Learn how to create and display a scene with a basemap layer and an elevation layer. Set properties of the scene's camera to control the 3D perspective.
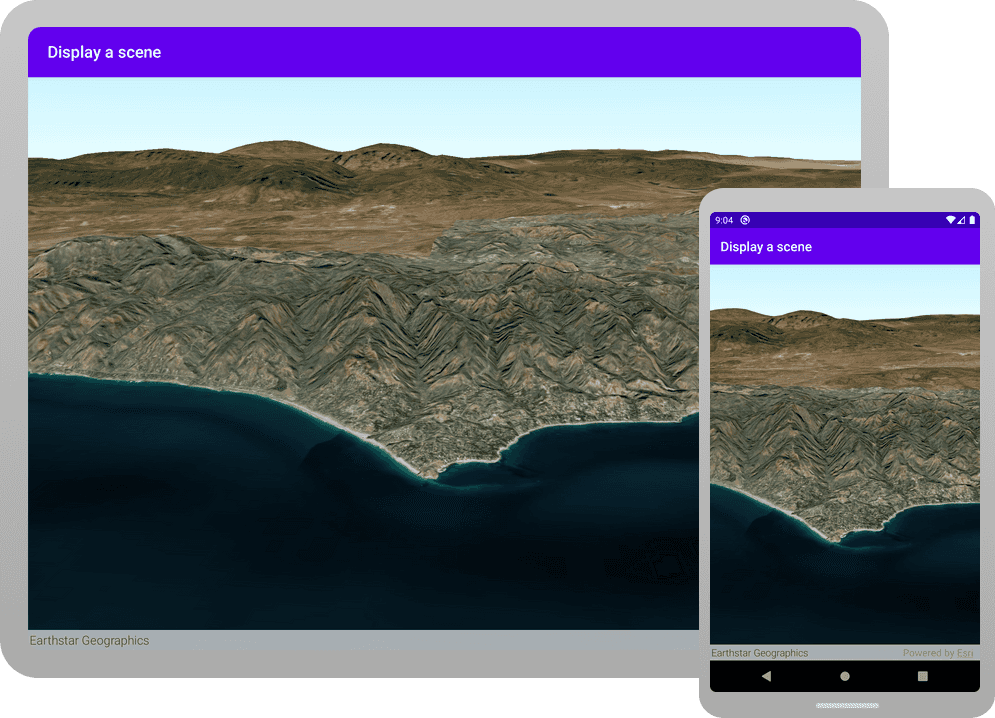
Like a map, a scene contains layers of geographic data. It contains a basemap layer and, optionally, one or more data layers. To provide a realistic view of the terrain, you can also add elevation layers to define the height of the surface across the scene. The 3D perspective of the scene is controlled by the scene's camera, which defines the position of the scene observer in 3D space.
In this tutorial, you create and display a scene using the imagery basemap layer. The surface of the scene is defined with an elevation layer and the camera is positioned to display an area of the Santa Monica Mountains in the scene view.
The scene and code will be used as the starting point for other 3D tutorials.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
A development and deployment environment that meets the system requirements.
-
An IDE for Android development in Kotlin.
Steps
Create a new Android Studio project
Use Android Studio to create an app and configure it to reference the API.
-
Open Android Studio.
-
In the Welcome to Android Studio window, click New Project.
Or if you already have Android Studio opened, click File > New > New Project in the menu bar.
-
In the New Project window, make sure Phone and Tablet tab is selected, and then select Empty Activity. Click Next.
-
In the next window, set the following options and then click Finish.
- Name:
Display a scene
. - Package name: Change to
com.example.app
. Or change to match your organization. - Save location: Set to a new folder.
- Minimum SDK: API 26 ("Oreo"; Android 8.0)
- Build configuration language: Kotlin DSL (build.gradle.kts)
- Name:
-
-
In the Project tool window, make sure that your current view is Android. These tutorial instructions refer to that view.
If your view name is something other than Android (such as Project or Packages), click on the leftmost control in the title bar of the Project tool window, and select Android from the list.
