indicator MapView
code.MainActivity.ktUse dark colors for code blocks Scaffold( topBar = { TopAppBar(title = { Text(text = stringResource(id = R.string.app_name)) }) } ) { Column( modifier = Modifier.fillMaxSize().padding(it) ) { if (showProgressBar.value) { LinearProgressIndicator( modifier = Modifier.fillMaxWidth(), progress = currentProgress.intValue / 100f ) } MapView( modifier = Modifier.fillMaxSize(), arcGISMap = map, ) } }
Display the web map
You can display a web map using the web map's item ID. Create a map from the web map portal item, and display it in your app.
-
In MainScreen.kt, delete the code inside
create
.Map() Create a
Portal
pointing to ArcGIS Online. Then create aPortalItem
for the Naperville water network, using the portal and the web map's item ID. Then return the map.MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { val portal = Portal( url = "https://www.arcgis.com", connection = Portal.Connection.Anonymous ) val portalItem = PortalItem( portal = portal, itemId = "acc027394bc84c2fb04d1ed317aac674" ) return ArcGISMap(portalItem) }
-
Click Run > Run > app to run the app.
You should see a map of the stormwater network within Naperville, IL, USA . Use the mouse to drag, scroll, and double-click the map view to explore the map.
Specify an area of the web map to take offline
You can specify an area of the web map that you want to be taken offline. Use an Envelope
to specify the geometry of the offline area and a transparent symbol with red outline to visually identify the area on the screen.
-
Create a graphic from an offline map area and a red-outline symbol.
-
Create a function named
create
that takes a lambda as parameter and returns anArea Of Interest() Envelope
. The type of the lambda takes aGraphic
and returns nothing. -
Create an
Envelope
with maximum and minimum coordinates in the center of Naperville, IL, and assign it to a variable namedoffline
.Area -
Create a
SimpleLineSymbol
with a red solid line. Then create a transparentSimpleFillSymbol
using the solid red line. Last, create aGraphic
using the offline area and the fill symbol. -
Use the
o
parameter to invoke the lambda, passing in then Offline Area Created offline
you created. Then returnArea Graphic offline
.Area MainScreen.ktUse dark colors for code blocks fun createAreaOfInterest( onOfflineAreaCreated: (Graphic) -> Unit ): Envelope { // Create an envelope that defines the area to take offline val offlineArea = Envelope( xMin = -88.1526, yMin = 41.7694, xMax = -88.1490, yMax = 41.7714, spatialReference = SpatialReference.wgs84() ) // Create a graphic to display the area to take offline val lineSymbol = SimpleLineSymbol( style = SimpleLineSymbolStyle.Solid, color = Color.red, width = 2f ) val fillSymbol = SimpleFillSymbol( style = SimpleFillSymbolStyle.Solid, color = Color.transparent, outline = lineSymbol ) val offlineAreaGraphic = Graphic( geometry = offlineArea, symbol = fillSymbol ) // Add the offline area outline to the graphics overlay onOfflineAreaCreated(offlineAreaGraphic) return offlineArea }
-
-
Near the top of the
Main
composable, create aScreen remember
variable namedgraphics
. Inside theOverlay remember
block, instantiateGraphicsOverlay
.Then create a
remember
variable namedgraphics
. Inside theOverlays remember
block, create a list that contains one item:graphics
.Overlay Last, pass
graphics
to the map view.Overlays MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { val context = LocalContext.current val coroutineScope = rememberCoroutineScope() val currentProgress = remember { mutableIntStateOf(0) } val showProgressBar = remember { mutableStateOf(false) } val graphicsOverlay = remember { GraphicsOverlay() } val graphicsOverlays = remember { listOf(graphicsOverlay) } var map = remember { createMap() } Scaffold( topBar = { TopAppBar(title = { Text(text = stringResource(id = R.string.app_name)) }) } ) { Column( modifier = Modifier.fillMaxSize().padding(it) ) { if (showProgressBar.value) { LinearProgressIndicator( modifier = Modifier.fillMaxWidth(), progress = currentProgress.intValue / 100f ) } MapView( modifier = Modifier.fillMaxSize(), arcGISMap = map, graphicsOverlays = graphicsOverlays ) } } }
-
Create a
remember
variable namedoffline
. Set it by calling theArea create
function you just created and passing a lambda as theArea Of Interest() o
parameter. Your lambda takes an Offline Area Created Graphic
and adds it to the graphics overlay.Consult the
create
function to see the code that invokes the lambda and passes theArea Of Interest() offline
.Area Graphic MainScreen.ktUse dark colors for code blocks Copy fun createAreaOfInterest( onOfflineAreaCreated: (Graphic) -> Unit ): Envelope { // Create an envelope that defines the area to take offline val offlineArea = Envelope( xMin = -88.1526, yMin = 41.7694, xMax = -88.1490, yMax = 41.7714, spatialReference = SpatialReference.wgs84() ) // Create a graphic to display the area to take offline val lineSymbol = SimpleLineSymbol( style = SimpleLineSymbolStyle.Solid, color = Color.red, width = 2f ) val fillSymbol = SimpleFillSymbol( style = SimpleFillSymbolStyle.Solid, color = Color.transparent, outline = lineSymbol ) val offlineAreaGraphic = Graphic( geometry = offlineArea, symbol = fillSymbol ) // Add the offline area outline to the graphics overlay onOfflineAreaCreated(offlineAreaGraphic) return offlineArea }
MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { val context = LocalContext.current val coroutineScope = rememberCoroutineScope() val currentProgress = remember { mutableIntStateOf(0) } val showProgressBar = remember { mutableStateOf(false) } val graphicsOverlay = remember { GraphicsOverlay() } val graphicsOverlays = remember { listOf(graphicsOverlay) } val offlineArea = remember { createAreaOfInterest( onOfflineAreaCreated = { graphic -> graphicsOverlay.graphics.add(graphic) } ) }
-
Click Run > Run > app to run the app.
You should see a red outline on the stormwater network within Naperville, IL, USA . This indicates the area of the web map that you are going to take offline.
Download an offline map area
The workflow for downloading an offline map area from the server to the Android device is as follows:
- Create an offline map task, passing in the web map.
- Create a default set of parameters to be used for downloading an offline map area. (Use the offline map task.)
- Create a job for downloading the offline map area. (Use the offline map task and pass the default parameters.)
- Start the job.
- Display the offline map area.
For the sake of this tutorial, we will first define a function named download
, which will take OfflineMapTask
and GenerateOfflineMapParameters
(and other values) and download the offline map area. Then we will go to the Main
block, create Offline
and Generate
objects, and call download
with the appropriate values.
Define downloadOfflineMapArea()
-
Create a top-level
suspend
function nameddownload
. Declare the parameters indicated in the code below.Offline M a p Area() MainScreen.ktUse dark colors for code blocks suspend fun downloadOfflineMapArea( context: Context, coroutineScope: CoroutineScope, offlineMapTask: OfflineMapTask, parameters: GenerateOfflineMapParameters, updateProgress: (Int) -> Unit, onDownloadOfflineMapSuccess: (ArcGISMap) -> Unit, onDownloadOfflineMapFailure: () -> Unit ) { }
-
Create a variable named
download
, which holds the path on the device system where offline map area will be downloaded. The path is a string.Location MainScreen.ktUse dark colors for code blocks suspend fun downloadOfflineMapArea( context: Context, coroutineScope: CoroutineScope, offlineMapTask: OfflineMapTask, parameters: GenerateOfflineMapParameters, updateProgress: (Int) -> Unit, onDownloadOfflineMapSuccess: (ArcGISMap) -> Unit, onDownloadOfflineMapFailure: () -> Unit ) { // Build a folder path named with today's date/time to store the offline map val downloadLocation = context.getExternalFilesDir(null)?.path + "OfflineMap_" + Calendar.getInstance().time }
-
Create a job that will generate the offline map area. To do this, call
OfflineMapTask.createGenerateOfflineMapJob()
and pass the default parameters and thedownload
.Location MainScreen.ktUse dark colors for code blocks suspend fun downloadOfflineMapArea( context: Context, coroutineScope: CoroutineScope, offlineMapTask: OfflineMapTask, parameters: GenerateOfflineMapParameters, updateProgress: (Int) -> Unit, onDownloadOfflineMapSuccess: (ArcGISMap) -> Unit, onDownloadOfflineMapFailure: () -> Unit ) { // Build a folder path named with today's date/time to store the offline map val downloadLocation = context.getExternalFilesDir(null)?.path + "OfflineMap_" + Calendar.getInstance().time // Create a job with GenerateOfflineMapParameters and a download directory path val generateOfflineMapJob = offlineMapTask.createGenerateOfflineMapJob( parameters = parameters, downloadDirectoryPath = downloadLocation ) }
-
Update the progress of the generate offline map job.
MainScreen.ktUse dark colors for code blocks suspend fun downloadOfflineMapArea( context: Context, coroutineScope: CoroutineScope, offlineMapTask: OfflineMapTask, parameters: GenerateOfflineMapParameters, updateProgress: (Int) -> Unit, onDownloadOfflineMapSuccess: (ArcGISMap) -> Unit, onDownloadOfflineMapFailure: () -> Unit ) { // Build a folder path named with today's date/time to store the offline map val downloadLocation = context.getExternalFilesDir(null)?.path + "OfflineMap_" + Calendar.getInstance().time // Create a job with GenerateOfflineMapParameters and a download directory path val generateOfflineMapJob = offlineMapTask.createGenerateOfflineMapJob( parameters = parameters, downloadDirectoryPath = downloadLocation ) coroutineScope.launch { generateOfflineMapJob.progress.collect { progress -> updateProgress(progress) } } }
-
Start the generate offline map job.
MainScreen.ktUse dark colors for code blocks suspend fun downloadOfflineMapArea( context: Context, coroutineScope: CoroutineScope, offlineMapTask: OfflineMapTask, parameters: GenerateOfflineMapParameters, updateProgress: (Int) -> Unit, onDownloadOfflineMapSuccess: (ArcGISMap) -> Unit, onDownloadOfflineMapFailure: () -> Unit ) { // Build a folder path named with today's date/time to store the offline map val downloadLocation = context.getExternalFilesDir(null)?.path + "OfflineMap_" + Calendar.getInstance().time // Create a job with GenerateOfflineMapParameters and a download directory path val generateOfflineMapJob = offlineMapTask.createGenerateOfflineMapJob( parameters = parameters, downloadDirectoryPath = downloadLocation ) coroutineScope.launch { generateOfflineMapJob.progress.collect { progress -> updateProgress(progress) } } // Start the job to download the offline map generateOfflineMapJob.start() showMessage( context, "Generate offline map job has started.") }
-
Respond to the result of the generate offline map job.
Among the parameters passed to
download
, two are particularly important here:Offline M a p Area() o
n Download Offline M a p Success o
n Download Offline M a p Failure
Both parameters are of function type (lambda).
In the
o
block, invoke then Success o
lambda, passing the offline map from the generate offline map result. In then Download Offline M a p Success() o
block, invoke then Failure o
lambda.n Download Offline M a p Failure() MainScreen.ktUse dark colors for code blocks suspend fun downloadOfflineMapArea( context: Context, coroutineScope: CoroutineScope, offlineMapTask: OfflineMapTask, parameters: GenerateOfflineMapParameters, updateProgress: (Int) -> Unit, onDownloadOfflineMapSuccess: (ArcGISMap) -> Unit, onDownloadOfflineMapFailure: () -> Unit ) { // Build a folder path named with today's date/time to store the offline map val downloadLocation = context.getExternalFilesDir(null)?.path + "OfflineMap_" + Calendar.getInstance().time // Create a job with GenerateOfflineMapParameters and a download directory path val generateOfflineMapJob = offlineMapTask.createGenerateOfflineMapJob( parameters = parameters, downloadDirectoryPath = downloadLocation ) coroutineScope.launch { generateOfflineMapJob.progress.collect { progress -> updateProgress(progress) } } // Start the job to download the offline map generateOfflineMapJob.start() showMessage( context, "Generate offline map job has started.") generateOfflineMapJob.result().onSuccess { generateOfflineMapResult -> onDownloadOfflineMapSuccess(generateOfflineMapResult.offlineMap) }.onFailure { onDownloadOfflineMapFailure() } }
Call downloadOfflineMapArea()
-
In the
Main
composable, after theScreen map
variable: add theLaunched
composable. Then create anEffect OfflineMapTask
, passingmap
.MainScreen.ktUse dark colors for code blocks val context = LocalContext.current val coroutineScope = rememberCoroutineScope() val currentProgress = remember { mutableIntStateOf(0) } val showProgressBar = remember { mutableStateOf(false) } val graphicsOverlay = remember { GraphicsOverlay() } val graphicsOverlays = remember { listOf(graphicsOverlay) } val offlineArea = remember { createAreaOfInterest( onOfflineAreaCreated = { graphic -> graphicsOverlay.graphics.add(graphic) } ) } var map = remember { createMap() } LaunchedEffect(Unit) { // Create an offline map task using the current map val offlineMapTask = OfflineMapTask(map) }
-
You will now create a default set of parameters for generating an offline map area on the server and and downloading the area.
Call
OfflineMapTask.createDefaultGenerateOfflineMapParameters()
, passing the offline map area. Then create ano
block to handle the successful creation of the default parameters.n Success MainScreen.ktUse dark colors for code blocks val context = LocalContext.current val coroutineScope = rememberCoroutineScope() val currentProgress = remember { mutableIntStateOf(0) } val showProgressBar = remember { mutableStateOf(false) } val graphicsOverlay = remember { GraphicsOverlay() } val graphicsOverlays = remember { listOf(graphicsOverlay) } val offlineArea = remember { createAreaOfInterest( onOfflineAreaCreated = { graphic -> graphicsOverlay.graphics.add(graphic) } ) } var map = remember { createMap() } LaunchedEffect(Unit) { // Create an offline map task using the current map val offlineMapTask = OfflineMapTask(map) // Create a default set of parameters for generating the offline map from the area of interest offlineMapTask.createDefaultGenerateOfflineMapParameters(offlineArea) .onSuccess { parameters -> } }
-
In the
o
block, set the value ofn Success show
and show a message saying that the default parameters have been created.Progress Bar MainScreen.ktUse dark colors for code blocks val context = LocalContext.current val coroutineScope = rememberCoroutineScope() val currentProgress = remember { mutableIntStateOf(0) } val showProgressBar = remember { mutableStateOf(false) } val graphicsOverlay = remember { GraphicsOverlay() } val graphicsOverlays = remember { listOf(graphicsOverlay) } val offlineArea = remember { createAreaOfInterest( onOfflineAreaCreated = { graphic -> graphicsOverlay.graphics.add(graphic) } ) } var map = remember { createMap() } LaunchedEffect(Unit) { // Create an offline map task using the current map val offlineMapTask = OfflineMapTask(map) // Create a default set of parameters for generating the offline map from the area of interest offlineMapTask.createDefaultGenerateOfflineMapParameters(offlineArea) .onSuccess { parameters -> // When the parameters are successfully created, show progress bar and message showProgressBar.value = true showMessage(context, "Default parameters have been created.") } }
-
Continuing in the
o
block, calln Success download
function, passing theOffline M a p Area() offline
and the defaultM a p Task parameters
. Also pass a lambda foro
and a lambda forn Download Offline M a p Success o
.n Download Offline M a p Failure The
o
lambda hides the progress bar and displays a message saying the offline map area downloaded successfully. Then it assigns the lambda'sn Download Offline M a p Success offline
parameter to theMap map
variable, which displays the offline map in the map view. Last, the lambda clears the red outline around the offline map area.The
o
lambda hides the progress bar and displays a message saying the offline map area failed to download.n Download Offline M a p Failure MainScreen.ktUse dark colors for code blocks val context = LocalContext.current val coroutineScope = rememberCoroutineScope() val currentProgress = remember { mutableIntStateOf(0) } val showProgressBar = remember { mutableStateOf(false) } val graphicsOverlay = remember { GraphicsOverlay() } val graphicsOverlays = remember { listOf(graphicsOverlay) } val offlineArea = remember { createAreaOfInterest( onOfflineAreaCreated = { graphic -> graphicsOverlay.graphics.add(graphic) } ) } var map = remember { createMap() } LaunchedEffect(Unit) { // Create an offline map task using the current map val offlineMapTask = OfflineMapTask(map) // Create a default set of parameters for generating the offline map from the area of interest offlineMapTask.createDefaultGenerateOfflineMapParameters(offlineArea) .onSuccess { parameters -> // When the parameters are successfully created, show progress bar and message showProgressBar.value = true showMessage(context, "Default parameters have been created.") // Download the offline map downloadOfflineMapArea( context = context, coroutineScope = coroutineScope, offlineMapTask = offlineMapTask, parameters = parameters, updateProgress = { currentProgress.intValue = it }, onDownloadOfflineMapSuccess = { offlineMap -> // Hide progress bar and show message when the job executes successfully showProgressBar.value = false showMessage(context, "Downloaded offline map area successfully.") // Replace the current map with the result offline map map = offlineMap // Clear the offline area outline graphicsOverlay.graphics.clear() }, onDownloadOfflineMapFailure = { showProgressBar.value = false showMessage(context, "Failed to download offline map area.") } ) } }
-
(Optional) The code in this tutorial calls a function to display messages to the user. One possible implementation of
show
is the following.Message() MainScreen.ktUse dark colors for code blocks fun showMessage(context: Context, message: String) { Toast.makeText(context, message, Toast.LENGTH_LONG).show() }
-
Click Run > Run > app to run the app.
Initially, you should see the web map centered on Naperville, IL, USA, with a red outline indicating the area that will be taken offline. The download starts automatically, and the progress bar displays at the top of the screen as a dark-blue line, expanding to the right as progress is made. The download might take from a few seconds to a minute or more, depending on your internet connection.
After the offline map area is downloaded to the Android device, you should see the offline map area, surrounded by a gray grid. You can now remove your network connection, and you will still be able to use the mouse to drag, scroll, and double-click the map view to explore this offline map area.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: