ArcGIS supports secure access to location services and private data. It ensures that only valid, authorized users and services access protected information. In order to access secure ArcGIS resources, you need an access token. To get an access token, you need to choose a type of authentication and implement an authentication workflow in your app. The type of authentication you use will depend on the security and access requirements of your app.
There are three types of authentication that you can use to get an access token:
- API key authentication: grants a long-lived access token to authenticate requests to ArcGIS location services and, with an ArcGIS Location Platform account, private content. It is the recommended type of authentication for developers new to ArcGIS or to development in general. Go to the Create an API key tutorial to obtain a new API Key access token.
- User authentication: a collection of authentication workflows that connect your app to a user's ArcGIS account.
- OAuth 2.0: manage ArcGIS authentication and grant a short-lived access token generated via OAuth 2.0. This gives your application permission to access ArcGIS secured services authorized to an existing ArcGIS user's account.
- Generate token: manages ArcGIS authentication and grants a short-lived access token generated via Esri's proprietary token-based authentication mechanism. This gives your application permission to access ArcGIS secured services authorized to an existing ArcGIS user's account.
- Network credential: manage network authentication (also known as web-tier authentication) for ArcGIS Enterprise. This gives your application permission to access network secured services authorized to your web-tier's identity store user accounts. Supports Public Key Infrastructure (PKI), Integrated Windows Authentication (IWA) and HTTP Basic.
- App authentication: uses the registered application's credentials to access location services on ArcGIS. It manages ArcGIS authentication and grants a short-lived access token generated via OAuth 2.0 using the Application item's Client ID and Client Secret outside of the context of a user.
For more information, see the Security and authentication guide.
Choose a type of authentication
The following considerations can help determine which type of authentication to implement:
-
Access to resources—Your app can access location services using API key authentication, User authentication, or App authentication. To access private data hosted in ArcGIS Online or ArcGIS Enterprise, or content that requires an ArcGIS Online subscription, your app needs an authenticated user to sign in with their account.
-
User experience—If you don't want to make users log in, your app can access location services using API key authentication or App authentication. In this case, users will not need to have an ArcGIS account in order to use your app.
-
Usage charges—If you want service usage to be charged to the user's account, your app must request that the user log in. When using API key authentication or App authentication, all access to services from your app will be charged to your ArcGIS account.
You might also need to consider the level of security required for your app, how your app will be distributed, and your available ArcGIS products and accounts.
Scenario | Solution |
---|---|
Your app requires access to location services only, you don't want to make users log in, and you are willing to pay for all charges incurred from usage of the app. | API key authentication or App authentication |
Your app requires access to location services only and you want usage charged to the user. | User authentication |
Your app needs to access content that requires an ArcGIS Online subscription. | User authentication |
Your app needs to access private hosted data on your ArcGIS Location Platform account. | API key authentication or App authentication |
Your app allows users to view and edit private data hosted in ArcGIS Online or ArcGIS Enterprise. | User authentication |
You plan to distribute your app through ArcGIS Marketplace. | User authentication |
API key authentication
An API Key can grant your public-facing application access to specific ArcGIS location services, and, with an ArcGIS Location Platform account, private content, items, and limited client referrers.
Use API key authentication when you want to:
- Quickly write applications that consume location services.
- Provide access to services without requiring users to sign in with an ArcGIS account.
Use API key access tokens
An API key, can be used to authorize access to specific ArcGIS location services, and, with an ArcGIS Location Platform account, private content, items, and limited client referrers. Go to the Create an API key tutorial to obtain a new access token.
If you set the access token on the ArcGISEnvironment
, all requests made by your app will be authorized using this token. You will be able to view the app's usage telemetry on the ArcGIS Location Platform.
You can also set the access token on any class that implements ApiKeyResource
. When you set an access token for a specific class, it will override any access token you may have set on ArcGISEnvironment
, enabling more granular usage telemetry and management for ArcGIS Online resources used by your app.
Classes that implement ApiKeyResource
include:
-
Basemap
-
ArcGISSceneLayer
-
ArcGISTiledLayer
-
ArcGISVectorTiledLayer
-
ServiceFeatureTable
-
ExportVectorTilesTask
-
LocatorTask
-
GeodatabaseSyncTask
-
ClosestFacilityTask
-
RouteTask
-
ServiceAreaTask
-
ExportTileCacheTask
User authentication
User authentication is a set of authentication workflows that allow users with an ArcGIS account to sign into an application and access ArcGIS content, services, and resources. The typical authentication protocol used is OAuth2.0. When a user signs into an application with their ArcGIS account, an access token is generated that authorizes the application to access services and content on their behalf. The resources and functionality available depend on the user type, roles, and privileges of the user's ArcGIS account.
Services that your app accesses with user authentication will be billed to the authenticated user's ArcGIS account and its associated ArcGIS subscription. If your application will access your users' secure content in ArcGIS or if you plan to distribute your application through ArcGIS Marketplace, you must use user authentication.
Implement user authentication when you want to:
- Ensure users are signed in and authenticated with their own ArcGIS account.
- Use your app user's credits to pay for their private data, content, or service transactions.
- Limit the length of time users can be signed in to your app with a temporary token.
- Distribute your app through ArcGIS Marketplace.
App authentication
App authentication grants a short-lived access token, generated via OAuth 2.0, authorizing your application to access location services, such as basemap layers, search, and routing.
Use app authentication when you want to:
- Access location services with a more secure process and a short-lived token.
- Provide access to services without requiring users to have an ArcGIS account.
Authentication tool
In this SDK, all aspects of ArcGIS and network authentication have been encapsulated into a single ArcGIS Maps SDK for Kotlin toolkit component called the Authenticator. This component can handle several types of authentication challenges, such as ArcGIS authentication (token and OAuth), Integrated Windows Authentication (IWA), and Client Certificate (PKI), and provides default UI for login prompts, certificate selection prompts, and server trust prompts. For example, here is the default alert prompting the user for username and password credentials.
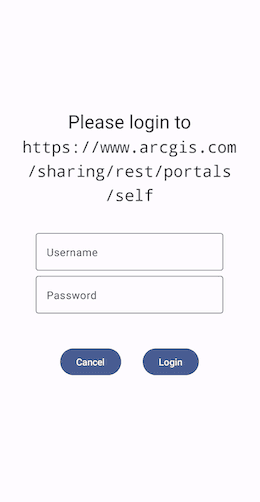
The Authenticator is designed to be displayed on top of your app's UI. It should be called at a near-root level, for example, at the same level as a Nav
. The Authenticator should also be the last call of the function so it draws over other content.
@Composable
fun MyApp() {
val authenticatorState: AuthenticatorState = remember { AuthenticatorState() }
MyAppContent()
Authenticator(authenticatorState)
}
The authentication toolkit library provides an extension function on AuthenticationManger that revokes all OAuth user tokens and clears all credentials from the credential stores.
ArcGISEnvironment.authenticationManager.signOut()
To see the Authenticator in action, including the use of OAuth, check out the Authentication Microapp.
Authentication manager
To manage the authentication process you must use the AuthenticationManager
that is available as a static property on the ArcGISEnvironment
.
ArcGISEnvironment.authenticationManager
The AuthenticationManager
provides:
- ArcGIS and network challenge handlers that allow you to respond to the authentication challenges. For example, you can write code to present the user with a login screen and then continue to authenticate with those credentials. For more information, see Handle authentication challenges.
- The credential stores are available for you to place ArcGIS and network credentials. These stores are automatically checked when your application attempts to connect to secure resources. These stores can also be persisted so that the user does not have to sign in again when the application is re-launched. For more information, see Create and store credentials.
Handle authentication challenges
If your application attempts to access a secure resource and there is no matching credential in the credential store, an authentication challenge is raised:
ArcGISAuthenticationChallenge
is raised if the ArcGIS secured resource requires OAuth or ArcGIS Token authentication.NetworkAuthenticationChallenge
is raised if the ArcGIS secured resource requires network credentials, such as Integrated Windows Authentication (IWA) or Public Key Infrastructure (PKI).
Catch and respond to these authentication challenges using the ArcGISAuthenticationChallengeHandler
and NetworkAuthenticationChallengeHandler
, respectively. These are functional interfaces that each implement a single abstract method called handle
and handle
respectively. Instead of creating a class that implements the interface, you can use a lambda expression.
ArcGIS authentication challenge handler
The ArcGISAuthenticationChallengeHandler
is used to handle authentication challenges from ArcGIS secured resources that require OAuth or ArcGIS Token authentication. Handle the challenge by returning the ArcGISAuthenticationChallengeResponse
. It has the following options:
Continue
- Handles the challenge with the specified credential.With Credential Continue
- Handles the challenge without a credential, causing it to fail with the original authentication errorA n d Fail Continue
- Handles the challenge with an error that occurred while trying to generate a credential. The request which issued the authentication challenge will fail with the given error.A n d Fail With Error Cancel
- Cancels the request that initiated the challenge.
Create a custom ArcGISAuthenticationChallengeHandler
and pass it to the AuthenticationManager.arcGISAuthenticationChallengeHandler
.
ArcGISEnvironment.authenticationManager.arcGISAuthenticationChallengeHandler =
ArcGISAuthenticationChallengeHandler { challenge ->
// If OAuth user configuration is available for the challenge then create `OAuthUserCredential`
if (oAuthConfiguration.canBeUsedForUrl(challenge.requestUrl)) {
// Prompt to open browser and sign in with OAuth username and password
// ...
ArcGISAuthenticationChallengeResponse
.ContinueWithCredential(oAuthUserCredential)
} else {
// Prompt user for getting the username and password to create the `TokenCredential`
// ...
// Create TokenCredential
val tokenCredential = TokenCredential.createWithChallenge(challenge, username, password, 30).getOrNull()
tokenCredential?.let { ArcGISAuthenticationChallengeResponse.ContinueWithCredential(it) } ?: ArcGISAuthenticationChallengeResponse.ContinueAndFail
}
}
Network authentication challenge handler
The NetworkAuthenticationChallengeHandler
is used to handle authentication challenges from ArcGIS secured resources that require network credentials, such as Integrated Windows Authentication (IWA) or Public Key Infrastructure (PKI). Handle the challenge by returning the NetworkAuthenticationChallengeResponse
. It has the following options:
Continue
- Handles the challenge with the specified credential.With Credential Continue
- Handles the challenge without a credential, causing it to fail with the original authentication errorA n d Fail Continue
- Handles the challenge with an error that occurred while trying to generate a credential. The request which issued the authentication challenge will fail with the given error.A n d Fail With Error Cancel
- Cancels the request that initiated the challenge.
Create a custom NetworkAuthenticationChallengeHandler
and pass it to the AuthenticationManager.networkAuthenticationChallengeHandler
.
ArcGISEnvironment.authenticationManager.networkAuthenticationChallengeHandler =
NetworkAuthenticationChallengeHandler { authenticationChallenge ->
when (authenticationChallenge.networkAuthenticationType) {
is NetworkAuthenticationType.UsernamePassword ->
// Create the Password Credential
val credential = PasswordCredential(username, password)
NetworkAuthenticationChallengeResponse.ContinueWithCredential(credential)
is NetworkAuthenticationType.ServerTrust ->
NetworkAuthenticationChallengeResponse.ContinueWithCredential(ServerTrust)
is NetworkAuthenticationType.Certificate -> {
val selectedAlias = showCertificatePicker(activityContext)
selectedAlias?.let {
NetworkAuthenticationChallengeResponse.ContinueWithCredential(CertificateCredential(it))
} ?: NetworkAuthenticationChallengeResponse.ContinueAndFail
}
}
}
Create and store credentials
You can create a credential in the authentication challenge handler when an authentication challenge is triggered. For more information, see Handle authentication challenges. The AuthenticationManager
stores these ArcGIS and network credentials in the following stores:
ArcGISCredentialStore
stores ArcGIS credentials.NetworkCredentialStore
stores Network credentials.
These credential stores exist for the lifetime of the application and ensure that an authentication challenge is not raised if a matching credential exists in the store. If you want to avoid prompting users for credentials between application sessions, make them persistent using the companion functions ArcGISCredential
and Network
. This uses Android's EncryptedSharedPreferences.
lifecycleScope.launch {
val arcGISCredentialStore = ArcGISCredentialStore.createWithPersistence().getOrThrow()
ArcGISEnvironment.authenticationManager.arcGISCredentialStore = arcGISCredentialStore
val networkCredentialStore = NetworkCredentialStore.createWithPersistence().getOrThrow()
ArcGISEnvironment.authenticationManager.networkCredentialStore = networkCredentialStore
}
During application sign-out you should revoke all OAuth user tokens and then clear all credentials from the credential stores.
// Iterate through the collection of `OAuthUserCredential` objects stored in the `ArcGISCredentialStore`.
// Revoke the refresh and access token of each `OAuthUserCredential`.
oAuthUserCredential.revokeToken()
// Clear all ArcGIS and network credentials from their respective stores.
ArcGISEnvironment.authenticationManager.arcGISCredentialStore.removeAll()
ArcGISEnvironment.authenticationManager.networkCredentialStore.removeAll()
ArcGIS credentials
You can access secure resources with user authentication or app authentication using the following credential types:
OAuthUserCredential
- A credential object used to access OAuth token-secured ArcGIS resources with a specificOAuthUserConfiguration
.OAuthApplicationCredential
- A credential object used to access OAuth token-secured ArcGIS resources using the application's credentials.TokenCredential
- A credential object used to access token-secured ArcGIS resources.PregeneratedTokenCredential
- A credential object used to access token-secured ArcGIS resources using a token that is generated outside of your application.
If you know the services domain/server context, you can create an ArcGIS credential independent of loading the specific resource and store it in the ArcGISCredentialStore
.
OAuthUserCredential
To create an OAuthUserCredential
, provide an OAuthUserConfiguration
with a valid portal URL, client ID, and redirect URL. You must present a prompt, in a browser supported by the device, for the user to enter their username and password. The response from the browser should be handled within your activity or the fragment that launched the browser prompt. Once the OAuthUserCredential
is created, you will be able to access the token information from the asynchronous token
property.
// Define OAuthConfiguration
private val oAuthConfiguration = OAuthUserConfiguration(
"<portal URL>",
"<ClientID>",
"<redirectURI>"
)
// Create OAuthUserCredential by passing OAuthConfiguration and initiate OAuth login process by invoking the provided startSignIn lambda
val oAuthUserCredential = OAuthUserCredential.create(oAuthConfiguration) { oAuthUserSignIn ->
promptForOAuthUserSignIn(oAuthUserSignIn)
}.getOrThrow()
See the Android details about how to launch custom chrome tabs in android and working with getting result from an activity
To see this authentication in action, look at the authenticate-with-oauth sample.
OAuthApplicationCredential
To create an OAuthApplicationCredential
, provide a valid portal URL, a client ID, and a client secret. Optionally, you can specify the token expiration in minutes. Once the OAuthApplicationCredential
is created, you will be able to access the token information from the asynchronous token
property.
scope.launch{
val oAuthApplicationCredential = OAuthApplicationCredential.create("portalUrl","clientId","clientSecret", 30).onSuccess {
var tokenInfo = it.getTokenInfo().getOrNull()
var accessToken = tokenInfo?.accessToken
}
}
TokenCredential
To create a TokenCredential
, provide a secured service URL, valid username, and password. Optionally, you can specify token expiration minutes. Once a TokenCredential
is created, you will be able to access token information from the asynchronous token
property.
scope.launch {
TokenCredential.create(requestUrl,username, password, 30).onSuccess {
// Get accesstoken from credential
var tokenInfo = it.getTokenInfo().getOrNull()
var accessToken = tokenInfo?.accessToken
}
}
PregeneratedTokenCredential
To create a PregeneratedTokenCredential
, provide a previously generated short or long-lived access token. Use this when the access token is created using the generateToken REST endpoint directly. You must provide the referer if one was used while generating the token.
var accessToken = "xxxxxxx"
var tokenInfo = val tokenInfo = TokenInfo(accessToken, Instant.now().plus(2, ChronoUnit.HOURS), true)
var pregeneratedTokenCredential = PregeneratedTokenCredential(requestUrl, tokenInfo)
Network credentials
You can access resources secured by network authentication using the following credential types:
PasswordCredential
- A credential object that is used to authenticate HTTP Basic or Integrated Windows Authentication (IWA) secured resources.CertificateCredential
- A credential object that is used to authenticate Public Key Infrastructure (PKI) secured resources.
PasswordCredential
The PasswordCredential
is used to authenticate HTTP Basic or Integrated Windows Authentication (IWA) secured resources.
var passwordCredential = PasswordCredential("username", "password")
CertificateCredential
CertificateCredential
represents a digital client certificate used to access certificate secured resources. All the digital certificates need to be pre-installed in the device keychain. The CertificateCredential
can be created using the alias of the chosen certificate.
private suspend fun showCertificatePicker(activityContext: Activity): String? =
suspendCancellableCoroutine { continuation ->
val aliasCallback = KeyChainAliasCallback { alias ->
continuation.resume(alias)
}
KeyChain.choosePrivateKeyAlias(
activityContext, aliasCallback, null, null, null, null
)
}
val selectedAlias = showCertificatePicker(activityContext)
var certificateCredential = selectedAlias?.let { CertificateCredential(it) } ?: null