-
From the Project tool window, open Gradle Scripts > build.gradle.kts (Project: Display_a_scene). Replace the contents of the file with the following code:
build.gradle.kts (Project: Display_a_scene)Use dark colors for code blocks // Top-level build file where you can add configuration options common to all sub-projects/modules. plugins { id("com.android.application") version "8.2.0" apply false id("org.jetbrains.kotlin.android") version "1.9.10" apply false }
-
From the Project tool window, open Gradle Scripts > build.gradle.kts (Module :app). Replace the contents of the file with the expanded code below:
build.gradle.kts (Module: app)Use dark colors for code blocks kotlinOptions { jvmTarget = "17" } packaging { resources { excludes += "/META-INF/DEPENDENCIES" } } namespace = "com.example.app" } dependencies { implementation("androidx.core:core-ktx:1.12.0") implementation("androidx.lifecycle:lifecycle-runtime-ktx:2.7.0") implementation("androidx.activity:activity-compose:1.8.2") // Jetpack Compose Bill of Materials implementation(platform("androidx.compose:compose-bom:2023.10.01")) // Jetpack Compose dependencies implementation("androidx.compose.ui:ui") implementation("androidx.compose.material3:material3") // ArcGIS Map Kotlin SDK dependencies implementation("com.esri:arcgis-maps-kotlin:200.4.0") // Toolkit dependencies implementation(platform("com.esri:arcgis-maps-kotlin-toolkit-bom:200.4.0")) implementation("com.esri:arcgis-maps-kotlin-toolkit-geoview-compose") }
-
From the Project tool window, open Gradle Scripts > settings.gradle.kts. Replace the contents of the file with the expanded code below:
settings.gradle.kts (Project Settings)Use dark colors for code blocks pluginManagement { repositories { google() mavenCentral() gradlePluginPortal() } } dependencyResolutionManagement { @Suppress("UnstableApiUsage") repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) @Suppress("UnstableApiUsage") repositories { google()
-
Sync the Gradle changes. Click the Sync now prompt or click the refresh icon (Sync Project with Gradle Files) in the toolbar. This may take several minutes.
-
From the Project tool window, open app > manifests > AndroidManifest.xml. Update the Android manifest to allow internet access.
Insert these new elements within the
manifest
element. Do not alter or remove any other statements.Depending on what ArcGIS functionality you add in future tutorials, it is likely you will need to add additional permissions to your manifest.
AndroidManifest.xmlUse dark colors for code blocks Add line. <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"> <uses-permission android:name="android.permission.INTERNET" />
Create a scene
-
From the Project tool window, right click on app > kotlin+java > com.example.app, select New > package from the list. Enter com.example.app.screens as the package name. Hit Enter on your keyboard. This step creates a new package that will contain all the UI files.
-
Right click on the screens package you just created, select New > Kotlin Class/File from the list. In the pop-up window, select File and enter MainScreen as the file name. Hit Enter on your keyboard.
-
In MainScreen.kt, delete any lines of code that were inserted automatically by Android Studio. Then add the following OptIn annotation, package name, and imports.
MainScreen.ktUse dark colors for code blocks @file:OptIn(ExperimentalMaterial3Api::class) package com.example.app.screens import androidx.compose.foundation.layout.fillMaxSize import androidx.compose.foundation.layout.padding import androidx.compose.material3.ExperimentalMaterial3Api import androidx.compose.material3.Scaffold import androidx.compose.material3.Text import androidx.compose.material3.TopAppBar import androidx.compose.runtime.Composable import androidx.compose.runtime.remember import androidx.compose.ui.Modifier import androidx.compose.ui.res.stringResource import com.arcgismaps.geometry.Point import com.arcgismaps.geometry.SpatialReference import com.arcgismaps.mapping.ArcGISScene import com.arcgismaps.mapping.ArcGISTiledElevationSource import com.arcgismaps.mapping.BasemapStyle import com.arcgismaps.mapping.Surface import com.arcgismaps.mapping.Viewpoint import com.arcgismaps.mapping.view.Camera import com.arcgismaps.toolkit.geoviewcompose.SceneView import com.example.app.R
-
You will start by creating a function named
create
.Scene() Inside that function, you will create an
ArcGISScene
, assign a base surface to it, and use the top-level composable functionremember
to retain state across recompositions.Then you will create a camera location and a
Camera
, use them to create aViewpoint
, and then assign the view point to theinitial
property of theViewpoint ArcGISScene
.-
Create a top-level function named
create
that returns anScene() ArcGISScene
.MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { }
-
Create a new
ArcGISTiledElevationSource
. Then create aSurface
and, inside theapply
block forSurface
, add the elevation source to theelevation
property, and set theSources elevation
property to 2.5f, which increases the 3D effect of the elevation.Exaggeration An elevation source can define a surface with 3D terrain in a scene. Without an elevation source, the default globe surface is used to display the scene.
MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { // add base surface for elevation data val elevationSource = ArcGISTiledElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer") val surface = Surface().apply { elevationSources.add(elevationSource) // add an exaggeration factor to increase the 3D effect of the elevation. elevationExaggeration = 2.5f } }
-
Create a
Point
for the camera and assign it to the variablecamera
. Then create aLocation Camera
, passing the camera ocation and values for the camera's heading, pitch, and roll.MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { // add base surface for elevation data val elevationSource = ArcGISTiledElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer") val surface = Surface().apply { elevationSources.add(elevationSource) // add an exaggeration factor to increase the 3D effect of the elevation. elevationExaggeration = 2.5f } val cameraLocation = Point( x = -118.794, y = 33.909, z = 5330.0, spatialReference = SpatialReference.wgs84() ) val camera = Camera( locationPoint = cameraLocation, heading = 355.0, pitch = 72.0, roll = 0.0 ) }
-
Create an
ArcGISScene
with aBasemapStyle.ArcGISImagery
. Then callapply()
on the newArcGISScene
. Thecreate
function returns thisScene() ArcGISScene
.For more information on
apply()
, see Kotlin scope functions.MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { // add base surface for elevation data val elevationSource = ArcGISTiledElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer") val surface = Surface().apply { elevationSources.add(elevationSource) // add an exaggeration factor to increase the 3D effect of the elevation. elevationExaggeration = 2.5f } val cameraLocation = Point( x = -118.794, y = 33.909, z = 5330.0, spatialReference = SpatialReference.wgs84() ) val camera = Camera( locationPoint = cameraLocation, heading = 355.0, pitch = 72.0, roll = 0.0 ) return ArcGISScene(BasemapStyle.ArcGISImagery).apply { } }
-
In the
apply
block, set thebase
property of theSurface ArcGISScene
tosurface
.MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { // add base surface for elevation data val elevationSource = ArcGISTiledElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer") val surface = Surface().apply { elevationSources.add(elevationSource) // add an exaggeration factor to increase the 3D effect of the elevation. elevationExaggeration = 2.5f } val cameraLocation = Point( x = -118.794, y = 33.909, z = 5330.0, spatialReference = SpatialReference.wgs84() ) val camera = Camera( locationPoint = cameraLocation, heading = 355.0, pitch = 72.0, roll = 0.0 ) return ArcGISScene(BasemapStyle.ArcGISImagery).apply { baseSurface = surface } }
-
Create a
Viewpoint
usingcamera
andLocation camera
and set it as the initial viewpoint for the scene.MainScreen.ktUse dark colors for code blocks fun createScene(): ArcGISScene { // add base surface for elevation data val elevationSource = ArcGISTiledElevationSource("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer") val surface = Surface().apply { elevationSources.add(elevationSource) // add an exaggeration factor to increase the 3D effect of the elevation. elevationExaggeration = 2.5f } val cameraLocation = Point( x = -118.794, y = 33.909, z = 5330.0, spatialReference = SpatialReference.wgs84() ) val camera = Camera( locationPoint = cameraLocation, heading = 355.0, pitch = 72.0, roll = 0.0 ) return ArcGISScene(BasemapStyle.ArcGISImagery).apply { baseSurface = surface initialViewpoint = Viewpoint(cameraLocation, camera) } }
-
Create a MainScreen to hold the scene
-
In MainScreen.kt, create a composable function named
Main
, which will callScreen SceneView
.MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { }
-
Add a
remember
block and callcreate
inside it. AssignScene() remember
to a local variable namedscene
.The top-level composable function
remember
is used to retain state across recompositions.MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { val scene = remember { createScene() } }
-
You will now call several composable functions from Android Jetpack Compose. Call
Scaffold
and pass aTop
with aA p p Bar Text
that contains the app name (R.string.app_
).name MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { val scene = remember { createScene() } Scaffold( topBar = { TopAppBar(title = { Text(text = stringResource(id = R.string.app_name)) }) } ) { } }
-
In the trailing lambda for
Scaffold
, call theSceneView
composable defined in the ArcGIS Maps SDK for Kotlin Toolkit. Pass a Modifier that has maximum size and default padding. And passscene
as thearc
parameterGISScene MainScreen.ktUse dark colors for code blocks @Composable fun MainScreen() { val scene = remember { createScene() } Scaffold( topBar = { TopAppBar(title = { Text(text = stringResource(id = R.string.app_name)) }) } ) { SceneView( modifier = Modifier.fillMaxSize().padding(it), arcGISScene = scene ) } }
Call MainScreen inside MainActivity class
-
Open the app > kotlin+java > com.example.app > MainActivity.kt. Delete all lines of code except for the package declaration (the first line) and the
Main
class definition.Activity MainActivity.ktUse dark colors for code blocks Copy package com.example.app class MainActivity : ComponentActivity() { }
-
Add import statements to MainActivity.kt.
MainActivity.ktUse dark colors for code blocks package com.example.app import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import com.arcgismaps.ApiKey import com.arcgismaps.ArcGISEnvironment import com.example.app.screens.MainScreen import com.example.app.ui.theme.DisplayASceneTheme class MainActivity : ComponentActivity() { }
-
In the
set
block of theContent o
lifecylce function, you will call the composable functionn Create() Main
, with default theming applied. To do this, addScreen o
with the following code.n Create() MainActivity.ktUse dark colors for code blocks class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { DisplayASceneTheme { MainScreen() } } } }
Get an access token
You need an access token to use the location services used in this tutorial.
-
Go to the Create an API key tutorial to obtain an access token.
-
Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service.
-
Copy the access token as it will be used in the next step.
To learn more about other ways to get an access token, go to Types of authentication.
Set an API Key
-
In the
Main
class, create theActivity set
method, where you set theA p i Key() ArcGISEnvironment.apiKey
property by callingApiKey.create()
and passing your access token as a string. Don't forget the double quotes.MainActivity.ktUse dark colors for code blocks class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { DisplayASceneTheme { MainScreen() } } } private fun setApiKey() { ArcGISEnvironment.apiKey = ApiKey.create("YOUR_ACCESS_TOKEN") } }
-
Call
set
in theA p i Key() o
lifecycle method, beforen Create() set
.Content {} MainActivity.ktUse dark colors for code blocks class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setApiKey() setContent { DisplayASceneTheme { MainScreen() } } } private fun setApiKey() { ArcGISEnvironment.apiKey = ApiKey.create("YOUR_ACCESS_TOKEN") } }
Run your app
-
Click Run > Run > app to run the app.
In Android Studio, you have two choices for running your app: an actual Android device or the Android Emulator.
Android device
Connect your computer to your Android device, using USB or Wi-Fi. For more details, see How to connect your Android device.
Android Emulator
Create an AVD (Android Virtual Device) to run in the Android Emulator. For details, see Run apps on the Android Emulator.
Selecting a device
When you build and run an app in Android Studio, you must first select a device. From the Android Studio toolbar, you can access the drop-down list of your currently available devices, both virtual and physical.
.
If you cannot access the list on the toolbar, click Tools > Device Manager.
You should see a scene with the imagery basemap layer centered on the Santa Monica Mountains in California. Pinch, drag, and double-tap the scene view to explore the scene.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: